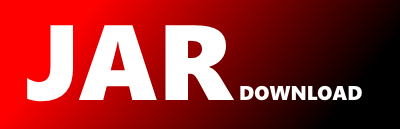
Alachisoft.NCache.Common.DataReader.ColumnCollection Maven / Gradle / Ivy
package Alachisoft.NCache.Common.DataReader;
import com.alachisoft.ncache.serialization.core.io.InternalCompactSerializable;
import com.alachisoft.ncache.serialization.standard.io.CompactReader;
import com.alachisoft.ncache.serialization.standard.io.CompactWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
// Copyright (c) 2020 Alachisoft
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License
public class ColumnCollection implements InternalCompactSerializable, Iterable {
private Map _columns = new HashMap();
private Map _nameToIndex = new HashMap();
public final int getCount() {
return _columns.size();
}
public final int getHiddenColumnCount() {
int hiddenColumns = 0;
for (Object entry : _columns.entrySet()) {
Map.Entry columnsEntry = (Map.Entry) entry;
if (((RecordColumn) columnsEntry.getValue()).getIsHidden()) {
hiddenColumns++;
}
}
return hiddenColumns;
}
public final void add(RecordColumn column) {
if (_nameToIndex.get(column.getColumnName()) != null) {
throw new RuntimeException("Invalid query. Same column cannot be selected twice.");
}
_nameToIndex.put(column.getColumnName(), _columns.size());
_columns.put(_columns.size(), column);
}
public final RecordColumn get(String columnName) {
return (RecordColumn) _columns.get(_nameToIndex.get(columnName));
}
public final RecordColumn get(int index) {
return (RecordColumn) _columns.get(index);
}
public final int getColumnIndex(String columnName) {
return (int) _nameToIndex.get(columnName);
}
public final String getColumnName(int index) {
return ((RecordColumn) _columns.get(index)).getColumnName();
}
public final boolean contains(String columnName) {
return _nameToIndex.get(columnName) != null;
}
@Override
public void Deserialize(CompactReader reader) throws IOException, ClassNotFoundException {
_columns = new HashMap();
int columnCount = reader.ReadInt32();
for (int i = 0; i < columnCount; i++) {
Object tempVar = reader.ReadObject();
_columns.put(reader.ReadInt32(), tempVar instanceof RecordColumn ? (RecordColumn) tempVar : null);
}
_nameToIndex = new HashMap();
int indexCount = reader.ReadInt32();
for (int i = 0; i < indexCount; i++) {
Object tempVar2 = reader.ReadObject();
_nameToIndex.put(tempVar2 instanceof String ? (String) tempVar2 : null, reader.ReadInt32());
}
}
@Override
public void Serialize(CompactWriter writer) throws IOException {
writer.Write(_columns.size());
for (Object kv : _columns.entrySet()) {
Map.Entry result = (Map.Entry) kv;
writer.Write((int) result.getKey());
writer.WriteObject(result.getValue());
}
writer.Write(_nameToIndex.size());
for (Object kv : _nameToIndex.entrySet()) {
Map.Entry result = (Map.Entry) kv;
writer.WriteObject(result.getKey());
writer.Write((int) result.getValue());
}
}
@Override
public Iterator iterator() {
return _columns.entrySet().iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy