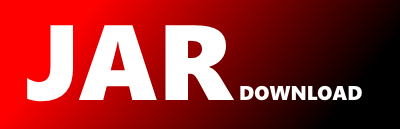
com.alachisoft.ncache.ncactivate.license.nbase64 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* nbase64.java
*
* Created on October 17, 2006, 12:15 AM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*/
package com.alachisoft.ncache.ncactivate.license;
import com.alachisoft.ncache.ncactivate.utils.StringRef;
/**
* @author Administrator
*/
public class nbase64 {
/**
* Creates a new instance of nbase64
*/
public nbase64() {
}
char encode(char uc) {
if (uc < 26) {
return (char) ('A' + uc);
}
if (uc < 52) {
return (char) ('a' + (uc - 26));
}
if (uc < 62) {
return (char) ('0' + (uc - 52));
}
if (uc == 62) {
return '+';
}
return '/';
}
;
char decode(char c) {
if (c >= 'A' && c <= 'Z') {
return (char) (c - 'A');
}
if (c >= 'a' && c <= 'z') {
return (char) (c - 'a' + 26);
}
if (c >= '0' && c <= '9') {
return (char) (c - '0' + 52);
}
if (c == '+') {
return 62;
}
;
return 63;
}
;
boolean is_base64(char c) {
if (c >= 'A' && c <= 'Z') {
return true;
}
if (c >= 'a' && c <= 'z') {
return true;
}
if (c >= '0' && c <= '9') {
return true;
}
if (c == '+') {
return true;
}
;
if (c == '/') {
return true;
}
;
if (c == '=') {
return true;
}
;
return false;
}
;
int decode_len(String str) {
int nlen = str.length();
if (nlen == 0) return 0;
int declen = (nlen >> 2) * 3;
if (str.charAt(nlen - 1) == '=') declen--;
if (str.charAt(nlen - 2) == '=') declen--;
return declen;
}
public String encode(StringRef parr, int ncount) {
String retval = new String();
if (ncount < 1) {
return retval;
}
for (int i = 0; i < ncount; i += 3) {
char by1 = 0, by2 = 0, by3 = 0;
by1 = parr.strData.charAt(i);
if (i + 1 < ncount) {
by2 = parr.strData.charAt(i + 1);
}
;
if (i + 2 < ncount) {
by3 = parr.strData.charAt(i + 2);
}
char by4 = 0, by5 = 0, by6 = 0, by7 = 0;
by4 = (char) (by1 >> 2);
by5 = (char) (((by1 & 0x3) << 4) | (by2 >> 4));
by6 = (char) (((by2 & 0xf) << 2) | (by3 >> 6));
by7 = (char) (by3 & 0x3f);
retval += encode(by4);
retval += encode(by5);
if (i + 1 < ncount) {
retval += encode(by6);
} else {
retval += "=";
}
;
if (i + 2 < ncount) {
retval += encode(by7);
} else {
retval += "=";
}
;
if ((i != 0) && (i % (32 / 4 * 3) == 0)) {
retval += "\r\n";
}
}
;
return retval;
}
;
public int decode(StringRef _str, StringRef parr1) {
String str = new String();
//str.reserve (_str.length ());
for (int j = 0; j < _str.strData.length(); j++) {
if (is_base64(_str.strData.charAt(j))) {
str += _str.strData.charAt(j);
}
}
if (str.length() == 0) {
return 0;
}
if (parr1 == null) return decode_len(str);
char[] parr2 = parr1.strData.toCharArray();
int nlen = str.length();
int j = 0;
for (int i = 0; i < nlen; i += 4) {
char c1 = 'A', c2 = 'A', c3 = 'A', c4 = 'A';
c1 = str.charAt(i);
if (i + 1 < str.length()) {
c2 = str.charAt(i + 1);
}
;
if (i + 2 < str.length()) {
c3 = str.charAt(i + 2);
}
;
if (i + 3 < str.length()) {
c4 = str.charAt(i + 3);
}
;
char by1 = 0, by2 = 0, by3 = 0, by4 = 0;
by1 = decode(c1);
by2 = decode(c2);
by3 = decode(c3);
by4 = decode(c4);
parr2[j++] = (char) ((by1 << 2) | (by2 >> 4));
if (c3 != '=') {
parr2[j++] = (char) (((by2 & 0xf) << 4) | (by3 >> 2));
}
if (c4 != '=') {
parr2[j++] = (char) (((by3 & 0x3) << 6) | by4);
}
;
}
;
parr1.strData = new String(parr2);
return j;
}
;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy