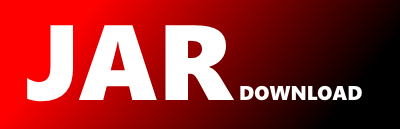
Alachisoft.NCache.Common.Remoting.RemotingChannels Maven / Gradle / Ivy
package Alachisoft.NCache.Common.Remoting;
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
//#if VS2005
//#endif
/**
* Summary description for NCacheChannels.
*/
//public class RemotingChannels
//{
// private IChannel _tcpChannel;
// private IChannel _httpChannel;
// public RemotingChannels()
// {
// //
// // TODO: Add constructor logic here
// //
// }
// ///
// ///
// ///
// ///
// ///
// public void RegisterTcpChannels(string channelName, int port)
// {
// _tcpChannel = ChannelServices.GetChannel(channelName);
// if (_tcpChannel == null)
// {
// IDictionary properties = new Hashtable();
// properties["name"] = channelName;
// //if(port > 0)
// {
// properties["port"] = port;
// }
// BinaryClientFormatterSinkProvider cprovider = new BinaryClientFormatterSinkProvider();
// BinaryServerFormatterSinkProvider sprovider = new BinaryServerFormatterSinkProvider();
// sprovider.TypeFilterLevel = TypeFilterLevel.Full;
// _tcpChannel = new TcpChannel(properties, cprovider, sprovider);
// ChannelServices.RegisterChannel(_tcpChannel);
// }
// }
// ///
// /// Registers the Http channel for objects.
// ///
// public void RegisterHttpChannels(string channelName, int port)
// {
// _httpChannel = ChannelServices.GetChannel(channelName);
// if (_httpChannel == null)
// {
// IDictionary properties = new Hashtable();
// properties["name"] = channelName;
// //if(port > 0)
// {
// properties["port"] = port;
// }
// BinaryClientFormatterSinkProvider cprovider = new BinaryClientFormatterSinkProvider();
// BinaryServerFormatterSinkProvider sprovider = new BinaryServerFormatterSinkProvider();
// sprovider.TypeFilterLevel = TypeFilterLevel.Full;
// _httpChannel = new HttpChannel(properties, cprovider, sprovider);
// ChannelServices.RegisterChannel(_httpChannel);
// }
// }
// ///
// /// Stop this service.
// ///
// public void UnregisterTcpChannels()
// {
// try
// {
// if (_tcpChannel != null)
// {
// ChannelServices.UnregisterChannel(_tcpChannel);
// _tcpChannel = null;
// }
// }
// catch (Exception)
// {
// throw;
// }
// }
// ///
// /// Stop this service.
// ///
// public void UnregisterHttpChannels()
// {
// try
// {
// if (_httpChannel != null)
// {
// ChannelServices.UnregisterChannel(_httpChannel);
// _httpChannel = null;
// }
// }
// catch (Exception)
// {
// throw;
// }
// }
//}
/**
Summary description for NCacheChannels.
*/
public class RemotingChannels {
// /** The underlying TCP server channel.
// */
// private IChannel _tcpServerChannel;
// /** The underlying TCP client channel.
// */
// private IChannel _tcpClientChannel;
// /** The underlying HTTP server channel.
// */
// private IChannel _httpServerChannel;
// /** The underlying HTTP client channel.
// */
// private IChannel _httpClientChannel;
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
////#if VS2005
// /** The underlying IPO Server channel.
// */
// private IChannel _ipcServerChannel;
// /** The underlying IPC client channel.
// */
// private IChannel _ipcClientChannel;
////#endif
// public RemotingChannels() {
// //
// // TODO: Add constructor logic here
// //
// }
//
// /** Returns the underlying TCP server channel.
// */
// public final IChannel getTcpServerChannel() {
// return _tcpServerChannel;
// }
// /** Returns the underlying TCP client channel.
// */
// public final IChannel getTcpClientChannel() {
// return _tcpClientChannel;
// }
// /** Returns the underlying HTTP server channel.
// */
// public final IChannel getHttpServerChannel() {
// return _httpServerChannel;
// }
// /** Returns the underlying HTTP client channel.
// */
// public final IChannel getHttpClientChannel() {
// return _httpClientChannel;
// }
//
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region / --- TcpChannel --- /
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterTcpChannels(String channelName, int port) {
// RegisterTcpServerChannel(String.Concat(channelName, ".s"), port);
// RegisterTcpClientChannel(String.Concat(channelName, ".c"));
// }
//
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterTcpChannels(String channelName, String ip, int port) {
// RegisterTcpServerChannel(String.Concat(channelName, ".s"), ip, port);
// RegisterTcpClientChannel(String.Concat(channelName, ".c"));
//
//
// }
//
//
// /**
// Stop this service.
// */
// public final void UnregisterTcpChannels() {
// UnregisterTcpServerChannel();
// UnregisterTcpClientChannel();
// }
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterTcpServerChannel(String channelName, int port) {
// _tcpServerChannel = ChannelServices.GetChannel(channelName);
// if (_tcpServerChannel == null) {
// BinaryServerFormatterSinkProvider sprovider = new BinaryServerFormatterSinkProvider();
// sprovider.TypeFilterLevel = TypeFilterLevel.Full;
// _tcpServerChannel = new TcpServerChannel(channelName, port, sprovider);
// ChannelServices.RegisterChannel(_tcpServerChannel);
// }
// }
//
// public final void RegisterTcpServerChannel(String channelName, String ip, int port) {
// try {
// _tcpServerChannel = ChannelServices.GetChannel(channelName);
// if (_tcpServerChannel == null) {
// java.util.Map properties = new java.util.Hashtable();
// properties.put("name", channelName);
// //if(port > 0)
// {
// properties.put("port", port);
// properties.put("bindTo", ip);
// }
// BinaryServerFormatterSinkProvider sprovider = new BinaryServerFormatterSinkProvider();
// sprovider.TypeFilterLevel = TypeFilterLevel.Full;
// _tcpServerChannel = new TcpServerChannel(properties, sprovider);
// ChannelServices.RegisterChannel(_tcpServerChannel);
// }
// } catch (System.Net.Sockets.SocketException se) {
// switch (se.ErrorCode) {
// // 10049 --> address not available.
// case 10049:
// throw new RuntimeException("The address " + ip + " specified for NCacheServer.BindToClusterIP is not valid");
//
// default:
// throw se;
// }
// }
// }
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterTcpClientChannel(String channelName) {
// _tcpClientChannel = ChannelServices.GetChannel(channelName);
// if (_tcpClientChannel == null) {
// BinaryClientFormatterSinkProvider cprovider = new BinaryClientFormatterSinkProvider();
// _tcpClientChannel = new TcpClientChannel(channelName, cprovider);
// ChannelServices.RegisterChannel(_tcpClientChannel);
// }
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterTcpServerChannel() {
// if (_tcpServerChannel != null) {
// ChannelServices.UnregisterChannel(_tcpServerChannel);
// _tcpServerChannel = null;
// }
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterTcpClientChannel() {
// if (_tcpClientChannel != null) {
// ChannelServices.UnregisterChannel(_tcpClientChannel);
// _tcpClientChannel = null;
// }
// }
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region / --- HttpChannel --- /
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterHttpChannels(String channelName, int port) {
// RegisterHttpServerChannel(String.Concat(channelName, ".sh"), port);
// RegisterHttpClientChannel(String.Concat(channelName, ".ch"));
// }
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterHttpChannels(String channelName, String ip, int port) {
// RegisterHttpServerChannel(String.Concat(channelName, ".sh"), ip, port);
// RegisterHttpClientChannel(String.Concat(channelName, ".ch"));
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterHttpChannels() {
// UnregisterHttpServerChannel();
// UnregisterHttpClientChannel();
// }
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterHttpServerChannel(String channelName, int port) {
// _httpServerChannel = ChannelServices.GetChannel(channelName);
// if (_httpServerChannel == null) {
// BinaryServerFormatterSinkProvider sprovider = new BinaryServerFormatterSinkProvider();
// sprovider.TypeFilterLevel = TypeFilterLevel.Full;
// _httpServerChannel = new HttpServerChannel(channelName, port, sprovider);
// ChannelServices.RegisterChannel(_httpServerChannel);
// }
// }
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterHttpServerChannel(String channelName, String ip, int port) {
// try {
// _httpServerChannel = ChannelServices.GetChannel(channelName);
// if (_httpServerChannel == null) {
// java.util.Map properties = new java.util.Hashtable();
// properties.put("name", channelName);
// //if(port > 0)
// {
// properties.put("port", port);
// properties.put("bindTo", ip);
// }
// BinaryServerFormatterSinkProvider sprovider = new BinaryServerFormatterSinkProvider();
// sprovider.TypeFilterLevel = TypeFilterLevel.Full;
// _httpServerChannel = new HttpServerChannel(properties, sprovider);
// ChannelServices.RegisterChannel(_httpServerChannel);
// }
// } catch (System.Net.Sockets.SocketException se) {
// switch (se.ErrorCode) {
// // 10049 --> address not available.
// case 10049:
// throw new RuntimeException("The address " + ip + " specified for NCacheServer.BindToClusterIP is not valid");
//
// default:
// throw se;
// }
// }
// }
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterHttpClientChannel(String channelName) {
// _httpClientChannel = ChannelServices.GetChannel(channelName);
// if (_httpClientChannel == null) {
// BinaryClientFormatterSinkProvider cprovider = new BinaryClientFormatterSinkProvider();
// _httpClientChannel = new HttpClientChannel(channelName, cprovider);
// ChannelServices.RegisterChannel(_httpClientChannel);
// }
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterHttpServerChannel() {
// if (_httpServerChannel != null) {
// ChannelServices.UnregisterChannel(_httpServerChannel);
// _httpServerChannel = null;
// }
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterHttpClientChannel() {
// if (_httpClientChannel != null) {
// ChannelServices.UnregisterChannel(_httpClientChannel);
// _httpClientChannel = null;
// }
// }
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
////#if VS2005
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region / --- IPCChannel --- /
//
// /**
//
//
// @param channelName
// @param port
// */
// public final void RegisterIPCChannels(String channelName, String portName) {
// RegisterIPCServerChannel(String.Concat(channelName, ".s"), portName);
// RegisterIPCClientChannel(String.Concat(channelName, ".c"));
// AppUtil.LogEvent("IPC channel registered at port: " + portName, System.Diagnostics.EventLogEntryType.Information);
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterIPCChannels() {
// UnregisterTcpServerChannel();
// UnregisterTcpClientChannel();
// }
//
// /**
// Registers IPC server channel.
//
// @param channelName
// @param port
// */
// public final void RegisterIPCServerChannel(String channelName, String portName) {
// _ipcServerChannel = ChannelServices.GetChannel(channelName);
// if (_ipcServerChannel == null) {
// BinaryServerFormatterSinkProvider sprovider = new BinaryServerFormatterSinkProvider();
// sprovider.TypeFilterLevel = TypeFilterLevel.Full;
// _ipcServerChannel = new IpcServerChannel(channelName,portName, sprovider);
// ;
// ChannelServices.RegisterChannel(_ipcServerChannel);
// }
// }
//
// /**
// Registers IPC Client Channel.
//
// @param channelName
// @param port
// */
// public final void RegisterIPCClientChannel(String channelName) {
// _ipcClientChannel = ChannelServices.GetChannel(channelName);
// if (_ipcClientChannel == null) {
// BinaryClientFormatterSinkProvider cprovider = new BinaryClientFormatterSinkProvider();
// _ipcClientChannel = new IpcClientChannel(channelName, cprovider);
// ChannelServices.RegisterChannel(_ipcClientChannel);
// }
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterIPCServerChannel() {
// if (_ipcServerChannel != null) {
// ChannelServices.UnregisterChannel(_ipcServerChannel);
// _ipcServerChannel = null;
// }
// }
//
// /**
// Stop this service.
// */
// public final void UnregisterIPCClientChannel() {
// if (_ipcClientChannel != null) {
// ChannelServices.UnregisterChannel(_ipcClientChannel);
// _ipcClientChannel = null;
// }
// }
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
////#endif
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy