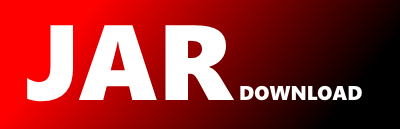
com.alachisoft.ncache.security.CacheXMLAuthorizationProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-security Show documentation
Show all versions of nc-security Show documentation
Internal package of Alachisoft.
package com.alachisoft.ncache.security;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import java.io.File;
/**
* Summary description for CacheXMLAuthorizationProvider.
*/
public class CacheXMLAuthorizationProvider implements IAuthorizationProvider {
public static java.util.HashMap securityMap = null;
public static boolean securityEnabled = false;
public static String ldapPath = "";
public static String ldapPort = "";
public CacheXMLAuthorizationProvider() {
//
// TODO: Add constructor logic here
//
}
public static String parsedAdminUser(String userName) {
String userNames = "";
String[] splitUser = userName.split(",");
String[] splits = new String[splitUser.length];
for (String str : splitUser) {
splits = str.split("=");
if (splits[0].contains("cn")) {
userNames = splits[1].toString();
break;
}
}
return userNames;
}
public static String GetUserFullDn(String userName) {
String userNames = "";
java.util.ArrayList users = (java.util.ArrayList) securityMap.get("administrators");
if (users != null) {
for (int i = 0; i < users.size(); i++) {
if (parsedAdminUser(users.get(i).toString().toLowerCase()).trim().equalsIgnoreCase(userName.trim())) {
userNames = users.get(i).toString().toLowerCase();
}
}
return userNames;
}
return userNames;
}
/**
* Loads security xml from the given file
*/
public static void LoadSecurity() {
try {
String filePath = SecurityConfiguration.getConfigurationPath();
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
Document document = null;
//XmlDocument document = new XmlDocument();
DocumentBuilder db = dbf.newDocumentBuilder();
File file = new File(filePath);
if (!(file.exists())) {
return;
}
document = db.parse(file);
//document.Load(fileName);
//Element docEle = document.getDocumentElement();
// NodeList cacheConfigList = docEle.getElementsByTagName("cache-security");
boolean done = false;
String enabled = "";
//if (cacheConfigList != null && cacheConfigList.getLength() > 0) {
//for (int nodeItem = 0; nodeItem < cacheConfigList.getLength(); nodeItem++) {
//Node cacheNode = cacheConfigList.item(nodeItem);
//if (cacheNode.getNodeType() == Node.ELEMENT_NODE) {
//Element cacheElement = (Element) cacheNode;
NodeList enable = document.getElementsByTagName("enabled");
NodeList ldap = document.getElementsByTagName("ldap");
NodeList port = document.getElementsByTagName("port");
if (enable != null) {
Element enableElement = (Element) enable.item(0);
enabled = enableElement.getFirstChild().getNodeValue().toLowerCase();
}
if (ldap != null) {
Element ldapElement = (Element) ldap.item(0);
ldapPath = ldapElement.getFirstChild().getNodeValue();
}
if (port != null) {
Element portElement = (Element) port.item(0);
ldapPort = portElement.getFirstChild().getNodeValue();
}
securityEnabled = Boolean.parseBoolean(enabled);
//}
// }
//}
if (securityEnabled == true) {
securityMap = LoadSecurityXML(document);
}
} catch (java.lang.Exception e) {
securityEnabled = false;
}
}
/**
* @return Reads security xml and returns True if security is enabled Else returns
* False;
*/
public static boolean isSecurityEnabled() {
try {
String filePath = SecurityConfiguration.getConfigurationPath();
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
Document document = null;
//XmlDocument document = new XmlDocument();
DocumentBuilder db = dbf.newDocumentBuilder();
File file = new File(filePath);
if (!(file.exists())) {
return false;
}
document = db.parse(file);
//document.Load(fileName);
// Element docEle = document.getDocumentElement();
//NodeList cacheConfigList = docEle.getElementsByTagName("cache-security");
boolean done = false;
String enabled = "";
//if (cacheConfigList != null && cacheConfigList.getLength() > 0) {
//for (int nodeItem = 0; nodeItem < cacheConfigList.getLength(); nodeItem++) {
//Node cacheNode = cacheConfigList.item(nodeItem);
//if (cacheNode.getNodeType() == Node.ELEMENT_NODE) {
// Element cacheElement = (Element) cacheNode;
NodeList enable = document.getElementsByTagName("enabled");
NodeList ldap = document.getElementsByTagName("ldap");
NodeList port = document.getElementsByTagName("port");
if (enable != null) {
Element enableElement = (Element) enable.item(0);
enabled = enableElement.getFirstChild().getNodeValue().toLowerCase();
}
if (ldap != null) {
Element ldapElement = (Element) ldap.item(0);
ldapPath = ldapElement.getFirstChild().getNodeValue();
}
if (port != null) {
Element portElement = (Element) port.item(0);
ldapPort = portElement.getFirstChild().getNodeValue();
}
securityEnabled = Boolean.parseBoolean(enabled);
//}
//}
//}
return securityEnabled;
} catch (java.lang.Exception e) {
securityEnabled = false;
return (securityEnabled);
}
}
/**
* Loads security xml from the given file
*
* @return HashMap of Security Information
*/
public static java.util.HashMap getSecurityMap() {
try {
String filePath = SecurityConfiguration.getConfigurationPath();
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
Document document = null;
//XmlDocument document = new XmlDocument();
DocumentBuilder db = dbf.newDocumentBuilder();
File file = new File(filePath);
if (!(file.exists())) {
return null;
}
document = db.parse(file);
securityMap = LoadSecurityXML(document);
return securityMap;
} catch (java.lang.Exception e) {
//throw new Exception("Invalid security configuration file");
return null;
}
}
/**
* Loads settings from specified xml document.
*/
private static java.util.HashMap LoadSecurityXML(Document document) {
java.util.HashMap cacheSecurity = new java.util.HashMap();
NodeList nodeSec = document.getElementsByTagName("administrators");
// NodeList apiSec = document.getElementsByTagName("users");
NodeList enabled = document.getElementsByTagName("enabled");
NodeList ldap = document.getElementsByTagName("ldap");
NodeList port = document.getElementsByTagName("port");
if (enabled.getLength() > 0) {
Element enableElement = (Element) enabled.item(0);
cacheSecurity.put("enabled", enableElement.getFirstChild().getNodeValue());
}
if (ldap.getLength() > 0) {
Element ldapElement = (Element) ldap.item(0);
cacheSecurity.put("ldap", ldapElement.getFirstChild().getNodeValue());
}
if (port.getLength() > 0) {
Element portElement = (Element) port.item(0);
cacheSecurity.put("port", portElement.getFirstChild().getNodeValue());
}
//as we know there is always going to be single element for these two node.
java.util.ArrayList cacheAdministrators = LoadNCacheAdministrators(nodeSec.item(0));
cacheSecurity.put("administrators", cacheAdministrators);
// java.util.HashMap cacheUsers = LoadNCacheUsers(apiSec.item(0));
// cacheSecurity.put("users", cacheUsers);
return cacheSecurity;
}
/**
* Loads settings from specified xml document.
*/
private static java.util.ArrayList LoadNCacheAdministrators(Node node) {
java.util.ArrayList uids = new java.util.ArrayList();
if (node != null) {
NodeList uidList = ((Element) node).getElementsByTagName("uid");
if (uidList != null && uidList.getLength() > 0) {
for (int usersItem = 0; usersItem < uidList.getLength(); usersItem++) {
Node userNode = uidList.item(usersItem);
if (userNode.getNodeType() == Node.ELEMENT_NODE) {
Element userElement = (Element) userNode;
uids.add(userElement.getFirstChild().getNodeValue());
}
}
}
}
return uids;
}
/**
* Loads settings from specified xml document.
*/
private static java.util.HashMap LoadNCacheUsers(Node node) {
if (node == null) {
return null;
}
NodeList uidList = ((Element) node).getElementsByTagName("cache");
java.util.HashMap caches = new java.util.HashMap();
if (uidList != null && uidList.getLength() > 0) {
for (int usersItem = 0; usersItem < uidList.getLength(); usersItem++) {
Node curNode = uidList.item(usersItem);
if (curNode.getNodeType() == Node.ELEMENT_NODE) {
Element cacheId = (Element) curNode;
if (cacheId.hasAttribute("id")) {
String cacheName = cacheId.getAttribute("id").toLowerCase();
java.util.ArrayList cacheData = LoadUsers(cacheId);
caches.put(cacheName, cacheData);
}
}
}
}
return caches;
}
/**
* Loads settings from specified xml document.
*/
private static java.util.ArrayList LoadUsers(Element credentials) {
java.util.ArrayList uids = new java.util.ArrayList();
if (credentials != null) {
NodeList uidList = credentials.getElementsByTagName("uid");
if (uidList != null && uidList.getLength() > 0) {
for (int usersItem = 0; usersItem < uidList.getLength(); usersItem++) {
Node userNode = uidList.item(usersItem);
if (userNode.getNodeType() == Node.ELEMENT_NODE) {
Element userElement = (Element) userNode;
uids.add(userElement.getFirstChild().getNodeValue());
}
}
}
}
return uids;
}
/**
* Evaluates the specified authority against the specified context.
*
* @param ruleName Must be a string that is the name of the rule to
* evaluate.
* @return true if the expression evaluates to true, otherwise
* false .
*/
@Override
public final boolean Authorize(String ruleName) {
// if (principal == null) {
// throw new IllegalArgumentException("principal");
// }
if (ruleName == null || ruleName.length() == 0) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: ruleName");
}
// IIdentity userIdentity = principal.Identity;
return true;
}
/**
* Evaluates the specified authority against the specified context.
*
* @param userName
* @param context Name of the rule to evaluate.
* @return True if the expression evaluates to true,
* otherwise false.
*/
@Override
public final boolean AuthorizeNode(String userName) {
boolean isAuthorize = false;
if (securityEnabled == false) {
return true;
}
java.util.ArrayList users = (java.util.ArrayList) securityMap.get("administrators");
if (users != null) {
for (int i = 0; i < users.size(); i++) {
isAuthorize = parsedAdminUser(users.get(i).toString().toLowerCase()).trim().equalsIgnoreCase(userName.trim());
if (isAuthorize) {
break;
}
}
}
return isAuthorize;
}
/**
* Evaluates the specified authority against the specified context.
*
* @param userId
* @param cacheId
* @param context Name of the rule to evaluate.
* @return True if the expression evaluates to true,
* otherwise false.
*/
@Override
public final boolean AuthorizeAPI(String userId, OperationCode context, String cacheId) {
if (securityEnabled == false) {
return true;
}
// java.util.HashMap caches = (java.util.HashMap) securityMap.get("users");
// java.util.ArrayList users = (java.util.ArrayList) caches.get(cacheId.toLowerCase());
java.util.ArrayList superUsers = (java.util.ArrayList) securityMap.get("administrators");
// CacheIdentity cIdentity = (CacheIdentity) principal.Identity;
// String userId = cIdentity.getName().toLowerCase();
// if (users != null) {
// if (users.contains(userId)) {
// return true;
// }
// }
if (superUsers != null) {
if (superUsers.contains(userId)) {
return true;
}
}
return false;
}
/**
* Loads settings from specified xml document.
*/
// private static HashMap LoadNodeCredentials(XmlNodeList crdentials)
// {
// HashMap credentialHash = new HashMap ();
// StringCollection uids = new StringCollection();
// string key = "";
// foreach(XmlElement credential in crdentials)
// {
// XmlNodeList uidList = credential.GetElementsByTagName("uid");
// String uid = uidList[0].InnerText.ToLower();
// uids.Add(uid);
// key = credential.Name;
// }
// credentialHash.Add(key,uids);
//
// return credentialHash;
//
// }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy