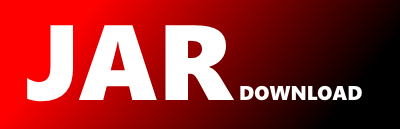
com.alachisoft.ncache.ncactivate.license.NetworkInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
package com.alachisoft.ncache.ncactivate.license;
import com.alachisoft.ncache.runtime.util.RuntimeUtil;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.Inet4Address;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Enumeration;
import java.util.StringTokenizer;
public final class NetworkInfo {
public final static ArrayList getMacAddress() throws IOException {
try {
return getMacAddresses();
} catch (Exception ex) {
//ex.printStackTrace();
throw new IOException("Unable to retrieve MAC address:" + ex.getMessage());
}
}
/*
* Linux stuff
*/
private final static ArrayList linuxParseMacAddress(String ipConfigResponse) throws ParseException {
ArrayList macAddr = new ArrayList(4);
String localHost = null;
try {
localHost = InetAddress.getLocalHost().getHostAddress();
} catch (java.net.UnknownHostException ex) {
ex.printStackTrace();
throw new ParseException(ex.getMessage(), 0);
}
StringTokenizer tokenizer = new StringTokenizer(ipConfigResponse, "\n");
String lastMacAddress = null;
int macCount = 0;
while (tokenizer.hasMoreTokens()) {
// String line = tokenizer.nextToken().trim();
// boolean containsLocalHost = line.indexOf(localHost) >= 0;
//
// // see if line contains IP address
// if(containsLocalHost && lastMacAddress != null)
// {
// return macAddr;
// }
//
// // see if line contains MAC address
// int macAddressPosition = line.indexOf("HWaddr");
// if(macAddressPosition <= 0) continue;
//
// String macAddressCandidate = line.substring(macAddressPosition + 6).trim();
String macAddressCandidate = tokenizer.nextToken().trim();
if (linuxIsMacAddress(macAddressCandidate)) {
lastMacAddress = macAddressCandidate;
macAddr.add(macAddressCandidate);
macCount++;
continue;
}
}
if (macCount > 0) {
return macAddr;
}
ParseException ex = new ParseException("cannot read MAC address for " + localHost + " from [" + ipConfigResponse + "]", 0);
//ex.printStackTrace();
throw ex;
}
private final static boolean linuxIsMacAddress(String macAddressCandidate) {
// TODO: use a smart regular expression
if (macAddressCandidate.length() != 17) {
return false;
}
return true;
}
private final static String linuxIpCommand() throws IOException {
String[] cmd =
{
"/bin/sh",
"-c",
"ip addr | awk '/ether/ {print $2}'"
};
Process p = Runtime.getRuntime().exec(cmd);
InputStream stdoutStream = new BufferedInputStream(p.getInputStream());
StringBuffer buffer = new StringBuffer();
for (; ; ) {
int c = stdoutStream.read();
if (c == -1) {
break;
}
buffer.append((char) c);
}
String outputText = buffer.toString();
stdoutStream.close();
if(outputText.isEmpty())
return linuxRunIfConfigCommand();
return outputText;
}
private final static String linuxRunIfConfigCommand() throws IOException {
String[] cmd =
{
"/bin/sh",
"-c",
"ifconfig -a | grep -o -E '([[:xdigit:]]{1,2}:){5}[[:xdigit:]]{1,2}'"
};
Process p = Runtime.getRuntime().exec(cmd);
InputStream stdoutStream = new BufferedInputStream(p.getInputStream());
StringBuffer buffer = new StringBuffer();
for (; ; ) {
int c = stdoutStream.read();
if (c == -1) {
break;
}
buffer.append((char) c);
}
String outputText = buffer.toString();
stdoutStream.close();
return outputText;
}
/*
* Windows stuff
*/
private final static ArrayList windowsParseMacAddress(String ipConfigResponse) throws ParseException {
ArrayList macAddr = new ArrayList(4);
// String localHost = null;
// try
// {
//
// localHost = InetAddress.getLocalHost().getHostAddress();
// }
// catch(java.net.UnknownHostException ex)
// {
// ex.printStackTrace();
// throw new ParseException(ex.getMessage(), 0);
// }
StringTokenizer tokenizer = new StringTokenizer(ipConfigResponse, "\n");
String lastMacAddress = null;
int macCount = 0; //It should be less than equal to 4
while (tokenizer.hasMoreTokens()) {
String line = tokenizer.nextToken().trim();
// // see if line contains IP address
// for(String ip : ipAddress)
// {
// if(line.contains(ip) && lastMacAddress != null)
// {
// return macAddr;
// }
// }
// see if line contains MAC address
int macAddressPosition = line.indexOf(":");
if (macAddressPosition <= 0) {
continue;
}
String macAddressCandidate = line.substring(macAddressPosition + 1).trim();
if (windowsIsMacAddress(macAddressCandidate)) {
lastMacAddress = macAddressCandidate;
macAddr.add(macAddressCandidate);
macCount++;
if (macCount > 3) //Not more than 4
{
break;
}
//macAddress.add(macAddressCandidate);
continue;
}
}
if (macCount > 0) {
return macAddr;
}
ParseException ex = new ParseException("cannot read MAC address from [" + ipConfigResponse + "]", 0);
//ex.printStackTrace();
throw ex;
}
private final static boolean windowsIsMacAddress(String macAddressCandidate) {
// TODO: use a smart regular expression
if (macAddressCandidate.length() != 17) {
return false;
}
return true;
}
private static ArrayList getMacAddresses() throws SocketException {
String format = "%02X";
ArrayList macs = new ArrayList();
// DHCP Enabled - InterfaceMetric
Enumeration nis = NetworkInterface.getNetworkInterfaces();
while( nis.hasMoreElements() ) {
NetworkInterface ni = nis.nextElement();
byte mac1 [] = ni.getHardwareAddress(); // Physical Address (MAC - Medium Access Control)
if(ni.isLoopback() )
continue;
Enumeration addresses = ni.getInetAddresses();
for (InetAddress address : Collections.list(addresses)) {
if(!(address instanceof Inet4Address)) {
continue;
}
byte mac [] = ni.getHardwareAddress(); // Physical Address (MAC - Medium Access Control)
if( mac != null ) {
final StringBuilder macAddress = new StringBuilder();
for (int i = 0; i < mac.length; i++) {
macAddress.append(String.format("%s"+format, "" , mac[i]) );
}
macs.add( macAddress.toString() );
}
}
}
return macs;
}
private final static String windowsRunIpConfigCommand() throws IOException {
Process p = Runtime.getRuntime().exec("ipconfig /all");
InputStream stdoutStream = new BufferedInputStream(p.getInputStream());
StringBuffer buffer = new StringBuffer();
for (; ; ) {
int c = stdoutStream.read();
if (c == -1) {
break;
}
buffer.append((char) c);
}
String outputText = buffer.toString();
stdoutStream.close();
return outputText;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy