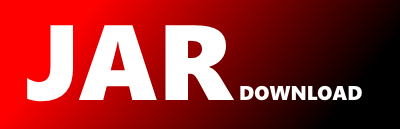
com.alachisoft.ncache.ncactivate.utils.AppUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* AppUtil.java
*
* Created on October 7, 2006, 3:50 PM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*/
package com.alachisoft.ncache.ncactivate.utils;
import com.alachisoft.ncache.licensing.config.InfoType;
import com.alachisoft.ncache.ncactivate.appdata.MachineInfo;
import com.alachisoft.ncache.ncactivate.appdata.ProductInfo;
import com.alachisoft.ncache.ncactivate.appdata.UserInf;
import com.alachisoft.ncache.ncactivate.cmdline.exceptions.InvalidKeyException;
import com.alachisoft.ncache.ncactivate.crypto.Crypto;
import com.alachisoft.ncache.ncactivate.license.ActivationStatus;
import com.alachisoft.ncache.ncactivate.license.NCCpuInfo;
import com.alachisoft.ncache.ncactivate.license.StubDll;
import com.alachisoft.ncache.runtime.exceptions.LicensingException;
import com.alachisoft.ncache.runtime.exceptions.LocalVerificationFailedException;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Properties;
import java.util.Random;
/**
* @author Administrator
*/
public class AppUtil {
public static boolean isCloudEdition;
public static char[][] szKeyEvals =
{
// Extention Keys for NCache 5.0
// Ext 1: 524QTUSLYPXXACY5O
{0xa6, 0xa1, 0xa7, 0xc2, 0xc7, 0xc6, 0xc0, 0xdf, 0xca, 0xc3, 0xcb, 0xcb, 0xd2, 0xd0, 0xca, 0xa6, 0xdc},
// Ext 2: E38MDGZDOIXC8MV22
{0xd6, 0xa0, 0xab, 0xde, 0xd7, 0xd4, 0xc9, 0xd7, 0xdc, 0xda, 0xcb, 0xd0, 0xab, 0xde, 0xc5, 0xa1, 0xa1},
//Ext 3: IW7ZJ6XTAWRX7UKLS
{0xda, 0xc4, 0xa4, 0xc9, 0xd9, 0xa5, 0xcb, 0xc7, 0xd2, 0xc4, 0xc1, 0xcb, 0xa4, 0xc6, 0xd8, 0xdf, 0xc0},
// Ext 4: PZZL2SF2JO73WY8QX
{0xc3, 0xc9, 0xc9, 0xdf, 0xa1, 0xc0, 0xd5, 0xa1, 0xd9, 0xdc, 0xa4, 0xa0, 0xc4, 0xca, 0xab, 0xc2, 0xcb}
};
static eActivationType g_actType;
// Data section
static eDeActivationType g_deActType;
static eLicenseType g_licType;
static ActivationData info = new ActivationData();
;
private static int editionId = -1;
;
private static String version = null;
;
private static boolean _isReactivation = false;
;
/**
* Creates a new instance of AppUtil
*/
public AppUtil() {
}
public static ActivationData getActivationData() {
//static ActivationData info = new ActivationData();
return info;
}
public static ProductInfo getProductInfo() {
return getActivationData().Product;
}
public static boolean GetReactivate() {
return _isReactivation;
}
public static VerifyResult VerifAuthKey(String szAuthKey) throws LicensingException, LocalVerificationFailedException {
int curPos = 0;
String szAuthText = Crypto.Decode(szAuthKey);
String scheme, machineName, numCpus, physicalCpus, editionID, expiryDate, gracePeriod, activationID,
version, mac1 = "", mac2 = "", mac3 = "", mac4 = "";
String[] tokens = szAuthText.split(":");
int nTokens = tokens.length;
scheme = tokens[0].toLowerCase();
machineName = tokens[1].toLowerCase();
numCpus = tokens[2].toLowerCase();
physicalCpus = tokens[3].toLowerCase();
version = tokens[4].toLowerCase();
editionID = tokens[5].toLowerCase();
expiryDate = tokens[6].toLowerCase();
gracePeriod = tokens[7].toLowerCase();
activationID = tokens[8].toLowerCase();
if (NCCpuInfo.getPhysicalCoreCount() > Integer.parseInt(numCpus) && NCCpuInfo.getLicenseCount() > Integer.parseInt(physicalCpus)) {
_isReactivation = true;
} else
_isReactivation = false;
if (nTokens >= 10) {
mac1 = tokens[9].toLowerCase();
}
if (nTokens >= 11) {
mac2 = tokens[10].toLowerCase();
}
if (nTokens >= 12) {
mac3 = tokens[11].toLowerCase();
}
if (nTokens >= 13) {
mac4 = tokens[12].toLowerCase();
}
// Fetch licensing scheme!
if (scheme == "") {
return VerifyResult.ErrKey;
}
eLicenseType licType = (scheme.charAt(0) == (char) 'c') ? eLicenseType.Production : eLicenseType.Developer;
// Check for machine name!
if (machineName.compareToIgnoreCase(EnvironmentUtil.getComputerName()) != 0) {
return VerifyResult.ErrMac;
}
if (version.compareToIgnoreCase(getProductInfo().Version) != 0) {
return VerifyResult.ErrVersion;
}
// Check if the machine addresses are the same!
try {
ArrayList adapters = EnvironmentUtil.GetAdaptersInfo();
if (EnvironmentUtil.GetAdapter(adapters, mac1) != -1) {
return VerifyResult.Success;
}
if (EnvironmentUtil.GetAdapter(adapters, mac2) != -1) {
return VerifyResult.Success;
}
if (EnvironmentUtil.GetAdapter(adapters, mac3) != -1) {
return VerifyResult.Success;
}
if (EnvironmentUtil.GetAdapter(adapters, mac4) != -1) {
return VerifyResult.Success;
}
} catch (IOException ioe) {
}
return VerifyResult.ErrMac;
}
//////////////////////////////////////////////
//
// Application specific functions.
//
public static String getActivationID(String szAuthKey) {
try {
if (szAuthKey == "")
return "";
if (VerifAuthKey(szAuthKey) != VerifyResult.Success)
return "";
String szAuthText = Crypto.Decode(szAuthKey);
String[] tokens = szAuthText.split(":");
if (tokens.length >= 9)
return tokens[8];
return "";
} catch (Exception ex) {
return "";
}
}
public static int getEditionIDFromServerValue(String installCodeFromServer) {
if (editionId == -1) {
version = installCodeFromServer;
String[] tokens = version.split("-");
if (tokens != null && tokens.length > 3) {
String edition = tokens[1];
edition = edition.toLowerCase();
if (edition.equals("cnto")) {
editionId = 71; //37;//29;
} else if (edition.equals("ento")) {
editionId = 70; //NCache Server
} else if (edition.equals("dev")) {
editionId = 19;
}
}
}
return editionId;
}
public static int getEditionID(String code) {
if (editionId == -1) {
version = code == null ? getVersion() : getVersion(code);
String[] tokens = version.split("-");
if (tokens != null && tokens.length > 3) {
String edition = tokens[1];
edition = edition.toLowerCase();
if (edition.equals("cnto")) {
editionId = 71; //37;//29;
} else if (edition.equals("ento")) {
editionId = 70; //NCache Server
} else if (edition.equals("dev")) {
editionId = 19;
}
}
}
return editionId;
}
public static int getEditionID() {
return getEditionID(null);
}
public static String getVersion() {
if (version == null) {
return getVersion(RegUtil.getInstallCode());
}
return version;
}
public static String getVersion(String installCode) {
if (version == null) {
try {
try {
installCode = Crypto.Decode(installCode);
} catch (Exception ex) {
throw new RuntimeException("Unable to decode the installcode found in license.properties file.");
}
if (installCode.contains(":")) {
version = installCode.substring(0, installCode.indexOf(":"));
} else if (installCode.contains("JVC-EXP")) {
version = installCode;
}
} catch (Exception e) {
}
}
if (version == null) {
throw new RuntimeException("Unable to detect the product version.");
}
return version;
}
public eDeActivationType getDeActivationType() {
return g_deActType;
}
public void setDeActivationType(eDeActivationType type) {
g_deActType = type;
}
public eActivationType getAppActivationType() {
return g_actType;
}
public void setAppActivationType(eActivationType type) {
g_actType = type;
}
public eLicenseType getLicenseType() {
return g_licType;
}
public void setLicenseType(eLicenseType type) {
g_licType = type;
}
//////////////////////////////////////////////////////////////////////
//
// Verifies if the given activation code is valid for this machine or not.
//
public UserInf getUserInfo() {
return getActivationData().User;
}
public MachineInfo getMachineInfo() {
return getActivationData().Machine;
}
public final boolean ValidateEvalKey(String key) throws InvalidKeyException {
AppUtil appUtil = new AppUtil();
if (key.length() != 17) {
throw new InvalidKeyException("The specified key is not valid. Please supply a valid extension key.");
}
int matchPeriod = appUtil.getExtKeyIndex(key.toCharArray());
if (matchPeriod < appUtil.getExtensionsUsed()) {
throw new InvalidKeyException("The specified key is not valid. Please supply a valid extension key.");
}
Random rand = new Random();
String period = String.format("%d:%d:xtension", matchPeriod + 1, rand.nextInt());
RegUtil.setKey(InfoType.LICENSE, RegKeys.ExtCode, Crypto.Encode(period));
System.out.println("NCache evaluation period has been extended.");
RegUtil.StoreRegistry();
return true;
}
public Properties loadResourceBundle(String bundleName) {
Properties props = null;
try {
InputStream in = AppUtil.class.getClassLoader().getResourceAsStream(bundleName);
props = new Properties();
props.load(in);
} catch (IOException iOException) {
System.err.println(iOException.toString());
}
return props;
}
public boolean isPerpetual(String szAuthKey) {
if (szAuthKey == "")
return false;
String szAuthText = Crypto.Decode(szAuthKey);
String[] tokens = szAuthText.split(":");
if (tokens.length >= 7 && tokens[6] == "null")
return true;
return false;
}
public String generateMachineInfoCode() {
if (!getProductInfo().Reactivation)
getProductInfo().ActId = EnvironmentUtil.NewGUID();
String xml = getActivationData().ToLinearText();
return Crypto.Encode(xml);
}
public int Activate(String szAuthKey) {
RegUtil.setKey(InfoType.LICENSE, RegKeys.DeactCode, "");
RegUtil.setKey(InfoType.LICENSE, RegKeys.AuthCode, szAuthKey);
getActivationData().Save();
RegUtil.StoreRegistry();
try {
return StubDll.InitializeStub(this.getProductInfo().VersionId, 0, 1, ActivationStatus.Activated);
} catch (Exception exception) {
return 1;
}
}
public int DeactKey(String szDeactKey) {
RegUtil.setKey(InfoType.LICENSE, RegKeys.DeactCode, szDeactKey);
RegUtil.setKey(InfoType.LICENSE, RegKeys.AuthCode, "");
RegUtil.StoreRegistry();
return 0;
}
public void DeactStub() throws Exception {
try {
int result = StubDll.InitializeStub(this.getProductInfo().VersionId, 0, 1, ActivationStatus.Deactivated);
if (result < 0)
throw new LicensingException();
} catch (Exception licensingException) {
throw new Exception("Failed to Deactivate, Please make sure to execute with administrative rights.");
}
}
public boolean isCloudEdition() {
getEditionID();
return isCloudEdition;
}
//////////////////////////////////////////////////////////////////////
//
// Returns the number of times the evaluation period has been extended so far.
//
public int getExtensionsUsed() {
int ext = -1;
if (ext == -1) {
String extCode = RegUtil.getKey(InfoType.LICENSE, RegKeys.ExtCode);
extCode = Crypto.Decode(extCode);
ext = 0;
if (extCode.length() > 3) {
if (extCode.charAt(0) >= '0' && extCode.charAt(0) <= '9') {
ext = extCode.charAt(0) - '0';
}
}
}
return ext;
}
public int getMaxExtensions() {
int arrLength = szKeyEvals.length;
char[] key = szKeyEvals[0];
int nKeylen = key.length;
int nMaxExt = arrLength / nKeylen;
return 4;
}
public int getExtKeyIndex(char[] szKey) {
for (int i = 0; i < getMaxExtensions(); i++) {
if (XorCmp(szKeyEvals[i], szKey)) {
return i;
}
}
return -1;
}
public boolean XorCmp(char[] szUserKey, char[] szEmbededKey) {
for (int i = 0; i < 17; i++) {
char ch = (char) (szUserKey[i] ^ (char) 147);
char vch = szEmbededKey[i];
if (ch != vch) {
return false;
}
}
return true;
}
public static enum eActivationType {
Online, Email, Manual
}
public static enum eDeActivationType {
Online, Email, Manual
}
public static enum eLicenseType {
Production, Developer
}
//public static enum VerifyResult {Success=-2};
//ErrLicScheme(-1), ErrNumCpu(-2), ErrMac(-3), ErrVersion(-4), ErrKey(-5), ErrPhysicalCpus(-6)};
public static enum VerifyResult {
Success, ErrLicScheme, ErrNumCpu, ErrMac, ErrVersion, ErrKey, ErrPhysicalCpus
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy