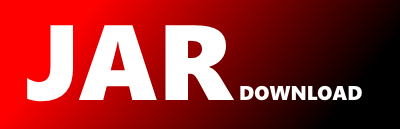
com.alachisoft.ncache.security.encryption.EncryptionMgr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-security Show documentation
Show all versions of nc-security Show documentation
Internal package of Alachisoft.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.security.encryption;
/**
* @author Basit Anwer
*/
import com.alachisoft.ncache.runtime.encryption.Encryption;
//using System.IO;
//using System.Security.Cryptography;
public class EncryptionMgr implements Alachisoft.NCache.Common.IDisposable {
//private static System.Security.Cryptography.TripleDESCryptoServiceProvider s_des;
private static java.util.HashMap _encryptionManagers = new java.util.HashMap();
private String _encryptionKey = null;
private Encryption _encryptionProvider = null;
private boolean _enabled = false;
//static string _key = "A41'D3a##asd[1-a;d zs[s`";
//private static string s_iv = "qwertyuiopasdfghjklmnbvc";
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public static byte[] Encryption(byte[] data, string cacheName)
public static byte[] Encryption(byte[] data, String cacheName) {
EncryptionMgr mgr = GetEncryptionMgr(cacheName);
if (mgr != null && mgr._enabled) {
return mgr._encryptionProvider.Encrypt(data, mgr._encryptionKey);
}
return data;
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public static byte[] Decrypt(byte[] data, string cacheName)
public static byte[] Decrypt(byte[] data, String cacheName) {
EncryptionMgr mgr = GetEncryptionMgr(cacheName);
if (mgr != null && mgr._enabled) {
return mgr._encryptionProvider.Decrypt(data, mgr._encryptionKey);
}
return data;
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
public static void InitializeEncryption(boolean enabled, String key, String cacheName, String provider) throws Exception {
_encryptionManagers.put(cacheName, CreateObject(enabled, key, cacheName, provider));
}
private static EncryptionMgr CreateObject(boolean enabled, String key, String cacheName, String provider) throws Exception {
EncryptionMgr encryptionMgr = new EncryptionMgr();
encryptionMgr._enabled = enabled;
if (encryptionMgr._enabled) {
if (provider == null || provider.isEmpty()) {
throw new Exception("Encryption provider is Empty or Null");
}
encryptionMgr._encryptionKey = Alachisoft.NCache.Common.EncryptionUtil.DecryptKey(key);
if (provider.contains("AES")) {
boolean validKeyLength = true;
if (provider.equals("AES 128")) {
validKeyLength = encryptionMgr._encryptionKey.length() == 16;
} else if (provider.equals("AES 192")) {
validKeyLength = encryptionMgr._encryptionKey.length() == 24;
} else if (provider.equals("AES 256")) {
validKeyLength = encryptionMgr._encryptionKey.length() == 32;
} else {
throw new Exception("Invalid AES ecnryption provider is specified");
}
if (!validKeyLength) {
throw new Exception("Invalid key length for " + provider + " provider");
} else {
encryptionMgr._encryptionProvider = new AESEncryption();
}
} else if (provider.contains("DES")) {
boolean validKeyLength = true;
if (provider.equals("3DES 128")) {
validKeyLength = encryptionMgr._encryptionKey.length() == 16;
} else if (provider.equals("3DES 192")) {
validKeyLength = encryptionMgr._encryptionKey.length() == 24;
} else {
throw new Exception("Invalid 3DES ecnryption provider is specified");
}
if (!validKeyLength) {
throw new Exception("Invalid key length for " + provider + " provider");
} else {
encryptionMgr._encryptionProvider = new TripleDESEncryption();
}
} else {
throw new Exception("Invalid encryption provider is specified");
}
}
return encryptionMgr;
}
private static EncryptionMgr GetEncryptionMgr(String cacheName) {
if (_encryptionManagers.containsKey(cacheName)) {
return _encryptionManagers.get(cacheName);
} else {
return null;
}
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region IDisposable Members
@Override
public final void dispose() {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy