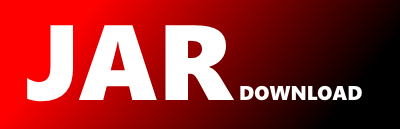
Alachisoft.NCache.Common.Threading.NotifyingThreadQueue Maven / Gradle / Ivy
package Alachisoft.NCache.Common.Threading;
import java.io.Serializable;
public class NotifyingThreadQueue implements Serializable {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region my events
//
// /**
// * signals when the start of the queue changes
// */
////C# TO JAVA CONVERTER TODO TASK: Events are not available in Java:
//// public event QueueStateChangedHandler QueueStateChanged;
// /**
// * signals when the current operation is finished processing
// */
////C# TO JAVA CONVERTER TODO TASK: Events are not available in Java:
//// public event ThreadFinishedHandler ThreadFinished;
// /**
// * signals when the current operation had an error OR when an enqueued item was removed from the queue prior to processing
// */
////C# TO JAVA CONVERTER TODO TASK: Events are not available in Java:
//// public event ThreadErrorHandler ThreadError;
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region my vars
// private Object syncobj;
// private Object _extraInfo;
// private int maxthreads;
// private int currentthreads;
// private QueueState qs = getQueueState().values()[0];
// private java.util.LinkedList>> queue;
// private QueueOperationHandler defaultop;
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region constructors
// /**
// * constructs the NotifyingThreadQueue. sets the state to QueueState.Idle
// *
// * @param defaultoperation the default operation to perform on an enqueued item
// * @exception System.IllegalArgumentException defaultoperation is null
// */
// public NotifyingThreadQueue(QueueOperationHandler defaultoperation)
// {
// QueueOperationHandler tempVar = new QueueOperationHandler()
// {
//
// @Override
// public void invoke(T item)
// {
// Integer.MAX_VALUE(item);
// }
// };
// QueueOperationHandler tempVar2 = new QueueOperationHandler()
// {
//
// @Override
// public void invoke(T item)
// {
// defaultoperation(item);
// }
// };
// this(tempVar, tempVar2);
// }
//
// /**
// * constructs the NotifyingThreadQueue. sets the state to QueueState.Idle
// *
// * @param defaultoperation the default operation to perform on an enqueued item
// * @exception System.IllegalArgumentException defaultoperation is null
// */
// public NotifyingThreadQueue(QueueOperationHandler defaultoperation, Object extraInfo)
// {
// QueueOperationHandler tempVar = new QueueOperationHandler()
// {
//
// @Override
// public void invoke(T item)
// {
// Integer.MAX_VALUE(item);
// }
// };
// QueueOperationHandler tempVar2 = new QueueOperationHandler()
// {
//
// @Override
// public void invoke(T item)
// {
// defaultoperation(item);
// }
// };
// this(tempVar, tempVar2);
// _extraInfo = extraInfo;
// }
//
// /**
// * constructs the NotifyingThreadQueue. sets the state to QueueState.Idle
// *
// * @param maxthreads sets the maximum number of simultaneous operations
// * @param defaultoperation the default operation to perform on an enqueued item
// * @exception System.ArgumentException maxthreads is less than or equal to 0
// * @exception System.IllegalArgumentException defaultoperation is null
// */
// public NotifyingThreadQueue(int maxthreads, QueueOperationHandler defaultoperation)
// {
// if (maxthreads <= 0)
// {
// throw new IllegalArgumentException("maxthreads can not be <= 0");
// }
// if (defaultoperation == null)
// {
// throw new IllegalArgumentException("defaultoperation can not be null");
// }
//
// this.qs = QueueState.Idle;
// this.syncobj = new Object();
// this.currentthreads = 0;
// this.maxthreads = maxthreads;
// this.queue = new java.util.LinkedList>>();
// this.defaultop = new QueueOperationHandler()
// {
//
// @Override
// public void invoke(T item)
// {
// defaultoperation(item);
// }
// };
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region Properties
// public final Object getExtraInfo()
// {
// return _extraInfo;
// }
//
// public final void setExtraInfo(Object value)
// {
// _extraInfo = value;
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region control ops
// /**
// * pauses the execution of future operations. the current operations are allowed to finish.
// */
// public final void Pause()
// {
// synchronized (syncobj)
// {
// if (qs == QueueState.Idle)
// {
// /**
// * this is a judgment call if you pause this when you don�t have any elements in it then you can go directly to paused and this means that you basically want to
// * keep queuing until something happens
// */
// qs = QueueState.Paused;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Idle, QueueState.Paused));
// }
// else if (qs == QueueState.Running)
// {
// qs = QueueState.Pauseing;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Running, QueueState.Pauseing));
//
// /**
// * running means you had some active threads so you couldn�t get to paused right away
// */
// }
// else if (qs == QueueState.Stopping)
// {
// ThreadErrorInternal(null, new ThreadStateException("Once the queue is stopping its done processing"));
// }
// /**
// * if we are already paused or pausing we dont need to do anything
// */
// }
// }
//
// /**
// * stops the execution of future operations. clears out all pending operations. no further operations are allowed to be enqueued. the current operations are allowed to finish.
// */
// public final void Stop()
// {
// synchronized (syncobj)
// {
// if ((qs == QueueState.Idle) || (qs == QueueState.Stopping))
// {
// /**
// * do nothing idle has nothing to stop and stopping is already working on stopping
// */
// return;
// }
// else if (qs == QueueState.Paused)
// {
// qs = QueueState.Stopping;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Paused, QueueState.Stopping));
//
// /**
// * if we are already paused then we have no threads running so just drop all the extra items in the queue
// */
// while (queue.size() != 0)
// {
// ThreadErrorInternal(queue.poll().Key, new ThreadStateException("the Queue is stopping . no processing done"));
// }
//
// /**
// * ensure proper event flow paused-> stopping -> idle
// */
// qs = QueueState.Idle;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Stopping, QueueState.Idle));
// }
// else
// {
// QueueState temState = this.getQueueState();
// qs = QueueState.Stopping;
// QueueStateChangedInternal(this, new QueueChangedState(temState, QueueState.Stopping));
//
// /**
// * why are we not dequeuing everything? that�s b/c if we have threads left they have to finish in their own good time so they can go through the process of getting
// * rid of all the others. both ways work
// */
// if (currentthreads == 0)
// {
// qs = QueueState.Idle;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Stopping, QueueState.Idle));
// }
// }
// }
// }
//
// /**
// * continues the execution of enqueued operations after a pause.
// */
// public final void Continue()
// {
// synchronized (syncobj)
// {
// if ((qs == QueueState.Pauseing) || (qs == QueueState.Paused))
// {
// qs = QueueState.Running;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Paused, QueueState.Running));
// //while (currentthreads < maxthreads)
// {
// TryNewThread();
// //Queuestate may have changed, so return if doing nothing
// //if (qs == QueueState.Idle || qs == QueueState.Paused) { return; }
// }
// }
// else if ((qs == QueueState.Idle) || (qs == QueueState.Running))
// {
// /**
// * Continuing to process while the queue is idle is meaning less just ignore the command
// */
// return;
// }
// else if (qs == QueueState.Stopping)
// {
// ThreadErrorInternal(null, new ThreadStateException("Once the queue is stopping its done processing"));
// }
// }
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region data axcessors
// /**
// * gets the current QueueState
// */
// public final QueueState getQueueState()
// {
// synchronized (syncobj)
// {
// return qs;
// }
// }
//
// /**
// * gets the maximum number of operations that can be executed at once
// */
// public final int getMaxThreads()
// {
// return maxthreads;
// }
//
// /**
// * gets the current number of current ongoing operations
// */
// public final int getCurrentRunningThreads()
// {
// synchronized (syncobj)
// {
// return currentthreads;
// }
//
// }
//
// public final int getCount()
// {
// return this.queue.size();
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region enque ops
// /**
// * Adds the item to the queue to process asynchronously.
// *
// * @param item the item to enqueue
// */
// public final void EnQueue(T item)
// {
// QueueOperationHandler tempVar = new QueueOperationHandler()
// {
//
// @Override
// public void invoke(T item)
// {
// defaultop(item);
// }
// };
// EnQueue(item, tempVar);
// }
//
// /**
// * Adds the item to the queue to process asynchronously and uses the different operation instead of the default.
// *
// * @param item the item to enqueue
// * @param opp the new operation that overrides the default
// * @exception System.IllegalArgumentException opp is null
// */
// public final void EnQueue(T item, QueueOperationHandler opp)
// {
// if (opp == null)
// {
// throw new IllegalArgumentException("operation can not be null");
// }
//
// synchronized (syncobj)
// {
// if (qs == QueueState.Idle)
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region idle
// qs = QueueState.Running;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Idle, QueueState.Running));
//
// /**
// * the problem with generics is that sometimes the fully descriptive name goes on for a while
// */
// java.util.Map.Entry> kvp = new KeyValuePair>(item, opp);
//
// /**
// * thread demands that its ParameterizedThreadStart take an object not a generic type one might have resonably thought that there would be a generic constructor
// * that took a strongly typed value but there is not one
// */
// currentthreads++;
// (new Thread(new ParameterizedThreadStart(RunOpp))).Start(kvp);
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// else if ((qs == QueueState.Paused) || (qs == QueueState.Pauseing))
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region pause
// /**
// * in the case that we are pausing or currently paused we just add the value to the queue we dont try to run the process
// */
// queue.offer(new KeyValuePair>(item, opp));
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// else if (qs == QueueState.Running)
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region running
// /**
// * you have to enqueue the item then try to execute the first item in the process always enqueue first as this ensures that you get the oldest item first since that
// * is what you wanted to do you did not want a stack
// */
// queue.offer(new KeyValuePair>(item, opp));
// TryNewThread();
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// else if (qs == QueueState.Stopping)
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region stopping
// /**
// * when you are stopping the queue i assume that you wanted to stop it not pause it this means that if you try to enqueue something it will throw an exception since
// * you shouldnt be enqueueing anything since when the queue gets done all its current threads it clears the rest out so why bother enqueueing it. at this point we
// * have a choice we can make the notifyer die or we can use the error event we already have built in to tell the sender. i chose the later. also try to pick an
// * appropriate exception not just the base
// */
// ThreadErrorInternal(item, new ThreadStateException("the Queue is stopping . no processing done"));
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// }
// }
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region tools
// private void RunOpp(Object o)
// {
// java.util.Map.Entry> kvp = (java.util.Map.Entry>) o;
//
// try
// {
// kvp.getValue()
// (kvp.getKey()
// );
// ThreadFinishedInternal(kvp.getKey());
// }
// catch (RuntimeException ex)
// {
// ThreadErrorInternal(kvp.getKey(), new ThreadStateException("error processing. partial processing done.", ex));
// }
// finally
// {
// synchronized (syncobj)
// {
// currentthreads--;
// }
// TryNewThread();
// }
// }
//
// private void TryNewThread()
// {
// synchronized (syncobj)
// {
// if (qs == QueueState.Running)
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region Running
// if (queue.size() != 0)
// {
// if (currentthreads < maxthreads)
// {
// currentthreads++;
// (new Thread(new ParameterizedThreadStart(RunOpp))).Start(queue.poll());
// }
// }
// else
// {
// if (currentthreads == 0)
// {
// qs = QueueState.Idle;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Running, QueueState.Idle));
// }
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// else if (qs == QueueState.Stopping)
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region stopping
// /**
// * normally when we stop a queue we can just clear out the remaining values and let the threads peter out. however, we made the decision to throw an exception by
// * way of our exception handler. it is therefore important to keep with that and get rid of all the queue items in that same way
// */
// while (queue.size() != 0)
// {
// ThreadErrorInternal(queue.poll().Key, new ThreadStateException("the Queue is stopping . no processing done"));
// }
//
// /**
// * all threads come through here so its up to us to single the change from stopping to idle
// */
// if (currentthreads == 0)
// {
// qs = QueueState.Idle;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Stopping, QueueState.Idle));
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// else if (qs == QueueState.Pauseing)
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region Pauseing
// if (currentthreads == 0)
// {
// qs = QueueState.Paused;
// QueueStateChangedInternal(this, new QueueChangedState(QueueState.Pauseing, QueueState.Paused));
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// else
// {
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region Idle / Paused
// /**
// * there should be no way to got in here while your idle or paused this is just an error check
// */
// ThreadErrorInternal(null, new RuntimeException("internal state bad"));
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
// }
// }
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
//
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#region event fowarders
// /**
// * during testing i found out that it is likely that the events will be updating controls and that this means that if care is not taken then the events throw exceptions this is
// * probably not desirable so i decided to fix this by just dumping these errors for you silently. this is a double edged sword though. if things are not appearing in your
// * controls this might be the culprit
// */
// private void QueueStateChangedInternal(Object sender, QueueChangedState qs)
// {
// try
// {
// if (QueueStateChanged != null)
// {
// QueueStateChanged(sender, qs);
// }
// }
// catch (UnsupportedOperationException ex)
// {
// if (ex.getMessage().startsWith("Cross-thread operation not valid"))
// {
// System.out.println(ex.getMessage());
// }
// else
// {
// throw ex;
// }
// }
// }
//
// private void ThreadFinishedInternal(T finisheditem)
// {
// try
// {
// if (ThreadFinished != null)
// {
// ThreadFinished(finisheditem);
// }
// }
// catch (UnsupportedOperationException ex)
// {
// if (ex.getMessage().startsWith("Cross-thread operation not valid"))
// {
// System.out.println(ex.getMessage());
// }
// else
// {
// throw ex;
// }
// }
// }
//
// private void ThreadErrorInternal(T unfinisheditem, RuntimeException ex)
// {
// try
// {
// if (ThreadError != null)
// {
// ThreadError(unfinisheditem, ex);
// }
// }
// catch (UnsupportedOperationException ex2)
// {
// if (ex.getMessage().startsWith("Cross-thread operation not valid"))
// {
// System.out.println(ex2.getMessage());
// }
// else
// {
// throw ex2;
// }
// }
// }
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
// ///#endregion
}
//#endif
© 2015 - 2025 Weber Informatics LLC | Privacy Policy