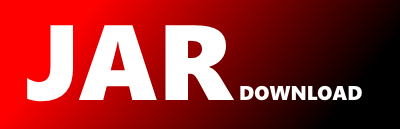
Alachisoft.NCache.Common.Util.CPUUsage Maven / Gradle / Ivy
package Alachisoft.NCache.Common.Util;
/*
* Mansoor: Native dll calls
**/
//C# TO JAVA CONVERTER NOTE: There is no Java equivalent to C# namespace aliases:
//using ComTypes = System.Runtime.InteropServices.ComTypes;
public class CPUUsage {
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
//#if !MONO
// private static native boolean GetSystemTimes(tangible.RefObject lpIdleTime, tangible.RefObject lpKernelTime, tangible.RefObject lpUserTime);
// static {
// System.loadLibrary("kernel32.dll");
// }
//
//
// private System.Runtime.InteropServices.ComTypes.FILETIME _prevSysKernel;
// private System.Runtime.InteropServices.ComTypes.FILETIME _prevSysUser;
////#endif
// private TimeSpan _prevProcTotal = new TimeSpan();
//
// private short _cpuUsage;
// private java.util.Date _lastRun = new java.util.Date(0);
// private long _runCount;
//
// public CPUUsage() {
// _cpuUsage = -1;
// _lastRun = new java.util.Date(0);
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
////#if !MONO
// _prevSysUser.dwHighDateTime = _prevSysUser.dwLowDateTime = 0;
// _prevSysKernel.dwHighDateTime = _prevSysKernel.dwLowDateTime = 0;
////#endif
// _prevProcTotal = TimeSpan.MinValue;
// _runCount = 0;
// }
//
// public final short GetUsage() {
// short cpuCopy = _cpuUsage;
////C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
////#if !MONO
// tangible.RefObject tempRef__runCount = new tangible.RefObject(_runCount);
// boolean tempVar = Interlocked.Increment(tempRef__runCount) == 1;
// _runCount = tempRef__runCount.argvalue;
// if (tempVar) {
// if (!getEnoughTimePassed()) {
// tangible.RefObject tempRef__runCount2 = new tangible.RefObject(_runCount);
// Interlocked.Decrement(tempRef__runCount2);
// _runCount = tempRef__runCount2.argvalue;
// return cpuCopy;
// }
//
// System.Runtime.InteropServices.ComTypes.FILETIME sysIdle, sysKernel, sysUser;
// TimeSpan procTime = new TimeSpan();
//
// Process process = Process.GetCurrentProcess();
//
// if (process == null) {
// return -1;
// }
//
// procTime = process.TotalProcessorTime;
//
//
// tangible.RefObject tempRef_sysIdle = new tangible.RefObject(sysIdle);
// tangible.RefObject tempRef_sysKernel = new tangible.RefObject(sysKernel);
// tangible.RefObject tempRef_sysUser = new tangible.RefObject(sysUser);
// boolean tempVar2 = !GetSystemTimes(tempRef_sysIdle, tempRef_sysKernel, tempRef_sysUser);
// sysIdle = tempRef_sysIdle.argvalue;
// sysKernel = tempRef_sysKernel.argvalue;
// sysUser = tempRef_sysUser.argvalue;
// if (tempVar2) {
// tangible.RefObject tempRef__runCount3 = new tangible.RefObject(_runCount);
// Interlocked.Decrement(tempRef__runCount3);
// _runCount = tempRef__runCount3.argvalue;
// return cpuCopy;
// }
//
// if (!getIsFirstRun()) {
////C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
////ORIGINAL LINE: UInt64 sysKernelDiff = SubtractTimes(sysKernel, _prevSysKernel);
// long sysKernelDiff = SubtractTimes(sysKernel, _prevSysKernel);
////C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
////ORIGINAL LINE: UInt64 sysUserDiff = SubtractTimes(sysUser, _prevSysUser);
// long sysUserDiff = SubtractTimes(sysUser, _prevSysUser);
//
////C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
////ORIGINAL LINE: UInt64 sysTotal = sysKernelDiff + sysUserDiff;
// long sysTotal = sysKernelDiff + sysUserDiff;
//
// long procTotal = procTime.Ticks - _prevProcTotal.Ticks;
//
// if (sysTotal > 0) {
// _cpuUsage = (short)((100.0 * procTotal) / sysTotal);
// }
// }
//
// _prevProcTotal = procTime;
// _prevSysKernel = sysKernel;
// _prevSysUser = sysUser;
//
// _lastRun = new java.util.Date();
//
// cpuCopy = _cpuUsage;
// }
// tangible.RefObject tempRef__runCount4 = new tangible.RefObject(_runCount);
// Interlocked.Decrement(tempRef__runCount4);
// _runCount = tempRef__runCount4.argvalue;
////#else
// //@UH
////#endif
// return cpuCopy;
//
// }
//
// private long SubtractTimes(System.Runtime.InteropServices.ComTypes.FILETIME a, System.Runtime.InteropServices.ComTypes.FILETIME b) {
////C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
////ORIGINAL LINE: UInt64 aInt = ((UInt64)(a.dwHighDateTime << 32)) | (UInt64)a.dwLowDateTime;
// long aInt = ((long)(a.dwHighDateTime << 32)) | (long)a.dwLowDateTime;
////C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
////ORIGINAL LINE: UInt64 bInt = ((UInt64)(b.dwHighDateTime << 32)) | (UInt64)b.dwLowDateTime;
// long bInt = ((long)(b.dwHighDateTime << 32)) | (long)b.dwLowDateTime;
//
// return aInt - bInt;
// }
//
// private boolean getEnoughTimePassed() {
// final int minimumElapsedMS = 250;
// TimeSpan sinceLast = new java.util.Date() - _lastRun;
// return sinceLast.TotalMilliseconds > minimumElapsedMS;
// }
//
// private boolean getIsFirstRun() {
// return (_lastRun.equals(new java.util.Date(0)));
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy