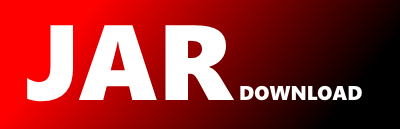
Alachisoft.NCache.Common.DataStructures.Enumerator Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package Alachisoft.NCache.Common.DataStructures;
//C# TO JAVA CONVERTER TODO TASK: The interface type was changed to the closest equivalent Java type, but the methods implemented will need adjustment:
import Alachisoft.NCache.Common.Stats.Clock;
public class Enumerator implements java.util.Iterator {
private java.util.ArrayList> _index = new java.util.ArrayList>();
private java.util.Iterator> _enumerator;
private java.util.Iterator _subIndexEnumerator;
private T _current;
private boolean _replicate = true;
public Enumerator(SlidingIndex slidingIndex) {
for (InstantaneousIndex indexEntry : slidingIndex._mainIndex) {
//for older enteries which are not supposed to change
if (indexEntry.getClockTime() != Clock.getCurrentTimeInSeconds()) {
_index.add(indexEntry);
} else {
//index being modified currently
Object tempVar = indexEntry.clone();
_index.add((InstantaneousIndex) tempVar);
}
}
_enumerator = _index.iterator();
}
public Enumerator(SlidingIndex slidingIndex, tangible.RefObject startTime, boolean replicate)
{
long initialTime = startTime.argvalue;
_replicate = replicate;
if (replicate)
{
for (InstantaneousIndex indexEntry : slidingIndex._mainIndex)
{
if (indexEntry.getClockTime() > initialTime)
{
//for older enteries which are not supposed to change
if (indexEntry.getClockTime() != Clock.getCurrentTimeInSeconds())
{
_index.add(indexEntry);
}
else
{
//index being modified currently
Object tempVar = indexEntry.clone();
_index.add((InstantaneousIndex)((tempVar instanceof InstantaneousIndex) ? tempVar : null));
}
}
startTime.argvalue = indexEntry.getClockTime();
}
}
else
{
_index = slidingIndex._mainIndex;
for (InstantaneousIndex indexEntry : slidingIndex._mainIndex)
{
startTime.argvalue = indexEntry.getClockTime();
}
}
_enumerator = _index.iterator();
}
public final T getCurrent() {
return _current;
}
public final void dispose() {
}
public final boolean MoveNext() {
do {
if (_subIndexEnumerator == null) {
if (_enumerator.hasNext()) {
InstantaneousIndex indexEntry = _enumerator.next();
_subIndexEnumerator = indexEntry.iterator();
}
}
if (_subIndexEnumerator != null) {
if (_subIndexEnumerator.hasNext()) {
_current = _subIndexEnumerator.next();
return true;
} else {
_subIndexEnumerator = null;
}
} else {
return false;
}
} while (true);
}
public final void Reset() {
_enumerator = _index.iterator();
_subIndexEnumerator = null;
_current = null;
}
@Override
public boolean hasNext() {
return _enumerator.hasNext();
}
@Override
public T next() {
do {
if (_subIndexEnumerator == null) {
if (_enumerator.hasNext()) {
InstantaneousIndex indexEntry = _enumerator.next();
_subIndexEnumerator = indexEntry.iterator();
}
}
if (_subIndexEnumerator != null) {
if (_subIndexEnumerator.hasNext()) {
_current = _subIndexEnumerator.next();
return _current;
} else {
_subIndexEnumerator = null;
}
} else {
return null;
}
} while (true);
}
@Override
public void remove() {
throw new UnsupportedOperationException("Not supported yet.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy