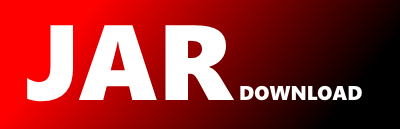
com.alachisoft.ncache.common.monitoring.ClientCustomCounters Maven / Gradle / Ivy
package com.alachisoft.ncache.common.monitoring;
import com.alachisoft.ncache.serialization.core.io.InternalCompactSerializable;
import com.alachisoft.ncache.serialization.standard.io.CompactReader;
import com.alachisoft.ncache.serialization.standard.io.CompactWriter;
import java.io.IOException;
// Copyright (c) 2018 Alachisoft
//
// Licensed under the Apache License, Version 2.0 (the "License") = 0;
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License
public class ClientCustomCounters implements InternalCompactSerializable
{
public double _pcClientRequestsPerSec = 0;
/** performance counter for cache responses per second by the client.
*/
public double _pcClientResponsesPerSec = 0;
/** performance counter for cache requests per second by all the clients.
*/
public double _pcTotalClientRequestsPerSec = 0;
/** performance counter for cache responses per second by the all clients.
*/
public double _pcTotalClientResponsesPerSec = 0;
/** performance counter for Cache get operations per second.
*/
public double _pcGetPerSec = 0;
/** performance counter for Cache add operations per second.
*/
public double _pcAddPerSec = 0;
/** performance counter for Cache update operations per second.
*/
public double _pcUpdPerSec = 0;
/** performance counter for Cache remove operations per second.
*/
public double _pcDelPerSec = 0;
/** performance counter read operations per second
*/
public double _pcReadOperationsPerSec = 0;
/** performance counter write operations per second
*/
public double _pcWriteOperationsPerSec = 0;
/** performance counter for Mirror Queue size.
*/
public double _pcReqrQueueSize = 0;
/** performance counter for Cache avg. per milli-second time of get operations.
*/
public double _pcMsecPerGetAvg = 0;
/** performance counter for Cache avg. per milli-second time of add operations.
*/
public double _pcMsecPerAddAvg = 0;
/** performance counter for Cache avg. per milli-second time of update operations.
*/
public double _pcMsecPerUpdAvg = 0;
/** performance counter for Cache avg. per milli-second time of remove operations.
*/
public double _pcMsecPerDelAvg = 0;
/** performance counter for Cache max. per milli-second time of get operations.
*/
public double _pcMsecPerGetBase = 0;
/** performance counter for Cache max. per milli-second time of add operations.
*/
public double _pcMsecPerAddBase = 0;
/** performance counter for Cache max. per milli-second time of update operations.
*/
public double _pcMsecPerUpdBase = 0;
/** performance counter for Cache max. per milli-second time of remove operations.
*/
public double _pcMsecPerDelBase = 0;
/** performance counter for Cache avg. per milli-second time of Event operations.
*/
public double _pcMsecPerEventAvg = 0;
/** performance counter for Cache max. per milli-second time of Event operations.
*/
public double _pcMsecPerEventBase = 0;
/** performance counter for events processed per second.
*/
public double _pcEventProcesedPerSec = 0;
/** performance counter for events Triggered/Received per second.
*/
public double _pcEventTriggeredPerSec = 0;
//Bulk Counters
public double _pcMsecPerAddBulkAvg = 0;
public double _pcMsecPerAddBulkBase = 0;
public double _pcMsecPerUpdBulkAvg = 0;
public double _pcMsecPerUpdBulkBase = 0;
public double _pcMsecPerGetBulkAvg = 0;
public double _pcMsecPerGetBulkBase = 0;
public double _pcMsecPerDelBulkAvg = 0;
public double _pcMsecPerDelBulkBase = 0;
/** performance counter for
*/
public double _pcAvgItemSize = 0;
/** base performance counter for
*/
public double _pcAvgItemSizeBase = 0;
/** performance counter for
*/
public double _pcCompressionPerSec = 0;
public double _pcMsecPerEncryptionAvg = 0;
public double _pcMsecPerDecryptionAvg = 0;
public double _pcMsecPerEncryptionAvgBase = 0;
public double _pcMsecPerDecryptionAvgBase = 0;
public double _pcMsecPerCompressionAvg = 0;
public double _pcMsecPerCompressionAvgBase = 0;
public double _pcMsecPerDecompressionAvg = 0;
public double _pcMsecPerDecompressionAvgBase = 0;
public double _pcAvgCompressedItemSize = 0;
public double _pcAvgCompressedItemSizeBase = 0;
public double _pcMsecPerSerializationAvg = 0;
public double _pcMsecPerSerializationAvgBase = 0;
public double _pcMsecPerDeserializationAvg = 0;
public double _pcMsecPerDeserializationAvgBase = 0;
//Polling counters
/**
Count of Poll requests made either after client interval or server notification
*/
public double _pollRequestsSent = 0;
/**
Resultant Updates from last poll request
*/
public double _pollLastUpdates = 0;
/**
Resultant Removes from last poll request
*/
public double _pollLastRemoves = 0;
public double _pcMsecPerMessagePublishAvg = 0;
public double _pcMsecPerMessagePublishBase = 0;
public double _pcMessagePublishPerSec = 0;
public double _pcMessageDeliverPerSec = 0;
public double _pcMemoryUsage=0;
public double _pcCpuUsage=0;
public ClientCustomCounters()
{
}
@Override
public void Deserialize(CompactReader reader) throws IOException {
_pcAddPerSec = reader.ReadDouble();
_pcAddPerSec = reader.ReadDouble();
_pcGetPerSec = reader.ReadDouble();
_pcUpdPerSec = reader.ReadDouble();
_pcDelPerSec = reader.ReadDouble();
_pcEventTriggeredPerSec = reader.ReadDouble();
_pcEventProcesedPerSec = reader.ReadDouble();
_pcReadOperationsPerSec = reader.ReadDouble();
_pcWriteOperationsPerSec = reader.ReadDouble();
_pcMsecPerAddBulkAvg = reader.ReadDouble();
_pcMsecPerAddBulkBase = reader.ReadDouble();
_pcMsecPerGetBulkAvg = reader.ReadDouble();
_pcMsecPerGetBulkBase = reader.ReadDouble();
_pcMsecPerUpdBulkAvg = reader.ReadDouble();
_pcMsecPerUpdBulkBase = reader.ReadDouble();
_pcMsecPerDelBulkAvg = reader.ReadDouble();
_pcMsecPerDelBulkBase = reader.ReadDouble();
_pcMsecPerGetAvg = reader.ReadDouble();
_pcMsecPerGetBase = reader.ReadDouble();
//_usMsecPerGet = _usMsecPerGet;
_pcMsecPerAddAvg = reader.ReadDouble();
_pcMsecPerAddBase = reader.ReadDouble();
//_usMsecPerAdd = _usMsecPerAdd;
_pcMsecPerUpdAvg = reader.ReadDouble();
_pcMsecPerUpdBase = reader.ReadDouble();
//_usMsecPerUpd = _usMsecPerUpd;
_pcMsecPerDelAvg = reader.ReadDouble();
_pcMsecPerDelBase = reader.ReadDouble();
//_usMsecPerDel = _usMsecPerDel;
_pcReqrQueueSize = reader.ReadDouble();
_pcCompressionPerSec = reader.ReadDouble();
_pcAvgItemSize = reader.ReadDouble();
_pcAvgItemSizeBase = reader.ReadDouble();
_pcMsecPerEventAvg = reader.ReadDouble();
_pcMsecPerEventBase = reader.ReadDouble();
//_usMsecPerEvent = _usMsecPerEvent;
//_usMsecPerDel = _usMsecPerDel;
_pcMsecPerCompressionAvg = reader.ReadDouble();
_pcMsecPerCompressionAvgBase = reader.ReadDouble();
_pcMsecPerDecompressionAvg = reader.ReadDouble();
_pcMsecPerDecompressionAvgBase = reader.ReadDouble();
_pcAvgCompressedItemSize = reader.ReadDouble();
_pcAvgCompressedItemSizeBase = reader.ReadDouble();
_pcMsecPerSerializationAvg = reader.ReadDouble();
_pcMsecPerSerializationAvgBase = reader.ReadDouble();
_pcMsecPerDeserializationAvg = reader.ReadDouble();
_pcMsecPerDeserializationAvgBase = reader.ReadDouble();
_pcMsecPerEncryptionAvg = reader.ReadDouble();
_pcMsecPerEncryptionAvgBase = reader.ReadDouble();
_pcMsecPerDecryptionAvg = reader.ReadDouble();
_pcMsecPerDecryptionAvgBase = reader.ReadDouble();
_pollRequestsSent = reader.ReadDouble();
_pollLastUpdates = reader.ReadDouble();
_pollLastRemoves = reader.ReadDouble();
//countes._pubsubCounterList = _pubsubCounterList;
_pcMsecPerMessagePublishAvg = reader.ReadDouble();
_pcMsecPerMessagePublishBase = reader.ReadDouble();
_pcMessagePublishPerSec = reader.ReadDouble();
_pcMessageDeliverPerSec = reader.ReadDouble();
_pcClientRequestsPerSec = reader.ReadDouble();
_pcClientResponsesPerSec = reader.ReadDouble();
_pcTotalClientRequestsPerSec = reader.ReadDouble();
_pcTotalClientResponsesPerSec = reader.ReadDouble();
_pcMemoryUsage=reader.ReadDouble();
_pcCpuUsage=reader.ReadDouble();
}
@Override
public void Serialize(CompactWriter writer) throws IOException {
writer.Write(_pcAddPerSec);
writer.Write(_pcAddPerSec);
writer.Write(_pcGetPerSec);
writer.Write(_pcUpdPerSec);
writer.Write(_pcDelPerSec);
writer.Write(_pcEventTriggeredPerSec);
writer.Write(_pcEventProcesedPerSec);
writer.Write(_pcReadOperationsPerSec);
writer.Write(_pcWriteOperationsPerSec);
writer.Write(_pcMsecPerAddBulkAvg);
writer.Write(_pcMsecPerAddBulkBase);
writer.Write(_pcMsecPerGetBulkAvg);
writer.Write(_pcMsecPerGetBulkBase);
writer.Write(_pcMsecPerUpdBulkAvg);
writer.Write(_pcMsecPerUpdBulkBase);
writer.Write(_pcMsecPerDelBulkAvg);
writer.Write(_pcMsecPerDelBulkBase);
writer.Write(_pcMsecPerGetAvg);
writer.Write(_pcMsecPerGetBase);
//_usMsecPerGet = _usMsecPerGet;
writer.Write(_pcMsecPerAddAvg);
writer.Write(_pcMsecPerAddBase);
//_usMsecPerAdd = _usMsecPerAdd;
writer.Write(_pcMsecPerUpdAvg);
writer.Write(_pcMsecPerUpdBase);
//_usMsecPerUpd = _usMsecPerUpd;
writer.Write(_pcMsecPerDelAvg);
writer.Write(_pcMsecPerDelBase);
//_usMsecPerDel = _usMsecPerDel;
writer.Write(_pcReqrQueueSize);
writer.Write(_pcCompressionPerSec);
writer.Write(_pcAvgItemSize);
writer.Write(_pcAvgItemSizeBase);
writer.Write(_pcMsecPerEventAvg);
writer.Write(_pcMsecPerEventBase);
//_usMsecPerEvent = _usMsecPerEvent;
//_usMsecPerDel = _usMsecPerDel;
writer.Write(_pcMsecPerCompressionAvg);
writer.Write(_pcMsecPerCompressionAvgBase);
writer.Write(_pcMsecPerDecompressionAvg);
writer.Write(_pcMsecPerDecompressionAvgBase);
writer.Write(_pcAvgCompressedItemSize);
writer.Write(_pcAvgCompressedItemSizeBase);
writer.Write(_pcMsecPerSerializationAvg);
writer.Write(_pcMsecPerSerializationAvgBase);
writer.Write(_pcMsecPerDeserializationAvg);
writer.Write(_pcMsecPerDeserializationAvgBase);
writer.Write(_pcMsecPerEncryptionAvg);
writer.Write(_pcMsecPerEncryptionAvgBase);
writer.Write(_pcMsecPerDecryptionAvg);
writer.Write(_pcMsecPerDecryptionAvgBase);
writer.Write(_pollRequestsSent);
writer.Write(_pollLastUpdates);
writer.Write(_pollLastRemoves);
//countes._pubsubCounterList = _pubsubCounterList;
writer.Write(_pcMsecPerMessagePublishAvg);
writer.Write(_pcMsecPerMessagePublishBase);
writer.Write(_pcMessagePublishPerSec);
writer.Write(_pcMessageDeliverPerSec);
writer.Write(_pcClientRequestsPerSec);
writer.Write(_pcClientResponsesPerSec);
writer.Write(_pcTotalClientRequestsPerSec);
writer.Write(_pcTotalClientResponsesPerSec);
writer.Write(_pcMemoryUsage);
writer.Write(_pcCpuUsage);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy