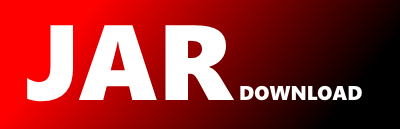
Alachisoft.NCache.Common.Common Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package Alachisoft.NCache.Common;
import com.alachisoft.ncache.ncactivate.utils.RegUtil;
import java.io.File;
/**
* @author Muneeb Shahid
*/
public class Common {
private final static Boolean debug = false;
/**
* java alterative to c# "as"
*
* @param obj
* @param cls
* @return
*/
public static Object readAs(Object obj, java.lang.Class cls) {
return (cls.isInstance(obj) ? obj : null);
}
/**
* java alternative to C# "as" removes the hassle of casting each time at
* users end in contrast to readAs(Object obj, java.lang.Class cls)
*
* @param
* @param
* @param obj
* @param type
* @return
*/
public static V as(K obj, Class type) {
return type.isInstance(obj) ? type.cast(obj) : null;
}
public static boolean is(Object obj, java.lang.Class cls) {
return cls.isInstance(obj);
}
/**
* Rounds off fraction to the given amount of precision. Faster than
* DecimalFormat
*
* @param fraction
* @param precision
* @return
*/
public static double roundOff(double fraction, int precision) {
int i = 0;
int finalNum = 1;
while (i++ < precision) {
finalNum *= 10;
}
return (double) Math.round(fraction * finalNum) / finalNum;
}
/**
* [Combine MultiplePaths] java alternative (OS independent) to
* System.IO.Path.Combine().
*
* @param parentDir
* @param childDirs
* @return
*/
public static String combinePath(String parentDir, String... childDirs) {
return combinePath(new java.io.File(parentDir), childDirs);
}
/**
* [Combine MultiplePaths] java alternative (OS independent) to
* System.IO.Path.Combine().
*
* @param parentDir
* @param childDirs
* @return
*/
public static String combinePath(java.io.File parentDir, String... childDirs) {
java.io.File path = parentDir;
for (String childDir : childDirs) {
path = new java.io.File(path, childDir);
}
return path.getPath();
}
/**
* Generates a random number in the provided range.
*
* @param max
* @param min
* @return
*/
public static int generateRandomNoInRange(int max, int min) {
return min + (int) Math.random() * ((max - min) + 1);
}
/**
* Equivalent to .Net String.isNullorEmpty()
*
* @param value String value to compare
* @return true if string is either null or length == 0 , otherwise false
*/
public static boolean isNullorEmpty(String value) {
if (value == null || value.isEmpty()) {
return true;
}
return false;
}
public static String getNCHome() {
String nchome = RegUtil.getNCHome();
// if (nchome == null) {
// throw new RuntimeException("Unable to read the environment variable NCHOME.");
// }
return nchome;
}
public static String getConfigPath() {
File configPath = null;
String path = "";
String[] paths = new String[]{
getNCHome(),
ServicePropValues.CACHE_USER_DIR,
BridgeServicePropValues.getUserDir(),
"../"
};
for (String pathValue : paths) {
if (pathValue != null) {
configPath = new File(pathValue);
if (configPath.exists()) {
path = configPath.getAbsolutePath();
break;
}
}
}
if (path.isEmpty()) {
path = "./";
}
return path;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy