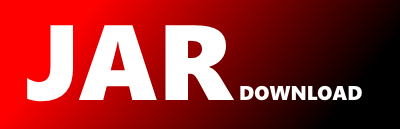
Alachisoft.NCache.Common.RegHelper Maven / Gradle / Ivy
package Alachisoft.NCache.Common;
/**
* Class that helps do common registry operations
*/
public class RegHelper {
// public static final String ROOT_KEY = "Software\\Alachisoft\\NCache";
// public static final String APPBASE_KEY = "\\NCache Manager";
// public static final String REMOTEAUTH_KEY = "Software\\Alachisoft\\NCache\\NCMonitor\\";
// public static final String MANAGER_OPTIONS = APPBASE_KEY + "\\Options";
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
////#if NCWOW64
// public static final String MONITOR_ROWS_BASE = "NCache Manager\\Options";
////#else
// public static final String MONITOR_ROWS_BASE = RegHelper.ROOT_KEY + RegHelper.MANAGER_OPTIONS;
////#endif
//
//
// public static RegistryKey NewKey(String keyPath, String key) {
// return Registry.LocalMachine.CreateSubKey(keyPath + key);
// }
//
// /**
// Get a key value from the registry.
//
// @param section
// @param key
// @return
// */
// public static Object GetDecryptedRegValue(String keypath, String key, short prodId) {
// try {
// Object val = GetRegValue(keypath, key, prodId);
// return Protector.DecryptString(String.valueOf(val));
// } catch (RuntimeException e) {
// return null;
// }
// }
//
// /**
// Save a registry value.
//
// @param section
// @param key
// @param val
// */
// public static void SetEncryptedRegValue(String keypath, String key, Object val) {
// try {
// SetRegValue(keypath, key, Protector.EncryptString(String.valueOf(val)), 0);
// } catch (RuntimeException e) {
// }
// }
//
// /**
// Get a key value from the registry. Automatically caters with wow64 registry read mechanism
//
// @param section
// @param key
// @return
// */
// public static Object GetRegValue(String section, String key, short prodId) {
/////#if NCWOW64 //+Asad if running as Wow64 then redirect for registry
// if (AppUtil.getIsRunningAsWow64()) {
// return GetRegValueInternal(section, key, prodId);
// }
/////#else
// try {
// RegistryKey root = Registry.LocalMachine.OpenSubKey(section);
// return root.GetValue(key);
// } catch (RuntimeException ex) {
// return null;
// }
/////#endif
// }
//
// public static String GetLicenseKey(short prodId) {
// return (String)RegHelper.GetRegValueInternal("UserInfo", "licensekey", prodId);
// }
//
// /**
// Get a key value from the registry.
//
// @param section
// @param key
// @return
// */
// public static int GetRegValues(String keypath, java.util.HashMap ht, short prodId) {
// RegistryKey root;
/////#if NCWOW64
// if (AppUtil.getIsRunningAsWow64()) {
// GetRegValuesInternalWow64(keypath, ht, prodId);
// return ht.size();
// }
/////#else
// try {
// root = Registry.LocalMachine.OpenSubKey(keypath);
// String[] keys = root.GetValueNames();
// for (int i = 0; i < keys.length; i++) {
// ht.put(keys[i], GetRegValue(keypath, keys[i], prodId));
// }
// } catch (RuntimeException e) {
// }
// return ht.size();
/////#endif
// }
//
// /**
// Get a key value from the registry.
//
// @param section
// @param key
// @return
// */
// public static int GetBooleanRegValues(String keypath, java.util.HashMap ht, short prodId) {
/////#if NCWOW64
// if (AppUtil.getIsRunningAsWow64()) {
// GetBooleanRegValuesInternalWow64(keypath, ht, prodId);
// return ht.size();
// }
/////#else
//
//RegistryKey root;
// try {
// root = Registry.LocalMachine.OpenSubKey(keypath);
// String[] keys = root.GetValueNames();
// for (int i = 0; i < keys.length; i++) {
// ht.put(keys[i], (Boolean)GetRegValue(keypath, keys[i], prodId));
// }
// } catch (RuntimeException e) {
// }
// return ht.size();
/////#endif
// }
//
// /**
// Save a registry value.
//
// @param section
// @param key
// @param val
// */
// public static void SetRegValue(String keypath, String key, Object val, short prodId) {
// try {
// RegistryKey root = Registry.LocalMachine.OpenSubKey(keypath, true);
// root.SetValue(key, val);
// } catch (RuntimeException e) {
//
// }
// }
//
// /**
// Save a registry value.
//
// @param section
// @param key
// @param val
// */
// public static void SetRegValues(String keypath, java.util.HashMap val, short prodId) {
// try {
// IDictionaryEnumerator ie = val.entrySet().iterator();
// while (ie.MoveNext()) {
// SetRegValue(keypath, String.valueOf(ie.Key), ie.Value, prodId);
// }
// } catch (RuntimeException e) {
// }
// }
//
// private static String GetRegValueInternal(String section, String key, short prodId) {
// try {
// StringBuilder regVal = new StringBuilder(500);
// StringBuilder sbSection = new StringBuilder(section);
// StringBuilder sbKey = new StringBuilder(key);
// StringBuilder sbDefaultVal = new StringBuilder("");
// NCLicenseDll.GetRegVal(regVal, sbSection, sbKey, sbDefaultVal,prodId);
// return regVal.toString();
// } catch (RuntimeException ex) {
// AppUtil.LogEvent("RegHelper::Stage 001" + ex.getMessage() + ex.getCause().getMessage(), EventLogEntryType.Error);
// }
// return "";
// }
//
// private static void GetRegValuesInternalWow64(String keypath, java.util.HashMap ht, short prodId) { //Added by Asif Imam Aug-08,08
// try {
// StringBuilder regVal = new StringBuilder(2048);
// StringBuilder sbSection = new StringBuilder(keypath);
// StringBuilder sbKey = new StringBuilder("");
// StringBuilder sbDefaultVal = new StringBuilder("");
// NCLicenseDll.GetRegKeys(regVal, sbSection, sbKey, sbDefaultVal,prodId);
//
// String keys = regVal.toString();
// String[] statKeys = keys.split("[:]", -1);
//
// for (int i = 0; i < statKeys.length; i++) {
// String subKey = statKeys[i];
// if (!tangible.DotNetToJavaStringHelper.isNullOrEmpty(subKey)) {
// String result = GetRegValueInternal(keypath, subKey, prodId);
// ht.put(statKeys[i], result);
// }
// }
// } catch (RuntimeException ex) {
// AppUtil.LogEvent("RegHelper::Stage 002" + ex.getMessage() + ex.getCause().getMessage(), EventLogEntryType.Error);
// }
// }
//
// private static void GetBooleanRegValuesInternalWow64(String keypath, java.util.HashMap ht, short prodId) { //Added by Asif Imam Aug-08,08
// try {
// StringBuilder regVal = new StringBuilder(2048);
// StringBuilder sbSection = new StringBuilder(keypath);
// StringBuilder sbKey = new StringBuilder("");
// StringBuilder sbDefaultVal = new StringBuilder("");
// NCLicenseDll.GetRegKeys(regVal, sbSection, sbKey, sbDefaultVal,prodId);
//
// String keys = regVal.toString();
// String[] statKeys = keys.split("[:]", -1);
//
// for (int i = 0; i < statKeys.length; i++) {
// String subKey = statKeys[i];
// if (!tangible.DotNetToJavaStringHelper.isNullOrEmpty(subKey)) {
// String result = GetRegValueInternal(keypath, subKey, prodId);
// ht.put(statKeys[i], Boolean.parseBoolean(result));
// }
// }
// } catch (RuntimeException ex) {
// AppUtil.LogEvent("RegHelper::Stage 003" + ex.getMessage() + ex.getCause().getMessage(), EventLogEntryType.Error);
// }
// }
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
////#if NCWOW64
// private static boolean SetRegValueInternal(String section, String key, String newVal, short prodId) {
// StringBuilder sbSection = new StringBuilder(section);
// StringBuilder sbKey = new StringBuilder(key);
// StringBuilder sbNewVal = new StringBuilder(newVal);
// return NCLicenseDll.SetRegVal(sbSection, sbKey, sbNewVal,prodId);
// }
//
////C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
////ORIGINAL LINE: private static bool SetRegValueInternal(string section, string key, ulong newVal, short prodId)
// private static boolean SetRegValueInternal(String section, String key, long newVal, short prodId) {
// StringBuilder sbSection = new StringBuilder(section);
// StringBuilder sbKey = new StringBuilder(key);
// return NCLicenseDll.SetRegValInt(sbSection, sbKey, newVal, prodId);
// }
////#endif
// /*
// [CLSCompliant(false)]
// static private bool SetRegValueInternal(string section, string key, ulong newVal,short prodId)
// {
// StringBuilder sbSection = new StringBuilder(section);
// StringBuilder sbKey = new StringBuilder(key);
// return NCLicenseDll.SetRegValInt(sbSection, sbKey, (long)newVal,prodId);
// }
// */
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy