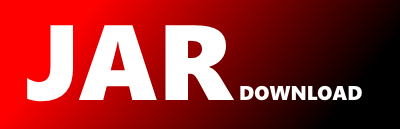
com.alachisoft.ncache.common.caching.statistics.customcounters.FlipManager Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.common.caching.statistics.customcounters;
/**
* @author Muneeb Shahid
*/
public class FlipManager implements Runnable {
private static final Object mutex = new Object();
static long timeLapse;
static InstantaneousFlip flip;
static Thread flipManagerThread;
private static boolean isStarted;
private static int refCount;
static {
flip = new InstantaneousFlip(0);
timeLapse = 1000; //1sec
isStarted = false;
}
static void timerElapsed(Object sender) {
flip.increment();
}
public static InstantaneousFlip getFlip() {
return flip;
}
public static void Stop() {
synchronized (mutex) {
if (refCount > 0) {
refCount--;
if (refCount == 0) {
isStarted = false;
if (flipManagerThread != null && flipManagerThread.isAlive()) {
flipManagerThread.interrupt();
}
}
}
}
}
public static void Start() {
synchronized (mutex) {
if (flipManagerThread == null) {
isStarted = true;
flipManagerThread = new Thread(new FlipManager());
flipManagerThread.setDaemon(true);
flipManagerThread.setName("FlipManager");
flipManagerThread.start();
}
refCount++;
}
}
@Override
public void run() {
if (!isStarted) {
return;
}
while (isStarted) {
FlipManager.timerElapsed(this);
try {
Thread.sleep(timeLapse);
} catch (InterruptedException interruptedException) {
isStarted = false;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy