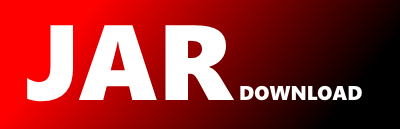
Alachisoft.NCache.Common.PortPool Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package Alachisoft.NCache.Common;
import java.net.InetAddress;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* @author Basit Anwer
*/
public class PortPool {
private static PortPool defaultPool = null;
private static String serverType = "";
int jmx = -1;
int snmp = -1;
int snmpPortPool = -1;
int jmxPortPool = -1;
int snmpClient = -1;
Map snmpPool = Collections.synchronizedMap(new HashMap());
Map jmxPool = Collections.synchronizedMap(new HashMap());
PortPool() {
if (serverType.equals("CacheServer")) {
if (ServicePropValues.SNMP_PORT != null) {
snmp = Integer.parseInt(ServicePropValues.SNMP_PORT);
}
if (ServicePropValues.JMXServer_PORT != null) {
jmx = Integer.parseInt(ServicePropValues.JMXServer_PORT);
}
if (ServicePropValues.SNMP_POOL != null) {
snmpPortPool = Integer.parseInt(ServicePropValues.SNMP_POOL);
} else {
snmpPortPool = 10100;
}
if (ServicePropValues.JMX__POOL != null) {
jmxPortPool = Integer.parseInt(ServicePropValues.JMX__POOL);
} else {
jmxPortPool = snmpPortPool + 5000;
}
} else {
if (BridgeServicePropValues.getJvBRIDGE_SNMP_PORT() != null) {
snmp = Integer.parseInt(BridgeServicePropValues.getJvBRIDGE_SNMP_PORT());
}
if (BridgeServicePropValues.getJvBRIDGE_JMXServer_PORT() != null) {
jmx = Integer.parseInt(BridgeServicePropValues.getJvBRIDGE_JMXServer_PORT());
}
if (BridgeServicePropValues.getJvBRIDGE_SNMP_POOL() != null) {
snmpPortPool = Integer.parseInt(BridgeServicePropValues.getJvBRIDGE_SNMP_POOL());
} else {
snmpPortPool = 20100;
}
if (BridgeServicePropValues.getJvBRIDGE_JMX__POOL() != null) {
jmxPortPool = Integer.parseInt(BridgeServicePropValues.getJvBRIDGE_JMX__POOL());
} else {
jmxPortPool = snmpPortPool + 5000;
}
}
}
public static void setServerType(String serverType) {
PortPool.serverType = serverType;
}
public static PortPool getInstance() {
if (defaultPool == null) {
defaultPool = new PortPool();
}
return defaultPool;
}
public int getNextSNMPPort(String cacheName, InetAddress addr) {
InetAddress address = addr == null ? ServicePropValues.getStatsServerIp() : addr;
if (snmpPool.containsKey(cacheName)) {
return (Integer) snmpPool.get(cacheName);
} else {
while (snmpPortPool < jmxPortPool) {
if (!snmpPool.containsValue(snmpPortPool) && Alachisoft.NCache.Common.Net.Helper.isPortFree(snmpPortPool, address)) {
snmpPool.put(cacheName, snmpPortPool);
snmpPortPool++;
return snmpPortPool - 1;
} else {
snmpPortPool++;
}
}
//should not happen
return 0;
}
}
/**
* Selects available port, returns the value if cache registered then returns the same already assigned port SnmpdPort will be less than jmx port.
*
* @param cacheName CacheName
* @return port for snmp, will reuse ports.
*/
public int getNextSNMPPort(String cacheName) {
return getNextSNMPPort(cacheName, null);
}
/**
* Will update pool
*
* @param cacheName
* @param port
* @throws IllegalArgumentException Throws it when either cache is already present or port is already in use
*/
public void assignPort(String cacheName, int port) {
if (!snmpPool.containsKey(cacheName) && !snmpPool.containsValue(port)) {
snmpPool.put(cacheName, port);
} else {
throw new IllegalArgumentException("Either " + cacheName + " already exists in pool or port " + port + " respecified");
}
}
/**
* Frees the port number to be reusable
*
* @param cacheName
*/
public void disposeSNMPPort(String cacheName) {
if (snmpPool.containsKey(cacheName)) {
snmpPortPool = snmpPool.get(cacheName);
}
snmpPool.remove(cacheName);
}
public Map getSNMPMap() {
return Collections.unmodifiableMap(snmpPool);
}
public Integer getSNMPPort(String cacheName) {
return snmpPool.get(cacheName);
}
public int getJMXPort() {
return getJMXPort(null);
}
public int getJMXPort(InetAddress addr) {
InetAddress address = addr == null ? ServicePropValues.getStatsServerIp() : addr;
if (jmx != -1 && Alachisoft.NCache.Common.Net.Helper.isPortFree(jmx, address)) {
return jmx;
}
return Alachisoft.NCache.Common.Net.Helper.getFreePort(address);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy