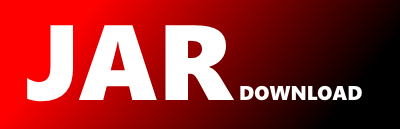
Alachisoft.NCache.Common.Net.NetworkData Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package Alachisoft.NCache.Common.Net;
/**
* @author taimoor_haider
*/
import com.alachisoft.ncache.common.caching.statistics.customcounters.systemcounters.SystemCounter;
import org.hyperic.sigar.*;
import java.io.BufferedInputStream;
import java.io.InputStream;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author taimoor_haider
*/
public class NetworkData {
static Map rxCurrentMap = new HashMap();
static Map> rxChangeMap = new HashMap>();
static Map txCurrentMap = new HashMap();
static Map> txChangeMap = new HashMap>();
private static Map filteredIP = new HashMap();
private static Map filteredSpeed = new HashMap();
private static boolean enabled = true;
/**
* @throws InterruptedException
*
*/
public static void registerIPToMonitor(String ip) {
if (SystemCounter.getSigar() == null)
return;
if (ip != null && !ip.isEmpty()) {
if (enabled) {
try {
for (String ni : SystemCounter.getSigar().getNetInterfaceList()) {
NetInterfaceConfig ifConfig = null;
try {
ifConfig = SystemCounter.getSigar().getNetInterfaceConfig(ni);
} catch (SigarException ex) {
Logger.getLogger(NetworkData.class.getName()).log(Level.SEVERE, null, ex);
}
if (ip != null && ip.equals(ifConfig.getAddress())) {
synchronized (filteredIP) {
if (!filteredIP.containsKey(ip)) {
filteredIP.put(ip, ni);
}
}
}
}
} catch (Throwable exc) {
enabled = false;
//System.err.println("JvCache unable to fetch network usage: Siagar Native libraries not found, please provide java.library.path to JVM pointing to TG_HOME/lib/resources/sigar");
}
}
}
}
/*
* Gets the network usage of registered NIC's. Call registerIPToMonitor before
* calling this function.
*/
public static double getNetworkUsage() throws SigarException {
if (SystemCounter.getSigar() == null)
return 0;
double speed = 1;
double totalUsage = -1;
for (String ni : filteredIP.values()) {
// System.out.println(ni);
NetInterfaceStat netStat = SystemCounter.getSigar().getNetInterfaceStat(ni);
NetInterfaceConfig ifConfig = SystemCounter.getSigar().getNetInterfaceConfig(ni);
String hwaddr = null;
speed = netStat.getSpeed();
if (speed == -1) {
if (!filteredSpeed.containsKey(ni)) {
filteredSpeed.put(ni, getNICLinuxSpeed());
}
speed = filteredSpeed.get(ni);
}
if (!NetFlags.NULL_HWADDR.equals(ifConfig.getHwaddr())) {
hwaddr = ifConfig.getHwaddr();
}
if (hwaddr != null) {
double rxCurrenttmp = netStat.getRxBytes();
saveChange(rxCurrentMap, rxChangeMap, hwaddr, (long) rxCurrenttmp, ni);
double txCurrenttmp = netStat.getTxBytes();
saveChange(txCurrentMap, txChangeMap, hwaddr, (long) txCurrenttmp, ni);
long totalrxDown = getMetricData(rxChangeMap);
long totaltxUp = getMetricData(txChangeMap);
for (List l : rxChangeMap.values()) {
l.clear();
}
for (List l : txChangeMap.values()) {
l.clear();
}
Sigar.formatSize(totaltxUp);
double usageForCurrentNIC = 0;
if (speed != 0) {
usageForCurrentNIC = (((totalrxDown + totaltxUp) * 8) / speed) * 100;
} else {
usageForCurrentNIC = 0;
}
if (totalUsage == -1) {
totalUsage = usageForCurrentNIC;
} else {
totalUsage = (totalUsage + usageForCurrentNIC) / 2;
}
}
}
if (totalUsage == -1) {
totalUsage = 0;
}
if (totalUsage > 100) {
totalUsage = 100;
}
return totalUsage;
}
public static int getNICLinuxSpeed() {
try {
String[] cmd =
{
"/bin/sh",
"-c",
"ethtool em1 2>/dev/null | grep Speed"
};
Process p = Runtime.getRuntime().exec(cmd);
InputStream stdoutStream = new BufferedInputStream(p.getInputStream());
StringBuffer buffer = new StringBuffer();
for (; ; ) {
int c = stdoutStream.read();
if (c == -1) {
break;
}
buffer.append((char) c);
}
String outputText = buffer.toString();
stdoutStream.close();
//Brute Force with regex
outputText = outputText.split(":")[1];
String number = outputText.replaceAll("[^0-9]", "");
String rate = outputText.trim().replace(number, "");
char metric = rate.charAt(0);
int mult = 1;
if (metric == 'M')
mult = 1000 * 1000;
else if (metric == 'G')
mult = 1000 * 1000 * 1000;
else if (metric == 'T')
mult = 1000 * 1000 * 1000 * 1000;
return Integer.parseInt(number) * mult;
} catch (Exception ex) {
return 0;
}
}
public static String getDefaultGateway() throws SigarException {
if (SystemCounter.getSigar() == null)
return "";
return SystemCounter.getSigar().getNetInfo().getDefaultGateway();
}
private static long getMetricData(Map> rxChangeMap) {
long total = 0;
for (Entry> entry : rxChangeMap.entrySet()) {
int average = 0;
for (Long l : entry.getValue()) {
average += l;
}
total += average / entry.getValue().size();
}
return total;
}
private static void saveChange(Map currentMap, Map> changeMap, String hwaddr, long current, String ni) {
Long oldCurrent = currentMap.get(ni);
if (oldCurrent != null) {
List list = changeMap.get(hwaddr);
if (list == null) {
list = new LinkedList();
changeMap.put(hwaddr, list);
}
list.add((current - oldCurrent));
}
currentMap.put(ni, current);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy