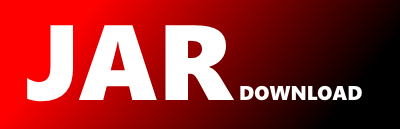
Alachisoft.NCache.Common.Net.Helper Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package Alachisoft.NCache.Common.Net;
import Alachisoft.NCache.Common.Common;
import java.io.IOException;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.ServerSocket;
/**
* @author Muneeb Shahid
*/
public class Helper {
public static int getFreeTCPPort(InetAddress ipAddress) {
ServerSocket ss = null;
try {
ss = new ServerSocket(0);
return ss.getLocalPort();
} catch (IOException iOE) {
} finally {
if (ss != null) {
try {
ss.close();
} catch (IOException e) {
}
}
}
return 0;
}
public static int getFreePort(InetAddress ipAddress) {
int port = getFreeTCPPort(ipAddress);
int retry = 20;
while (retry > 0) {
if (isUDPPortFree(port, ipAddress)) {
return port;
}
retry -= 1;
}
return 0;
}
public static boolean isTCPPortFree(int port, InetAddress addr) {
ServerSocket ss = null;
try {
ss = new ServerSocket(port);
ss.setReuseAddress(true);
return true;
} catch (IOException io) {
} finally {
if (ss != null) {
try {
ss.close();
} catch (IOException e) {
}
}
}
return false;
}
public static boolean isUDPPortFree(int port, InetAddress addr) {
DatagramSocket ds = null;
try {
ds = new DatagramSocket(port, addr);
ds.setReuseAddress(true);
return true;
} catch (IOException io) {
} finally {
if (ds != null) {
ds.close();
}
}
return false;
}
/**
* @param port
* @param addr
* @return true if port is not being used by any TCP and UDP Socket.
*/
public static boolean isPortFree(int port, InetAddress addr) {
return isTCPPortFree(port, addr) && isUDPPortFree(port, addr);
}
public static java.net.InetAddress getFirstNonLoopbackAddress() {
try {
java.util.Enumeration networkInterfaces = NetworkInterface.getNetworkInterfaces();
while (networkInterfaces.hasMoreElements()) {
NetworkInterface networkdInterface = (NetworkInterface) networkInterfaces.nextElement();
java.util.Enumeration inetAddresses = networkdInterface.getInetAddresses();
while (inetAddresses.hasMoreElements()) {
java.net.InetAddress address = (java.net.InetAddress) inetAddresses.nextElement();
if (address.isLoopbackAddress()) {
continue;
}
if (Common.is(address, java.net.Inet4Address.class)) {
return address;
}
}
}
} catch (IOException iOException) {
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy