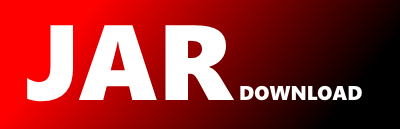
Alachisoft.NCache.Common.DirectoryUtil Maven / Gradle / Ivy
package Alachisoft.NCache.Common;
import java.io.File;
public class DirectoryUtil {
/**
* search for the specified file in the executing assembly's working folder if the file is found, then a path string is returned back. otherwise it returns null.
*
* @param fileName
* @return
*/
public static String GetFileLocalPath(String fileName) {
String path = (new java.io.File(System.getProperty("user.dir")).getParent() + "\\" + fileName);
if ((new java.io.File(path)).isFile()) {
return path;
}
return null;
}
/**
* search for the specified file in NCache install directory. if the file is found then returns the path string from where the file can be loaded. otherwise it returns null.
*
* @param fileName
* @return
*/
public static String GetFileGlobalPath(String fileName, String directoryName) {
String directoryPath = "";
String filePath = "";
tangible.RefObject tempRef_directoryPath = new tangible.RefObject(directoryPath);
boolean tempVar = !SearchGlobalDirectory(directoryName, false, tempRef_directoryPath);
directoryPath = tempRef_directoryPath.argvalue;
if (tempVar) {
return null;
}
File directoryPathFile = new File(directoryPath);
filePath = new File(directoryPathFile, fileName).getPath();
if (!(new java.io.File(filePath)).isFile()) {
return null;
}
return filePath;
}
public static boolean SearchLocalDirectory(String directoryName, boolean createNew, tangible.RefObject path) {
path.argvalue = (new java.io.File(System.getProperty("user.dir")).getParent());
if (!(new java.io.File(path.argvalue)).isDirectory()) {
if (createNew) {
try {
(new java.io.File(path.argvalue)).mkdir();
return true;
} catch (RuntimeException e) {
throw e;
}
}
return false;
}
return true;
}
public static boolean SearchGlobalDirectory(String directoryName, boolean createNew, tangible.RefObject path) {
String ncacheInstallDirectory = AppUtil.getInstallDir();
path.argvalue = "";
if (ncacheInstallDirectory == null) {
return false;
}
File ncacheInstallDirectoryFile = new File(ncacheInstallDirectory);
path.argvalue = new File(ncacheInstallDirectoryFile, directoryName).getPath();
if (!(new java.io.File(path.argvalue)).isDirectory()) {
if (createNew) {
try {
(new java.io.File(path.argvalue)).mkdir();
return true;
} catch (RuntimeException e) {
throw e;
}
}
return false;
}
return true;
}
public static String getDeployedAssemblyFolder() {
return Common.combinePath(AppUtil.getInstallDir(), AppUtil.DeployedAssemblyDir);
}
public static File createDeployAssemblyFolder(String cacheID) {
return new File(Common.combinePath(getDeployedAssemblyFolder(), cacheID.toLowerCase()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy