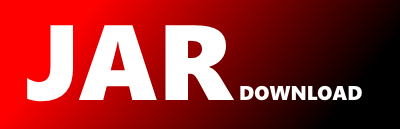
Alachisoft.NCache.Common.DataStructures.BridgeOperation Maven / Gradle / Ivy
package Alachisoft.NCache.Common.DataStructures;
import Alachisoft.NCache.Common.Stats.HPTime;
import com.alachisoft.ncache.serialization.core.io.ICompactSerializable;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectInput;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectOutput;
import java.io.IOException;
/**
* [Author:Taimoor Haider] BridgeOperation encapsulates all the necessary information for any operation which is to be perfrormed across the bridge.
*/
public class BridgeOperation implements ICompactSerializable, IOptimizedQueueOperation {
Object _queueCacheKey;
private BridgeOpCodes _opCode = BridgeOpCodes.values()[0];
private Object _key;
private Object _data;
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: byte _flags;
private byte _flags;
private int _size = 10;
private boolean _stateTxferOperation;
private boolean _doWriteBehind;
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: byte _redoCount;
private byte _redoCount;
private HPTime _bridgeOpTimeStamp;
public BridgeOperation() {
}
public BridgeOperation(BridgeOpCodes opCode, HPTime bridgeOpTimeStamp) {
this(opCode, null, null, bridgeOpTimeStamp);
}
public BridgeOperation(BridgeOpCodes opCode, String key, HPTime bridgeOpTimeStamp) {
this(opCode, key, null, 0, bridgeOpTimeStamp);
}
public BridgeOperation(BridgeOpCodes opCode, String key, boolean doWriteBehind, HPTime bridgeOpTimeStamp) {
this(opCode, key, null, 0, bridgeOpTimeStamp);
_doWriteBehind = doWriteBehind;
}
public BridgeOperation(BridgeOpCodes opCode, String key, Object data, HPTime bridgeOpTimeStamp) {
this(opCode, key, data, (byte) 0, 10, bridgeOpTimeStamp);
}
public BridgeOperation(BridgeOpCodes opCode, String key, Object data, int size, HPTime bridgeOpTimeStamp) {
this(opCode, key, data, (byte) 0, size, bridgeOpTimeStamp);
}
/**
* Initializes the an instance of the bridge operation.
*
* @param opCode OpCode of the operation to be performed across the bridge.
* @param key Key associataed with the operation.
* @param data Data associated with the operation which in most of the cases will be CacheEntry.
* @param flag An additional flags.
* @param size It is the size of the data associated with the operation.
*/
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public BridgeOperation(BridgeOpCodes opCode, string key, Object data, byte flag, int size, HPTime bridgeOpTimeStamp)
public BridgeOperation(BridgeOpCodes opCode, String key, Object data, byte flag, int size, HPTime bridgeOpTimeStamp) {
_opCode = opCode;
_key = key;
_data = data;
_flags = flag;
_size = size;
_bridgeOpTimeStamp = bridgeOpTimeStamp;
}
/**
* Gets/Sets the Operation code of the operation.
*/
public final BridgeOpCodes getOpCode() {
return _opCode;
}
public final void setOpCode(BridgeOpCodes value) {
_opCode = value;
}
public Object getQueueCacheKey() {
return _queueCacheKey;
}
public void setQueueCacheKey(Object _queueCacheKey) {
this._queueCacheKey = _queueCacheKey;
}
/**
* Gets/Sets the key which is associated with this operation.
*/
public final Object getKey() {
return _key;
}
public final void setKey(Object value) {
_key = value;
}
/**
* Gets/Sets the data associated with this operation.
*/
public final Object getData() {
return _data;
}
public final void setData(Object value) {
_data = value;
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public byte getRedoCount()
public final byte getRedoCount() {
return _redoCount;
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public void setRedoCount(byte value)
public final void setRedoCount(byte value) {
_redoCount = value;
}
/**
* Gets/Sets the value which indicates that this operation is state transfer operation or not.
*/
public final boolean getIsStateTransferOperation() {
return _stateTxferOperation;
}
public final void setIsStateTransferOperation(boolean value) {
_stateTxferOperation = value;
}
/**
* Gets/Sets any additional flag attached with this operation.
*/
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public byte getFlags()
public final byte getFlags() {
return _flags;
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public void setFlags(byte value)
public final void setFlags(byte value) {
_flags = value;
}
/**
* Gets/Sets the size of the operation. It is actuall the size of the data associated with any operation.
*/
public final int getSize() {
return _size;
}
public final void setSize(int value) {
_size = value;
}
/**
* Gets/Sets the value which indicates whether write behind operation is to be performed on the target cache.
*/
public final boolean getDoWriteBehind() {
return _doWriteBehind;
}
public final void setDoWriteBehind(boolean value) {
_doWriteBehind = value;
}
public final Object[] getUserPayLoad() {
return null;
}
public final void setUserPayLoad(Object[] value) {
;
}
public final long getPayLoadSize() {
return 0;
}
public final void setPayLoadSize(long value) {
;
}
public final HPTime getBridgeOpTimeStamp() {
return _bridgeOpTimeStamp;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("key = " + getKey().toString() + ",");
sb.append("operation = " + getOpCode().name());
return sb.toString();
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region ICompactSerializable Members
public final void Deserialize(com.alachisoft.ncache.serialization.standard.io.CompactReader reader) throws IOException, ClassNotFoundException {
_opCode = BridgeOpCodes.forValue(reader.ReadByte());
_key = reader.ReadObject();
_flags = reader.ReadByte();
_data = reader.ReadObject();
_size = reader.ReadInt32();
_stateTxferOperation = reader.ReadBoolean();
_doWriteBehind = reader.ReadBoolean();
_redoCount = reader.ReadByte();
_bridgeOpTimeStamp = (HPTime) reader.ReadObject();
_queueCacheKey = reader.ReadObject();
}
public final void Serialize(com.alachisoft.ncache.serialization.standard.io.CompactWriter writer) throws IOException, ClassNotFoundException {
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: writer.Write((byte)_opCode);
writer.Write((byte) _opCode.getValue());
writer.WriteObject(_key);
writer.Write(_flags);
writer.WriteObject(_data);
writer.Write(_size);
writer.Write(_stateTxferOperation);
writer.Write(_doWriteBehind);
writer.Write(_redoCount);
writer.WriteObject(_bridgeOpTimeStamp);
writer.WriteObject(_queueCacheKey);
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
@Override
public void serialize(NCacheObjectOutput writer) throws IOException {
// writer.write((byte)_opCode.getValue());
// writer.writeObject(_key);
// writer.write(_flags);
// writer.writeObject(_data);
// writer.write(_size);
// writer.writeBoolean(_stateTxferOperation);
// writer.writeBoolean(_doWriteBehind);
// writer.write(_redoCount);
// writer.writeObject(_bridgeOpTimeStamp);
writer.writeByte(_opCode.getValue());
writer.writeObject(_key);
writer.writeByte(_flags);
writer.writeObject(_data);
writer.writeInt(_size);
writer.writeBoolean(_stateTxferOperation);
writer.writeBoolean(_doWriteBehind);
writer.writeByte(_redoCount);
writer.writeObject(_bridgeOpTimeStamp);
writer.writeObject(_queueCacheKey);
writer.flush();
}
@Override
public void deserialize(NCacheObjectInput reader) throws IOException, ClassNotFoundException {
_opCode = BridgeOpCodes.forValue(reader.readByte());
_key = reader.readObject();
_flags = reader.readByte();
_data = reader.readObject();
_size = reader.readInt();
_stateTxferOperation = reader.readBoolean();
_doWriteBehind = reader.readBoolean();
_redoCount = reader.readByte();
_bridgeOpTimeStamp = (HPTime) reader.readObject();
_queueCacheKey = reader.readObject();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy