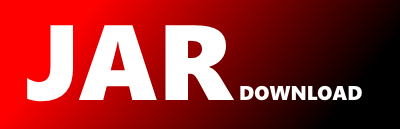
Alachisoft.NCache.Common.EncryptionUtil Maven / Gradle / Ivy
package Alachisoft.NCache.Common;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.io.UnsupportedEncodingException;
public class EncryptionUtil {
private static String algorithm = "DESede";
private static String transformation = "DESede/CBC/PKCS5Padding";
private static String s_key = "A41'D3a##asd[1-a;d zs[s`";
private static String s_iv = "KKNWLCZU";
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public static Byte[] ConvertStringToByteArray(String s)
public static byte[] ConvertStringToByteArray(String s) {
try {
return s.getBytes("ASCII");
} catch (UnsupportedEncodingException ex) {
return null;
}
}
// Encrypt the string.
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public static byte[] Encrypt(string PlainText)
public static byte[] Encrypt(String PlainText) {
if (PlainText == null) {
return null;
}
try {
byte[] data = ConvertStringToByteArray(PlainText);
byte[] k = ConvertStringToByteArray(s_key);
byte[] IV = ConvertStringToByteArray(s_iv);
SecretKeySpec securityKey = new SecretKeySpec(k, algorithm);
Cipher encrypter = Cipher.getInstance(transformation);
encrypter.init(Cipher.ENCRYPT_MODE, securityKey, new IvParameterSpec(IV));
return encrypter.doFinal(data);
} catch (Exception e) {
return null;
}
// byte[] stringToByte = null;
//
// try
// {
// stringToByte = PlainText.getBytes("UTF-8");
// }
// catch (UnsupportedEncodingException ch)
// {
// }
//
// return stringToByte;
// try
// {
// s_des = new DESCryptoServiceProvider();
// int i = s_des.BlockSize;
// int j = s_des.KeySize;
// s_des.Key = ConvertStringToByteArray(s_key);
// s_des.IV = ConvertStringToByteArray(s_iv);
//
// // Create a memory stream.
// MemoryStream ms = new MemoryStream();
//
// // Create a CryptoStream using the memory stream and the
// // CSP DES key.
// CryptoStream encStream = new CryptoStream(ms, s_des.CreateEncryptor(), CryptoStreamMode.Write);
//
// // Create a StreamWriter to write a string
// // to the stream.
// StreamWriter sw = new StreamWriter(encStream);
//
// // Write the plaintext to the stream.
// sw.WriteLine(PlainText);
//
// // Close the StreamWriter and CryptoStream.
// sw.Close();
// encStream.Close();
//
// // Get an array of bytes that represents
// // the memory stream.
////C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
////ORIGINAL LINE: byte[] buffer = ms.ToArray();
// byte[] buffer = ms.toArray();
//
// // Close the memory stream.
// ms.Close();
//
// // Return the encrypted byte array.
// return buffer;
// }
// catch (RuntimeException e)
// {
// }
// return null;
}
// Decrypt the byte array.
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public static string Decrypt(byte[] CypherText)
public static String Decrypt(byte[] CypherText) {
if (CypherText == null) {
return new String();
}
try {
byte[] k = ConvertStringToByteArray(s_key);
byte[] IV = ConvertStringToByteArray(s_iv);
SecretKeySpec securityKey = new SecretKeySpec(k, algorithm);
Cipher encrypter = Cipher.getInstance(transformation);
encrypter.init(Cipher.DECRYPT_MODE, securityKey, new IvParameterSpec(IV));
return new String(encrypter.doFinal(CypherText), "ASCII");
} catch (Exception ex) {
return null;
}
// String byteToString = "";
//
// try
// {
// byteToString = new String(CypherText, "UTF-8");
// }
// catch (UnsupportedEncodingException ch)
// {
// return new String();
// }
//
// return byteToString;
// try
// {
// s_des = new DESCryptoServiceProvider();
// s_des.Key = ConvertStringToByteArray(s_key);
// s_des.IV = ConvertStringToByteArray(s_iv);
//
//
// // Create a memory stream to the passed buffer.
// MemoryStream ms = new MemoryStream(CypherText);
//
// // Create a CryptoStream using the memory stream and the
// // CSP DES key.
// CryptoStream encStream = new CryptoStream(ms, s_des.CreateDecryptor(), CryptoStreamMode.Read);
//
// // Create a StreamReader for reading the stream.
// StreamReader sr = new StreamReader(encStream);
//
// // Read the stream as a string.
// String val = sr.ReadLine();
//
// // Close the streams.
// sr.Close();
// encStream.Close();
// ms.Close();
// return val;
// }
// catch (RuntimeException e)
// {
// }
// return null;
}
///
/// Encrypt user provided key with the default key stored; This key is obfuscated
///
/// Key
/// encrypted string
public static String EncryptKey(String key) {
if (key == null && key.isEmpty()) {
return new String();
}
try {
byte[] data = key.getBytes("US-ASCII");
byte[] k = ConvertStringToByteArray(s_key);
byte[] IV = ConvertStringToByteArray(s_iv);
SecretKeySpec securityKey = new SecretKeySpec(k, algorithm);
Cipher encrypter = Cipher.getInstance(transformation);
encrypter.init(Cipher.ENCRYPT_MODE, securityKey, new IvParameterSpec(IV));
byte[] encryptedText = encrypter.doFinal(data);
return Base64.encodeBytes(encryptedText);
} catch (Exception ex) {
return null;
}
}
public static String DecryptKey(String encodedkey) {
if (encodedkey == null && encodedkey.isEmpty()) {
return new String();
}
try {
byte[] data = Base64.decode(encodedkey);
byte[] k = ConvertStringToByteArray(s_key);
byte[] IV = ConvertStringToByteArray(s_iv);
SecretKeySpec securityKey = new SecretKeySpec(k, algorithm);
Cipher encrypter = Cipher.getInstance(transformation);
encrypter.init(Cipher.DECRYPT_MODE, securityKey, new IvParameterSpec(IV));
byte[] encryptedText = encrypter.doFinal(data);
return new String(encryptedText, "US-ASCII");
} catch (Exception ex) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy