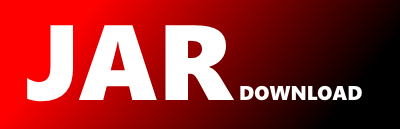
Alachisoft.NCache.Common.DataStructures.RecordColumn Maven / Gradle / Ivy
package Alachisoft.NCache.Common.DataStructures;
import Alachisoft.NCache.Common.Enum.AggregateFunctionType;
import com.alachisoft.ncache.serialization.core.io.ICompactSerializable;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectInput;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectOutput;
import java.io.IOException;
public class RecordColumn implements ICompactSerializable {
private String _name;
private boolean _isHidden;
private ColumnType _columnType = ColumnType.AttributeColumn;
private ColumnDataType _dataType = ColumnDataType.Object;
private AggregateFunctionType _aggregateFunctionType = getAggregateFunctionType().NOTAPPLICABLE;
//rowID-Object
private java.util.HashMap _data;
public RecordColumn(String name, boolean isHidden) {
_name = name;
_isHidden = isHidden;
_data = new java.util.HashMap();
}
public final java.util.HashMap getData() {
return _data;
}
//data-type
public final String getName() {
return _name;
}
public final boolean getIsHidden() {
return _isHidden;
}
public final void setIsHidden(boolean value) {
_isHidden = value;
}
public final ColumnType getType() {
return _columnType;
}
public final void setType(ColumnType value) {
_columnType = value;
}
public final ColumnDataType getDataType() {
return _dataType;
}
public final void setDataType(ColumnDataType value) {
_dataType = value;
}
public final AggregateFunctionType getAggregateFunctionType() {
return _aggregateFunctionType;
}
public final void setAggregateFunctionType(AggregateFunctionType value) {
_aggregateFunctionType = value;
}
public final void Add(Object value, int rowID) {
_data.put(rowID, value);
}
public final Object Get(int rowID) {
return _data.get(rowID);
}
@Override
public void serialize(NCacheObjectOutput out) throws IOException {
out.writeObject(_name);
out.writeBoolean(_isHidden);
out.write(_columnType.getValue());
out.write(_data.size());
for (java.util.Map.Entry de : _data.entrySet()) {
out.write(de.getKey());
out.writeObject(de.getValue());
}
}
@Override
public void deserialize(NCacheObjectInput in) throws IOException, ClassNotFoundException {
Object tempVar = in.readObject();
_name = (String) ((tempVar instanceof String) ? tempVar : null);
_isHidden = in.readBoolean();
_columnType = ColumnType.forValue(in.readInt());
int count = in.readInt();
for (int i = 0; i < count; i++) {
_data.put(in.readInt(), in.readObject());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy