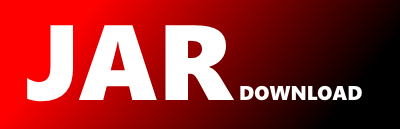
Alachisoft.NCache.Common.DataStructures.VirtualIndex Maven / Gradle / Ivy
package Alachisoft.NCache.Common.DataStructures;
public class VirtualIndex implements java.lang.Comparable {
private static final int maxSize = 79 * 1024;
private int x, y;
public VirtualIndex() {
}
public VirtualIndex(int index) {
IncrementBy(index);
}
public final void Increment() {
x++;
if (x == maxSize) {
x = 0;
y++;
}
}
public final void IncrementBy(int count) {
int number = (this.y * maxSize) + this.x + count;
this.x = number % maxSize;
this.y = number / maxSize;
}
public final int getXIndex() {
return x;
}
public final int getYIndex() {
return y;
}
public final int getIndexValue() {
return (y * maxSize) + x;
}
public final VirtualIndex clone() {
VirtualIndex clone = new VirtualIndex();
clone.x = x;
clone.y = y;
return clone;
}
//
public final int compareTo(Object obj) {
VirtualIndex other = null;
if (obj instanceof VirtualIndex) {
other = (VirtualIndex) ((obj instanceof VirtualIndex) ? obj : null);
} else if (obj instanceof Integer) {
other = new VirtualIndex((Integer) obj);
} else {
return -1;
}
if (other.getIndexValue() == getIndexValue()) {
return 0;
} else if (other.getIndexValue() > getIndexValue()) {
return -1;
} else {
return 1;
}
}
//
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy