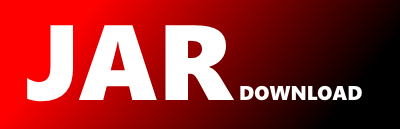
Alachisoft.NCache.Common.ErrorHandling.ErrorMessages Maven / Gradle / Ivy
package Alachisoft.NCache.Common.ErrorHandling;
import java.util.HashMap;
public class ErrorMessages {
private static java.util.Map _errorMessageMap = new HashMap();
static {
//region Common Exceptions
_errorMessageMap.put(ErrorCodes.Common.NO_SERVER_AVAILABLE, "No server is available to process the request.");
_errorMessageMap.put(ErrorCodes.Common.OUTPUT_CORRUPTED_ON_NODE, "Output corrupted on node : '%s'and Exception is :");
_errorMessageMap.put(ErrorCodes.Common.NUMBER_OF_EVENTS_PROCESSING_THREADS, "Invalid value specified for NCacheClient.NumberofEventProccesingThreads.");
_errorMessageMap.put(ErrorCodes.Common.INVALID_VALUE_ASYNC_EVENT_NOTIF, "Invalid value specified for NCacheClient.AsynchronousEventNotification.");
_errorMessageMap.put(ErrorCodes.Common.OPERATION_INTERRUPTED, "Operation has been interrupted due to loss of connectivity between server and client. ");
_errorMessageMap.put(ErrorCodes.Common.ENUMERATION_MODIFIED, "Enumeration has been modified");
_errorMessageMap.put(ErrorCodes.Common.REQUEST_TIMEOUT, "Request timeout due to node down");
_errorMessageMap.put(ErrorCodes.Common.NO_SERVER_AVAILABLE_FOR_CACHE, "No server is available to process the request for '%s'");
_errorMessageMap.put(ErrorCodes.Common.NULL_VALUE, "value cannot be null.");
_errorMessageMap.put(ErrorCodes.Common.EMPTY_KEY, "key cannot be empty string");
_errorMessageMap.put(ErrorCodes.Common.DEPENDENCY_KEY_NOT_FOUND, "One of the dependency keys does not exist.");
_errorMessageMap.put(ErrorCodes.Common.NOT_ENOUGH_ITEMS_EVICTED, "The cache is full and not enough items could be evicted.");
//endregion
//region Cache Init Exceptions
_errorMessageMap.put(ErrorCodes.CacheInit.SAME_NAME_FOR_BOTH_CACHES, "Same cache-name cannot be specified for both Primary and Client caches.");
_errorMessageMap.put(ErrorCodes.CacheInit.CLUSTER_INIT_IN_INPROC, "Cluster cache cannot be initialized in In-Proc mode.");
_errorMessageMap.put(ErrorCodes.CacheInit.CANT_START_AS_INPROC, "Cannot start cache of type '%s' as in-proc cache.");
_errorMessageMap.put(ErrorCodes.CacheInit.CACHE_KEY_NULL, "Cache key null");
_errorMessageMap.put(ErrorCodes.CacheInit.CACHE_ID_NULL, "Cache id can not be null");
_errorMessageMap.put(ErrorCodes.CacheInit.INVALID_BIND_IP_CLIENT_CONFIG, "Invalid bind-ip-address specified in client configuration");
_errorMessageMap.put(ErrorCodes.CacheInit.CACHE_NOT_INIT, "Cache is not initialized");
_errorMessageMap.put(ErrorCodes.CacheInit.L1_CACHE_NOT_INIT, "level1Cache not initialized");
_errorMessageMap.put(ErrorCodes.CacheInit.L2_CACHE_NOT_INIT, "level2Cache not initialized");
_errorMessageMap.put(ErrorCodes.CacheInit.SERVER_INFO_NOT_FOUND, "'client.ncconf' not found or does not contain server information");
_errorMessageMap.put(ErrorCodes.CacheInit.CACHE_ID_EMPTY_STRING, "cacheId cannot be an empty string");
_errorMessageMap.put(ErrorCodes.CacheInit.CACHE_NOT_REGISTERED_ON_NODE, "cache with name '%s' not registered on specified node");
_errorMessageMap.put(ErrorCodes.CacheInit.CACHE_ALREADY_RUNNING, "Specified cacheId is already running");
_errorMessageMap.put(ErrorCodes.CacheInit.CACHE_ID_NOT_REGISTERED, "Specified cacheId is not registered.");
//endregion
//region Licensing exceptions
_errorMessageMap.put(ErrorCodes.Licensing.INVALID_INSTALLCODE, "Invalid Install Code");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_EXPIRED, "NCache license is expired.");
_errorMessageMap.put(ErrorCodes.Licensing.INVALID_LICENSE_PROVIDER, "Invalid License provider.");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_001, "Data not found -- Stage 001");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_002, "Product is deactivated -- Stage 002");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_003, "Invalid Authentication Code--Stage03");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_004, "Invalid Authentication Code--Stage04");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_005, "");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_006, "");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_007, "Invalid Authentication Code--Stage07");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_008, "Invalid Authentication Code--Stage08");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_009, "Invalid Authentication Code--Stage09");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_010, "Invalid Authentication Code--Stage10");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_STAGE_011, "Invalid Authentication Code--Stage11");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_EXPIRED_STAGE_011, "License for NCache has been expired.--Stage11");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_EXPIRED_STAGE_012, "License for NCache has been expired--Stage12");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSE_NOT_VERIFIED, "License not verified.'%s'");
_errorMessageMap.put(ErrorCodes.Licensing.EVALUATION_PERIOD_EXPIRED, "Your library has expired the evaluation period of '%s' days.");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSING_DLL_MISSING, "DLL NOT FOUND EXCEPTION {0}");
_errorMessageMap.put(ErrorCodes.Licensing.LICENSING_INFO_CORRUPTED, "EvaluationLicense information is either missing or corrupted.");
_errorMessageMap.put(ErrorCodes.Licensing.NCLICENSE_LOAD_FAILURE, "Unable to load nclicense.dll");
//endregion
//region PubSub Exceptions
_errorMessageMap.put(ErrorCodes.PubSub.MESSAGE_ID_ALREADY_EXISTS, "The specified message id already exists.");
_errorMessageMap.put(ErrorCodes.PubSub.TOPIC_NOT_FOUND, "Topic '%s' does not exists.");
_errorMessageMap.put(ErrorCodes.PubSub.DEFAULT_TOPICS, "Operation cannot be performed on default topics.");
_errorMessageMap.put(ErrorCodes.PubSub.GET_MESSAGE_OPERATION_FAILED, "Get Message operation failed. Error : '%s' inner");
_errorMessageMap.put(ErrorCodes.PubSub.ACKNOWLEDGE_MESSAGE_FAILURE, "AcknowledgeMessageReceipt operation failed.Error: {0}");
_errorMessageMap.put(ErrorCodes.PubSub.SUBSCRIPTION_EXISTS, "Active subscription with this name already exists");
_errorMessageMap.put(ErrorCodes.PubSub.PATTERN_BASED_PUBLISHING_NOT_ALLOWED, "Message publishing on pattern based topic is not allowed");
_errorMessageMap.put(ErrorCodes.PubSub.TOPIC_DISPOSED, "Topic '%s' is disposed.");
_errorMessageMap.put(ErrorCodes.PubSub.INVALID_TOPIC_NAME, "TopicName is null or empty string");
_errorMessageMap.put(ErrorCodes.PubSub.INVALID_TOPIC_PATTERN, "Invalid topic pattern is specified");
_errorMessageMap.put(ErrorCodes.PubSub.NULL_EXCEPTION, "");
_errorMessageMap.put(ErrorCodes.PubSub.TOPIC_OPERATION_FAILED, "Topic operation failed. Error: '%s'");
//endregion
//region QUERIES Related Exceptions
_errorMessageMap.put(ErrorCodes.Queries.AVERAGE_FOR_INTEGRAL_DATATYPES_ONLY, "AVG can only be applied to integral data types.");
_errorMessageMap.put(ErrorCodes.Queries.LESS_VALUES_SPECIFIED, "Less value(s) are specified for indexed attribute '%s'.");
_errorMessageMap.put(ErrorCodes.Queries.MAX_APPLIED_ON_BOOL, "MAX cannot be applied to Boolean data type.");
_errorMessageMap.put(ErrorCodes.Queries.DUPLICATE_COLUMNS, "Invalid query. Duplicate columns found in orderby clause.");
_errorMessageMap.put(ErrorCodes.Queries.MIN_APPLIED_ON_BOOL, "MIN cannot be applied to Boolean data type.");
_errorMessageMap.put(ErrorCodes.Queries.SOLE_PROJECTION_MEMBER_SPECIFIED, "Sole projection member is specified along other projection(s).");
_errorMessageMap.put(ErrorCodes.Queries.SOLE_PROJECTION_ALONG_CLAUSES, "Sole projection member is specified along prohibited clause(s).");
_errorMessageMap.put(ErrorCodes.Queries.SUM_FOR_INTEGRAL_DATATYPES, "SUM can only be applied to integral data types.");
_errorMessageMap.put(ErrorCodes.Queries.INDEX_NOT_DEFINED, "Index is not defined for attribute '%s'");
_errorMessageMap.put(ErrorCodes.Queries.NULL_VALUES_NOT_SUPPORTED, "NCache query does not support null values");
_errorMessageMap.put(ErrorCodes.Queries.UNABLE_EXTRACTING_QUERY_INFO, "Unable extracting query information from user object.");
_errorMessageMap.put(ErrorCodes.Queries.SAME_COLUMN_SELECTED_TWICE, "Invalid query. Same column cannot be selected twice.");
_errorMessageMap.put(ErrorCodes.Queries.INVALID_TYPE_SPECIFIED, "Invalid type is specified");
_errorMessageMap.put(ErrorCodes.Queries.SYNTAX_NOT_SUPPORTED, "This syntax is currently not supported.");
_errorMessageMap.put(ErrorCodes.Queries.EXTRA_VALUES_SPECIFIED, "Extra value(s) are specified for indexed attribute '%s'.");
_errorMessageMap.put(ErrorCodes.Queries.INCORRECT_FORMAT_NOT_SUPPORTED, "Incorrect query format. \'*\' is not supported.");
_errorMessageMap.put(ErrorCodes.Queries.PROJECTION_TYPE_NOT_SUPPORTED, "The {0} projection type is not supported in the ProjectionPredicate.");
_errorMessageMap.put(ErrorCodes.Queries.INCORRECT_FORMAT_SPEC, "Incorrect query format. '%s' not supported.");
_errorMessageMap.put(ErrorCodes.Queries.INCORRECT_FORMAT_GIVEN, "Incorrect query format \'*\'.");
_errorMessageMap.put(ErrorCodes.Queries.SPECIFIED_FEILD_PROPERTY_NOT_FOUND, "Provided data contains no field or property named {0}");
_errorMessageMap.put(ErrorCodes.Queries.ORDER_BY_ATTRIBUTES_MISSING, "Invalid query. All ORDER BY attributes must be included in GROUP BY clause.");
_errorMessageMap.put(ErrorCodes.Queries.GROUP_BY_ONLY_WITH_AGGREGATE, "Invalid query. GROUP BY can only be used with Aggregate functions.");
_errorMessageMap.put(ErrorCodes.Queries.INVALID_TERM_FREQ_SPEC, "Invalid query. Term frequency aggregation function should be the only projection and be specified only with FROM clause.");
_errorMessageMap.put(ErrorCodes.Queries.MISSING_GROUP_BY, "Invalid Query. Group By clause containing the projected attributes, must be specified if Aggregate Function is used.");
_errorMessageMap.put(ErrorCodes.Queries.ENTRY_GROUPING, "Invalid Query. Cache entries can not be grouped/aggregated");
_errorMessageMap.put(ErrorCodes.Queries.COLLECTION_WITHOUT_STAR, "Selective projection is not supported with type $DataType$");
_errorMessageMap.put(ErrorCodes.Queries.GROUP_BY_WITHOUT_AGGREGATE, "Invalid query. Aggregate function is expected.");
_errorMessageMap.put(ErrorCodes.Queries.INCORRECT_QUERY_FORMAT, "Incorrect query format. '%s' not supported in this format.");
_errorMessageMap.put(ErrorCodes.Queries.OBJECT_TYPE, "Object must be of type {0}");
_errorMessageMap.put(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, "Operation is not valid due to the current state of the object");
_errorMessageMap.put(ErrorCodes.Queries.DATAREADER_STATE_LOST, "Data reader has lost its state");
_errorMessageMap.put(ErrorCodes.Queries.READER_STATE_LOST, "Reader state has been lost.");
_errorMessageMap.put(ErrorCodes.Queries.SAME_VALUE_SELECTED_TWICE, "Invalid query. Same value cannot be selected twice.");
_errorMessageMap.put(ErrorCodes.Queries.ATTRIBUTE_MISSING_IN_GROUP_BY, "Invalid query. '%s' must be specified in GROUP BY clause.");
_errorMessageMap.put(ErrorCodes.Queries.GROUP_BY_MISSING_ATTRIBUTE, "Invalid query. Not all projected columns specified in GROUP BY clause.");
_errorMessageMap.put(ErrorCodes.Queries.REQUIRES_SAME_CLASS_IN_ALL_AGGREGATE_FUNCTIONS, "Invalid query. Same class should be specified in all aggregate functions.");
_errorMessageMap.put(ErrorCodes.Queries.EVENT_REGISTRATION_REQUIRED, "There is no event registered for this query. Please register at least one event (add/update/remove) before activating query.");
_errorMessageMap.put(ErrorCodes.Queries.EVENT_REGISTRATION_REQUIRED_CQ, "There is no event registered for this query. Please register at least one event (add/update/remove) before activating continuous query.");
_errorMessageMap.put(ErrorCodes.Queries.INDEX_MISSING_IN_RECORDSET, "Invalid index. Specified index does not exist in RecordSet.");
_errorMessageMap.put(ErrorCodes.Queries.TYPE_NOT_INDEXABLE, "The provided type is not indexable.");
_errorMessageMap.put(ErrorCodes.Queries.INDEX_OUTSIDE_BOUNDS, "Index was outside the bounds of the array.");
_errorMessageMap.put(ErrorCodes.Queries.COMPARISION_OPERATOR_NOT_SUPPORTED_HS, "The specified Comparison operator '%s' is not supported for HashStore Index.");
_errorMessageMap.put(ErrorCodes.Queries.LUCENE_PREDICATES_SUPPORTED_FOR_LUCENE_ATTRIBUTES, "Lucene Predicates are only supported for Lucene Indexed attributes");
_errorMessageMap.put(ErrorCodes.Queries.COMPARISION_OPERATOR_NOT_SUPPORTED_RB, "The specified Comparison operator '%s' is not supported for RBSTore Index.");
_errorMessageMap.put(ErrorCodes.Queries.TERMFREQ_SUPPORTED_FOR_LUCENE, "TERMFREQ is only supported for Lucene Indexed attributes.");
_errorMessageMap.put(ErrorCodes.Queries.HS_GET_TAG_DATA, "");
_errorMessageMap.put(ErrorCodes.Queries.TAGINDEX_INDEX_MEMORY_SIZE, "");
_errorMessageMap.put(ErrorCodes.Queries.CONVERSION_ERROR, "Cannot convert '%s' to '%s'");
_errorMessageMap.put(ErrorCodes.Queries.VALUES_NOT_SPECIFIED, "Value(s) not specified for indexed attribute '%s'.");
_errorMessageMap.put(ErrorCodes.Queries.SEARCH_OPERATION_FAILED, "search operation failed. Error: '%s'");
_errorMessageMap.put(ErrorCodes.Queries.DELETE_QUERY_OP_FAILED, "delete query operation failed. Error: '%s'");
_errorMessageMap.put(ErrorCodes.Queries.EXECUTE_READER_OP_FAILED, "ExecuteReader operation failed. Error: '%s'");
_errorMessageMap.put(ErrorCodes.Queries.INDEX_NOT_DEFINED_ON_ATTRIBUE, "The queried attribute does not have an index defined on it.");
_errorMessageMap.put(ErrorCodes.Queries.GROUP_BY_SECTION_NOT_SUPPORTED, "Search query doesn't support Group By Section");
_errorMessageMap.put(ErrorCodes.Queries.ONLY_SELECT_SUPPORTED, "Only select query is supported.");
_errorMessageMap.put(ErrorCodes.Queries.EXECUTE_NON_QUERY, "ExecuteNonQuery only supports delete query");
_errorMessageMap.put(ErrorCodes.Queries.TEXT_BASED_SEARCHING_NOT_SUPPORTED, "Text based searching is not supported in Continous Query");
_errorMessageMap.put(ErrorCodes.Queries.ORDER_BY_SECTION_NOT_SUPPORTED, "Search query doesn't support Order By Section");
_errorMessageMap.put(ErrorCodes.Queries.INCORRECT_FORMAT, "Incorrect query format");
//endregion
//region Expiration Exceptions
_errorMessageMap.put(ErrorCodes.Expiration.ERROR_LOADING_ORACLE_DATA_ACCESS, "Could not load assembly 'Oracle.DataAccess.dll'. Please make sure Oracle Data Provider for .NET is installed");
_errorMessageMap.put(ErrorCodes.Expiration.ABSOLUTE_DEFAULT_EXPIRATION, "Absolute Default expiration value is less than 5 seconds.");
_errorMessageMap.put(ErrorCodes.Expiration.ABSOLUTE_LONGER_EXPIRATION, "Absolute Longer expiration value is less than 5 seconds.");
_errorMessageMap.put(ErrorCodes.Expiration.SLIDING_DEFAULT_EXPIRATION, "Sliding Default expiration value is less than 5 seconds.");
_errorMessageMap.put(ErrorCodes.Expiration.SLIDING_LONGER_EXPIRATION, "Sliding Longer expiration value is less than 5 seconds.");
//endregion
//region Bridge Exceptions
_errorMessageMap.put(ErrorCodes.Bridge.BRIDGE_CONFLICT_RESOLUTION_MGR, "Unable to instantiate BridgeConflictResolutionMgr.");
_errorMessageMap.put(ErrorCodes.Bridge.SHUTDOWN_STARTED, "Shutdown is started");
_errorMessageMap.put(ErrorCodes.Bridge.NO_SERVER_AVAILABLE, "No server is available to process the request");
_errorMessageMap.put(ErrorCodes.Bridge.OPERATION_TIMED_OUT, "Operation timed out. No response from the server ['%s'] for '%s'");
_errorMessageMap.put(ErrorCodes.Bridge.RESOLVE_FAILED, "IBridgeConflictResolver.Resolve failed '%s'");
_errorMessageMap.put(ErrorCodes.Bridge.ACTIVE_PASSIVE_OPERATION_FAILED, "MakeCacheActivePassive operation failed. Error: {0}");
//endregion
//region CacheLoader Exceptions
_errorMessageMap.put(ErrorCodes.CacheLoader.NAMED_TAG_VALUE_NULL, "Named Tag value cannot be null");
//endregion
//region DataTypes Exceptions
_errorMessageMap.put(ErrorCodes.DataTypes.NO_ITEM_IN_HASHSET, "No item present in HashSet");
_errorMessageMap.put(ErrorCodes.DataTypes.NULL_ITEMS_IN_COLLECTION, "some items in collection are null");
_errorMessageMap.put(ErrorCodes.DataTypes.DATATYPE_SPECIFIED, "DataType must be properly specified");
_errorMessageMap.put(ErrorCodes.DataTypes.ARRAY_FAILED_TO_HOLD_DS_ITEMS, "Target array starting from the index specified is not big enough to hold data structure's items.");
_errorMessageMap.put(ErrorCodes.DataTypes.INVALID_COLLECTION_RESP, "Invalid collection response type!");
_errorMessageMap.put(ErrorCodes.DataTypes.CACHEITEM_IN_DATA_STRUCTURES, "CacheItem cannot be used in Data structures");
_errorMessageMap.put(ErrorCodes.DataTypes.COUNT_NON_POSITIVE, "Count is non positive integer");
_errorMessageMap.put(ErrorCodes.DataTypes.NOT_A_DICTIONARY, "Type mismatch: Corresponding item in cache is not a Dictionary.");
_errorMessageMap.put(ErrorCodes.DataTypes.NOT_A_LIST, "Type mismatch: Corresponding item in cache is not a List.");
_errorMessageMap.put(ErrorCodes.DataTypes.NOT_A_QUEUE, "Type mismatch: Corresponding item in cache is not a Queue.");
_errorMessageMap.put(ErrorCodes.DataTypes.NOT_A_SET, "Type mismatch: Corresponding item in cache is not a Set.");
_errorMessageMap.put(ErrorCodes.DataTypes.NOT_A_HASHSET, "Type mismatch: Corresponding item in cache is not a HashSet.");
_errorMessageMap.put(ErrorCodes.DataTypes.NOT_A_COUNTER, "Type mismatch: Corresponding item in cache is not a Counter.");
_errorMessageMap.put(ErrorCodes.DataTypes.TYPE_NOT_ALLOWED, "Type is not allowed");
//endregion
//region MapReduce Exceptions
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_FAILED_IN_SUBMISSION, "Task failed during submission.");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_STARTING_FAILED, "Task failed while starting.");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_CANT_BE_SUBMITTED, "No more task can be submitted");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_WITH_KEY_DONT_EXIST, "Task with specified key does not exist.");
_errorMessageMap.put(ErrorCodes.MapReduce.NULL_OR_EMPTY_TASK, "Task can not be null or empty");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_CANCELLED, "Task with Specified TaskId was cancelled.");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_CANCELLED_1, "Task with specified Task ID was cancelled");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_CANCELLED_2, "Task with specified Task ID was Cancelled.");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_DOES_NOT_EXIST, "Task with Specified Task id does not exists");
_errorMessageMap.put(ErrorCodes.MapReduce.NULL_PROVIDER, "Input Provider Cannot be null.");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_SUBMISSION_FAILED, "Task Submission Failed");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_IN_SUBMISSION_FAILED_NODE, "Task failed during submission on Node : '%s'");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_FAILED_WHILE_STARTING, "Task failed while starting on Node : '%s'");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_ENUMERATOR_INVALID, "Task Enumerator is Invalid");
_errorMessageMap.put(ErrorCodes.MapReduce.SUBMIT_TASK_FAILED, "SubmitMapReduceTask failed. Error: '%s'");
_errorMessageMap.put(ErrorCodes.MapReduce.GET_TIMEOUT, "GetResult request timeout.");
_errorMessageMap.put(ErrorCodes.MapReduce.ENUMERATION_ERROR, "Enumeration has either not started or already finished.");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_CANCELLED_REASON, "Task was canceled. Reason: '%s'");
_errorMessageMap.put(ErrorCodes.MapReduce.TASK_FAILED, "Task was failed. Reason: '%s'. Please refer to logs for detailed information.");
_errorMessageMap.put(ErrorCodes.MapReduce.CALLBACK_REGISTERED, "You have already registered a callback (async method for Get Result)");
//endregion
//region EntryProcessor Exceptions
_errorMessageMap.put(ErrorCodes.EntryProcessor.NOT_SUPPORTED_FOR_COLLECTIONS, "EntryProcessor is not supported for Collections.");
//endregion
//region Streaming Exceptions
_errorMessageMap.put(ErrorCodes.Streaming.GROUP_STREAM_MISMATCH, "Data group of the stream does not match the existing stream's data group");
_errorMessageMap.put(ErrorCodes.Streaming.GROUP_SUBGROUP_STREAM_MISMATCH, "Data group/subgroup of the stream does not match the existing stream's data group/subgroup");
//endregion
//region Serialization Exceptions
_errorMessageMap.put(ErrorCodes.Serialization.ASSEMBLY_VERSION_DIFFERENT, "Loaded assembly version is different from the registered version");
//endregion
//region BackingSource Exceptions
_errorMessageMap.put(ErrorCodes.BackingSource.BACKING_SOURCE_NOT_AVAILABLE, "Backing source not available. Verify backing source settings.");
_errorMessageMap.put(ErrorCodes.BackingSource.SYNCHRONIZATION_WITH_DATASOURCE, "Error occurred while synchronization with data source: '%s'");
_errorMessageMap.put(ErrorCodes.BackingSource.SYNCHRONIZATION_CACHE_WITH_DATASOURCE, "error while trying to synchronize the cache with data source. Error: '%s'");
_errorMessageMap.put(ErrorCodes.BackingSource.WRITE_OPERATION_FAILED, "Data Source write operation failed. Error: '%s'");
_errorMessageMap.put(ErrorCodes.BackingSource.IWRITE_THRU_PROVIDER_FAILED, "IWriteThruProvider failed.'%s'");
_errorMessageMap.put(ErrorCodes.BackingSource.INVALID_IWRITE_THRU_PROVIDER, "Could not found IWriteThruProvider '%s'.");
_errorMessageMap.put(ErrorCodes.BackingSource.INVALID_IREAD_THRU_PROVIDER, "Could not found IReadThruProvider '%s'.");
//endregion
//region Search Operations(tags,groups,subgroups) Exceptions
_errorMessageMap.put(ErrorCodes.SearchOperations.GET_KEYS_BY_TAG_FAILED, "GetKeysByTag operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.SearchOperations.GET_BY_TAGS_FAILED, "GetByTag operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.SearchOperations.REMOVE_BY_TAG_FAILED, "RemoveByTag operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.SearchOperations.GROUP_TAGS_ON_SAME_ITEM, "You cannot set both groups and tags on the same cache item.");
_errorMessageMap.put(ErrorCodes.SearchOperations.KEY_CONFLICTS_WITH_INDEXED_ATTRIBUTE, "Key in named tags conflicts with the indexed attribute name of the specified object.");
_errorMessageMap.put(ErrorCodes.SearchOperations.GROUP_FOR_SUBGROUP, "group must be specified for sub group");
//endregion
//region Basic operations Exceptions
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.GET_OPERATION_FAILED, "Get operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.KEY_ALREADY_EXISTS, "The specified key already exists.");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.ADD_OPERATION_FAILED, "Add operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.INSERTED_ITEM_DATAGROUP_MISMATCH, "Data group of the inserted item does not match the existing item's data group.");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.REMOVE_OPERATION_FAILED, "Remove operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.DELETE_OPERATION_FAILED, "Delete operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.INSERT_OPERATION_FAILED, "Insert operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.CONTAINS_OPERATION_FAILED, "Contains operation failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.ITEM_LOCKED, "Item is locked.");
_errorMessageMap.put(ErrorCodes.BasicCacheOperations.ITEM_WITH_VERSION_DOESNT_EXIST, "An item with specified version doesn't exist.");
//endregion
//region Notifications Exceptions
_errorMessageMap.put(ErrorCodes.Notifications.REGISTER_KEY_NOTIFICATION, "RegisterKeyNotification failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.Notifications.UNREGISTER_KEY_NOTIFICATION, "UnregisterKeyNotification failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.Notifications.REGISTER_COLLECTION_NOTIFICATION, "RegisterCollectionNotification failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.Notifications.UNREGISTER_COLLECTION_NOTIFICATON, "UnregisterCollectionNotification failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.Notifications.REGISTER_TASK_NOTIF_CALLBACK, "RegisterTaskNotificationCallback failed. Error : '%s'");
_errorMessageMap.put(ErrorCodes.Notifications.COLLECTION_EVENTS_ON_NONCOLLECTION, "Cannot register collection events on non-collection item.");
_errorMessageMap.put(ErrorCodes.Notifications.COLLECTION_TYPE_MISMATCH, "Collection type mismatch. Notifications cannot be registered via the handle at hand.");
//endregion
_errorMessageMap.put(ErrorCodes.Security.CLIENT_SERVER_SECURITY_MISMATCH, "Mistmatch between Client - Server connection security detected.The '%s' node must have Secured-Connection(SSL / TLS) enabled in order to communicate with a {1} node with Secured-Connection.");
_errorMessageMap.put(ErrorCodes.SQLDependency.INCORRECT_SYNTAX, "'%s'"); //"Incorrect syntax near the keyword"
//region Json Exceptions
_errorMessageMap.put(ErrorCodes.Json.ATTRIBUTE_ALREADY_EXISTS, "An attribute with the same name aleady exists.");
_errorMessageMap.put(ErrorCodes.Json.REFERENCE_TO_PARENT, "Reference to parent at nested level detected.");
_errorMessageMap.put(ErrorCodes.Json.REFERENCE_TO_SELF, "'{0}' cannot contain an attribute that is a reference to self.");
_errorMessageMap.put(ErrorCodes.Json.VALUE_NULL, "Value cannot be null. Parameter name: '%s'");
//endregion
//region Inproc Exceptions
_errorMessageMap.put(ErrorCodes.ClientCache.InprocCacheNotSuportedInJavaClient, "Inproc local cache is not supported from java applications.");
//endregion
}
public static String getErrorMessage(int errorCode, String... parameters) {
String errormessage = "exception";
//return errormessage;
return resolveError(errorCode, parameters);
}
public static String resolveError(int errorCode, String... parameters) {
String message = null;
if ((message = _errorMessageMap.get(errorCode)) != null) {
if (parameters == null || parameters.length == 0) {
return message;
}
return String.format(message, parameters);
}
return String.format("Missing error message for code (%1$s) in error to exception map", new Object[]{errorCode});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy