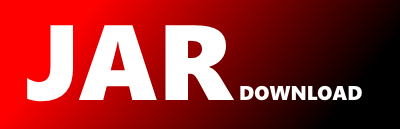
com.alachisoft.ncache.security.encryption.AESEncryption Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-security Show documentation
Show all versions of nc-security Show documentation
Internal package of Alachisoft.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.security.encryption;
import com.alachisoft.ncache.runtime.encryption.Encryption;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.util.HashMap;
/**
* @author muhammad_sami
*/
public class AESEncryption implements Encryption {
private static String s_iv = "ZCBMTYIPQEFGJHAL";
private static String algorithm = "AES";
private static String transformation = "AES/CBC/PKCS5Padding";
public static byte[] ConvertStringToByteArray(String s) {
try {
return s.getBytes();//s.getBytes("ASCII");
} catch (Exception ex) {
return null;
}
}
public static String ConvertBytesToString(byte[] bytes) {
try {
return new String(bytes, "UTF-8");
} catch (Throwable ex) {
return null;
}
}
@Override
public void Intialize(HashMap parameters) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public byte[] Encrypt(byte[] data, String key) {
try {
byte[] k = ConvertStringToByteArray(key);
byte[] IV = ConvertStringToByteArray(s_iv);
SecretKeySpec securityKey = new SecretKeySpec(k, algorithm);
Cipher encrypter = Cipher.getInstance(transformation);
encrypter.init(Cipher.ENCRYPT_MODE, securityKey, new IvParameterSpec(IV));
return encrypter.doFinal(data);
} catch (Exception e) {
return data;
}
}
@Override
public byte[] Decrypt(byte[] data, String key) {
try {
byte[] k = ConvertStringToByteArray(key);
byte[] IV = ConvertStringToByteArray(s_iv);
SecretKeySpec securityKey = new SecretKeySpec(k, algorithm);
Cipher encrypter = Cipher.getInstance(transformation);
encrypter.init(Cipher.DECRYPT_MODE, securityKey, new IvParameterSpec(IV));
byte[] returner = encrypter.doFinal(data);
return returner;
} catch (Exception e) {
return data;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy