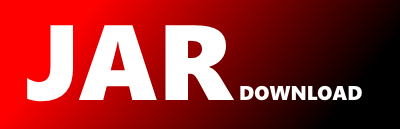
com.alachisoft.ncache.ncactivate.nwizard.NCWizardController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
package com.alachisoft.ncache.ncactivate.nwizard;
import java.awt.event.ActionListener;
/**
* This class is responsible for reacting to events generated by pushing any of the
* three buttons, 'Next', 'Previous', and 'Cancel.' Based on what button is pressed,
* the controller will update the model to show a new panel and reset the state of
* the buttons as necessary.
*/
public class NCWizardController implements ActionListener {
private NCWizard wizard;
/**
* This constructor accepts a reference to the Wizard component that created it,
* which it uses to update the button components and access the WizardModel.
*
* @param w A callback to the Wizard component that created this controller.
*/
public NCWizardController(NCWizard w) {
wizard = w;
}
/**
* Calling method for the action listener interface. This class listens for actions
* performed by the buttons in the Wizard class, and calls methods below to determine
* the correct course of action.
*
* @param evt The ActionEvent that occurred.
*/
public void actionPerformed(java.awt.event.ActionEvent evt) {
if (evt.getActionCommand().equals(NCWizard.CANCEL_BUTTON_ACTION_COMMAND))
cancelButtonPressed();
else if (evt.getActionCommand().equals(NCWizard.BACK_BUTTON_ACTION_COMMAND))
backButtonPressed();
else if (evt.getActionCommand().equals(NCWizard.NEXT_BUTTON_ACTION_COMMAND))
nextButtonPressed();
}
private void cancelButtonPressed() {
wizard.close(NCWizard.CANCEL_RETURN_CODE);
}
private void nextButtonPressed() {
NCWizardModel model = wizard.getModel();
NCWizardPanelDescriptor descriptor = model.getCurrentPanelDescriptor();
// If it is a finishable panel, close down the dialog. Otherwise,
// get the ID that the current panel identifies as the next panel,
// and display it.
Object nextPanelDescriptor = descriptor.getNextPanelDescriptor();
if (nextPanelDescriptor instanceof NCWizardPanelDescriptor.FinishIdentifier) {
if (descriptor.aboutToExitPanel() == true)
wizard.close(NCWizard.FINISH_RETURN_CODE);
else
return;
} else {
wizard.setCurrentPanel(nextPanelDescriptor);
}
}
private void backButtonPressed() {
NCWizardModel model = wizard.getModel();
NCWizardPanelDescriptor descriptor = model.getCurrentPanelDescriptor();
// Get the descriptor that the current panel identifies as the previous
// panel, and display it.
Object backPanelDescriptor = descriptor.getBackPanelDescriptor();
wizard.setCurrentPanel(backPanelDescriptor);
}
void resetButtonsToPanelRules() {
// Reset the buttons to support the original panel rules,
// including whether the next or back buttons are enabled or
// disabled, or if the panel is finishable.
NCWizardModel model = wizard.getModel();
NCWizardPanelDescriptor descriptor = model.getCurrentPanelDescriptor();
model.setCancelButtonText(NCWizard.CANCEL_TEXT);
// If the panel in question has another panel behind it, enable
// the back button. Otherwise, disable it.
model.setBackButtonText(NCWizard.BACK_TEXT);
if (descriptor.getBackPanelDescriptor() != null)
model.setBackButtonEnabled(Boolean.TRUE);
else
model.setBackButtonEnabled(Boolean.FALSE);
// If the panel in question has one or more panels in front of it,
// enable the next button. Otherwise, disable it.
if (descriptor.getNextPanelDescriptor() != null)
model.setNextFinishButtonEnabled(Boolean.TRUE);
else
model.setNextFinishButtonEnabled(Boolean.FALSE);
// If the panel in question is the last panel in the series, change
// the Next button to Finish. Otherwise, set the text back to Next.
if (descriptor.getNextPanelDescriptor() instanceof NCWizardPanelDescriptor.FinishIdentifier) {
model.setNextFinishButtonText(NCWizard.FINISH_TEXT);
} else {
model.setNextFinishButtonText(NCWizard.NEXT_TEXT);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy