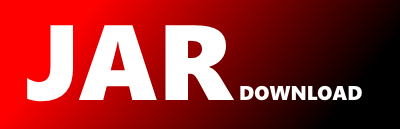
Alachisoft.NCache.Common.MemoryUtil Maven / Gradle / Ivy
package Alachisoft.NCache.Common;
// TODO (IN FUTURE) 5.0 SP3: Verify if sizes mentioned in MemoryUtil are ok
public class MemoryUtil
{
public static final int KB = 1024;
//24 Bytes overhead for every .net class in x64
public static final int NetOverHead = 24;
public static final int NetHashtableOverHead = 45;
public static final int NetListOverHead = 8;
public static final int NetClassOverHead = 16;
public static final int NetIntSize = 4;
public static final int NetEnumSize = 4;
public static final int NetByteSize = 1;
public static final int NetShortSize = 2;
public static final int NetStringCharSize = 16;
public static final int NetLongSize = 8;
public static final int NetDateTimeSize = 8;
public static final int NetReferenceSize = 8;
///#region Dot Net Primitive Tyes String Constants
public static final String Net_bool = "bool";
public static final String Net_System_Boolean = "System.Boolean";
public static final String Net_char = "char";
public static final String Net_System_Char = "System.Char";
public static final String Net_string = "string";
public static final String Net_System_String = "System.String";
public static final String Net_float = "float";
public static final String Net_System_Single = "System.Single";
public static final String Net_double = "double";
public static final String Net_System_Double = "System.Double";
public static final String Net_short = "short";
public static final String Net_ushort = "ushort";
public static final String Net_System_Int16 = "System.Int16";
public static final String Net_System_UInt16 = "System.UInt16";
public static final String Net_int = "int";
public static final String Net_System_Int32 = "System.Int32";
public static final String Net_uint = "uint";
public static final String Net_System_UInt32 = "System.UInt32";
public static final String Net_long = "long";
public static final String Net_System_Int64 = "System.Int64";
public static final String Net_ulong = "ulong";
public static final String Net_SystemUInt64 = "System.UInt64";
public static final String Net_byte = "byte";
public static final String Net_System_Byte = "System.Byte";
public static final String Net_sbyte = "sbyte";
public static final String Net_System_SByte = "System.SByte";
public static final String Net_System_Object = "System.Object";
public static final String Net_System_DateTime = "System.DateTime";
public static final String Net_decimal = "decimal";
public static final String Net_System_Decimal = "System.Decimal";
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Java Primitive Types String Constants
public static final String Java_Lang_Boolean = "java.lang.Boolean"; // True/false value
public static final String Java_Lang_Character = "java.lang.Character"; // Unicode character (16 bit;
public static final String Java_Lang_String = "java.lang.String"; // Unicode String
public static final String Java_Lang_Float = "java.lang.Float"; // IEEE 32-bit float
public static final String Java_Lang_Double = "java.lang.Double"; // IEEE 64-bit float
public static final String Java_Lang_Short = "java.lang.Short"; // Signed 16-bit integer
public static final String Java_Lang_Integer = "java.lang.Integer"; // Signed 32-bit integer
public static final String Java_Lang_Long = "java.lang.Long"; // Signed 32-bit integer
public static final String Java_Lang_Byte = "java.lang.Byte"; // Unsigned 8-bit integer
public static final String Java_Lang_Object = "java.lang.Object"; // Base class for all objects
public static final String Java_Util_Date = "java.util.Date"; // Dates will always be serialized (passed by value); according to .NET Remoting
public static final String Java_Match_BigDecimal = "java.math.BigDecimal"; // Will always be serialized (passed by value); according to .NET Remoting
///#endregion
/**
Hashcode algorithm returning same hash code for both 32bit and 64 bit apps.
Used for data distribution under por/partitioned topologies.
@param strArg
@return
*/
public static int getStringSize(Object key)
{
if (key != null && key instanceof String)
{
//size of .net charater is 2 bytes so multiply by 2 length of key, 24 bytes are extra overhead(header) of each instance
return (2 * ((String)key).length()) + MemoryUtil.NetOverHead;
}
return 0;
}
/**
Returns memory occupied in bytes by string instance without .NET overhead.
@param arg String value whose size without .NET overhead is to be determined.
@return Integer representing size of string instance without .NET overhead. 0 value corresponds to null or non-string argument.
*/
public static int getStringSizeWithoutNetOverhead(Object arg)
{
if (arg != null && arg instanceof String)
{
//size of .net charater is 2 bytes so multiply by 2 length of key
return (2 * ((String)arg).length());
}
return 0;
}
/**
Hashcode algorithm returning same hash code for both 32bit and 64 bit apps.
Used for data distribution under por/partitioned topologies.
@param strArg
@return
*/
public static int getStringSize(Object[] keys)
{
int totalSize = 0;
if (keys != null)
{
for (Object key : keys)
{
//size of .net charater is 2 bytes so multiply by 2 length of key, 24 bytes are extra overhead(header) of each instance
totalSize += (2 * ((String)key).length()) + MemoryUtil.NetOverHead;
}
}
return totalSize;
}
/**
Used to get DataType Size for provided AttributeSize.
@param strArg
@return
*/
public static int getTypeSize(AttributeTypeSize type)
{
switch (type)
{
case Byte1:
return 1;
case Byte2:
return 2;
case Byte4:
return 4;
case Byte8:
return 8;
case Byte16:
return 16;
}
return 0;
}
public static AttributeTypeSize getAttributeTypeSize(String type)
{
// switch (type)
if (Net_bool.equals(type) || Net_byte.equals(type) || Net_System_Byte.equals(type) || Net_sbyte.equals(type) || Net_System_SByte.equals(type) || Net_System_Boolean.equals(type) || Java_Lang_Boolean.equals(type) || Java_Lang_Byte.equals(type))
{
return AttributeTypeSize.Byte1;
}
else if (Net_char.equals(type) || Net_short.equals(type) || Net_ushort.equals(type) || Net_System_Int16.equals(type) || Net_System_UInt16.equals(type) || Net_System_Char.equals(type) || Java_Lang_Character.equals(type) || Java_Lang_Float.equals(type) || Java_Lang_Short.equals(type))
{
return AttributeTypeSize.Byte2;
}
else if (Net_float.equals(type) || Net_int.equals(type) || Net_System_Int32.equals(type) || Net_uint.equals(type) || Net_System_UInt32.equals(type) || Net_System_Single.equals(type) || Java_Lang_Integer.equals(type))
{
return AttributeTypeSize.Byte4;
}
else if (Net_double.equals(type) || Net_System_Double.equals(type) || Net_long.equals(type) || Net_System_Int64.equals(type) || Net_ulong.equals(type) || Net_System_DateTime.equals(type) || Net_SystemUInt64.equals(type) || Java_Lang_Double.equals(type) || Java_Lang_Long.equals(type) || Java_Util_Date.equals(type))
{
return AttributeTypeSize.Byte8;
}
else if (Net_decimal.equals(type) || Net_System_Decimal.equals(type) || Java_Match_BigDecimal.equals(type))
return AttributeTypeSize.Byte16;
return AttributeTypeSize.Variable;
}
public static Class> getDataType(String typeName)
{
// switch (typeName)
if (Net_string.equals(typeName))
return String.class;
else if (Net_System_String.equals(typeName))
return String.class;
else if (Java_Lang_String.equals(typeName))
return String.class;
else if (Net_bool.equals(typeName))
return Boolean.class;
else if (Net_byte.equals(typeName))
return Byte.class;
else if (Net_System_Byte.equals(typeName))
return Byte.class;
else if (Net_sbyte.equals(typeName))
return Byte.class;
else if (Net_System_SByte.equals(typeName))
return Byte.class;
else if (Net_System_Boolean.equals(typeName))
{
return Boolean.class;
}
else if (Java_Lang_Boolean.equals(typeName))
return Boolean.class;
else if (Java_Lang_Byte.equals(typeName))
{
return Byte.class;
}
else if (Net_char.equals(typeName))
return Character.class;
else if (Net_short.equals(typeName))
return Short.class;
else if (Net_ushort.equals(typeName))
return Short.class;
else if (Net_System_Int16.equals(typeName))
return Short.class;
else if (Net_System_UInt16.equals(typeName))
return Short.class;
else if (Net_System_Char.equals(typeName))
{
return Character.class;
}
else if (Java_Lang_Character.equals(typeName))
return Character.class;
else if (Java_Lang_Float.equals(typeName))
return Float.class;
else if (Java_Lang_Short.equals(typeName))
{
return Short.class;
}
else if (Net_float.equals(typeName))
return Float.class;
else if (Net_int.equals(typeName))
return Integer.class;
else if (Net_System_Int32.equals(typeName))
return Integer.class;
else if (Net_uint.equals(typeName))
return Integer.class;
else if (Net_System_UInt32.equals(typeName))
return Integer.class;
else if (Net_System_Single.equals(typeName))
{
return Float.class;
}
else if (Java_Lang_Integer.equals(typeName))
{
return Integer.class;
}
else if (Net_double.equals(typeName))
return Double.class;
else if (Net_System_Double.equals(typeName))
return Double.class;
else if (Net_long.equals(typeName))
return Long.class;
else if (Net_System_Int64.equals(typeName))
return Long.class;
else if (Net_ulong.equals(typeName))
return Long.class;
else if (Net_System_DateTime.equals(typeName))
return java.util.Date.class;
else if (Net_SystemUInt64.equals(typeName))
{
return Long.class;
}
else if (Java_Lang_Double.equals(typeName))
return Double.class;
else if (Java_Lang_Long.equals(typeName))
return Long.class;
else if (Java_Util_Date.equals(typeName))
{
return java.util.Date.class;
}
else if (Net_decimal.equals(typeName))
return java.math.BigDecimal.class;
else if (Net_System_Decimal.equals(typeName))
return java.math.BigDecimal.class;
else if (Java_Match_BigDecimal.equals(typeName))
return java.math.BigDecimal.class;
else
{
return null;
}
}
public static int getInMemoryInstanceSize(int actualDataBytes)
{
int temp = MemoryUtil.NetClassOverHead;
short remainder = (short)(actualDataBytes & 7);
if (remainder != 0)
remainder = (short)(8 - remainder);
temp += actualDataBytes + remainder;
return temp;
}
public static long getInMemoryInstanceSize(long actualDataBytes)
{
long temp = MemoryUtil.NetClassOverHead;
short remainder = (short)(actualDataBytes & 7);
if (remainder != 0)
remainder = (short)(8 - remainder);
temp += actualDataBytes + remainder;
return temp;
}
/**
Returns .Net's LOH safe generic collection count...
@param length
@return
*/
public static int getSafeByteCollectionCount(long length)
{
return ((length > 81920) ? 81920 : (int)length);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy