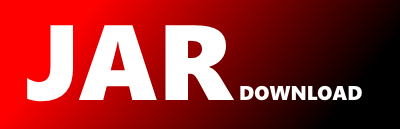
Alachisoft.NCache.Common.DataStructures.HashMapBucket Maven / Gradle / Ivy
package Alachisoft.NCache.Common.DataStructures;
import Alachisoft.NCache.Common.Net.Address;
import Alachisoft.NCache.Common.Threading.Latch;
import Alachisoft.NCache.Common.Threading.Monitor;
import com.alachisoft.ncache.serialization.core.io.ICompactSerializable;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectInput;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectOutput;
import java.io.IOException;
/**
* Each key based on the hashcode belongs to a bucket. This class keeps the overall stats for the bucket and points to its owner.
*/
public class HashMapBucket implements ICompactSerializable, Cloneable {
private int _bucketId;
private Address _tempAddress;
private Address _permanentAddress;
private Latch _stateTxfrLatch = new Latch(BucketStatus.Functional);
private Object _status_wait_mutex = new Object();
public HashMapBucket(Address address, int id) {
_tempAddress = _permanentAddress = address;
_bucketId = id;
_stateTxfrLatch = new Latch(BucketStatus.Functional);
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public HashMapBucket(Address address, int id,byte status)
public HashMapBucket(Address address, int id, byte status) {
this(address, id);
setStatus(status);
}
/**
* @deprecated used only for ICompactSerializable
*/
@Deprecated
public HashMapBucket() {
}
public final int getBucketId() {
return _bucketId;
}
public final Address getTempAddress() {
return _tempAddress;
}
public final void setTempAddress(Address value) {
_tempAddress = value;
}
public final Address getPermanentAddress() {
return _permanentAddress;
}
public final void setPermanentAddress(Address value) {
_permanentAddress = value;
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public void WaitForStatus(Address tmpOwner, byte status)
public final void WaitForStatus(Address tmpOwner, byte status) throws InterruptedException {
if (tmpOwner != null) {
while (tmpOwner == _tempAddress) {
if (_stateTxfrLatch.IsAnyBitsSet(status)) {
return;
}
synchronized (_status_wait_mutex) {
if ((tmpOwner == _tempAddress) || _stateTxfrLatch.IsAnyBitsSet(status)) {
return;
}
//Basit: Interrupt exception thrown (added)
Monitor.wait(_status_wait_mutex); //_status_wait_mutex.wait();
}
}
}
}
public final void NotifyBucketUpdate() {
synchronized (_status_wait_mutex) {
Monitor.pulse(_status_wait_mutex); //_status_wait_mutex.notifyAll();
}
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public void setStatus(byte value)
/**
* Sets the status of the bucket. A bucket can have any of the following status 1- Functional 2- UnderStateTxfr 3- NeedStateTransfer.
*/
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public byte getStatus()
public final byte getStatus() {
return _stateTxfrLatch.getStatus().getData();
}
public final void setStatus(byte value) {
switch (value) {
case BucketStatus.Functional:
case BucketStatus.NeedTransfer:
case BucketStatus.UnderStateTxfr:
//these are valid status,we allow them to be set.
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: byte oldStatus = _stateTxfrLatch.Status.Data;
byte oldStatus = _stateTxfrLatch.getStatus().getData();
if (oldStatus == value) {
return;
}
_stateTxfrLatch.SetStatusBit(value, oldStatus);
break;
}
}
public final Latch getStateTxfrLatch() {
return _stateTxfrLatch;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof HashMapBucket) {
return this.getBucketId() == ((HashMapBucket) obj).getBucketId();
}
return false;
}
public final Object clone() {
HashMapBucket hmBucket = new HashMapBucket(_permanentAddress, _bucketId);
hmBucket.setTempAddress(_tempAddress);
hmBucket.setStatus(getStatus());
return hmBucket;
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region ICompactSerializable Members
public void deserialize(NCacheObjectInput reader) throws ClassNotFoundException, IOException {
//Trace.error("HashMapBucket.Deserialize", "Deserialize Called");
_bucketId = reader.readInt();
_tempAddress = (Address) reader.readObject();
_permanentAddress = (Address) reader.readObject();
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: byte status = reader.ReadByte();
byte status = reader.readByte();
_stateTxfrLatch = new Latch(status);
}
public void serialize(NCacheObjectOutput writer) throws IOException {
//Trace.error("HashMapBucket.Serialize", "Serialize Called");
writer.writeInt(_bucketId);
writer.writeObject(_tempAddress);
writer.writeObject(_permanentAddress);
writer.writeByte(_stateTxfrLatch.getStatus().getData());
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Bucket[" + _bucketId + " ; ");
sb.append("owner = " + _permanentAddress + " ; ");
sb.append("temp = " + _tempAddress + " ; ");
String status = BucketStatus.StatusToString(_stateTxfrLatch.getStatus().getData());
sb.append(status + " ]");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy