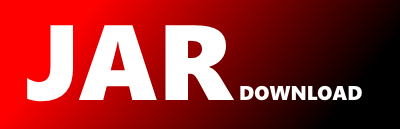
com.alachisoft.ncache.common.protobuf.CommandProtocol Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Command.proto
package com.alachisoft.ncache.common.protobuf;
public final class CommandProtocol {
private CommandProtocol() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public static final class Command extends
com.google.protobuf.GeneratedMessage {
// Use Command.newBuilder() to construct.
private Command() {
initFields();
}
private Command(boolean noInit) {}
private static final Command defaultInstance;
public static Command getDefaultInstance() {
return defaultInstance;
}
public Command getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.CommandProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Command_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.CommandProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Command_fieldAccessorTable;
}
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
ADD(0, 1),
ADD_DEPENDENCY(1, 2),
ADD_SYNC_DEPENDENCY(2, 3),
ADD_BULK(3, 4),
GET_BULK(4, 5),
INSERT_BULK(5, 6),
REMOVE_BULK(6, 7),
CLEAR(7, 8),
CONTAINS(8, 9),
COUNT(9, 10),
DISPOSE(10, 11),
GET_CACHE_ITEM(11, 12),
GET(12, 13),
GET_COMPACT_TYPES(13, 14),
GET_ENUMERATOR(14, 15),
GET_GROUP(15, 16),
GET_HASHMAP(16, 17),
GET_OPTIMAL_SERVER(17, 18),
GET_THRESHOLD_SIZE(18, 19),
GET_TYPEINFO_MAP(19, 20),
INIT(20, 21),
INSERT(21, 22),
RAISE_CUSTOM_EVENT(22, 23),
REGISTER_KEY_NOTIF(23, 24),
REGISTER_NOTIF(24, 25),
REMOVE(25, 26),
REMOVE_GROUP(26, 27),
SEARCH(27, 28),
GET_TAG(28, 29),
LOCK(29, 30),
UNLOCK(30, 31),
ISLOCKED(31, 32),
LOCK_VERIFY(32, 33),
UNREGISTER_KEY_NOTIF(33, 34),
UNREGISTER_BULK_KEY_NOTIF(34, 35),
REGISTER_BULK_KEY_NOTIF(35, 36),
HYBRID_BULK(36, 37),
GET_LOGGING_INFO(37, 38),
CLOSE_STREAM(38, 39),
GET_STREAM_LENGTH(39, 40),
OPEN_STREAM(40, 41),
WRITE_TO_STREAM(41, 42),
READ_FROM_STREAM(42, 43),
BRIDGE_GET_STATE_TRANSFER_INFO(43, 44),
BRIDGE_GET_TRANSFERABLE_KEY_LIST(44, 45),
BRIDGE_HYBRID_BULK(45, 46),
BRIDGE_INIT(46, 47),
BRIDGE_SET_TRANSFERABLE_KEY_LIST(47, 48),
BRIDGE_SIGNAL_END_OF_STATE_TRANSFER(48, 49),
BRIDGE_SYNC_TIME(49, 50),
BRIDGE_HEART_BEAT_RECEIVED(50, 51),
REMOVE_BY_TAG(51, 52),
BRIDGE_MAKE_TARGET_CACHE_ACTIVE_PASSIVE(52, 53),
UNREGISTER_CQ(53, 54),
SEARCH_CQ(54, 55),
REGISTER_CQ(55, 56),
GET_KEYS_TAG(56, 57),
DELETE_BULK(57, 58),
DELETE(58, 59),
GET_NEXT_CHUNK(59, 60),
GET_GROUP_NEXT_CHUNK(60, 61),
ADD_ATTRIBUTE(61, 62),
GET_ENCRYPTION(62, 63),
GET_RUNNING_SERVERS(63, 64),
SYNC_EVENTS(64, 65),
DELETEQUERY(65, 66),
GET_PRODUCT_VERSION(66, 67),
BRIDGE_GET_REPLICATOR_STATUS_INFO(67, 68),
BRIDGE_REQUEST_STATE_TRANSFER(68, 69),
GET_SERVER_MAPPING(69, 70),
INQUIRY_REQUEST(70, 71),
GET_CACHE_BINDING(71, 72),
MAP_REDUCE_TASK(72, 74),
TASK_CALLBACK(73, 75),
RUNNING_TASKS(74, 76),
TASK_PROGRESS(75, 77),
CANCEL_TASK(76, 78),
TASK_NEXT_RECORD(77, 79),
TASK_ENUMERATOR(78, 80),
INVOKE_ENTRY_PROCESSOR(79, 81),
EXECUTE_READER(80, 82),
GET_READER_CHUNK(81, 83),
DISPOSE_READER(82, 84),
EXECUTE_READER_CQ(83, 85),
GET_EXPIRATION(84, 86),
GET_LC_DATA(85, 87),
POLL(86, 88),
REGISTER_POLLING_NOTIFICATION(87, 89),
SECURITY_AUTHORIZATION(88, 90),
GET_CONNECTED_CLIENTS(89, 91),
TOUCH(90, 92),
GET_CACHE_MANAGEMENT_PORT(91, 94),
GET_TOPIC(92, 95),
SUBSCRIBE_TOPIC(93, 97),
REMOVE_TOPIC(94, 98),
UNSUBSCRIBE_TOPIC(95, 99),
MESSAGE_PUBLISH(96, 100),
GET_MESSAGE(97, 101),
MESSAGE_ACKNOWLEDGMENT(98, 102),
PING(99, 103),
MESSAGE_COUNT(100, 104),
DATATYPES_COMMAND(101, 105),
GET_SERIALIZATION_FORMAT(102, 106),
GET_BULK_CACHEITEM(103, 107),
CONTAINS_BULK(104, 108),
DATATYPE_MANAGEMENT(105, 109),
LIST_COMMAND(106, 110),
DICTIONARY_COMMAND(107, 111),
HASHSET_COMMAND(108, 112),
QUEUE_COMMAND(109, 113),
COLLECTION_COMMAND(110, 114),
COLLECTION_NOTIFICATION(111, 115),
COUNTER_COMMAND(112, 116),
MODULE(113, 117),
SURROGATE(114, 118),
MESSAGE_PUBLISH_BULK(115, 119),
BRIDGE_POLL_FOR_RESUMING_REPLICATION(116, 120),
GETMODULESTATE(117, 121),
SETMODULESTATE(118, 122),
;
public final int getNumber() { return value; }
public static Type valueOf(int value) {
switch (value) {
case 1: return ADD;
case 2: return ADD_DEPENDENCY;
case 3: return ADD_SYNC_DEPENDENCY;
case 4: return ADD_BULK;
case 5: return GET_BULK;
case 6: return INSERT_BULK;
case 7: return REMOVE_BULK;
case 8: return CLEAR;
case 9: return CONTAINS;
case 10: return COUNT;
case 11: return DISPOSE;
case 12: return GET_CACHE_ITEM;
case 13: return GET;
case 14: return GET_COMPACT_TYPES;
case 15: return GET_ENUMERATOR;
case 16: return GET_GROUP;
case 17: return GET_HASHMAP;
case 18: return GET_OPTIMAL_SERVER;
case 19: return GET_THRESHOLD_SIZE;
case 20: return GET_TYPEINFO_MAP;
case 21: return INIT;
case 22: return INSERT;
case 23: return RAISE_CUSTOM_EVENT;
case 24: return REGISTER_KEY_NOTIF;
case 25: return REGISTER_NOTIF;
case 26: return REMOVE;
case 27: return REMOVE_GROUP;
case 28: return SEARCH;
case 29: return GET_TAG;
case 30: return LOCK;
case 31: return UNLOCK;
case 32: return ISLOCKED;
case 33: return LOCK_VERIFY;
case 34: return UNREGISTER_KEY_NOTIF;
case 35: return UNREGISTER_BULK_KEY_NOTIF;
case 36: return REGISTER_BULK_KEY_NOTIF;
case 37: return HYBRID_BULK;
case 38: return GET_LOGGING_INFO;
case 39: return CLOSE_STREAM;
case 40: return GET_STREAM_LENGTH;
case 41: return OPEN_STREAM;
case 42: return WRITE_TO_STREAM;
case 43: return READ_FROM_STREAM;
case 44: return BRIDGE_GET_STATE_TRANSFER_INFO;
case 45: return BRIDGE_GET_TRANSFERABLE_KEY_LIST;
case 46: return BRIDGE_HYBRID_BULK;
case 47: return BRIDGE_INIT;
case 48: return BRIDGE_SET_TRANSFERABLE_KEY_LIST;
case 49: return BRIDGE_SIGNAL_END_OF_STATE_TRANSFER;
case 50: return BRIDGE_SYNC_TIME;
case 51: return BRIDGE_HEART_BEAT_RECEIVED;
case 52: return REMOVE_BY_TAG;
case 53: return BRIDGE_MAKE_TARGET_CACHE_ACTIVE_PASSIVE;
case 54: return UNREGISTER_CQ;
case 55: return SEARCH_CQ;
case 56: return REGISTER_CQ;
case 57: return GET_KEYS_TAG;
case 58: return DELETE_BULK;
case 59: return DELETE;
case 60: return GET_NEXT_CHUNK;
case 61: return GET_GROUP_NEXT_CHUNK;
case 62: return ADD_ATTRIBUTE;
case 63: return GET_ENCRYPTION;
case 64: return GET_RUNNING_SERVERS;
case 65: return SYNC_EVENTS;
case 66: return DELETEQUERY;
case 67: return GET_PRODUCT_VERSION;
case 68: return BRIDGE_GET_REPLICATOR_STATUS_INFO;
case 69: return BRIDGE_REQUEST_STATE_TRANSFER;
case 70: return GET_SERVER_MAPPING;
case 71: return INQUIRY_REQUEST;
case 72: return GET_CACHE_BINDING;
case 74: return MAP_REDUCE_TASK;
case 75: return TASK_CALLBACK;
case 76: return RUNNING_TASKS;
case 77: return TASK_PROGRESS;
case 78: return CANCEL_TASK;
case 79: return TASK_NEXT_RECORD;
case 80: return TASK_ENUMERATOR;
case 81: return INVOKE_ENTRY_PROCESSOR;
case 82: return EXECUTE_READER;
case 83: return GET_READER_CHUNK;
case 84: return DISPOSE_READER;
case 85: return EXECUTE_READER_CQ;
case 86: return GET_EXPIRATION;
case 87: return GET_LC_DATA;
case 88: return POLL;
case 89: return REGISTER_POLLING_NOTIFICATION;
case 90: return SECURITY_AUTHORIZATION;
case 91: return GET_CONNECTED_CLIENTS;
case 92: return TOUCH;
case 94: return GET_CACHE_MANAGEMENT_PORT;
case 95: return GET_TOPIC;
case 97: return SUBSCRIBE_TOPIC;
case 98: return REMOVE_TOPIC;
case 99: return UNSUBSCRIBE_TOPIC;
case 100: return MESSAGE_PUBLISH;
case 101: return GET_MESSAGE;
case 102: return MESSAGE_ACKNOWLEDGMENT;
case 103: return PING;
case 104: return MESSAGE_COUNT;
case 105: return DATATYPES_COMMAND;
case 106: return GET_SERIALIZATION_FORMAT;
case 107: return GET_BULK_CACHEITEM;
case 108: return CONTAINS_BULK;
case 109: return DATATYPE_MANAGEMENT;
case 110: return LIST_COMMAND;
case 111: return DICTIONARY_COMMAND;
case 112: return HASHSET_COMMAND;
case 113: return QUEUE_COMMAND;
case 114: return COLLECTION_COMMAND;
case 115: return COLLECTION_NOTIFICATION;
case 116: return COUNTER_COMMAND;
case 117: return MODULE;
case 118: return SURROGATE;
case 119: return MESSAGE_PUBLISH_BULK;
case 120: return BRIDGE_POLL_FOR_RESUMING_REPLICATION;
case 121: return GETMODULESTATE;
case 122: return SETMODULESTATE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.valueOf(number)
; }
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = {
ADD, ADD_DEPENDENCY, ADD_SYNC_DEPENDENCY, ADD_BULK, GET_BULK, INSERT_BULK, REMOVE_BULK, CLEAR, CONTAINS, COUNT, DISPOSE, GET_CACHE_ITEM, GET, GET_COMPACT_TYPES, GET_ENUMERATOR, GET_GROUP, GET_HASHMAP, GET_OPTIMAL_SERVER, GET_THRESHOLD_SIZE, GET_TYPEINFO_MAP, INIT, INSERT, RAISE_CUSTOM_EVENT, REGISTER_KEY_NOTIF, REGISTER_NOTIF, REMOVE, REMOVE_GROUP, SEARCH, GET_TAG, LOCK, UNLOCK, ISLOCKED, LOCK_VERIFY, UNREGISTER_KEY_NOTIF, UNREGISTER_BULK_KEY_NOTIF, REGISTER_BULK_KEY_NOTIF, HYBRID_BULK, GET_LOGGING_INFO, CLOSE_STREAM, GET_STREAM_LENGTH, OPEN_STREAM, WRITE_TO_STREAM, READ_FROM_STREAM, BRIDGE_GET_STATE_TRANSFER_INFO, BRIDGE_GET_TRANSFERABLE_KEY_LIST, BRIDGE_HYBRID_BULK, BRIDGE_INIT, BRIDGE_SET_TRANSFERABLE_KEY_LIST, BRIDGE_SIGNAL_END_OF_STATE_TRANSFER, BRIDGE_SYNC_TIME, BRIDGE_HEART_BEAT_RECEIVED, REMOVE_BY_TAG, BRIDGE_MAKE_TARGET_CACHE_ACTIVE_PASSIVE, UNREGISTER_CQ, SEARCH_CQ, REGISTER_CQ, GET_KEYS_TAG, DELETE_BULK, DELETE, GET_NEXT_CHUNK, GET_GROUP_NEXT_CHUNK, ADD_ATTRIBUTE, GET_ENCRYPTION, GET_RUNNING_SERVERS, SYNC_EVENTS, DELETEQUERY, GET_PRODUCT_VERSION, BRIDGE_GET_REPLICATOR_STATUS_INFO, BRIDGE_REQUEST_STATE_TRANSFER, GET_SERVER_MAPPING, INQUIRY_REQUEST, GET_CACHE_BINDING, MAP_REDUCE_TASK, TASK_CALLBACK, RUNNING_TASKS, TASK_PROGRESS, CANCEL_TASK, TASK_NEXT_RECORD, TASK_ENUMERATOR, INVOKE_ENTRY_PROCESSOR, EXECUTE_READER, GET_READER_CHUNK, DISPOSE_READER, EXECUTE_READER_CQ, GET_EXPIRATION, GET_LC_DATA, POLL, REGISTER_POLLING_NOTIFICATION, SECURITY_AUTHORIZATION, GET_CONNECTED_CLIENTS, TOUCH, GET_CACHE_MANAGEMENT_PORT, GET_TOPIC, SUBSCRIBE_TOPIC, REMOVE_TOPIC, UNSUBSCRIBE_TOPIC, MESSAGE_PUBLISH, GET_MESSAGE, MESSAGE_ACKNOWLEDGMENT, PING, MESSAGE_COUNT, DATATYPES_COMMAND, GET_SERIALIZATION_FORMAT, GET_BULK_CACHEITEM, CONTAINS_BULK, DATATYPE_MANAGEMENT, LIST_COMMAND, DICTIONARY_COMMAND, HASHSET_COMMAND, QUEUE_COMMAND, COLLECTION_COMMAND, COLLECTION_NOTIFICATION, COUNTER_COMMAND, MODULE, SURROGATE, MESSAGE_PUBLISH_BULK, BRIDGE_POLL_FOR_RESUMING_REPLICATION, GETMODULESTATE, SETMODULESTATE,
};
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Type(int index, int value) {
this.index = index;
this.value = value;
}
static {
com.alachisoft.ncache.common.protobuf.CommandProtocol.getDescriptor();
}
// @@protoc_insertion_point(enum_scope:com.alachisoft.ncache.common.protobuf.Command.Type)
}
// optional .com.alachisoft.ncache.common.protobuf.Command.Type type = 1;
public static final int TYPE_FIELD_NUMBER = 1;
private boolean hasType;
private com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type type_;
public boolean hasType() { return hasType; }
public com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type getType() { return type_; }
// optional string version = 2;
public static final int VERSION_FIELD_NUMBER = 2;
private boolean hasVersion;
private java.lang.String version_ = "";
public boolean hasVersion() { return hasVersion; }
public java.lang.String getVersion() { return version_; }
// optional .com.alachisoft.ncache.common.protobuf.AddCommand addCommand = 3;
public static final int ADDCOMMAND_FIELD_NUMBER = 3;
private boolean hasAddCommand;
private com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand addCommand_;
public boolean hasAddCommand() { return hasAddCommand; }
public com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand getAddCommand() { return addCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.AddDependencyCommand addDependencyCommand = 4;
public static final int ADDDEPENDENCYCOMMAND_FIELD_NUMBER = 4;
private boolean hasAddDependencyCommand;
private com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand addDependencyCommand_;
public boolean hasAddDependencyCommand() { return hasAddDependencyCommand; }
public com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand getAddDependencyCommand() { return addDependencyCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommand addSyncDependencyCommand = 5;
public static final int ADDSYNCDEPENDENCYCOMMAND_FIELD_NUMBER = 5;
private boolean hasAddSyncDependencyCommand;
private com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand addSyncDependencyCommand_;
public boolean hasAddSyncDependencyCommand() { return hasAddSyncDependencyCommand; }
public com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand getAddSyncDependencyCommand() { return addSyncDependencyCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BulkAddCommand bulkAddCommand = 6;
public static final int BULKADDCOMMAND_FIELD_NUMBER = 6;
private boolean hasBulkAddCommand;
private com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand bulkAddCommand_;
public boolean hasBulkAddCommand() { return hasBulkAddCommand; }
public com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand getBulkAddCommand() { return bulkAddCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BulkGetCommand bulkGetCommand = 7;
public static final int BULKGETCOMMAND_FIELD_NUMBER = 7;
private boolean hasBulkGetCommand;
private com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand bulkGetCommand_;
public boolean hasBulkGetCommand() { return hasBulkGetCommand; }
public com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand getBulkGetCommand() { return bulkGetCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BulkInsertCommand bulkInsertCommand = 8;
public static final int BULKINSERTCOMMAND_FIELD_NUMBER = 8;
private boolean hasBulkInsertCommand;
private com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand bulkInsertCommand_;
public boolean hasBulkInsertCommand() { return hasBulkInsertCommand; }
public com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand getBulkInsertCommand() { return bulkInsertCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BulkRemoveCommand bulkRemoveCommand = 9;
public static final int BULKREMOVECOMMAND_FIELD_NUMBER = 9;
private boolean hasBulkRemoveCommand;
private com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand bulkRemoveCommand_;
public boolean hasBulkRemoveCommand() { return hasBulkRemoveCommand; }
public com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand getBulkRemoveCommand() { return bulkRemoveCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.ClearCommand clearCommand = 10;
public static final int CLEARCOMMAND_FIELD_NUMBER = 10;
private boolean hasClearCommand;
private com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand clearCommand_;
public boolean hasClearCommand() { return hasClearCommand; }
public com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand getClearCommand() { return clearCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.ContainsCommand containsCommand = 11;
public static final int CONTAINSCOMMAND_FIELD_NUMBER = 11;
private boolean hasContainsCommand;
private com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand containsCommand_;
public boolean hasContainsCommand() { return hasContainsCommand; }
public com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand getContainsCommand() { return containsCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.CountCommand countCommand = 12;
public static final int COUNTCOMMAND_FIELD_NUMBER = 12;
private boolean hasCountCommand;
private com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand countCommand_;
public boolean hasCountCommand() { return hasCountCommand; }
public com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand getCountCommand() { return countCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.DisposeCommand disposeCommand = 13;
public static final int DISPOSECOMMAND_FIELD_NUMBER = 13;
private boolean hasDisposeCommand;
private com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand disposeCommand_;
public boolean hasDisposeCommand() { return hasDisposeCommand; }
public com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand getDisposeCommand() { return disposeCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetCacheItemCommand getCacheItemCommand = 14;
public static final int GETCACHEITEMCOMMAND_FIELD_NUMBER = 14;
private boolean hasGetCacheItemCommand;
private com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand getCacheItemCommand_;
public boolean hasGetCacheItemCommand() { return hasGetCacheItemCommand; }
public com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand getGetCacheItemCommand() { return getCacheItemCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetCommand getCommand = 15;
public static final int GETCOMMAND_FIELD_NUMBER = 15;
private boolean hasGetCommand;
private com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand getCommand_;
public boolean hasGetCommand() { return hasGetCommand; }
public com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand getGetCommand() { return getCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetCompactTypesCommand getCompactTypesCommand = 16;
public static final int GETCOMPACTTYPESCOMMAND_FIELD_NUMBER = 16;
private boolean hasGetCompactTypesCommand;
private com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand getCompactTypesCommand_;
public boolean hasGetCompactTypesCommand() { return hasGetCompactTypesCommand; }
public com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand getGetCompactTypesCommand() { return getCompactTypesCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetEnumeratorCommand getEnumeratorCommand = 17;
public static final int GETENUMERATORCOMMAND_FIELD_NUMBER = 17;
private boolean hasGetEnumeratorCommand;
private com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand getEnumeratorCommand_;
public boolean hasGetEnumeratorCommand() { return hasGetEnumeratorCommand; }
public com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand getGetEnumeratorCommand() { return getEnumeratorCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetGroupCommand getGroupCommand = 18;
public static final int GETGROUPCOMMAND_FIELD_NUMBER = 18;
private boolean hasGetGroupCommand;
private com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand getGroupCommand_;
public boolean hasGetGroupCommand() { return hasGetGroupCommand; }
public com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand getGetGroupCommand() { return getGroupCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetHashmapCommand getHashmapCommand = 19;
public static final int GETHASHMAPCOMMAND_FIELD_NUMBER = 19;
private boolean hasGetHashmapCommand;
private com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand getHashmapCommand_;
public boolean hasGetHashmapCommand() { return hasGetHashmapCommand; }
public com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand getGetHashmapCommand() { return getHashmapCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetOptimalServerCommand getOptimalServerCommand = 20;
public static final int GETOPTIMALSERVERCOMMAND_FIELD_NUMBER = 20;
private boolean hasGetOptimalServerCommand;
private com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand getOptimalServerCommand_;
public boolean hasGetOptimalServerCommand() { return hasGetOptimalServerCommand; }
public com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand getGetOptimalServerCommand() { return getOptimalServerCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommand getThresholdSizeCommand = 21;
public static final int GETTHRESHOLDSIZECOMMAND_FIELD_NUMBER = 21;
private boolean hasGetThresholdSizeCommand;
private com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand getThresholdSizeCommand_;
public boolean hasGetThresholdSizeCommand() { return hasGetThresholdSizeCommand; }
public com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand getGetThresholdSizeCommand() { return getThresholdSizeCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommand getTypeInfoMapCommand = 22;
public static final int GETTYPEINFOMAPCOMMAND_FIELD_NUMBER = 22;
private boolean hasGetTypeInfoMapCommand;
private com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand getTypeInfoMapCommand_;
public boolean hasGetTypeInfoMapCommand() { return hasGetTypeInfoMapCommand; }
public com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand getGetTypeInfoMapCommand() { return getTypeInfoMapCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.InitCommand initCommand = 23;
public static final int INITCOMMAND_FIELD_NUMBER = 23;
private boolean hasInitCommand;
private com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand initCommand_;
public boolean hasInitCommand() { return hasInitCommand; }
public com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand getInitCommand() { return initCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.InsertCommand insertCommand = 24;
public static final int INSERTCOMMAND_FIELD_NUMBER = 24;
private boolean hasInsertCommand;
private com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand insertCommand_;
public boolean hasInsertCommand() { return hasInsertCommand; }
public com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand getInsertCommand() { return insertCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommand raiseCustomEventCommand = 25;
public static final int RAISECUSTOMEVENTCOMMAND_FIELD_NUMBER = 25;
private boolean hasRaiseCustomEventCommand;
private com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand raiseCustomEventCommand_;
public boolean hasRaiseCustomEventCommand() { return hasRaiseCustomEventCommand; }
public com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand getRaiseCustomEventCommand() { return raiseCustomEventCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommand registerKeyNotifCommand = 26;
public static final int REGISTERKEYNOTIFCOMMAND_FIELD_NUMBER = 26;
private boolean hasRegisterKeyNotifCommand;
private com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand registerKeyNotifCommand_;
public boolean hasRegisterKeyNotifCommand() { return hasRegisterKeyNotifCommand; }
public com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand getRegisterKeyNotifCommand() { return registerKeyNotifCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RegisterNotifCommand registerNotifCommand = 27;
public static final int REGISTERNOTIFCOMMAND_FIELD_NUMBER = 27;
private boolean hasRegisterNotifCommand;
private com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand registerNotifCommand_;
public boolean hasRegisterNotifCommand() { return hasRegisterNotifCommand; }
public com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand getRegisterNotifCommand() { return registerNotifCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RemoveCommand removeCommand = 28;
public static final int REMOVECOMMAND_FIELD_NUMBER = 28;
private boolean hasRemoveCommand;
private com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand removeCommand_;
public boolean hasRemoveCommand() { return hasRemoveCommand; }
public com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand getRemoveCommand() { return removeCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RemoveGroupCommand removeGroupCommand = 29;
public static final int REMOVEGROUPCOMMAND_FIELD_NUMBER = 29;
private boolean hasRemoveGroupCommand;
private com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand removeGroupCommand_;
public boolean hasRemoveGroupCommand() { return hasRemoveGroupCommand; }
public com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand getRemoveGroupCommand() { return removeGroupCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SearchCommand searchCommand = 30;
public static final int SEARCHCOMMAND_FIELD_NUMBER = 30;
private boolean hasSearchCommand;
private com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand searchCommand_;
public boolean hasSearchCommand() { return hasSearchCommand; }
public com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand getSearchCommand() { return searchCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetTagCommand getTagCommand = 31;
public static final int GETTAGCOMMAND_FIELD_NUMBER = 31;
private boolean hasGetTagCommand;
private com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand getTagCommand_;
public boolean hasGetTagCommand() { return hasGetTagCommand; }
public com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand getGetTagCommand() { return getTagCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.LockCommand lockCommand = 32;
public static final int LOCKCOMMAND_FIELD_NUMBER = 32;
private boolean hasLockCommand;
private com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand lockCommand_;
public boolean hasLockCommand() { return hasLockCommand; }
public com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand getLockCommand() { return lockCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.UnlockCommand unlockCommand = 33;
public static final int UNLOCKCOMMAND_FIELD_NUMBER = 33;
private boolean hasUnlockCommand;
private com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand unlockCommand_;
public boolean hasUnlockCommand() { return hasUnlockCommand; }
public com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand getUnlockCommand() { return unlockCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.IsLockedCommand isLockedCommand = 34;
public static final int ISLOCKEDCOMMAND_FIELD_NUMBER = 34;
private boolean hasIsLockedCommand;
private com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand isLockedCommand_;
public boolean hasIsLockedCommand() { return hasIsLockedCommand; }
public com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand getIsLockedCommand() { return isLockedCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.LockVerifyCommand lockVerifyCommand = 35;
public static final int LOCKVERIFYCOMMAND_FIELD_NUMBER = 35;
private boolean hasLockVerifyCommand;
private com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand lockVerifyCommand_;
public boolean hasLockVerifyCommand() { return hasLockVerifyCommand; }
public com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand getLockVerifyCommand() { return lockVerifyCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommand unRegisterKeyNotifCommand = 36;
public static final int UNREGISTERKEYNOTIFCOMMAND_FIELD_NUMBER = 36;
private boolean hasUnRegisterKeyNotifCommand;
private com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand unRegisterKeyNotifCommand_;
public boolean hasUnRegisterKeyNotifCommand() { return hasUnRegisterKeyNotifCommand; }
public com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand getUnRegisterKeyNotifCommand() { return unRegisterKeyNotifCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommand unRegisterBulkKeyNotifCommand = 37;
public static final int UNREGISTERBULKKEYNOTIFCOMMAND_FIELD_NUMBER = 37;
private boolean hasUnRegisterBulkKeyNotifCommand;
private com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand unRegisterBulkKeyNotifCommand_;
public boolean hasUnRegisterBulkKeyNotifCommand() { return hasUnRegisterBulkKeyNotifCommand; }
public com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand getUnRegisterBulkKeyNotifCommand() { return unRegisterBulkKeyNotifCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommand registerBulkKeyNotifCommand = 38;
public static final int REGISTERBULKKEYNOTIFCOMMAND_FIELD_NUMBER = 38;
private boolean hasRegisterBulkKeyNotifCommand;
private com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand registerBulkKeyNotifCommand_;
public boolean hasRegisterBulkKeyNotifCommand() { return hasRegisterBulkKeyNotifCommand; }
public com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand getRegisterBulkKeyNotifCommand() { return registerBulkKeyNotifCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.HybridBulkCommand hybridBulkCommand = 39;
public static final int HYBRIDBULKCOMMAND_FIELD_NUMBER = 39;
private boolean hasHybridBulkCommand;
private com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand hybridBulkCommand_;
public boolean hasHybridBulkCommand() { return hasHybridBulkCommand; }
public com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand getHybridBulkCommand() { return hybridBulkCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommand getLoggingInfoCommand = 40;
public static final int GETLOGGINGINFOCOMMAND_FIELD_NUMBER = 40;
private boolean hasGetLoggingInfoCommand;
private com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand getLoggingInfoCommand_;
public boolean hasGetLoggingInfoCommand() { return hasGetLoggingInfoCommand; }
public com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand getGetLoggingInfoCommand() { return getLoggingInfoCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.CloseStreamCommand closeStreamCommand = 41;
public static final int CLOSESTREAMCOMMAND_FIELD_NUMBER = 41;
private boolean hasCloseStreamCommand;
private com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand closeStreamCommand_;
public boolean hasCloseStreamCommand() { return hasCloseStreamCommand; }
public com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand getCloseStreamCommand() { return closeStreamCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetStreamLengthCommand getStreamLengthCommand = 42;
public static final int GETSTREAMLENGTHCOMMAND_FIELD_NUMBER = 42;
private boolean hasGetStreamLengthCommand;
private com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand getStreamLengthCommand_;
public boolean hasGetStreamLengthCommand() { return hasGetStreamLengthCommand; }
public com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand getGetStreamLengthCommand() { return getStreamLengthCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.OpenStreamCommand openStreamCommand = 43;
public static final int OPENSTREAMCOMMAND_FIELD_NUMBER = 43;
private boolean hasOpenStreamCommand;
private com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand openStreamCommand_;
public boolean hasOpenStreamCommand() { return hasOpenStreamCommand; }
public com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand getOpenStreamCommand() { return openStreamCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.WriteToStreamCommand writeToStreamCommand = 44;
public static final int WRITETOSTREAMCOMMAND_FIELD_NUMBER = 44;
private boolean hasWriteToStreamCommand;
private com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand writeToStreamCommand_;
public boolean hasWriteToStreamCommand() { return hasWriteToStreamCommand; }
public com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand getWriteToStreamCommand() { return writeToStreamCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.ReadFromStreamCommand readFromStreamCommand = 45;
public static final int READFROMSTREAMCOMMAND_FIELD_NUMBER = 45;
private boolean hasReadFromStreamCommand;
private com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand readFromStreamCommand_;
public boolean hasReadFromStreamCommand() { return hasReadFromStreamCommand; }
public com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand getReadFromStreamCommand() { return readFromStreamCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommand bridgeGetStateTransferInfoCommand = 46;
public static final int BRIDGEGETSTATETRANSFERINFOCOMMAND_FIELD_NUMBER = 46;
private boolean hasBridgeGetStateTransferInfoCommand;
private com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand bridgeGetStateTransferInfoCommand_;
public boolean hasBridgeGetStateTransferInfoCommand() { return hasBridgeGetStateTransferInfoCommand; }
public com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand getBridgeGetStateTransferInfoCommand() { return bridgeGetStateTransferInfoCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommand bridgeGetTransferableKeyListCommand = 47;
public static final int BRIDGEGETTRANSFERABLEKEYLISTCOMMAND_FIELD_NUMBER = 47;
private boolean hasBridgeGetTransferableKeyListCommand;
private com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand bridgeGetTransferableKeyListCommand_;
public boolean hasBridgeGetTransferableKeyListCommand() { return hasBridgeGetTransferableKeyListCommand; }
public com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand getBridgeGetTransferableKeyListCommand() { return bridgeGetTransferableKeyListCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommand bridgeHybridBulkCommand = 48;
public static final int BRIDGEHYBRIDBULKCOMMAND_FIELD_NUMBER = 48;
private boolean hasBridgeHybridBulkCommand;
private com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand bridgeHybridBulkCommand_;
public boolean hasBridgeHybridBulkCommand() { return hasBridgeHybridBulkCommand; }
public com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand getBridgeHybridBulkCommand() { return bridgeHybridBulkCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BridgeInitCommand bridgeInitCommand = 49;
public static final int BRIDGEINITCOMMAND_FIELD_NUMBER = 49;
private boolean hasBridgeInitCommand;
private com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand bridgeInitCommand_;
public boolean hasBridgeInitCommand() { return hasBridgeInitCommand; }
public com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand getBridgeInitCommand() { return bridgeInitCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommand bridgeSetTransferableKeyListCommand = 50;
public static final int BRIDGESETTRANSFERABLEKEYLISTCOMMAND_FIELD_NUMBER = 50;
private boolean hasBridgeSetTransferableKeyListCommand;
private com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand bridgeSetTransferableKeyListCommand_;
public boolean hasBridgeSetTransferableKeyListCommand() { return hasBridgeSetTransferableKeyListCommand; }
public com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand getBridgeSetTransferableKeyListCommand() { return bridgeSetTransferableKeyListCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommand bridgeSignlEndOfStateTransferCommand = 51;
public static final int BRIDGESIGNLENDOFSTATETRANSFERCOMMAND_FIELD_NUMBER = 51;
private boolean hasBridgeSignlEndOfStateTransferCommand;
private com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand bridgeSignlEndOfStateTransferCommand_;
public boolean hasBridgeSignlEndOfStateTransferCommand() { return hasBridgeSignlEndOfStateTransferCommand; }
public com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand getBridgeSignlEndOfStateTransferCommand() { return bridgeSignlEndOfStateTransferCommand_; }
// optional int64 requestID = 52;
public static final int REQUESTID_FIELD_NUMBER = 52;
private boolean hasRequestID;
private long requestID_ = 0L;
public boolean hasRequestID() { return hasRequestID; }
public long getRequestID() { return requestID_; }
// optional int32 commandVersion = 53 [default = 0];
public static final int COMMANDVERSION_FIELD_NUMBER = 53;
private boolean hasCommandVersion;
private int commandVersion_ = 0;
public boolean hasCommandVersion() { return hasCommandVersion; }
public int getCommandVersion() { return commandVersion_; }
// optional .com.alachisoft.ncache.common.protobuf.BridgeSyncTimeCommand bridgeSyncTimeCommand = 54;
public static final int BRIDGESYNCTIMECOMMAND_FIELD_NUMBER = 54;
private boolean hasBridgeSyncTimeCommand;
private com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand bridgeSyncTimeCommand_;
public boolean hasBridgeSyncTimeCommand() { return hasBridgeSyncTimeCommand; }
public com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand getBridgeSyncTimeCommand() { return bridgeSyncTimeCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedCommand bridgeHeartBeatReceivedCommand = 55;
public static final int BRIDGEHEARTBEATRECEIVEDCOMMAND_FIELD_NUMBER = 55;
private boolean hasBridgeHeartBeatReceivedCommand;
private com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand bridgeHeartBeatReceivedCommand_;
public boolean hasBridgeHeartBeatReceivedCommand() { return hasBridgeHeartBeatReceivedCommand; }
public com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand getBridgeHeartBeatReceivedCommand() { return bridgeHeartBeatReceivedCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RemoveByTagCommand removeByTagCommand = 56;
public static final int REMOVEBYTAGCOMMAND_FIELD_NUMBER = 56;
private boolean hasRemoveByTagCommand;
private com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand removeByTagCommand_;
public boolean hasRemoveByTagCommand() { return hasRemoveByTagCommand; }
public com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand getRemoveByTagCommand() { return removeByTagCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommand bridgeMakeTargetCacheActivePassiveCommand = 57;
public static final int BRIDGEMAKETARGETCACHEACTIVEPASSIVECOMMAND_FIELD_NUMBER = 57;
private boolean hasBridgeMakeTargetCacheActivePassiveCommand;
private com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand bridgeMakeTargetCacheActivePassiveCommand_;
public boolean hasBridgeMakeTargetCacheActivePassiveCommand() { return hasBridgeMakeTargetCacheActivePassiveCommand; }
public com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand getBridgeMakeTargetCacheActivePassiveCommand() { return bridgeMakeTargetCacheActivePassiveCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.UnRegisterCQCommand unRegisterCQCommand = 58;
public static final int UNREGISTERCQCOMMAND_FIELD_NUMBER = 58;
private boolean hasUnRegisterCQCommand;
private com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand unRegisterCQCommand_;
public boolean hasUnRegisterCQCommand() { return hasUnRegisterCQCommand; }
public com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand getUnRegisterCQCommand() { return unRegisterCQCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SearchCQCommand searchCQCommand = 59;
public static final int SEARCHCQCOMMAND_FIELD_NUMBER = 59;
private boolean hasSearchCQCommand;
private com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand searchCQCommand_;
public boolean hasSearchCQCommand() { return hasSearchCQCommand; }
public com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand getSearchCQCommand() { return searchCQCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RegisterCQCommand registerCQCommand = 60;
public static final int REGISTERCQCOMMAND_FIELD_NUMBER = 60;
private boolean hasRegisterCQCommand;
private com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand registerCQCommand_;
public boolean hasRegisterCQCommand() { return hasRegisterCQCommand; }
public com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand getRegisterCQCommand() { return registerCQCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetKeysByTagCommand getKeysByTagCommand = 61;
public static final int GETKEYSBYTAGCOMMAND_FIELD_NUMBER = 61;
private boolean hasGetKeysByTagCommand;
private com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand getKeysByTagCommand_;
public boolean hasGetKeysByTagCommand() { return hasGetKeysByTagCommand; }
public com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand getGetKeysByTagCommand() { return getKeysByTagCommand_; }
// optional int64 clientLastViewId = 62 [default = -1];
public static final int CLIENTLASTVIEWID_FIELD_NUMBER = 62;
private boolean hasClientLastViewId;
private long clientLastViewId_ = -1L;
public boolean hasClientLastViewId() { return hasClientLastViewId; }
public long getClientLastViewId() { return clientLastViewId_; }
// optional .com.alachisoft.ncache.common.protobuf.BulkDeleteCommand bulkDeleteCommand = 63;
public static final int BULKDELETECOMMAND_FIELD_NUMBER = 63;
private boolean hasBulkDeleteCommand;
private com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand bulkDeleteCommand_;
public boolean hasBulkDeleteCommand() { return hasBulkDeleteCommand; }
public com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand getBulkDeleteCommand() { return bulkDeleteCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.DeleteCommand deleteCommand = 64;
public static final int DELETECOMMAND_FIELD_NUMBER = 64;
private boolean hasDeleteCommand;
private com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand deleteCommand_;
public boolean hasDeleteCommand() { return hasDeleteCommand; }
public com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand getDeleteCommand() { return deleteCommand_; }
// optional string intendedRecipient = 65 [default = ""];
public static final int INTENDEDRECIPIENT_FIELD_NUMBER = 65;
private boolean hasIntendedRecipient;
private java.lang.String intendedRecipient_ = "";
public boolean hasIntendedRecipient() { return hasIntendedRecipient; }
public java.lang.String getIntendedRecipient() { return intendedRecipient_; }
// optional .com.alachisoft.ncache.common.protobuf.GetNextChunkCommand getNextChunkCommand = 66;
public static final int GETNEXTCHUNKCOMMAND_FIELD_NUMBER = 66;
private boolean hasGetNextChunkCommand;
private com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand getNextChunkCommand_;
public boolean hasGetNextChunkCommand() { return hasGetNextChunkCommand; }
public com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand getGetNextChunkCommand() { return getNextChunkCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommand getGroupNextChunkCommand = 67;
public static final int GETGROUPNEXTCHUNKCOMMAND_FIELD_NUMBER = 67;
private boolean hasGetGroupNextChunkCommand;
private com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand getGroupNextChunkCommand_;
public boolean hasGetGroupNextChunkCommand() { return hasGetGroupNextChunkCommand; }
public com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand getGetGroupNextChunkCommand() { return getGroupNextChunkCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.AddAttributeCommand addAttributeCommand = 68;
public static final int ADDATTRIBUTECOMMAND_FIELD_NUMBER = 68;
private boolean hasAddAttributeCommand;
private com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand addAttributeCommand_;
public boolean hasAddAttributeCommand() { return hasAddAttributeCommand; }
public com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand getAddAttributeCommand() { return addAttributeCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetEncryptionCommand getEncryptionCommand = 69;
public static final int GETENCRYPTIONCOMMAND_FIELD_NUMBER = 69;
private boolean hasGetEncryptionCommand;
private com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand getEncryptionCommand_;
public boolean hasGetEncryptionCommand() { return hasGetEncryptionCommand; }
public com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand getGetEncryptionCommand() { return getEncryptionCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetRunningServersCommand getRunningServersCommand = 70;
public static final int GETRUNNINGSERVERSCOMMAND_FIELD_NUMBER = 70;
private boolean hasGetRunningServersCommand;
private com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand getRunningServersCommand_;
public boolean hasGetRunningServersCommand() { return hasGetRunningServersCommand; }
public com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand getGetRunningServersCommand() { return getRunningServersCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SyncEventsCommand syncEventsCommand = 71;
public static final int SYNCEVENTSCOMMAND_FIELD_NUMBER = 71;
private boolean hasSyncEventsCommand;
private com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand syncEventsCommand_;
public boolean hasSyncEventsCommand() { return hasSyncEventsCommand; }
public com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand getSyncEventsCommand() { return syncEventsCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.DeleteQueryCommand deleteQueryCommand = 72;
public static final int DELETEQUERYCOMMAND_FIELD_NUMBER = 72;
private boolean hasDeleteQueryCommand;
private com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand deleteQueryCommand_;
public boolean hasDeleteQueryCommand() { return hasDeleteQueryCommand; }
public com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand getDeleteQueryCommand() { return deleteQueryCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetProductVersionCommand getProductVersionCommand = 73;
public static final int GETPRODUCTVERSIONCOMMAND_FIELD_NUMBER = 73;
private boolean hasGetProductVersionCommand;
private com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand getProductVersionCommand_;
public boolean hasGetProductVersionCommand() { return hasGetProductVersionCommand; }
public com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand getGetProductVersionCommand() { return getProductVersionCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommand bridgeGetReplicatorStatusInfoCommand = 74;
public static final int BRIDGEGETREPLICATORSTATUSINFOCOMMAND_FIELD_NUMBER = 74;
private boolean hasBridgeGetReplicatorStatusInfoCommand;
private com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand bridgeGetReplicatorStatusInfoCommand_;
public boolean hasBridgeGetReplicatorStatusInfoCommand() { return hasBridgeGetReplicatorStatusInfoCommand; }
public com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand getBridgeGetReplicatorStatusInfoCommand() { return bridgeGetReplicatorStatusInfoCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RequestStateTransferCommand bridgeRequestStateTransferCommand = 75;
public static final int BRIDGEREQUESTSTATETRANSFERCOMMAND_FIELD_NUMBER = 75;
private boolean hasBridgeRequestStateTransferCommand;
private com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand bridgeRequestStateTransferCommand_;
public boolean hasBridgeRequestStateTransferCommand() { return hasBridgeRequestStateTransferCommand; }
public com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand getBridgeRequestStateTransferCommand() { return bridgeRequestStateTransferCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetServerMappingCommand getServerMappingCommand = 76;
public static final int GETSERVERMAPPINGCOMMAND_FIELD_NUMBER = 76;
private boolean hasGetServerMappingCommand;
private com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand getServerMappingCommand_;
public boolean hasGetServerMappingCommand() { return hasGetServerMappingCommand; }
public com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand getGetServerMappingCommand() { return getServerMappingCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.InquiryRequestCommand inquiryRequestCommand = 77;
public static final int INQUIRYREQUESTCOMMAND_FIELD_NUMBER = 77;
private boolean hasInquiryRequestCommand;
private com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand inquiryRequestCommand_;
public boolean hasInquiryRequestCommand() { return hasInquiryRequestCommand; }
public com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand getInquiryRequestCommand() { return inquiryRequestCommand_; }
// optional bool isRetryCommand = 78 [default = false];
public static final int ISRETRYCOMMAND_FIELD_NUMBER = 78;
private boolean hasIsRetryCommand;
private boolean isRetryCommand_ = false;
public boolean hasIsRetryCommand() { return hasIsRetryCommand; }
public boolean getIsRetryCommand() { return isRetryCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.MapReduceTaskCommand mapReduceTaskCommand = 79;
public static final int MAPREDUCETASKCOMMAND_FIELD_NUMBER = 79;
private boolean hasMapReduceTaskCommand;
private com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand mapReduceTaskCommand_;
public boolean hasMapReduceTaskCommand() { return hasMapReduceTaskCommand; }
public com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand getMapReduceTaskCommand() { return mapReduceTaskCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.TaskCallbackCommand TaskCallbackCommand = 80;
public static final int TASKCALLBACKCOMMAND_FIELD_NUMBER = 80;
private boolean hasTaskCallbackCommand;
private com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand taskCallbackCommand_;
public boolean hasTaskCallbackCommand() { return hasTaskCallbackCommand; }
public com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand getTaskCallbackCommand() { return taskCallbackCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetRunningTasksCommand RunningTasksCommand = 81;
public static final int RUNNINGTASKSCOMMAND_FIELD_NUMBER = 81;
private boolean hasRunningTasksCommand;
private com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand runningTasksCommand_;
public boolean hasRunningTasksCommand() { return hasRunningTasksCommand; }
public com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand getRunningTasksCommand() { return runningTasksCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.TaskCancelCommand TaskCancelCommand = 82;
public static final int TASKCANCELCOMMAND_FIELD_NUMBER = 82;
private boolean hasTaskCancelCommand;
private com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand taskCancelCommand_;
public boolean hasTaskCancelCommand() { return hasTaskCancelCommand; }
public com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand getTaskCancelCommand() { return taskCancelCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.TaskProgressCommand TaskProgressCommand = 83;
public static final int TASKPROGRESSCOMMAND_FIELD_NUMBER = 83;
private boolean hasTaskProgressCommand;
private com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand taskProgressCommand_;
public boolean hasTaskProgressCommand() { return hasTaskProgressCommand; }
public com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand getTaskProgressCommand() { return taskProgressCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetNextRecordCommand NextRecordCommand = 84;
public static final int NEXTRECORDCOMMAND_FIELD_NUMBER = 84;
private boolean hasNextRecordCommand;
private com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand nextRecordCommand_;
public boolean hasNextRecordCommand() { return hasNextRecordCommand; }
public com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand getNextRecordCommand() { return nextRecordCommand_; }
// optional int32 commandID = 85 [default = -1];
public static final int COMMANDID_FIELD_NUMBER = 85;
private boolean hasCommandID;
private int commandID_ = -1;
public boolean hasCommandID() { return hasCommandID; }
public int getCommandID() { return commandID_; }
// optional .com.alachisoft.ncache.common.protobuf.GetCacheBindingCommand getCacheBindingCommand = 86;
public static final int GETCACHEBINDINGCOMMAND_FIELD_NUMBER = 86;
private boolean hasGetCacheBindingCommand;
private com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand getCacheBindingCommand_;
public boolean hasGetCacheBindingCommand() { return hasGetCacheBindingCommand; }
public com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand getGetCacheBindingCommand() { return getCacheBindingCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommand TaskEnumeratorCommand = 87;
public static final int TASKENUMERATORCOMMAND_FIELD_NUMBER = 87;
private boolean hasTaskEnumeratorCommand;
private com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand taskEnumeratorCommand_;
public boolean hasTaskEnumeratorCommand() { return hasTaskEnumeratorCommand; }
public com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand getTaskEnumeratorCommand() { return taskEnumeratorCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorCommand invokeEntryProcessorCommand = 88;
public static final int INVOKEENTRYPROCESSORCOMMAND_FIELD_NUMBER = 88;
private boolean hasInvokeEntryProcessorCommand;
private com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand invokeEntryProcessorCommand_;
public boolean hasInvokeEntryProcessorCommand() { return hasInvokeEntryProcessorCommand; }
public com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand getInvokeEntryProcessorCommand() { return invokeEntryProcessorCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.ExecuteReaderCommand executeReaderCommand = 89;
public static final int EXECUTEREADERCOMMAND_FIELD_NUMBER = 89;
private boolean hasExecuteReaderCommand;
private com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand executeReaderCommand_;
public boolean hasExecuteReaderCommand() { return hasExecuteReaderCommand; }
public com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand getExecuteReaderCommand() { return executeReaderCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommand getReaderNextChunkCommand = 90;
public static final int GETREADERNEXTCHUNKCOMMAND_FIELD_NUMBER = 90;
private boolean hasGetReaderNextChunkCommand;
private com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand getReaderNextChunkCommand_;
public boolean hasGetReaderNextChunkCommand() { return hasGetReaderNextChunkCommand; }
public com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand getGetReaderNextChunkCommand() { return getReaderNextChunkCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.DisposeReaderCommand disposeReaderCommand = 91;
public static final int DISPOSEREADERCOMMAND_FIELD_NUMBER = 91;
private boolean hasDisposeReaderCommand;
private com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand disposeReaderCommand_;
public boolean hasDisposeReaderCommand() { return hasDisposeReaderCommand; }
public com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand getDisposeReaderCommand() { return disposeReaderCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommand executeReaderCQCommand = 92;
public static final int EXECUTEREADERCQCOMMAND_FIELD_NUMBER = 92;
private boolean hasExecuteReaderCQCommand;
private com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand executeReaderCQCommand_;
public boolean hasExecuteReaderCQCommand() { return hasExecuteReaderCQCommand; }
public com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand getExecuteReaderCQCommand() { return executeReaderCQCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetExpirationCommand getExpirationCommand = 93;
public static final int GETEXPIRATIONCOMMAND_FIELD_NUMBER = 93;
private boolean hasGetExpirationCommand;
private com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand getExpirationCommand_;
public boolean hasGetExpirationCommand() { return hasGetExpirationCommand; }
public com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand getGetExpirationCommand() { return getExpirationCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetLCCommand getLCCommand = 94;
public static final int GETLCCOMMAND_FIELD_NUMBER = 94;
private boolean hasGetLCCommand;
private com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand getLCCommand_;
public boolean hasGetLCCommand() { return hasGetLCCommand; }
public com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand getGetLCCommand() { return getLCCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommand cacheSecurityAuthorizationCommand = 95;
public static final int CACHESECURITYAUTHORIZATIONCOMMAND_FIELD_NUMBER = 95;
private boolean hasCacheSecurityAuthorizationCommand;
private com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand cacheSecurityAuthorizationCommand_;
public boolean hasCacheSecurityAuthorizationCommand() { return hasCacheSecurityAuthorizationCommand; }
public com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand getCacheSecurityAuthorizationCommand() { return cacheSecurityAuthorizationCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.PollCommand pollCommand = 96;
public static final int POLLCOMMAND_FIELD_NUMBER = 96;
private boolean hasPollCommand;
private com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand pollCommand_;
public boolean hasPollCommand() { return hasPollCommand; }
public com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand getPollCommand() { return pollCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommand registerPollNotifCommand = 97;
public static final int REGISTERPOLLNOTIFCOMMAND_FIELD_NUMBER = 97;
private boolean hasRegisterPollNotifCommand;
private com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand registerPollNotifCommand_;
public boolean hasRegisterPollNotifCommand() { return hasRegisterPollNotifCommand; }
public com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand getRegisterPollNotifCommand() { return registerPollNotifCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommand getConnectedClientsCommand = 98;
public static final int GETCONNECTEDCLIENTSCOMMAND_FIELD_NUMBER = 98;
private boolean hasGetConnectedClientsCommand;
private com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand getConnectedClientsCommand_;
public boolean hasGetConnectedClientsCommand() { return hasGetConnectedClientsCommand; }
public com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand getGetConnectedClientsCommand() { return getConnectedClientsCommand_; }
// optional int32 MethodOverload = 99 [default = 0];
public static final int METHODOVERLOAD_FIELD_NUMBER = 99;
private boolean hasMethodOverload;
private int methodOverload_ = 0;
public boolean hasMethodOverload() { return hasMethodOverload; }
public int getMethodOverload() { return methodOverload_; }
// optional .com.alachisoft.ncache.common.protobuf.TouchCommand touchCommand = 100;
public static final int TOUCHCOMMAND_FIELD_NUMBER = 100;
private boolean hasTouchCommand;
private com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand touchCommand_;
public boolean hasTouchCommand() { return hasTouchCommand; }
public com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand getTouchCommand() { return touchCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommand getCacheManagementPortCommand = 101;
public static final int GETCACHEMANAGEMENTPORTCOMMAND_FIELD_NUMBER = 101;
private boolean hasGetCacheManagementPortCommand;
private com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand getCacheManagementPortCommand_;
public boolean hasGetCacheManagementPortCommand() { return hasGetCacheManagementPortCommand; }
public com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand getGetCacheManagementPortCommand() { return getCacheManagementPortCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetTopicCommand getTopicCommand = 102;
public static final int GETTOPICCOMMAND_FIELD_NUMBER = 102;
private boolean hasGetTopicCommand;
private com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand getTopicCommand_;
public boolean hasGetTopicCommand() { return hasGetTopicCommand; }
public com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand getGetTopicCommand() { return getTopicCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SubscribeTopicCommand subscribeTopicCommand = 103;
public static final int SUBSCRIBETOPICCOMMAND_FIELD_NUMBER = 103;
private boolean hasSubscribeTopicCommand;
private com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand subscribeTopicCommand_;
public boolean hasSubscribeTopicCommand() { return hasSubscribeTopicCommand; }
public com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand getSubscribeTopicCommand() { return subscribeTopicCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.RemoveTopicCommand removeTopicCommand = 104;
public static final int REMOVETOPICCOMMAND_FIELD_NUMBER = 104;
private boolean hasRemoveTopicCommand;
private com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand removeTopicCommand_;
public boolean hasRemoveTopicCommand() { return hasRemoveTopicCommand; }
public com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand getRemoveTopicCommand() { return removeTopicCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommand unSubscribeTopicCommand = 105;
public static final int UNSUBSCRIBETOPICCOMMAND_FIELD_NUMBER = 105;
private boolean hasUnSubscribeTopicCommand;
private com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand unSubscribeTopicCommand_;
public boolean hasUnSubscribeTopicCommand() { return hasUnSubscribeTopicCommand; }
public com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand getUnSubscribeTopicCommand() { return unSubscribeTopicCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.MessagePublishCommand messagePublishCommand = 106;
public static final int MESSAGEPUBLISHCOMMAND_FIELD_NUMBER = 106;
private boolean hasMessagePublishCommand;
private com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand messagePublishCommand_;
public boolean hasMessagePublishCommand() { return hasMessagePublishCommand; }
public com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand getMessagePublishCommand() { return messagePublishCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.GetMessageCommand getMessageCommand = 107;
public static final int GETMESSAGECOMMAND_FIELD_NUMBER = 107;
private boolean hasGetMessageCommand;
private com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand getMessageCommand_;
public boolean hasGetMessageCommand() { return hasGetMessageCommand; }
public com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand getGetMessageCommand() { return getMessageCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommand mesasgeAcknowledgmentCommand = 108;
public static final int MESASGEACKNOWLEDGMENTCOMMAND_FIELD_NUMBER = 108;
private boolean hasMesasgeAcknowledgmentCommand;
private com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand mesasgeAcknowledgmentCommand_;
public boolean hasMesasgeAcknowledgmentCommand() { return hasMesasgeAcknowledgmentCommand; }
public com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand getMesasgeAcknowledgmentCommand() { return mesasgeAcknowledgmentCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.PingCommand pingCommand = 109;
public static final int PINGCOMMAND_FIELD_NUMBER = 109;
private boolean hasPingCommand;
private com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand pingCommand_;
public boolean hasPingCommand() { return hasPingCommand; }
public com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand getPingCommand() { return pingCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.MessageCountCommand messageCountCommand = 110;
public static final int MESSAGECOUNTCOMMAND_FIELD_NUMBER = 110;
private boolean hasMessageCountCommand;
private com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand messageCountCommand_;
public boolean hasMessageCountCommand() { return hasMessageCountCommand; }
public com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand getMessageCountCommand() { return messageCountCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.DataTypes dataTypes = 111;
public static final int DATATYPES_FIELD_NUMBER = 111;
private boolean hasDataTypes;
private com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes dataTypes_;
public boolean hasDataTypes() { return hasDataTypes; }
public com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes getDataTypes() { return dataTypes_; }
// optional .com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommand getSerializationFormatCommand = 112;
public static final int GETSERIALIZATIONFORMATCOMMAND_FIELD_NUMBER = 112;
private boolean hasGetSerializationFormatCommand;
private com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand getSerializationFormatCommand_;
public boolean hasGetSerializationFormatCommand() { return hasGetSerializationFormatCommand; }
public com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand getGetSerializationFormatCommand() { return getSerializationFormatCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommand bulkGetCacheItemCommand = 113;
public static final int BULKGETCACHEITEMCOMMAND_FIELD_NUMBER = 113;
private boolean hasBulkGetCacheItemCommand;
private com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand bulkGetCacheItemCommand_;
public boolean hasBulkGetCacheItemCommand() { return hasBulkGetCacheItemCommand; }
public com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand getBulkGetCacheItemCommand() { return bulkGetCacheItemCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.ContainsBulkCommand containsBulkCommand = 114;
public static final int CONTAINSBULKCOMMAND_FIELD_NUMBER = 114;
private boolean hasContainsBulkCommand;
private com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand containsBulkCommand_;
public boolean hasContainsBulkCommand() { return hasContainsBulkCommand; }
public com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand getContainsBulkCommand() { return containsBulkCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.ModuleCommand moduleCommand = 115;
public static final int MODULECOMMAND_FIELD_NUMBER = 115;
private boolean hasModuleCommand;
private com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand moduleCommand_;
public boolean hasModuleCommand() { return hasModuleCommand; }
public com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand getModuleCommand() { return moduleCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SurrogateCommand surrogateCommand = 116;
public static final int SURROGATECOMMAND_FIELD_NUMBER = 116;
private boolean hasSurrogateCommand;
private com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand surrogateCommand_;
public boolean hasSurrogateCommand() { return hasSurrogateCommand; }
public com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand getSurrogateCommand() { return surrogateCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommand messagePublishBulkCommand = 117;
public static final int MESSAGEPUBLISHBULKCOMMAND_FIELD_NUMBER = 117;
private boolean hasMessagePublishBulkCommand;
private com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand messagePublishBulkCommand_;
public boolean hasMessagePublishBulkCommand() { return hasMessagePublishBulkCommand; }
public com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand getMessagePublishBulkCommand() { return messagePublishBulkCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplication bridgePollForResumingReplication = 118;
public static final int BRIDGEPOLLFORRESUMINGREPLICATION_FIELD_NUMBER = 118;
private boolean hasBridgePollForResumingReplication;
private com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication bridgePollForResumingReplication_;
public boolean hasBridgePollForResumingReplication() { return hasBridgePollForResumingReplication; }
public com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication getBridgePollForResumingReplication() { return bridgePollForResumingReplication_; }
// optional .com.alachisoft.ncache.common.protobuf.GetModuleStateCommand getModuleStateCommand = 119;
public static final int GETMODULESTATECOMMAND_FIELD_NUMBER = 119;
private boolean hasGetModuleStateCommand;
private com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand getModuleStateCommand_;
public boolean hasGetModuleStateCommand() { return hasGetModuleStateCommand; }
public com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand getGetModuleStateCommand() { return getModuleStateCommand_; }
// optional .com.alachisoft.ncache.common.protobuf.SetModuleStateCommand setModuleStateCommand = 120;
public static final int SETMODULESTATECOMMAND_FIELD_NUMBER = 120;
private boolean hasSetModuleStateCommand;
private com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand setModuleStateCommand_;
public boolean hasSetModuleStateCommand() { return hasSetModuleStateCommand; }
public com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand getSetModuleStateCommand() { return setModuleStateCommand_; }
private void initFields() {
type_ = com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type.ADD;
addCommand_ = com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand.getDefaultInstance();
addDependencyCommand_ = com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand.getDefaultInstance();
addSyncDependencyCommand_ = com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand.getDefaultInstance();
bulkAddCommand_ = com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand.getDefaultInstance();
bulkGetCommand_ = com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand.getDefaultInstance();
bulkInsertCommand_ = com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand.getDefaultInstance();
bulkRemoveCommand_ = com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand.getDefaultInstance();
clearCommand_ = com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand.getDefaultInstance();
containsCommand_ = com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand.getDefaultInstance();
countCommand_ = com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand.getDefaultInstance();
disposeCommand_ = com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand.getDefaultInstance();
getCacheItemCommand_ = com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand.getDefaultInstance();
getCommand_ = com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand.getDefaultInstance();
getCompactTypesCommand_ = com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand.getDefaultInstance();
getEnumeratorCommand_ = com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand.getDefaultInstance();
getGroupCommand_ = com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand.getDefaultInstance();
getHashmapCommand_ = com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand.getDefaultInstance();
getOptimalServerCommand_ = com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand.getDefaultInstance();
getThresholdSizeCommand_ = com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand.getDefaultInstance();
getTypeInfoMapCommand_ = com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand.getDefaultInstance();
initCommand_ = com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand.getDefaultInstance();
insertCommand_ = com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand.getDefaultInstance();
raiseCustomEventCommand_ = com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand.getDefaultInstance();
registerKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand.getDefaultInstance();
registerNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand.getDefaultInstance();
removeCommand_ = com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand.getDefaultInstance();
removeGroupCommand_ = com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand.getDefaultInstance();
searchCommand_ = com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand.getDefaultInstance();
getTagCommand_ = com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand.getDefaultInstance();
lockCommand_ = com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand.getDefaultInstance();
unlockCommand_ = com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand.getDefaultInstance();
isLockedCommand_ = com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand.getDefaultInstance();
lockVerifyCommand_ = com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand.getDefaultInstance();
unRegisterKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand.getDefaultInstance();
unRegisterBulkKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand.getDefaultInstance();
registerBulkKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand.getDefaultInstance();
hybridBulkCommand_ = com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand.getDefaultInstance();
getLoggingInfoCommand_ = com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand.getDefaultInstance();
closeStreamCommand_ = com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand.getDefaultInstance();
getStreamLengthCommand_ = com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand.getDefaultInstance();
openStreamCommand_ = com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand.getDefaultInstance();
writeToStreamCommand_ = com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand.getDefaultInstance();
readFromStreamCommand_ = com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand.getDefaultInstance();
bridgeGetStateTransferInfoCommand_ = com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand.getDefaultInstance();
bridgeGetTransferableKeyListCommand_ = com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand.getDefaultInstance();
bridgeHybridBulkCommand_ = com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand.getDefaultInstance();
bridgeInitCommand_ = com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand.getDefaultInstance();
bridgeSetTransferableKeyListCommand_ = com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand.getDefaultInstance();
bridgeSignlEndOfStateTransferCommand_ = com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand.getDefaultInstance();
bridgeSyncTimeCommand_ = com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand.getDefaultInstance();
bridgeHeartBeatReceivedCommand_ = com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand.getDefaultInstance();
removeByTagCommand_ = com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand.getDefaultInstance();
bridgeMakeTargetCacheActivePassiveCommand_ = com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand.getDefaultInstance();
unRegisterCQCommand_ = com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand.getDefaultInstance();
searchCQCommand_ = com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand.getDefaultInstance();
registerCQCommand_ = com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand.getDefaultInstance();
getKeysByTagCommand_ = com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand.getDefaultInstance();
bulkDeleteCommand_ = com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand.getDefaultInstance();
deleteCommand_ = com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand.getDefaultInstance();
getNextChunkCommand_ = com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand.getDefaultInstance();
getGroupNextChunkCommand_ = com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand.getDefaultInstance();
addAttributeCommand_ = com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand.getDefaultInstance();
getEncryptionCommand_ = com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand.getDefaultInstance();
getRunningServersCommand_ = com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand.getDefaultInstance();
syncEventsCommand_ = com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand.getDefaultInstance();
deleteQueryCommand_ = com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand.getDefaultInstance();
getProductVersionCommand_ = com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand.getDefaultInstance();
bridgeGetReplicatorStatusInfoCommand_ = com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand.getDefaultInstance();
bridgeRequestStateTransferCommand_ = com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand.getDefaultInstance();
getServerMappingCommand_ = com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand.getDefaultInstance();
inquiryRequestCommand_ = com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand.getDefaultInstance();
mapReduceTaskCommand_ = com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand.getDefaultInstance();
taskCallbackCommand_ = com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand.getDefaultInstance();
runningTasksCommand_ = com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand.getDefaultInstance();
taskCancelCommand_ = com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand.getDefaultInstance();
taskProgressCommand_ = com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand.getDefaultInstance();
nextRecordCommand_ = com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand.getDefaultInstance();
getCacheBindingCommand_ = com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand.getDefaultInstance();
taskEnumeratorCommand_ = com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand.getDefaultInstance();
invokeEntryProcessorCommand_ = com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand.getDefaultInstance();
executeReaderCommand_ = com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand.getDefaultInstance();
getReaderNextChunkCommand_ = com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand.getDefaultInstance();
disposeReaderCommand_ = com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand.getDefaultInstance();
executeReaderCQCommand_ = com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand.getDefaultInstance();
getExpirationCommand_ = com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand.getDefaultInstance();
getLCCommand_ = com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand.getDefaultInstance();
cacheSecurityAuthorizationCommand_ = com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand.getDefaultInstance();
pollCommand_ = com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand.getDefaultInstance();
registerPollNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand.getDefaultInstance();
getConnectedClientsCommand_ = com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand.getDefaultInstance();
touchCommand_ = com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand.getDefaultInstance();
getCacheManagementPortCommand_ = com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand.getDefaultInstance();
getTopicCommand_ = com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand.getDefaultInstance();
subscribeTopicCommand_ = com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand.getDefaultInstance();
removeTopicCommand_ = com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand.getDefaultInstance();
unSubscribeTopicCommand_ = com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand.getDefaultInstance();
messagePublishCommand_ = com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand.getDefaultInstance();
getMessageCommand_ = com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand.getDefaultInstance();
mesasgeAcknowledgmentCommand_ = com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand.getDefaultInstance();
pingCommand_ = com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand.getDefaultInstance();
messageCountCommand_ = com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand.getDefaultInstance();
dataTypes_ = com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes.getDefaultInstance();
getSerializationFormatCommand_ = com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand.getDefaultInstance();
bulkGetCacheItemCommand_ = com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand.getDefaultInstance();
containsBulkCommand_ = com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand.getDefaultInstance();
moduleCommand_ = com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand.getDefaultInstance();
surrogateCommand_ = com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand.getDefaultInstance();
messagePublishBulkCommand_ = com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.getDefaultInstance();
bridgePollForResumingReplication_ = com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication.getDefaultInstance();
getModuleStateCommand_ = com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand.getDefaultInstance();
setModuleStateCommand_ = com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand.getDefaultInstance();
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasType()) {
output.writeEnum(1, getType().getNumber());
}
if (hasVersion()) {
output.writeString(2, getVersion());
}
if (hasAddCommand()) {
output.writeMessage(3, getAddCommand());
}
if (hasAddDependencyCommand()) {
output.writeMessage(4, getAddDependencyCommand());
}
if (hasAddSyncDependencyCommand()) {
output.writeMessage(5, getAddSyncDependencyCommand());
}
if (hasBulkAddCommand()) {
output.writeMessage(6, getBulkAddCommand());
}
if (hasBulkGetCommand()) {
output.writeMessage(7, getBulkGetCommand());
}
if (hasBulkInsertCommand()) {
output.writeMessage(8, getBulkInsertCommand());
}
if (hasBulkRemoveCommand()) {
output.writeMessage(9, getBulkRemoveCommand());
}
if (hasClearCommand()) {
output.writeMessage(10, getClearCommand());
}
if (hasContainsCommand()) {
output.writeMessage(11, getContainsCommand());
}
if (hasCountCommand()) {
output.writeMessage(12, getCountCommand());
}
if (hasDisposeCommand()) {
output.writeMessage(13, getDisposeCommand());
}
if (hasGetCacheItemCommand()) {
output.writeMessage(14, getGetCacheItemCommand());
}
if (hasGetCommand()) {
output.writeMessage(15, getGetCommand());
}
if (hasGetCompactTypesCommand()) {
output.writeMessage(16, getGetCompactTypesCommand());
}
if (hasGetEnumeratorCommand()) {
output.writeMessage(17, getGetEnumeratorCommand());
}
if (hasGetGroupCommand()) {
output.writeMessage(18, getGetGroupCommand());
}
if (hasGetHashmapCommand()) {
output.writeMessage(19, getGetHashmapCommand());
}
if (hasGetOptimalServerCommand()) {
output.writeMessage(20, getGetOptimalServerCommand());
}
if (hasGetThresholdSizeCommand()) {
output.writeMessage(21, getGetThresholdSizeCommand());
}
if (hasGetTypeInfoMapCommand()) {
output.writeMessage(22, getGetTypeInfoMapCommand());
}
if (hasInitCommand()) {
output.writeMessage(23, getInitCommand());
}
if (hasInsertCommand()) {
output.writeMessage(24, getInsertCommand());
}
if (hasRaiseCustomEventCommand()) {
output.writeMessage(25, getRaiseCustomEventCommand());
}
if (hasRegisterKeyNotifCommand()) {
output.writeMessage(26, getRegisterKeyNotifCommand());
}
if (hasRegisterNotifCommand()) {
output.writeMessage(27, getRegisterNotifCommand());
}
if (hasRemoveCommand()) {
output.writeMessage(28, getRemoveCommand());
}
if (hasRemoveGroupCommand()) {
output.writeMessage(29, getRemoveGroupCommand());
}
if (hasSearchCommand()) {
output.writeMessage(30, getSearchCommand());
}
if (hasGetTagCommand()) {
output.writeMessage(31, getGetTagCommand());
}
if (hasLockCommand()) {
output.writeMessage(32, getLockCommand());
}
if (hasUnlockCommand()) {
output.writeMessage(33, getUnlockCommand());
}
if (hasIsLockedCommand()) {
output.writeMessage(34, getIsLockedCommand());
}
if (hasLockVerifyCommand()) {
output.writeMessage(35, getLockVerifyCommand());
}
if (hasUnRegisterKeyNotifCommand()) {
output.writeMessage(36, getUnRegisterKeyNotifCommand());
}
if (hasUnRegisterBulkKeyNotifCommand()) {
output.writeMessage(37, getUnRegisterBulkKeyNotifCommand());
}
if (hasRegisterBulkKeyNotifCommand()) {
output.writeMessage(38, getRegisterBulkKeyNotifCommand());
}
if (hasHybridBulkCommand()) {
output.writeMessage(39, getHybridBulkCommand());
}
if (hasGetLoggingInfoCommand()) {
output.writeMessage(40, getGetLoggingInfoCommand());
}
if (hasCloseStreamCommand()) {
output.writeMessage(41, getCloseStreamCommand());
}
if (hasGetStreamLengthCommand()) {
output.writeMessage(42, getGetStreamLengthCommand());
}
if (hasOpenStreamCommand()) {
output.writeMessage(43, getOpenStreamCommand());
}
if (hasWriteToStreamCommand()) {
output.writeMessage(44, getWriteToStreamCommand());
}
if (hasReadFromStreamCommand()) {
output.writeMessage(45, getReadFromStreamCommand());
}
if (hasBridgeGetStateTransferInfoCommand()) {
output.writeMessage(46, getBridgeGetStateTransferInfoCommand());
}
if (hasBridgeGetTransferableKeyListCommand()) {
output.writeMessage(47, getBridgeGetTransferableKeyListCommand());
}
if (hasBridgeHybridBulkCommand()) {
output.writeMessage(48, getBridgeHybridBulkCommand());
}
if (hasBridgeInitCommand()) {
output.writeMessage(49, getBridgeInitCommand());
}
if (hasBridgeSetTransferableKeyListCommand()) {
output.writeMessage(50, getBridgeSetTransferableKeyListCommand());
}
if (hasBridgeSignlEndOfStateTransferCommand()) {
output.writeMessage(51, getBridgeSignlEndOfStateTransferCommand());
}
if (hasRequestID()) {
output.writeInt64(52, getRequestID());
}
if (hasCommandVersion()) {
output.writeInt32(53, getCommandVersion());
}
if (hasBridgeSyncTimeCommand()) {
output.writeMessage(54, getBridgeSyncTimeCommand());
}
if (hasBridgeHeartBeatReceivedCommand()) {
output.writeMessage(55, getBridgeHeartBeatReceivedCommand());
}
if (hasRemoveByTagCommand()) {
output.writeMessage(56, getRemoveByTagCommand());
}
if (hasBridgeMakeTargetCacheActivePassiveCommand()) {
output.writeMessage(57, getBridgeMakeTargetCacheActivePassiveCommand());
}
if (hasUnRegisterCQCommand()) {
output.writeMessage(58, getUnRegisterCQCommand());
}
if (hasSearchCQCommand()) {
output.writeMessage(59, getSearchCQCommand());
}
if (hasRegisterCQCommand()) {
output.writeMessage(60, getRegisterCQCommand());
}
if (hasGetKeysByTagCommand()) {
output.writeMessage(61, getGetKeysByTagCommand());
}
if (hasClientLastViewId()) {
output.writeInt64(62, getClientLastViewId());
}
if (hasBulkDeleteCommand()) {
output.writeMessage(63, getBulkDeleteCommand());
}
if (hasDeleteCommand()) {
output.writeMessage(64, getDeleteCommand());
}
if (hasIntendedRecipient()) {
output.writeString(65, getIntendedRecipient());
}
if (hasGetNextChunkCommand()) {
output.writeMessage(66, getGetNextChunkCommand());
}
if (hasGetGroupNextChunkCommand()) {
output.writeMessage(67, getGetGroupNextChunkCommand());
}
if (hasAddAttributeCommand()) {
output.writeMessage(68, getAddAttributeCommand());
}
if (hasGetEncryptionCommand()) {
output.writeMessage(69, getGetEncryptionCommand());
}
if (hasGetRunningServersCommand()) {
output.writeMessage(70, getGetRunningServersCommand());
}
if (hasSyncEventsCommand()) {
output.writeMessage(71, getSyncEventsCommand());
}
if (hasDeleteQueryCommand()) {
output.writeMessage(72, getDeleteQueryCommand());
}
if (hasGetProductVersionCommand()) {
output.writeMessage(73, getGetProductVersionCommand());
}
if (hasBridgeGetReplicatorStatusInfoCommand()) {
output.writeMessage(74, getBridgeGetReplicatorStatusInfoCommand());
}
if (hasBridgeRequestStateTransferCommand()) {
output.writeMessage(75, getBridgeRequestStateTransferCommand());
}
if (hasGetServerMappingCommand()) {
output.writeMessage(76, getGetServerMappingCommand());
}
if (hasInquiryRequestCommand()) {
output.writeMessage(77, getInquiryRequestCommand());
}
if (hasIsRetryCommand()) {
output.writeBool(78, getIsRetryCommand());
}
if (hasMapReduceTaskCommand()) {
output.writeMessage(79, getMapReduceTaskCommand());
}
if (hasTaskCallbackCommand()) {
output.writeMessage(80, getTaskCallbackCommand());
}
if (hasRunningTasksCommand()) {
output.writeMessage(81, getRunningTasksCommand());
}
if (hasTaskCancelCommand()) {
output.writeMessage(82, getTaskCancelCommand());
}
if (hasTaskProgressCommand()) {
output.writeMessage(83, getTaskProgressCommand());
}
if (hasNextRecordCommand()) {
output.writeMessage(84, getNextRecordCommand());
}
if (hasCommandID()) {
output.writeInt32(85, getCommandID());
}
if (hasGetCacheBindingCommand()) {
output.writeMessage(86, getGetCacheBindingCommand());
}
if (hasTaskEnumeratorCommand()) {
output.writeMessage(87, getTaskEnumeratorCommand());
}
if (hasInvokeEntryProcessorCommand()) {
output.writeMessage(88, getInvokeEntryProcessorCommand());
}
if (hasExecuteReaderCommand()) {
output.writeMessage(89, getExecuteReaderCommand());
}
if (hasGetReaderNextChunkCommand()) {
output.writeMessage(90, getGetReaderNextChunkCommand());
}
if (hasDisposeReaderCommand()) {
output.writeMessage(91, getDisposeReaderCommand());
}
if (hasExecuteReaderCQCommand()) {
output.writeMessage(92, getExecuteReaderCQCommand());
}
if (hasGetExpirationCommand()) {
output.writeMessage(93, getGetExpirationCommand());
}
if (hasGetLCCommand()) {
output.writeMessage(94, getGetLCCommand());
}
if (hasCacheSecurityAuthorizationCommand()) {
output.writeMessage(95, getCacheSecurityAuthorizationCommand());
}
if (hasPollCommand()) {
output.writeMessage(96, getPollCommand());
}
if (hasRegisterPollNotifCommand()) {
output.writeMessage(97, getRegisterPollNotifCommand());
}
if (hasGetConnectedClientsCommand()) {
output.writeMessage(98, getGetConnectedClientsCommand());
}
if (hasMethodOverload()) {
output.writeInt32(99, getMethodOverload());
}
if (hasTouchCommand()) {
output.writeMessage(100, getTouchCommand());
}
if (hasGetCacheManagementPortCommand()) {
output.writeMessage(101, getGetCacheManagementPortCommand());
}
if (hasGetTopicCommand()) {
output.writeMessage(102, getGetTopicCommand());
}
if (hasSubscribeTopicCommand()) {
output.writeMessage(103, getSubscribeTopicCommand());
}
if (hasRemoveTopicCommand()) {
output.writeMessage(104, getRemoveTopicCommand());
}
if (hasUnSubscribeTopicCommand()) {
output.writeMessage(105, getUnSubscribeTopicCommand());
}
if (hasMessagePublishCommand()) {
output.writeMessage(106, getMessagePublishCommand());
}
if (hasGetMessageCommand()) {
output.writeMessage(107, getGetMessageCommand());
}
if (hasMesasgeAcknowledgmentCommand()) {
output.writeMessage(108, getMesasgeAcknowledgmentCommand());
}
if (hasPingCommand()) {
output.writeMessage(109, getPingCommand());
}
if (hasMessageCountCommand()) {
output.writeMessage(110, getMessageCountCommand());
}
if (hasDataTypes()) {
output.writeMessage(111, getDataTypes());
}
if (hasGetSerializationFormatCommand()) {
output.writeMessage(112, getGetSerializationFormatCommand());
}
if (hasBulkGetCacheItemCommand()) {
output.writeMessage(113, getBulkGetCacheItemCommand());
}
if (hasContainsBulkCommand()) {
output.writeMessage(114, getContainsBulkCommand());
}
if (hasModuleCommand()) {
output.writeMessage(115, getModuleCommand());
}
if (hasSurrogateCommand()) {
output.writeMessage(116, getSurrogateCommand());
}
if (hasMessagePublishBulkCommand()) {
output.writeMessage(117, getMessagePublishBulkCommand());
}
if (hasBridgePollForResumingReplication()) {
output.writeMessage(118, getBridgePollForResumingReplication());
}
if (hasGetModuleStateCommand()) {
output.writeMessage(119, getGetModuleStateCommand());
}
if (hasSetModuleStateCommand()) {
output.writeMessage(120, getSetModuleStateCommand());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasType()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, getType().getNumber());
}
if (hasVersion()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getVersion());
}
if (hasAddCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getAddCommand());
}
if (hasAddDependencyCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getAddDependencyCommand());
}
if (hasAddSyncDependencyCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getAddSyncDependencyCommand());
}
if (hasBulkAddCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getBulkAddCommand());
}
if (hasBulkGetCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getBulkGetCommand());
}
if (hasBulkInsertCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getBulkInsertCommand());
}
if (hasBulkRemoveCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getBulkRemoveCommand());
}
if (hasClearCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getClearCommand());
}
if (hasContainsCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getContainsCommand());
}
if (hasCountCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getCountCommand());
}
if (hasDisposeCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getDisposeCommand());
}
if (hasGetCacheItemCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getGetCacheItemCommand());
}
if (hasGetCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, getGetCommand());
}
if (hasGetCompactTypesCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getGetCompactTypesCommand());
}
if (hasGetEnumeratorCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, getGetEnumeratorCommand());
}
if (hasGetGroupCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getGetGroupCommand());
}
if (hasGetHashmapCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, getGetHashmapCommand());
}
if (hasGetOptimalServerCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, getGetOptimalServerCommand());
}
if (hasGetThresholdSizeCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getGetThresholdSizeCommand());
}
if (hasGetTypeInfoMapCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, getGetTypeInfoMapCommand());
}
if (hasInitCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, getInitCommand());
}
if (hasInsertCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, getInsertCommand());
}
if (hasRaiseCustomEventCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(25, getRaiseCustomEventCommand());
}
if (hasRegisterKeyNotifCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, getRegisterKeyNotifCommand());
}
if (hasRegisterNotifCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(27, getRegisterNotifCommand());
}
if (hasRemoveCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(28, getRemoveCommand());
}
if (hasRemoveGroupCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(29, getRemoveGroupCommand());
}
if (hasSearchCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(30, getSearchCommand());
}
if (hasGetTagCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(31, getGetTagCommand());
}
if (hasLockCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(32, getLockCommand());
}
if (hasUnlockCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(33, getUnlockCommand());
}
if (hasIsLockedCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(34, getIsLockedCommand());
}
if (hasLockVerifyCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(35, getLockVerifyCommand());
}
if (hasUnRegisterKeyNotifCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(36, getUnRegisterKeyNotifCommand());
}
if (hasUnRegisterBulkKeyNotifCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(37, getUnRegisterBulkKeyNotifCommand());
}
if (hasRegisterBulkKeyNotifCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(38, getRegisterBulkKeyNotifCommand());
}
if (hasHybridBulkCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(39, getHybridBulkCommand());
}
if (hasGetLoggingInfoCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(40, getGetLoggingInfoCommand());
}
if (hasCloseStreamCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(41, getCloseStreamCommand());
}
if (hasGetStreamLengthCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(42, getGetStreamLengthCommand());
}
if (hasOpenStreamCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(43, getOpenStreamCommand());
}
if (hasWriteToStreamCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(44, getWriteToStreamCommand());
}
if (hasReadFromStreamCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(45, getReadFromStreamCommand());
}
if (hasBridgeGetStateTransferInfoCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(46, getBridgeGetStateTransferInfoCommand());
}
if (hasBridgeGetTransferableKeyListCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(47, getBridgeGetTransferableKeyListCommand());
}
if (hasBridgeHybridBulkCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(48, getBridgeHybridBulkCommand());
}
if (hasBridgeInitCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(49, getBridgeInitCommand());
}
if (hasBridgeSetTransferableKeyListCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(50, getBridgeSetTransferableKeyListCommand());
}
if (hasBridgeSignlEndOfStateTransferCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(51, getBridgeSignlEndOfStateTransferCommand());
}
if (hasRequestID()) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(52, getRequestID());
}
if (hasCommandVersion()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(53, getCommandVersion());
}
if (hasBridgeSyncTimeCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(54, getBridgeSyncTimeCommand());
}
if (hasBridgeHeartBeatReceivedCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(55, getBridgeHeartBeatReceivedCommand());
}
if (hasRemoveByTagCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(56, getRemoveByTagCommand());
}
if (hasBridgeMakeTargetCacheActivePassiveCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(57, getBridgeMakeTargetCacheActivePassiveCommand());
}
if (hasUnRegisterCQCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(58, getUnRegisterCQCommand());
}
if (hasSearchCQCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(59, getSearchCQCommand());
}
if (hasRegisterCQCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(60, getRegisterCQCommand());
}
if (hasGetKeysByTagCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(61, getGetKeysByTagCommand());
}
if (hasClientLastViewId()) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(62, getClientLastViewId());
}
if (hasBulkDeleteCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(63, getBulkDeleteCommand());
}
if (hasDeleteCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(64, getDeleteCommand());
}
if (hasIntendedRecipient()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(65, getIntendedRecipient());
}
if (hasGetNextChunkCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(66, getGetNextChunkCommand());
}
if (hasGetGroupNextChunkCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(67, getGetGroupNextChunkCommand());
}
if (hasAddAttributeCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(68, getAddAttributeCommand());
}
if (hasGetEncryptionCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(69, getGetEncryptionCommand());
}
if (hasGetRunningServersCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(70, getGetRunningServersCommand());
}
if (hasSyncEventsCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(71, getSyncEventsCommand());
}
if (hasDeleteQueryCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(72, getDeleteQueryCommand());
}
if (hasGetProductVersionCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(73, getGetProductVersionCommand());
}
if (hasBridgeGetReplicatorStatusInfoCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(74, getBridgeGetReplicatorStatusInfoCommand());
}
if (hasBridgeRequestStateTransferCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(75, getBridgeRequestStateTransferCommand());
}
if (hasGetServerMappingCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(76, getGetServerMappingCommand());
}
if (hasInquiryRequestCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(77, getInquiryRequestCommand());
}
if (hasIsRetryCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(78, getIsRetryCommand());
}
if (hasMapReduceTaskCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(79, getMapReduceTaskCommand());
}
if (hasTaskCallbackCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(80, getTaskCallbackCommand());
}
if (hasRunningTasksCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(81, getRunningTasksCommand());
}
if (hasTaskCancelCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(82, getTaskCancelCommand());
}
if (hasTaskProgressCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(83, getTaskProgressCommand());
}
if (hasNextRecordCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(84, getNextRecordCommand());
}
if (hasCommandID()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(85, getCommandID());
}
if (hasGetCacheBindingCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(86, getGetCacheBindingCommand());
}
if (hasTaskEnumeratorCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(87, getTaskEnumeratorCommand());
}
if (hasInvokeEntryProcessorCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(88, getInvokeEntryProcessorCommand());
}
if (hasExecuteReaderCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(89, getExecuteReaderCommand());
}
if (hasGetReaderNextChunkCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(90, getGetReaderNextChunkCommand());
}
if (hasDisposeReaderCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(91, getDisposeReaderCommand());
}
if (hasExecuteReaderCQCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(92, getExecuteReaderCQCommand());
}
if (hasGetExpirationCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(93, getGetExpirationCommand());
}
if (hasGetLCCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(94, getGetLCCommand());
}
if (hasCacheSecurityAuthorizationCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(95, getCacheSecurityAuthorizationCommand());
}
if (hasPollCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(96, getPollCommand());
}
if (hasRegisterPollNotifCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(97, getRegisterPollNotifCommand());
}
if (hasGetConnectedClientsCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(98, getGetConnectedClientsCommand());
}
if (hasMethodOverload()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(99, getMethodOverload());
}
if (hasTouchCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, getTouchCommand());
}
if (hasGetCacheManagementPortCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(101, getGetCacheManagementPortCommand());
}
if (hasGetTopicCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(102, getGetTopicCommand());
}
if (hasSubscribeTopicCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(103, getSubscribeTopicCommand());
}
if (hasRemoveTopicCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(104, getRemoveTopicCommand());
}
if (hasUnSubscribeTopicCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(105, getUnSubscribeTopicCommand());
}
if (hasMessagePublishCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(106, getMessagePublishCommand());
}
if (hasGetMessageCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(107, getGetMessageCommand());
}
if (hasMesasgeAcknowledgmentCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(108, getMesasgeAcknowledgmentCommand());
}
if (hasPingCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(109, getPingCommand());
}
if (hasMessageCountCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(110, getMessageCountCommand());
}
if (hasDataTypes()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(111, getDataTypes());
}
if (hasGetSerializationFormatCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(112, getGetSerializationFormatCommand());
}
if (hasBulkGetCacheItemCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(113, getBulkGetCacheItemCommand());
}
if (hasContainsBulkCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(114, getContainsBulkCommand());
}
if (hasModuleCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(115, getModuleCommand());
}
if (hasSurrogateCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(116, getSurrogateCommand());
}
if (hasMessagePublishBulkCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(117, getMessagePublishBulkCommand());
}
if (hasBridgePollForResumingReplication()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(118, getBridgePollForResumingReplication());
}
if (hasGetModuleStateCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(119, getGetModuleStateCommand());
}
if (hasSetModuleStateCommand()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(120, getSetModuleStateCommand());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.CommandProtocol.Command parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.CommandProtocol.Command prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.CommandProtocol.Command result;
// Construct using com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.CommandProtocol.Command();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.CommandProtocol.Command internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.CommandProtocol.Command();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.CommandProtocol.Command getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.CommandProtocol.Command build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.CommandProtocol.Command buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.CommandProtocol.Command buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.CommandProtocol.Command returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.CommandProtocol.Command) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.CommandProtocol.Command)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.CommandProtocol.Command other) {
if (other == com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
if (other.hasAddCommand()) {
mergeAddCommand(other.getAddCommand());
}
if (other.hasAddDependencyCommand()) {
mergeAddDependencyCommand(other.getAddDependencyCommand());
}
if (other.hasAddSyncDependencyCommand()) {
mergeAddSyncDependencyCommand(other.getAddSyncDependencyCommand());
}
if (other.hasBulkAddCommand()) {
mergeBulkAddCommand(other.getBulkAddCommand());
}
if (other.hasBulkGetCommand()) {
mergeBulkGetCommand(other.getBulkGetCommand());
}
if (other.hasBulkInsertCommand()) {
mergeBulkInsertCommand(other.getBulkInsertCommand());
}
if (other.hasBulkRemoveCommand()) {
mergeBulkRemoveCommand(other.getBulkRemoveCommand());
}
if (other.hasClearCommand()) {
mergeClearCommand(other.getClearCommand());
}
if (other.hasContainsCommand()) {
mergeContainsCommand(other.getContainsCommand());
}
if (other.hasCountCommand()) {
mergeCountCommand(other.getCountCommand());
}
if (other.hasDisposeCommand()) {
mergeDisposeCommand(other.getDisposeCommand());
}
if (other.hasGetCacheItemCommand()) {
mergeGetCacheItemCommand(other.getGetCacheItemCommand());
}
if (other.hasGetCommand()) {
mergeGetCommand(other.getGetCommand());
}
if (other.hasGetCompactTypesCommand()) {
mergeGetCompactTypesCommand(other.getGetCompactTypesCommand());
}
if (other.hasGetEnumeratorCommand()) {
mergeGetEnumeratorCommand(other.getGetEnumeratorCommand());
}
if (other.hasGetGroupCommand()) {
mergeGetGroupCommand(other.getGetGroupCommand());
}
if (other.hasGetHashmapCommand()) {
mergeGetHashmapCommand(other.getGetHashmapCommand());
}
if (other.hasGetOptimalServerCommand()) {
mergeGetOptimalServerCommand(other.getGetOptimalServerCommand());
}
if (other.hasGetThresholdSizeCommand()) {
mergeGetThresholdSizeCommand(other.getGetThresholdSizeCommand());
}
if (other.hasGetTypeInfoMapCommand()) {
mergeGetTypeInfoMapCommand(other.getGetTypeInfoMapCommand());
}
if (other.hasInitCommand()) {
mergeInitCommand(other.getInitCommand());
}
if (other.hasInsertCommand()) {
mergeInsertCommand(other.getInsertCommand());
}
if (other.hasRaiseCustomEventCommand()) {
mergeRaiseCustomEventCommand(other.getRaiseCustomEventCommand());
}
if (other.hasRegisterKeyNotifCommand()) {
mergeRegisterKeyNotifCommand(other.getRegisterKeyNotifCommand());
}
if (other.hasRegisterNotifCommand()) {
mergeRegisterNotifCommand(other.getRegisterNotifCommand());
}
if (other.hasRemoveCommand()) {
mergeRemoveCommand(other.getRemoveCommand());
}
if (other.hasRemoveGroupCommand()) {
mergeRemoveGroupCommand(other.getRemoveGroupCommand());
}
if (other.hasSearchCommand()) {
mergeSearchCommand(other.getSearchCommand());
}
if (other.hasGetTagCommand()) {
mergeGetTagCommand(other.getGetTagCommand());
}
if (other.hasLockCommand()) {
mergeLockCommand(other.getLockCommand());
}
if (other.hasUnlockCommand()) {
mergeUnlockCommand(other.getUnlockCommand());
}
if (other.hasIsLockedCommand()) {
mergeIsLockedCommand(other.getIsLockedCommand());
}
if (other.hasLockVerifyCommand()) {
mergeLockVerifyCommand(other.getLockVerifyCommand());
}
if (other.hasUnRegisterKeyNotifCommand()) {
mergeUnRegisterKeyNotifCommand(other.getUnRegisterKeyNotifCommand());
}
if (other.hasUnRegisterBulkKeyNotifCommand()) {
mergeUnRegisterBulkKeyNotifCommand(other.getUnRegisterBulkKeyNotifCommand());
}
if (other.hasRegisterBulkKeyNotifCommand()) {
mergeRegisterBulkKeyNotifCommand(other.getRegisterBulkKeyNotifCommand());
}
if (other.hasHybridBulkCommand()) {
mergeHybridBulkCommand(other.getHybridBulkCommand());
}
if (other.hasGetLoggingInfoCommand()) {
mergeGetLoggingInfoCommand(other.getGetLoggingInfoCommand());
}
if (other.hasCloseStreamCommand()) {
mergeCloseStreamCommand(other.getCloseStreamCommand());
}
if (other.hasGetStreamLengthCommand()) {
mergeGetStreamLengthCommand(other.getGetStreamLengthCommand());
}
if (other.hasOpenStreamCommand()) {
mergeOpenStreamCommand(other.getOpenStreamCommand());
}
if (other.hasWriteToStreamCommand()) {
mergeWriteToStreamCommand(other.getWriteToStreamCommand());
}
if (other.hasReadFromStreamCommand()) {
mergeReadFromStreamCommand(other.getReadFromStreamCommand());
}
if (other.hasBridgeGetStateTransferInfoCommand()) {
mergeBridgeGetStateTransferInfoCommand(other.getBridgeGetStateTransferInfoCommand());
}
if (other.hasBridgeGetTransferableKeyListCommand()) {
mergeBridgeGetTransferableKeyListCommand(other.getBridgeGetTransferableKeyListCommand());
}
if (other.hasBridgeHybridBulkCommand()) {
mergeBridgeHybridBulkCommand(other.getBridgeHybridBulkCommand());
}
if (other.hasBridgeInitCommand()) {
mergeBridgeInitCommand(other.getBridgeInitCommand());
}
if (other.hasBridgeSetTransferableKeyListCommand()) {
mergeBridgeSetTransferableKeyListCommand(other.getBridgeSetTransferableKeyListCommand());
}
if (other.hasBridgeSignlEndOfStateTransferCommand()) {
mergeBridgeSignlEndOfStateTransferCommand(other.getBridgeSignlEndOfStateTransferCommand());
}
if (other.hasRequestID()) {
setRequestID(other.getRequestID());
}
if (other.hasCommandVersion()) {
setCommandVersion(other.getCommandVersion());
}
if (other.hasBridgeSyncTimeCommand()) {
mergeBridgeSyncTimeCommand(other.getBridgeSyncTimeCommand());
}
if (other.hasBridgeHeartBeatReceivedCommand()) {
mergeBridgeHeartBeatReceivedCommand(other.getBridgeHeartBeatReceivedCommand());
}
if (other.hasRemoveByTagCommand()) {
mergeRemoveByTagCommand(other.getRemoveByTagCommand());
}
if (other.hasBridgeMakeTargetCacheActivePassiveCommand()) {
mergeBridgeMakeTargetCacheActivePassiveCommand(other.getBridgeMakeTargetCacheActivePassiveCommand());
}
if (other.hasUnRegisterCQCommand()) {
mergeUnRegisterCQCommand(other.getUnRegisterCQCommand());
}
if (other.hasSearchCQCommand()) {
mergeSearchCQCommand(other.getSearchCQCommand());
}
if (other.hasRegisterCQCommand()) {
mergeRegisterCQCommand(other.getRegisterCQCommand());
}
if (other.hasGetKeysByTagCommand()) {
mergeGetKeysByTagCommand(other.getGetKeysByTagCommand());
}
if (other.hasClientLastViewId()) {
setClientLastViewId(other.getClientLastViewId());
}
if (other.hasBulkDeleteCommand()) {
mergeBulkDeleteCommand(other.getBulkDeleteCommand());
}
if (other.hasDeleteCommand()) {
mergeDeleteCommand(other.getDeleteCommand());
}
if (other.hasIntendedRecipient()) {
setIntendedRecipient(other.getIntendedRecipient());
}
if (other.hasGetNextChunkCommand()) {
mergeGetNextChunkCommand(other.getGetNextChunkCommand());
}
if (other.hasGetGroupNextChunkCommand()) {
mergeGetGroupNextChunkCommand(other.getGetGroupNextChunkCommand());
}
if (other.hasAddAttributeCommand()) {
mergeAddAttributeCommand(other.getAddAttributeCommand());
}
if (other.hasGetEncryptionCommand()) {
mergeGetEncryptionCommand(other.getGetEncryptionCommand());
}
if (other.hasGetRunningServersCommand()) {
mergeGetRunningServersCommand(other.getGetRunningServersCommand());
}
if (other.hasSyncEventsCommand()) {
mergeSyncEventsCommand(other.getSyncEventsCommand());
}
if (other.hasDeleteQueryCommand()) {
mergeDeleteQueryCommand(other.getDeleteQueryCommand());
}
if (other.hasGetProductVersionCommand()) {
mergeGetProductVersionCommand(other.getGetProductVersionCommand());
}
if (other.hasBridgeGetReplicatorStatusInfoCommand()) {
mergeBridgeGetReplicatorStatusInfoCommand(other.getBridgeGetReplicatorStatusInfoCommand());
}
if (other.hasBridgeRequestStateTransferCommand()) {
mergeBridgeRequestStateTransferCommand(other.getBridgeRequestStateTransferCommand());
}
if (other.hasGetServerMappingCommand()) {
mergeGetServerMappingCommand(other.getGetServerMappingCommand());
}
if (other.hasInquiryRequestCommand()) {
mergeInquiryRequestCommand(other.getInquiryRequestCommand());
}
if (other.hasIsRetryCommand()) {
setIsRetryCommand(other.getIsRetryCommand());
}
if (other.hasMapReduceTaskCommand()) {
mergeMapReduceTaskCommand(other.getMapReduceTaskCommand());
}
if (other.hasTaskCallbackCommand()) {
mergeTaskCallbackCommand(other.getTaskCallbackCommand());
}
if (other.hasRunningTasksCommand()) {
mergeRunningTasksCommand(other.getRunningTasksCommand());
}
if (other.hasTaskCancelCommand()) {
mergeTaskCancelCommand(other.getTaskCancelCommand());
}
if (other.hasTaskProgressCommand()) {
mergeTaskProgressCommand(other.getTaskProgressCommand());
}
if (other.hasNextRecordCommand()) {
mergeNextRecordCommand(other.getNextRecordCommand());
}
if (other.hasCommandID()) {
setCommandID(other.getCommandID());
}
if (other.hasGetCacheBindingCommand()) {
mergeGetCacheBindingCommand(other.getGetCacheBindingCommand());
}
if (other.hasTaskEnumeratorCommand()) {
mergeTaskEnumeratorCommand(other.getTaskEnumeratorCommand());
}
if (other.hasInvokeEntryProcessorCommand()) {
mergeInvokeEntryProcessorCommand(other.getInvokeEntryProcessorCommand());
}
if (other.hasExecuteReaderCommand()) {
mergeExecuteReaderCommand(other.getExecuteReaderCommand());
}
if (other.hasGetReaderNextChunkCommand()) {
mergeGetReaderNextChunkCommand(other.getGetReaderNextChunkCommand());
}
if (other.hasDisposeReaderCommand()) {
mergeDisposeReaderCommand(other.getDisposeReaderCommand());
}
if (other.hasExecuteReaderCQCommand()) {
mergeExecuteReaderCQCommand(other.getExecuteReaderCQCommand());
}
if (other.hasGetExpirationCommand()) {
mergeGetExpirationCommand(other.getGetExpirationCommand());
}
if (other.hasGetLCCommand()) {
mergeGetLCCommand(other.getGetLCCommand());
}
if (other.hasCacheSecurityAuthorizationCommand()) {
mergeCacheSecurityAuthorizationCommand(other.getCacheSecurityAuthorizationCommand());
}
if (other.hasPollCommand()) {
mergePollCommand(other.getPollCommand());
}
if (other.hasRegisterPollNotifCommand()) {
mergeRegisterPollNotifCommand(other.getRegisterPollNotifCommand());
}
if (other.hasGetConnectedClientsCommand()) {
mergeGetConnectedClientsCommand(other.getGetConnectedClientsCommand());
}
if (other.hasMethodOverload()) {
setMethodOverload(other.getMethodOverload());
}
if (other.hasTouchCommand()) {
mergeTouchCommand(other.getTouchCommand());
}
if (other.hasGetCacheManagementPortCommand()) {
mergeGetCacheManagementPortCommand(other.getGetCacheManagementPortCommand());
}
if (other.hasGetTopicCommand()) {
mergeGetTopicCommand(other.getGetTopicCommand());
}
if (other.hasSubscribeTopicCommand()) {
mergeSubscribeTopicCommand(other.getSubscribeTopicCommand());
}
if (other.hasRemoveTopicCommand()) {
mergeRemoveTopicCommand(other.getRemoveTopicCommand());
}
if (other.hasUnSubscribeTopicCommand()) {
mergeUnSubscribeTopicCommand(other.getUnSubscribeTopicCommand());
}
if (other.hasMessagePublishCommand()) {
mergeMessagePublishCommand(other.getMessagePublishCommand());
}
if (other.hasGetMessageCommand()) {
mergeGetMessageCommand(other.getGetMessageCommand());
}
if (other.hasMesasgeAcknowledgmentCommand()) {
mergeMesasgeAcknowledgmentCommand(other.getMesasgeAcknowledgmentCommand());
}
if (other.hasPingCommand()) {
mergePingCommand(other.getPingCommand());
}
if (other.hasMessageCountCommand()) {
mergeMessageCountCommand(other.getMessageCountCommand());
}
if (other.hasDataTypes()) {
mergeDataTypes(other.getDataTypes());
}
if (other.hasGetSerializationFormatCommand()) {
mergeGetSerializationFormatCommand(other.getGetSerializationFormatCommand());
}
if (other.hasBulkGetCacheItemCommand()) {
mergeBulkGetCacheItemCommand(other.getBulkGetCacheItemCommand());
}
if (other.hasContainsBulkCommand()) {
mergeContainsBulkCommand(other.getContainsBulkCommand());
}
if (other.hasModuleCommand()) {
mergeModuleCommand(other.getModuleCommand());
}
if (other.hasSurrogateCommand()) {
mergeSurrogateCommand(other.getSurrogateCommand());
}
if (other.hasMessagePublishBulkCommand()) {
mergeMessagePublishBulkCommand(other.getMessagePublishBulkCommand());
}
if (other.hasBridgePollForResumingReplication()) {
mergeBridgePollForResumingReplication(other.getBridgePollForResumingReplication());
}
if (other.hasGetModuleStateCommand()) {
mergeGetModuleStateCommand(other.getGetModuleStateCommand());
}
if (other.hasSetModuleStateCommand()) {
mergeSetModuleStateCommand(other.getSetModuleStateCommand());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type value = com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
setType(value);
}
break;
}
case 18: {
setVersion(input.readString());
break;
}
case 26: {
com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand.newBuilder();
if (hasAddCommand()) {
subBuilder.mergeFrom(getAddCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setAddCommand(subBuilder.buildPartial());
break;
}
case 34: {
com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand.newBuilder();
if (hasAddDependencyCommand()) {
subBuilder.mergeFrom(getAddDependencyCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setAddDependencyCommand(subBuilder.buildPartial());
break;
}
case 42: {
com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand.newBuilder();
if (hasAddSyncDependencyCommand()) {
subBuilder.mergeFrom(getAddSyncDependencyCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setAddSyncDependencyCommand(subBuilder.buildPartial());
break;
}
case 50: {
com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand.newBuilder();
if (hasBulkAddCommand()) {
subBuilder.mergeFrom(getBulkAddCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBulkAddCommand(subBuilder.buildPartial());
break;
}
case 58: {
com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand.newBuilder();
if (hasBulkGetCommand()) {
subBuilder.mergeFrom(getBulkGetCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBulkGetCommand(subBuilder.buildPartial());
break;
}
case 66: {
com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand.newBuilder();
if (hasBulkInsertCommand()) {
subBuilder.mergeFrom(getBulkInsertCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBulkInsertCommand(subBuilder.buildPartial());
break;
}
case 74: {
com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand.newBuilder();
if (hasBulkRemoveCommand()) {
subBuilder.mergeFrom(getBulkRemoveCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBulkRemoveCommand(subBuilder.buildPartial());
break;
}
case 82: {
com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand.newBuilder();
if (hasClearCommand()) {
subBuilder.mergeFrom(getClearCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setClearCommand(subBuilder.buildPartial());
break;
}
case 90: {
com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand.newBuilder();
if (hasContainsCommand()) {
subBuilder.mergeFrom(getContainsCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setContainsCommand(subBuilder.buildPartial());
break;
}
case 98: {
com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand.newBuilder();
if (hasCountCommand()) {
subBuilder.mergeFrom(getCountCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setCountCommand(subBuilder.buildPartial());
break;
}
case 106: {
com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand.newBuilder();
if (hasDisposeCommand()) {
subBuilder.mergeFrom(getDisposeCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setDisposeCommand(subBuilder.buildPartial());
break;
}
case 114: {
com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand.newBuilder();
if (hasGetCacheItemCommand()) {
subBuilder.mergeFrom(getGetCacheItemCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetCacheItemCommand(subBuilder.buildPartial());
break;
}
case 122: {
com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand.newBuilder();
if (hasGetCommand()) {
subBuilder.mergeFrom(getGetCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetCommand(subBuilder.buildPartial());
break;
}
case 130: {
com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand.newBuilder();
if (hasGetCompactTypesCommand()) {
subBuilder.mergeFrom(getGetCompactTypesCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetCompactTypesCommand(subBuilder.buildPartial());
break;
}
case 138: {
com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand.newBuilder();
if (hasGetEnumeratorCommand()) {
subBuilder.mergeFrom(getGetEnumeratorCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetEnumeratorCommand(subBuilder.buildPartial());
break;
}
case 146: {
com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand.newBuilder();
if (hasGetGroupCommand()) {
subBuilder.mergeFrom(getGetGroupCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetGroupCommand(subBuilder.buildPartial());
break;
}
case 154: {
com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand.newBuilder();
if (hasGetHashmapCommand()) {
subBuilder.mergeFrom(getGetHashmapCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetHashmapCommand(subBuilder.buildPartial());
break;
}
case 162: {
com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand.newBuilder();
if (hasGetOptimalServerCommand()) {
subBuilder.mergeFrom(getGetOptimalServerCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetOptimalServerCommand(subBuilder.buildPartial());
break;
}
case 170: {
com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand.newBuilder();
if (hasGetThresholdSizeCommand()) {
subBuilder.mergeFrom(getGetThresholdSizeCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetThresholdSizeCommand(subBuilder.buildPartial());
break;
}
case 178: {
com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand.newBuilder();
if (hasGetTypeInfoMapCommand()) {
subBuilder.mergeFrom(getGetTypeInfoMapCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetTypeInfoMapCommand(subBuilder.buildPartial());
break;
}
case 186: {
com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand.newBuilder();
if (hasInitCommand()) {
subBuilder.mergeFrom(getInitCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setInitCommand(subBuilder.buildPartial());
break;
}
case 194: {
com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand.newBuilder();
if (hasInsertCommand()) {
subBuilder.mergeFrom(getInsertCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setInsertCommand(subBuilder.buildPartial());
break;
}
case 202: {
com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand.newBuilder();
if (hasRaiseCustomEventCommand()) {
subBuilder.mergeFrom(getRaiseCustomEventCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRaiseCustomEventCommand(subBuilder.buildPartial());
break;
}
case 210: {
com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand.newBuilder();
if (hasRegisterKeyNotifCommand()) {
subBuilder.mergeFrom(getRegisterKeyNotifCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRegisterKeyNotifCommand(subBuilder.buildPartial());
break;
}
case 218: {
com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand.newBuilder();
if (hasRegisterNotifCommand()) {
subBuilder.mergeFrom(getRegisterNotifCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRegisterNotifCommand(subBuilder.buildPartial());
break;
}
case 226: {
com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand.newBuilder();
if (hasRemoveCommand()) {
subBuilder.mergeFrom(getRemoveCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRemoveCommand(subBuilder.buildPartial());
break;
}
case 234: {
com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand.newBuilder();
if (hasRemoveGroupCommand()) {
subBuilder.mergeFrom(getRemoveGroupCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRemoveGroupCommand(subBuilder.buildPartial());
break;
}
case 242: {
com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand.newBuilder();
if (hasSearchCommand()) {
subBuilder.mergeFrom(getSearchCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setSearchCommand(subBuilder.buildPartial());
break;
}
case 250: {
com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand.newBuilder();
if (hasGetTagCommand()) {
subBuilder.mergeFrom(getGetTagCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetTagCommand(subBuilder.buildPartial());
break;
}
case 258: {
com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand.newBuilder();
if (hasLockCommand()) {
subBuilder.mergeFrom(getLockCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setLockCommand(subBuilder.buildPartial());
break;
}
case 266: {
com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand.newBuilder();
if (hasUnlockCommand()) {
subBuilder.mergeFrom(getUnlockCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setUnlockCommand(subBuilder.buildPartial());
break;
}
case 274: {
com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand.newBuilder();
if (hasIsLockedCommand()) {
subBuilder.mergeFrom(getIsLockedCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setIsLockedCommand(subBuilder.buildPartial());
break;
}
case 282: {
com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand.newBuilder();
if (hasLockVerifyCommand()) {
subBuilder.mergeFrom(getLockVerifyCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setLockVerifyCommand(subBuilder.buildPartial());
break;
}
case 290: {
com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand.newBuilder();
if (hasUnRegisterKeyNotifCommand()) {
subBuilder.mergeFrom(getUnRegisterKeyNotifCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setUnRegisterKeyNotifCommand(subBuilder.buildPartial());
break;
}
case 298: {
com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand.newBuilder();
if (hasUnRegisterBulkKeyNotifCommand()) {
subBuilder.mergeFrom(getUnRegisterBulkKeyNotifCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setUnRegisterBulkKeyNotifCommand(subBuilder.buildPartial());
break;
}
case 306: {
com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand.newBuilder();
if (hasRegisterBulkKeyNotifCommand()) {
subBuilder.mergeFrom(getRegisterBulkKeyNotifCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRegisterBulkKeyNotifCommand(subBuilder.buildPartial());
break;
}
case 314: {
com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand.newBuilder();
if (hasHybridBulkCommand()) {
subBuilder.mergeFrom(getHybridBulkCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setHybridBulkCommand(subBuilder.buildPartial());
break;
}
case 322: {
com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand.newBuilder();
if (hasGetLoggingInfoCommand()) {
subBuilder.mergeFrom(getGetLoggingInfoCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetLoggingInfoCommand(subBuilder.buildPartial());
break;
}
case 330: {
com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand.newBuilder();
if (hasCloseStreamCommand()) {
subBuilder.mergeFrom(getCloseStreamCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setCloseStreamCommand(subBuilder.buildPartial());
break;
}
case 338: {
com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand.newBuilder();
if (hasGetStreamLengthCommand()) {
subBuilder.mergeFrom(getGetStreamLengthCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetStreamLengthCommand(subBuilder.buildPartial());
break;
}
case 346: {
com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand.newBuilder();
if (hasOpenStreamCommand()) {
subBuilder.mergeFrom(getOpenStreamCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setOpenStreamCommand(subBuilder.buildPartial());
break;
}
case 354: {
com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand.newBuilder();
if (hasWriteToStreamCommand()) {
subBuilder.mergeFrom(getWriteToStreamCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setWriteToStreamCommand(subBuilder.buildPartial());
break;
}
case 362: {
com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand.newBuilder();
if (hasReadFromStreamCommand()) {
subBuilder.mergeFrom(getReadFromStreamCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setReadFromStreamCommand(subBuilder.buildPartial());
break;
}
case 370: {
com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand.newBuilder();
if (hasBridgeGetStateTransferInfoCommand()) {
subBuilder.mergeFrom(getBridgeGetStateTransferInfoCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeGetStateTransferInfoCommand(subBuilder.buildPartial());
break;
}
case 378: {
com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand.newBuilder();
if (hasBridgeGetTransferableKeyListCommand()) {
subBuilder.mergeFrom(getBridgeGetTransferableKeyListCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeGetTransferableKeyListCommand(subBuilder.buildPartial());
break;
}
case 386: {
com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand.newBuilder();
if (hasBridgeHybridBulkCommand()) {
subBuilder.mergeFrom(getBridgeHybridBulkCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeHybridBulkCommand(subBuilder.buildPartial());
break;
}
case 394: {
com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand.newBuilder();
if (hasBridgeInitCommand()) {
subBuilder.mergeFrom(getBridgeInitCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeInitCommand(subBuilder.buildPartial());
break;
}
case 402: {
com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand.newBuilder();
if (hasBridgeSetTransferableKeyListCommand()) {
subBuilder.mergeFrom(getBridgeSetTransferableKeyListCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeSetTransferableKeyListCommand(subBuilder.buildPartial());
break;
}
case 410: {
com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand.newBuilder();
if (hasBridgeSignlEndOfStateTransferCommand()) {
subBuilder.mergeFrom(getBridgeSignlEndOfStateTransferCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeSignlEndOfStateTransferCommand(subBuilder.buildPartial());
break;
}
case 416: {
setRequestID(input.readInt64());
break;
}
case 424: {
setCommandVersion(input.readInt32());
break;
}
case 434: {
com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand.newBuilder();
if (hasBridgeSyncTimeCommand()) {
subBuilder.mergeFrom(getBridgeSyncTimeCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeSyncTimeCommand(subBuilder.buildPartial());
break;
}
case 442: {
com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand.newBuilder();
if (hasBridgeHeartBeatReceivedCommand()) {
subBuilder.mergeFrom(getBridgeHeartBeatReceivedCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeHeartBeatReceivedCommand(subBuilder.buildPartial());
break;
}
case 450: {
com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand.newBuilder();
if (hasRemoveByTagCommand()) {
subBuilder.mergeFrom(getRemoveByTagCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRemoveByTagCommand(subBuilder.buildPartial());
break;
}
case 458: {
com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand.newBuilder();
if (hasBridgeMakeTargetCacheActivePassiveCommand()) {
subBuilder.mergeFrom(getBridgeMakeTargetCacheActivePassiveCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeMakeTargetCacheActivePassiveCommand(subBuilder.buildPartial());
break;
}
case 466: {
com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand.newBuilder();
if (hasUnRegisterCQCommand()) {
subBuilder.mergeFrom(getUnRegisterCQCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setUnRegisterCQCommand(subBuilder.buildPartial());
break;
}
case 474: {
com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand.newBuilder();
if (hasSearchCQCommand()) {
subBuilder.mergeFrom(getSearchCQCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setSearchCQCommand(subBuilder.buildPartial());
break;
}
case 482: {
com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand.newBuilder();
if (hasRegisterCQCommand()) {
subBuilder.mergeFrom(getRegisterCQCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRegisterCQCommand(subBuilder.buildPartial());
break;
}
case 490: {
com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand.newBuilder();
if (hasGetKeysByTagCommand()) {
subBuilder.mergeFrom(getGetKeysByTagCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetKeysByTagCommand(subBuilder.buildPartial());
break;
}
case 496: {
setClientLastViewId(input.readInt64());
break;
}
case 506: {
com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand.newBuilder();
if (hasBulkDeleteCommand()) {
subBuilder.mergeFrom(getBulkDeleteCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBulkDeleteCommand(subBuilder.buildPartial());
break;
}
case 514: {
com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand.newBuilder();
if (hasDeleteCommand()) {
subBuilder.mergeFrom(getDeleteCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setDeleteCommand(subBuilder.buildPartial());
break;
}
case 522: {
setIntendedRecipient(input.readString());
break;
}
case 530: {
com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand.newBuilder();
if (hasGetNextChunkCommand()) {
subBuilder.mergeFrom(getGetNextChunkCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetNextChunkCommand(subBuilder.buildPartial());
break;
}
case 538: {
com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand.newBuilder();
if (hasGetGroupNextChunkCommand()) {
subBuilder.mergeFrom(getGetGroupNextChunkCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetGroupNextChunkCommand(subBuilder.buildPartial());
break;
}
case 546: {
com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand.newBuilder();
if (hasAddAttributeCommand()) {
subBuilder.mergeFrom(getAddAttributeCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setAddAttributeCommand(subBuilder.buildPartial());
break;
}
case 554: {
com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand.newBuilder();
if (hasGetEncryptionCommand()) {
subBuilder.mergeFrom(getGetEncryptionCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetEncryptionCommand(subBuilder.buildPartial());
break;
}
case 562: {
com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand.newBuilder();
if (hasGetRunningServersCommand()) {
subBuilder.mergeFrom(getGetRunningServersCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetRunningServersCommand(subBuilder.buildPartial());
break;
}
case 570: {
com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand.newBuilder();
if (hasSyncEventsCommand()) {
subBuilder.mergeFrom(getSyncEventsCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setSyncEventsCommand(subBuilder.buildPartial());
break;
}
case 578: {
com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand.newBuilder();
if (hasDeleteQueryCommand()) {
subBuilder.mergeFrom(getDeleteQueryCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setDeleteQueryCommand(subBuilder.buildPartial());
break;
}
case 586: {
com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand.newBuilder();
if (hasGetProductVersionCommand()) {
subBuilder.mergeFrom(getGetProductVersionCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetProductVersionCommand(subBuilder.buildPartial());
break;
}
case 594: {
com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand.newBuilder();
if (hasBridgeGetReplicatorStatusInfoCommand()) {
subBuilder.mergeFrom(getBridgeGetReplicatorStatusInfoCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeGetReplicatorStatusInfoCommand(subBuilder.buildPartial());
break;
}
case 602: {
com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand.newBuilder();
if (hasBridgeRequestStateTransferCommand()) {
subBuilder.mergeFrom(getBridgeRequestStateTransferCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgeRequestStateTransferCommand(subBuilder.buildPartial());
break;
}
case 610: {
com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand.newBuilder();
if (hasGetServerMappingCommand()) {
subBuilder.mergeFrom(getGetServerMappingCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetServerMappingCommand(subBuilder.buildPartial());
break;
}
case 618: {
com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand.newBuilder();
if (hasInquiryRequestCommand()) {
subBuilder.mergeFrom(getInquiryRequestCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setInquiryRequestCommand(subBuilder.buildPartial());
break;
}
case 624: {
setIsRetryCommand(input.readBool());
break;
}
case 634: {
com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand.newBuilder();
if (hasMapReduceTaskCommand()) {
subBuilder.mergeFrom(getMapReduceTaskCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setMapReduceTaskCommand(subBuilder.buildPartial());
break;
}
case 642: {
com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand.newBuilder();
if (hasTaskCallbackCommand()) {
subBuilder.mergeFrom(getTaskCallbackCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setTaskCallbackCommand(subBuilder.buildPartial());
break;
}
case 650: {
com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand.newBuilder();
if (hasRunningTasksCommand()) {
subBuilder.mergeFrom(getRunningTasksCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRunningTasksCommand(subBuilder.buildPartial());
break;
}
case 658: {
com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand.newBuilder();
if (hasTaskCancelCommand()) {
subBuilder.mergeFrom(getTaskCancelCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setTaskCancelCommand(subBuilder.buildPartial());
break;
}
case 666: {
com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand.newBuilder();
if (hasTaskProgressCommand()) {
subBuilder.mergeFrom(getTaskProgressCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setTaskProgressCommand(subBuilder.buildPartial());
break;
}
case 674: {
com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand.newBuilder();
if (hasNextRecordCommand()) {
subBuilder.mergeFrom(getNextRecordCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setNextRecordCommand(subBuilder.buildPartial());
break;
}
case 680: {
setCommandID(input.readInt32());
break;
}
case 690: {
com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand.newBuilder();
if (hasGetCacheBindingCommand()) {
subBuilder.mergeFrom(getGetCacheBindingCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetCacheBindingCommand(subBuilder.buildPartial());
break;
}
case 698: {
com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand.newBuilder();
if (hasTaskEnumeratorCommand()) {
subBuilder.mergeFrom(getTaskEnumeratorCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setTaskEnumeratorCommand(subBuilder.buildPartial());
break;
}
case 706: {
com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand.newBuilder();
if (hasInvokeEntryProcessorCommand()) {
subBuilder.mergeFrom(getInvokeEntryProcessorCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setInvokeEntryProcessorCommand(subBuilder.buildPartial());
break;
}
case 714: {
com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand.newBuilder();
if (hasExecuteReaderCommand()) {
subBuilder.mergeFrom(getExecuteReaderCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setExecuteReaderCommand(subBuilder.buildPartial());
break;
}
case 722: {
com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand.newBuilder();
if (hasGetReaderNextChunkCommand()) {
subBuilder.mergeFrom(getGetReaderNextChunkCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetReaderNextChunkCommand(subBuilder.buildPartial());
break;
}
case 730: {
com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand.newBuilder();
if (hasDisposeReaderCommand()) {
subBuilder.mergeFrom(getDisposeReaderCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setDisposeReaderCommand(subBuilder.buildPartial());
break;
}
case 738: {
com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand.newBuilder();
if (hasExecuteReaderCQCommand()) {
subBuilder.mergeFrom(getExecuteReaderCQCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setExecuteReaderCQCommand(subBuilder.buildPartial());
break;
}
case 746: {
com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand.newBuilder();
if (hasGetExpirationCommand()) {
subBuilder.mergeFrom(getGetExpirationCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetExpirationCommand(subBuilder.buildPartial());
break;
}
case 754: {
com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand.newBuilder();
if (hasGetLCCommand()) {
subBuilder.mergeFrom(getGetLCCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetLCCommand(subBuilder.buildPartial());
break;
}
case 762: {
com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand.newBuilder();
if (hasCacheSecurityAuthorizationCommand()) {
subBuilder.mergeFrom(getCacheSecurityAuthorizationCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setCacheSecurityAuthorizationCommand(subBuilder.buildPartial());
break;
}
case 770: {
com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand.newBuilder();
if (hasPollCommand()) {
subBuilder.mergeFrom(getPollCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setPollCommand(subBuilder.buildPartial());
break;
}
case 778: {
com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand.newBuilder();
if (hasRegisterPollNotifCommand()) {
subBuilder.mergeFrom(getRegisterPollNotifCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRegisterPollNotifCommand(subBuilder.buildPartial());
break;
}
case 786: {
com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand.newBuilder();
if (hasGetConnectedClientsCommand()) {
subBuilder.mergeFrom(getGetConnectedClientsCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetConnectedClientsCommand(subBuilder.buildPartial());
break;
}
case 792: {
setMethodOverload(input.readInt32());
break;
}
case 802: {
com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand.newBuilder();
if (hasTouchCommand()) {
subBuilder.mergeFrom(getTouchCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setTouchCommand(subBuilder.buildPartial());
break;
}
case 810: {
com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand.newBuilder();
if (hasGetCacheManagementPortCommand()) {
subBuilder.mergeFrom(getGetCacheManagementPortCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetCacheManagementPortCommand(subBuilder.buildPartial());
break;
}
case 818: {
com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand.newBuilder();
if (hasGetTopicCommand()) {
subBuilder.mergeFrom(getGetTopicCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetTopicCommand(subBuilder.buildPartial());
break;
}
case 826: {
com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand.newBuilder();
if (hasSubscribeTopicCommand()) {
subBuilder.mergeFrom(getSubscribeTopicCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setSubscribeTopicCommand(subBuilder.buildPartial());
break;
}
case 834: {
com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand.newBuilder();
if (hasRemoveTopicCommand()) {
subBuilder.mergeFrom(getRemoveTopicCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setRemoveTopicCommand(subBuilder.buildPartial());
break;
}
case 842: {
com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand.newBuilder();
if (hasUnSubscribeTopicCommand()) {
subBuilder.mergeFrom(getUnSubscribeTopicCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setUnSubscribeTopicCommand(subBuilder.buildPartial());
break;
}
case 850: {
com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand.newBuilder();
if (hasMessagePublishCommand()) {
subBuilder.mergeFrom(getMessagePublishCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setMessagePublishCommand(subBuilder.buildPartial());
break;
}
case 858: {
com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand.newBuilder();
if (hasGetMessageCommand()) {
subBuilder.mergeFrom(getGetMessageCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetMessageCommand(subBuilder.buildPartial());
break;
}
case 866: {
com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand.newBuilder();
if (hasMesasgeAcknowledgmentCommand()) {
subBuilder.mergeFrom(getMesasgeAcknowledgmentCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setMesasgeAcknowledgmentCommand(subBuilder.buildPartial());
break;
}
case 874: {
com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand.newBuilder();
if (hasPingCommand()) {
subBuilder.mergeFrom(getPingCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setPingCommand(subBuilder.buildPartial());
break;
}
case 882: {
com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand.newBuilder();
if (hasMessageCountCommand()) {
subBuilder.mergeFrom(getMessageCountCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setMessageCountCommand(subBuilder.buildPartial());
break;
}
case 890: {
com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes.newBuilder();
if (hasDataTypes()) {
subBuilder.mergeFrom(getDataTypes());
}
input.readMessage(subBuilder, extensionRegistry);
setDataTypes(subBuilder.buildPartial());
break;
}
case 898: {
com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand.newBuilder();
if (hasGetSerializationFormatCommand()) {
subBuilder.mergeFrom(getGetSerializationFormatCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetSerializationFormatCommand(subBuilder.buildPartial());
break;
}
case 906: {
com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand.newBuilder();
if (hasBulkGetCacheItemCommand()) {
subBuilder.mergeFrom(getBulkGetCacheItemCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setBulkGetCacheItemCommand(subBuilder.buildPartial());
break;
}
case 914: {
com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand.newBuilder();
if (hasContainsBulkCommand()) {
subBuilder.mergeFrom(getContainsBulkCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setContainsBulkCommand(subBuilder.buildPartial());
break;
}
case 922: {
com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand.newBuilder();
if (hasModuleCommand()) {
subBuilder.mergeFrom(getModuleCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setModuleCommand(subBuilder.buildPartial());
break;
}
case 930: {
com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand.newBuilder();
if (hasSurrogateCommand()) {
subBuilder.mergeFrom(getSurrogateCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setSurrogateCommand(subBuilder.buildPartial());
break;
}
case 938: {
com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.newBuilder();
if (hasMessagePublishBulkCommand()) {
subBuilder.mergeFrom(getMessagePublishBulkCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setMessagePublishBulkCommand(subBuilder.buildPartial());
break;
}
case 946: {
com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication.Builder subBuilder = com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication.newBuilder();
if (hasBridgePollForResumingReplication()) {
subBuilder.mergeFrom(getBridgePollForResumingReplication());
}
input.readMessage(subBuilder, extensionRegistry);
setBridgePollForResumingReplication(subBuilder.buildPartial());
break;
}
case 954: {
com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand.newBuilder();
if (hasGetModuleStateCommand()) {
subBuilder.mergeFrom(getGetModuleStateCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setGetModuleStateCommand(subBuilder.buildPartial());
break;
}
case 962: {
com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand.Builder subBuilder = com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand.newBuilder();
if (hasSetModuleStateCommand()) {
subBuilder.mergeFrom(getSetModuleStateCommand());
}
input.readMessage(subBuilder, extensionRegistry);
setSetModuleStateCommand(subBuilder.buildPartial());
break;
}
}
}
}
// optional .com.alachisoft.ncache.common.protobuf.Command.Type type = 1;
public boolean hasType() {
return result.hasType();
}
public com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type getType() {
return result.getType();
}
public Builder setType(com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type value) {
if (value == null) {
throw new NullPointerException();
}
result.hasType = true;
result.type_ = value;
return this;
}
public Builder clearType() {
result.hasType = false;
result.type_ = com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Type.ADD;
return this;
}
// optional string version = 2;
public boolean hasVersion() {
return result.hasVersion();
}
public java.lang.String getVersion() {
return result.getVersion();
}
public Builder setVersion(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasVersion = true;
result.version_ = value;
return this;
}
public Builder clearVersion() {
result.hasVersion = false;
result.version_ = getDefaultInstance().getVersion();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.AddCommand addCommand = 3;
public boolean hasAddCommand() {
return result.hasAddCommand();
}
public com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand getAddCommand() {
return result.getAddCommand();
}
public Builder setAddCommand(com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasAddCommand = true;
result.addCommand_ = value;
return this;
}
public Builder setAddCommand(com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand.Builder builderForValue) {
result.hasAddCommand = true;
result.addCommand_ = builderForValue.build();
return this;
}
public Builder mergeAddCommand(com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand value) {
if (result.hasAddCommand() &&
result.addCommand_ != com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand.getDefaultInstance()) {
result.addCommand_ =
com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand.newBuilder(result.addCommand_).mergeFrom(value).buildPartial();
} else {
result.addCommand_ = value;
}
result.hasAddCommand = true;
return this;
}
public Builder clearAddCommand() {
result.hasAddCommand = false;
result.addCommand_ = com.alachisoft.ncache.common.protobuf.AddCommandProtocol.AddCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.AddDependencyCommand addDependencyCommand = 4;
public boolean hasAddDependencyCommand() {
return result.hasAddDependencyCommand();
}
public com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand getAddDependencyCommand() {
return result.getAddDependencyCommand();
}
public Builder setAddDependencyCommand(com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasAddDependencyCommand = true;
result.addDependencyCommand_ = value;
return this;
}
public Builder setAddDependencyCommand(com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand.Builder builderForValue) {
result.hasAddDependencyCommand = true;
result.addDependencyCommand_ = builderForValue.build();
return this;
}
public Builder mergeAddDependencyCommand(com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand value) {
if (result.hasAddDependencyCommand() &&
result.addDependencyCommand_ != com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand.getDefaultInstance()) {
result.addDependencyCommand_ =
com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand.newBuilder(result.addDependencyCommand_).mergeFrom(value).buildPartial();
} else {
result.addDependencyCommand_ = value;
}
result.hasAddDependencyCommand = true;
return this;
}
public Builder clearAddDependencyCommand() {
result.hasAddDependencyCommand = false;
result.addDependencyCommand_ = com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.AddDependencyCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommand addSyncDependencyCommand = 5;
public boolean hasAddSyncDependencyCommand() {
return result.hasAddSyncDependencyCommand();
}
public com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand getAddSyncDependencyCommand() {
return result.getAddSyncDependencyCommand();
}
public Builder setAddSyncDependencyCommand(com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasAddSyncDependencyCommand = true;
result.addSyncDependencyCommand_ = value;
return this;
}
public Builder setAddSyncDependencyCommand(com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand.Builder builderForValue) {
result.hasAddSyncDependencyCommand = true;
result.addSyncDependencyCommand_ = builderForValue.build();
return this;
}
public Builder mergeAddSyncDependencyCommand(com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand value) {
if (result.hasAddSyncDependencyCommand() &&
result.addSyncDependencyCommand_ != com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand.getDefaultInstance()) {
result.addSyncDependencyCommand_ =
com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand.newBuilder(result.addSyncDependencyCommand_).mergeFrom(value).buildPartial();
} else {
result.addSyncDependencyCommand_ = value;
}
result.hasAddSyncDependencyCommand = true;
return this;
}
public Builder clearAddSyncDependencyCommand() {
result.hasAddSyncDependencyCommand = false;
result.addSyncDependencyCommand_ = com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.AddSyncDependencyCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BulkAddCommand bulkAddCommand = 6;
public boolean hasBulkAddCommand() {
return result.hasBulkAddCommand();
}
public com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand getBulkAddCommand() {
return result.getBulkAddCommand();
}
public Builder setBulkAddCommand(com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBulkAddCommand = true;
result.bulkAddCommand_ = value;
return this;
}
public Builder setBulkAddCommand(com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand.Builder builderForValue) {
result.hasBulkAddCommand = true;
result.bulkAddCommand_ = builderForValue.build();
return this;
}
public Builder mergeBulkAddCommand(com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand value) {
if (result.hasBulkAddCommand() &&
result.bulkAddCommand_ != com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand.getDefaultInstance()) {
result.bulkAddCommand_ =
com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand.newBuilder(result.bulkAddCommand_).mergeFrom(value).buildPartial();
} else {
result.bulkAddCommand_ = value;
}
result.hasBulkAddCommand = true;
return this;
}
public Builder clearBulkAddCommand() {
result.hasBulkAddCommand = false;
result.bulkAddCommand_ = com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.BulkAddCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BulkGetCommand bulkGetCommand = 7;
public boolean hasBulkGetCommand() {
return result.hasBulkGetCommand();
}
public com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand getBulkGetCommand() {
return result.getBulkGetCommand();
}
public Builder setBulkGetCommand(com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBulkGetCommand = true;
result.bulkGetCommand_ = value;
return this;
}
public Builder setBulkGetCommand(com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand.Builder builderForValue) {
result.hasBulkGetCommand = true;
result.bulkGetCommand_ = builderForValue.build();
return this;
}
public Builder mergeBulkGetCommand(com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand value) {
if (result.hasBulkGetCommand() &&
result.bulkGetCommand_ != com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand.getDefaultInstance()) {
result.bulkGetCommand_ =
com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand.newBuilder(result.bulkGetCommand_).mergeFrom(value).buildPartial();
} else {
result.bulkGetCommand_ = value;
}
result.hasBulkGetCommand = true;
return this;
}
public Builder clearBulkGetCommand() {
result.hasBulkGetCommand = false;
result.bulkGetCommand_ = com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.BulkGetCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BulkInsertCommand bulkInsertCommand = 8;
public boolean hasBulkInsertCommand() {
return result.hasBulkInsertCommand();
}
public com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand getBulkInsertCommand() {
return result.getBulkInsertCommand();
}
public Builder setBulkInsertCommand(com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBulkInsertCommand = true;
result.bulkInsertCommand_ = value;
return this;
}
public Builder setBulkInsertCommand(com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand.Builder builderForValue) {
result.hasBulkInsertCommand = true;
result.bulkInsertCommand_ = builderForValue.build();
return this;
}
public Builder mergeBulkInsertCommand(com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand value) {
if (result.hasBulkInsertCommand() &&
result.bulkInsertCommand_ != com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand.getDefaultInstance()) {
result.bulkInsertCommand_ =
com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand.newBuilder(result.bulkInsertCommand_).mergeFrom(value).buildPartial();
} else {
result.bulkInsertCommand_ = value;
}
result.hasBulkInsertCommand = true;
return this;
}
public Builder clearBulkInsertCommand() {
result.hasBulkInsertCommand = false;
result.bulkInsertCommand_ = com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.BulkInsertCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BulkRemoveCommand bulkRemoveCommand = 9;
public boolean hasBulkRemoveCommand() {
return result.hasBulkRemoveCommand();
}
public com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand getBulkRemoveCommand() {
return result.getBulkRemoveCommand();
}
public Builder setBulkRemoveCommand(com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBulkRemoveCommand = true;
result.bulkRemoveCommand_ = value;
return this;
}
public Builder setBulkRemoveCommand(com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand.Builder builderForValue) {
result.hasBulkRemoveCommand = true;
result.bulkRemoveCommand_ = builderForValue.build();
return this;
}
public Builder mergeBulkRemoveCommand(com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand value) {
if (result.hasBulkRemoveCommand() &&
result.bulkRemoveCommand_ != com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand.getDefaultInstance()) {
result.bulkRemoveCommand_ =
com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand.newBuilder(result.bulkRemoveCommand_).mergeFrom(value).buildPartial();
} else {
result.bulkRemoveCommand_ = value;
}
result.hasBulkRemoveCommand = true;
return this;
}
public Builder clearBulkRemoveCommand() {
result.hasBulkRemoveCommand = false;
result.bulkRemoveCommand_ = com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.BulkRemoveCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.ClearCommand clearCommand = 10;
public boolean hasClearCommand() {
return result.hasClearCommand();
}
public com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand getClearCommand() {
return result.getClearCommand();
}
public Builder setClearCommand(com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasClearCommand = true;
result.clearCommand_ = value;
return this;
}
public Builder setClearCommand(com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand.Builder builderForValue) {
result.hasClearCommand = true;
result.clearCommand_ = builderForValue.build();
return this;
}
public Builder mergeClearCommand(com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand value) {
if (result.hasClearCommand() &&
result.clearCommand_ != com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand.getDefaultInstance()) {
result.clearCommand_ =
com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand.newBuilder(result.clearCommand_).mergeFrom(value).buildPartial();
} else {
result.clearCommand_ = value;
}
result.hasClearCommand = true;
return this;
}
public Builder clearClearCommand() {
result.hasClearCommand = false;
result.clearCommand_ = com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.ClearCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.ContainsCommand containsCommand = 11;
public boolean hasContainsCommand() {
return result.hasContainsCommand();
}
public com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand getContainsCommand() {
return result.getContainsCommand();
}
public Builder setContainsCommand(com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasContainsCommand = true;
result.containsCommand_ = value;
return this;
}
public Builder setContainsCommand(com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand.Builder builderForValue) {
result.hasContainsCommand = true;
result.containsCommand_ = builderForValue.build();
return this;
}
public Builder mergeContainsCommand(com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand value) {
if (result.hasContainsCommand() &&
result.containsCommand_ != com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand.getDefaultInstance()) {
result.containsCommand_ =
com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand.newBuilder(result.containsCommand_).mergeFrom(value).buildPartial();
} else {
result.containsCommand_ = value;
}
result.hasContainsCommand = true;
return this;
}
public Builder clearContainsCommand() {
result.hasContainsCommand = false;
result.containsCommand_ = com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.ContainsCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.CountCommand countCommand = 12;
public boolean hasCountCommand() {
return result.hasCountCommand();
}
public com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand getCountCommand() {
return result.getCountCommand();
}
public Builder setCountCommand(com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasCountCommand = true;
result.countCommand_ = value;
return this;
}
public Builder setCountCommand(com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand.Builder builderForValue) {
result.hasCountCommand = true;
result.countCommand_ = builderForValue.build();
return this;
}
public Builder mergeCountCommand(com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand value) {
if (result.hasCountCommand() &&
result.countCommand_ != com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand.getDefaultInstance()) {
result.countCommand_ =
com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand.newBuilder(result.countCommand_).mergeFrom(value).buildPartial();
} else {
result.countCommand_ = value;
}
result.hasCountCommand = true;
return this;
}
public Builder clearCountCommand() {
result.hasCountCommand = false;
result.countCommand_ = com.alachisoft.ncache.common.protobuf.CountCommandProtocol.CountCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.DisposeCommand disposeCommand = 13;
public boolean hasDisposeCommand() {
return result.hasDisposeCommand();
}
public com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand getDisposeCommand() {
return result.getDisposeCommand();
}
public Builder setDisposeCommand(com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDisposeCommand = true;
result.disposeCommand_ = value;
return this;
}
public Builder setDisposeCommand(com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand.Builder builderForValue) {
result.hasDisposeCommand = true;
result.disposeCommand_ = builderForValue.build();
return this;
}
public Builder mergeDisposeCommand(com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand value) {
if (result.hasDisposeCommand() &&
result.disposeCommand_ != com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand.getDefaultInstance()) {
result.disposeCommand_ =
com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand.newBuilder(result.disposeCommand_).mergeFrom(value).buildPartial();
} else {
result.disposeCommand_ = value;
}
result.hasDisposeCommand = true;
return this;
}
public Builder clearDisposeCommand() {
result.hasDisposeCommand = false;
result.disposeCommand_ = com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.DisposeCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetCacheItemCommand getCacheItemCommand = 14;
public boolean hasGetCacheItemCommand() {
return result.hasGetCacheItemCommand();
}
public com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand getGetCacheItemCommand() {
return result.getGetCacheItemCommand();
}
public Builder setGetCacheItemCommand(com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetCacheItemCommand = true;
result.getCacheItemCommand_ = value;
return this;
}
public Builder setGetCacheItemCommand(com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand.Builder builderForValue) {
result.hasGetCacheItemCommand = true;
result.getCacheItemCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetCacheItemCommand(com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand value) {
if (result.hasGetCacheItemCommand() &&
result.getCacheItemCommand_ != com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand.getDefaultInstance()) {
result.getCacheItemCommand_ =
com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand.newBuilder(result.getCacheItemCommand_).mergeFrom(value).buildPartial();
} else {
result.getCacheItemCommand_ = value;
}
result.hasGetCacheItemCommand = true;
return this;
}
public Builder clearGetCacheItemCommand() {
result.hasGetCacheItemCommand = false;
result.getCacheItemCommand_ = com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.GetCacheItemCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetCommand getCommand = 15;
public boolean hasGetCommand() {
return result.hasGetCommand();
}
public com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand getGetCommand() {
return result.getGetCommand();
}
public Builder setGetCommand(com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetCommand = true;
result.getCommand_ = value;
return this;
}
public Builder setGetCommand(com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand.Builder builderForValue) {
result.hasGetCommand = true;
result.getCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetCommand(com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand value) {
if (result.hasGetCommand() &&
result.getCommand_ != com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand.getDefaultInstance()) {
result.getCommand_ =
com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand.newBuilder(result.getCommand_).mergeFrom(value).buildPartial();
} else {
result.getCommand_ = value;
}
result.hasGetCommand = true;
return this;
}
public Builder clearGetCommand() {
result.hasGetCommand = false;
result.getCommand_ = com.alachisoft.ncache.common.protobuf.GetCommandProtocol.GetCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetCompactTypesCommand getCompactTypesCommand = 16;
public boolean hasGetCompactTypesCommand() {
return result.hasGetCompactTypesCommand();
}
public com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand getGetCompactTypesCommand() {
return result.getGetCompactTypesCommand();
}
public Builder setGetCompactTypesCommand(com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetCompactTypesCommand = true;
result.getCompactTypesCommand_ = value;
return this;
}
public Builder setGetCompactTypesCommand(com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand.Builder builderForValue) {
result.hasGetCompactTypesCommand = true;
result.getCompactTypesCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetCompactTypesCommand(com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand value) {
if (result.hasGetCompactTypesCommand() &&
result.getCompactTypesCommand_ != com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand.getDefaultInstance()) {
result.getCompactTypesCommand_ =
com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand.newBuilder(result.getCompactTypesCommand_).mergeFrom(value).buildPartial();
} else {
result.getCompactTypesCommand_ = value;
}
result.hasGetCompactTypesCommand = true;
return this;
}
public Builder clearGetCompactTypesCommand() {
result.hasGetCompactTypesCommand = false;
result.getCompactTypesCommand_ = com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.GetCompactTypesCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetEnumeratorCommand getEnumeratorCommand = 17;
public boolean hasGetEnumeratorCommand() {
return result.hasGetEnumeratorCommand();
}
public com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand getGetEnumeratorCommand() {
return result.getGetEnumeratorCommand();
}
public Builder setGetEnumeratorCommand(com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetEnumeratorCommand = true;
result.getEnumeratorCommand_ = value;
return this;
}
public Builder setGetEnumeratorCommand(com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand.Builder builderForValue) {
result.hasGetEnumeratorCommand = true;
result.getEnumeratorCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetEnumeratorCommand(com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand value) {
if (result.hasGetEnumeratorCommand() &&
result.getEnumeratorCommand_ != com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand.getDefaultInstance()) {
result.getEnumeratorCommand_ =
com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand.newBuilder(result.getEnumeratorCommand_).mergeFrom(value).buildPartial();
} else {
result.getEnumeratorCommand_ = value;
}
result.hasGetEnumeratorCommand = true;
return this;
}
public Builder clearGetEnumeratorCommand() {
result.hasGetEnumeratorCommand = false;
result.getEnumeratorCommand_ = com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.GetEnumeratorCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetGroupCommand getGroupCommand = 18;
public boolean hasGetGroupCommand() {
return result.hasGetGroupCommand();
}
public com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand getGetGroupCommand() {
return result.getGetGroupCommand();
}
public Builder setGetGroupCommand(com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetGroupCommand = true;
result.getGroupCommand_ = value;
return this;
}
public Builder setGetGroupCommand(com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand.Builder builderForValue) {
result.hasGetGroupCommand = true;
result.getGroupCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetGroupCommand(com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand value) {
if (result.hasGetGroupCommand() &&
result.getGroupCommand_ != com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand.getDefaultInstance()) {
result.getGroupCommand_ =
com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand.newBuilder(result.getGroupCommand_).mergeFrom(value).buildPartial();
} else {
result.getGroupCommand_ = value;
}
result.hasGetGroupCommand = true;
return this;
}
public Builder clearGetGroupCommand() {
result.hasGetGroupCommand = false;
result.getGroupCommand_ = com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.GetGroupCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetHashmapCommand getHashmapCommand = 19;
public boolean hasGetHashmapCommand() {
return result.hasGetHashmapCommand();
}
public com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand getGetHashmapCommand() {
return result.getGetHashmapCommand();
}
public Builder setGetHashmapCommand(com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetHashmapCommand = true;
result.getHashmapCommand_ = value;
return this;
}
public Builder setGetHashmapCommand(com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand.Builder builderForValue) {
result.hasGetHashmapCommand = true;
result.getHashmapCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetHashmapCommand(com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand value) {
if (result.hasGetHashmapCommand() &&
result.getHashmapCommand_ != com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand.getDefaultInstance()) {
result.getHashmapCommand_ =
com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand.newBuilder(result.getHashmapCommand_).mergeFrom(value).buildPartial();
} else {
result.getHashmapCommand_ = value;
}
result.hasGetHashmapCommand = true;
return this;
}
public Builder clearGetHashmapCommand() {
result.hasGetHashmapCommand = false;
result.getHashmapCommand_ = com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.GetHashmapCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetOptimalServerCommand getOptimalServerCommand = 20;
public boolean hasGetOptimalServerCommand() {
return result.hasGetOptimalServerCommand();
}
public com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand getGetOptimalServerCommand() {
return result.getGetOptimalServerCommand();
}
public Builder setGetOptimalServerCommand(com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetOptimalServerCommand = true;
result.getOptimalServerCommand_ = value;
return this;
}
public Builder setGetOptimalServerCommand(com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand.Builder builderForValue) {
result.hasGetOptimalServerCommand = true;
result.getOptimalServerCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetOptimalServerCommand(com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand value) {
if (result.hasGetOptimalServerCommand() &&
result.getOptimalServerCommand_ != com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand.getDefaultInstance()) {
result.getOptimalServerCommand_ =
com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand.newBuilder(result.getOptimalServerCommand_).mergeFrom(value).buildPartial();
} else {
result.getOptimalServerCommand_ = value;
}
result.hasGetOptimalServerCommand = true;
return this;
}
public Builder clearGetOptimalServerCommand() {
result.hasGetOptimalServerCommand = false;
result.getOptimalServerCommand_ = com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.GetOptimalServerCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommand getThresholdSizeCommand = 21;
public boolean hasGetThresholdSizeCommand() {
return result.hasGetThresholdSizeCommand();
}
public com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand getGetThresholdSizeCommand() {
return result.getGetThresholdSizeCommand();
}
public Builder setGetThresholdSizeCommand(com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetThresholdSizeCommand = true;
result.getThresholdSizeCommand_ = value;
return this;
}
public Builder setGetThresholdSizeCommand(com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand.Builder builderForValue) {
result.hasGetThresholdSizeCommand = true;
result.getThresholdSizeCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetThresholdSizeCommand(com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand value) {
if (result.hasGetThresholdSizeCommand() &&
result.getThresholdSizeCommand_ != com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand.getDefaultInstance()) {
result.getThresholdSizeCommand_ =
com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand.newBuilder(result.getThresholdSizeCommand_).mergeFrom(value).buildPartial();
} else {
result.getThresholdSizeCommand_ = value;
}
result.hasGetThresholdSizeCommand = true;
return this;
}
public Builder clearGetThresholdSizeCommand() {
result.hasGetThresholdSizeCommand = false;
result.getThresholdSizeCommand_ = com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.GetThresholdSizeCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommand getTypeInfoMapCommand = 22;
public boolean hasGetTypeInfoMapCommand() {
return result.hasGetTypeInfoMapCommand();
}
public com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand getGetTypeInfoMapCommand() {
return result.getGetTypeInfoMapCommand();
}
public Builder setGetTypeInfoMapCommand(com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetTypeInfoMapCommand = true;
result.getTypeInfoMapCommand_ = value;
return this;
}
public Builder setGetTypeInfoMapCommand(com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand.Builder builderForValue) {
result.hasGetTypeInfoMapCommand = true;
result.getTypeInfoMapCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetTypeInfoMapCommand(com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand value) {
if (result.hasGetTypeInfoMapCommand() &&
result.getTypeInfoMapCommand_ != com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand.getDefaultInstance()) {
result.getTypeInfoMapCommand_ =
com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand.newBuilder(result.getTypeInfoMapCommand_).mergeFrom(value).buildPartial();
} else {
result.getTypeInfoMapCommand_ = value;
}
result.hasGetTypeInfoMapCommand = true;
return this;
}
public Builder clearGetTypeInfoMapCommand() {
result.hasGetTypeInfoMapCommand = false;
result.getTypeInfoMapCommand_ = com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.GetTypeInfoMapCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.InitCommand initCommand = 23;
public boolean hasInitCommand() {
return result.hasInitCommand();
}
public com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand getInitCommand() {
return result.getInitCommand();
}
public Builder setInitCommand(com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasInitCommand = true;
result.initCommand_ = value;
return this;
}
public Builder setInitCommand(com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand.Builder builderForValue) {
result.hasInitCommand = true;
result.initCommand_ = builderForValue.build();
return this;
}
public Builder mergeInitCommand(com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand value) {
if (result.hasInitCommand() &&
result.initCommand_ != com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand.getDefaultInstance()) {
result.initCommand_ =
com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand.newBuilder(result.initCommand_).mergeFrom(value).buildPartial();
} else {
result.initCommand_ = value;
}
result.hasInitCommand = true;
return this;
}
public Builder clearInitCommand() {
result.hasInitCommand = false;
result.initCommand_ = com.alachisoft.ncache.common.protobuf.InitCommandProtocol.InitCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.InsertCommand insertCommand = 24;
public boolean hasInsertCommand() {
return result.hasInsertCommand();
}
public com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand getInsertCommand() {
return result.getInsertCommand();
}
public Builder setInsertCommand(com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasInsertCommand = true;
result.insertCommand_ = value;
return this;
}
public Builder setInsertCommand(com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand.Builder builderForValue) {
result.hasInsertCommand = true;
result.insertCommand_ = builderForValue.build();
return this;
}
public Builder mergeInsertCommand(com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand value) {
if (result.hasInsertCommand() &&
result.insertCommand_ != com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand.getDefaultInstance()) {
result.insertCommand_ =
com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand.newBuilder(result.insertCommand_).mergeFrom(value).buildPartial();
} else {
result.insertCommand_ = value;
}
result.hasInsertCommand = true;
return this;
}
public Builder clearInsertCommand() {
result.hasInsertCommand = false;
result.insertCommand_ = com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.InsertCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommand raiseCustomEventCommand = 25;
public boolean hasRaiseCustomEventCommand() {
return result.hasRaiseCustomEventCommand();
}
public com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand getRaiseCustomEventCommand() {
return result.getRaiseCustomEventCommand();
}
public Builder setRaiseCustomEventCommand(com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRaiseCustomEventCommand = true;
result.raiseCustomEventCommand_ = value;
return this;
}
public Builder setRaiseCustomEventCommand(com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand.Builder builderForValue) {
result.hasRaiseCustomEventCommand = true;
result.raiseCustomEventCommand_ = builderForValue.build();
return this;
}
public Builder mergeRaiseCustomEventCommand(com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand value) {
if (result.hasRaiseCustomEventCommand() &&
result.raiseCustomEventCommand_ != com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand.getDefaultInstance()) {
result.raiseCustomEventCommand_ =
com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand.newBuilder(result.raiseCustomEventCommand_).mergeFrom(value).buildPartial();
} else {
result.raiseCustomEventCommand_ = value;
}
result.hasRaiseCustomEventCommand = true;
return this;
}
public Builder clearRaiseCustomEventCommand() {
result.hasRaiseCustomEventCommand = false;
result.raiseCustomEventCommand_ = com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.RaiseCustomEventCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommand registerKeyNotifCommand = 26;
public boolean hasRegisterKeyNotifCommand() {
return result.hasRegisterKeyNotifCommand();
}
public com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand getRegisterKeyNotifCommand() {
return result.getRegisterKeyNotifCommand();
}
public Builder setRegisterKeyNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRegisterKeyNotifCommand = true;
result.registerKeyNotifCommand_ = value;
return this;
}
public Builder setRegisterKeyNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand.Builder builderForValue) {
result.hasRegisterKeyNotifCommand = true;
result.registerKeyNotifCommand_ = builderForValue.build();
return this;
}
public Builder mergeRegisterKeyNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand value) {
if (result.hasRegisterKeyNotifCommand() &&
result.registerKeyNotifCommand_ != com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand.getDefaultInstance()) {
result.registerKeyNotifCommand_ =
com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand.newBuilder(result.registerKeyNotifCommand_).mergeFrom(value).buildPartial();
} else {
result.registerKeyNotifCommand_ = value;
}
result.hasRegisterKeyNotifCommand = true;
return this;
}
public Builder clearRegisterKeyNotifCommand() {
result.hasRegisterKeyNotifCommand = false;
result.registerKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.RegisterKeyNotifCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RegisterNotifCommand registerNotifCommand = 27;
public boolean hasRegisterNotifCommand() {
return result.hasRegisterNotifCommand();
}
public com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand getRegisterNotifCommand() {
return result.getRegisterNotifCommand();
}
public Builder setRegisterNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRegisterNotifCommand = true;
result.registerNotifCommand_ = value;
return this;
}
public Builder setRegisterNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand.Builder builderForValue) {
result.hasRegisterNotifCommand = true;
result.registerNotifCommand_ = builderForValue.build();
return this;
}
public Builder mergeRegisterNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand value) {
if (result.hasRegisterNotifCommand() &&
result.registerNotifCommand_ != com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand.getDefaultInstance()) {
result.registerNotifCommand_ =
com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand.newBuilder(result.registerNotifCommand_).mergeFrom(value).buildPartial();
} else {
result.registerNotifCommand_ = value;
}
result.hasRegisterNotifCommand = true;
return this;
}
public Builder clearRegisterNotifCommand() {
result.hasRegisterNotifCommand = false;
result.registerNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.RegisterNotifCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RemoveCommand removeCommand = 28;
public boolean hasRemoveCommand() {
return result.hasRemoveCommand();
}
public com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand getRemoveCommand() {
return result.getRemoveCommand();
}
public Builder setRemoveCommand(com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRemoveCommand = true;
result.removeCommand_ = value;
return this;
}
public Builder setRemoveCommand(com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand.Builder builderForValue) {
result.hasRemoveCommand = true;
result.removeCommand_ = builderForValue.build();
return this;
}
public Builder mergeRemoveCommand(com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand value) {
if (result.hasRemoveCommand() &&
result.removeCommand_ != com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand.getDefaultInstance()) {
result.removeCommand_ =
com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand.newBuilder(result.removeCommand_).mergeFrom(value).buildPartial();
} else {
result.removeCommand_ = value;
}
result.hasRemoveCommand = true;
return this;
}
public Builder clearRemoveCommand() {
result.hasRemoveCommand = false;
result.removeCommand_ = com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.RemoveCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RemoveGroupCommand removeGroupCommand = 29;
public boolean hasRemoveGroupCommand() {
return result.hasRemoveGroupCommand();
}
public com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand getRemoveGroupCommand() {
return result.getRemoveGroupCommand();
}
public Builder setRemoveGroupCommand(com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRemoveGroupCommand = true;
result.removeGroupCommand_ = value;
return this;
}
public Builder setRemoveGroupCommand(com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand.Builder builderForValue) {
result.hasRemoveGroupCommand = true;
result.removeGroupCommand_ = builderForValue.build();
return this;
}
public Builder mergeRemoveGroupCommand(com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand value) {
if (result.hasRemoveGroupCommand() &&
result.removeGroupCommand_ != com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand.getDefaultInstance()) {
result.removeGroupCommand_ =
com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand.newBuilder(result.removeGroupCommand_).mergeFrom(value).buildPartial();
} else {
result.removeGroupCommand_ = value;
}
result.hasRemoveGroupCommand = true;
return this;
}
public Builder clearRemoveGroupCommand() {
result.hasRemoveGroupCommand = false;
result.removeGroupCommand_ = com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.RemoveGroupCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SearchCommand searchCommand = 30;
public boolean hasSearchCommand() {
return result.hasSearchCommand();
}
public com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand getSearchCommand() {
return result.getSearchCommand();
}
public Builder setSearchCommand(com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSearchCommand = true;
result.searchCommand_ = value;
return this;
}
public Builder setSearchCommand(com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand.Builder builderForValue) {
result.hasSearchCommand = true;
result.searchCommand_ = builderForValue.build();
return this;
}
public Builder mergeSearchCommand(com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand value) {
if (result.hasSearchCommand() &&
result.searchCommand_ != com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand.getDefaultInstance()) {
result.searchCommand_ =
com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand.newBuilder(result.searchCommand_).mergeFrom(value).buildPartial();
} else {
result.searchCommand_ = value;
}
result.hasSearchCommand = true;
return this;
}
public Builder clearSearchCommand() {
result.hasSearchCommand = false;
result.searchCommand_ = com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.SearchCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetTagCommand getTagCommand = 31;
public boolean hasGetTagCommand() {
return result.hasGetTagCommand();
}
public com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand getGetTagCommand() {
return result.getGetTagCommand();
}
public Builder setGetTagCommand(com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetTagCommand = true;
result.getTagCommand_ = value;
return this;
}
public Builder setGetTagCommand(com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand.Builder builderForValue) {
result.hasGetTagCommand = true;
result.getTagCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetTagCommand(com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand value) {
if (result.hasGetTagCommand() &&
result.getTagCommand_ != com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand.getDefaultInstance()) {
result.getTagCommand_ =
com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand.newBuilder(result.getTagCommand_).mergeFrom(value).buildPartial();
} else {
result.getTagCommand_ = value;
}
result.hasGetTagCommand = true;
return this;
}
public Builder clearGetTagCommand() {
result.hasGetTagCommand = false;
result.getTagCommand_ = com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.GetTagCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.LockCommand lockCommand = 32;
public boolean hasLockCommand() {
return result.hasLockCommand();
}
public com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand getLockCommand() {
return result.getLockCommand();
}
public Builder setLockCommand(com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasLockCommand = true;
result.lockCommand_ = value;
return this;
}
public Builder setLockCommand(com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand.Builder builderForValue) {
result.hasLockCommand = true;
result.lockCommand_ = builderForValue.build();
return this;
}
public Builder mergeLockCommand(com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand value) {
if (result.hasLockCommand() &&
result.lockCommand_ != com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand.getDefaultInstance()) {
result.lockCommand_ =
com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand.newBuilder(result.lockCommand_).mergeFrom(value).buildPartial();
} else {
result.lockCommand_ = value;
}
result.hasLockCommand = true;
return this;
}
public Builder clearLockCommand() {
result.hasLockCommand = false;
result.lockCommand_ = com.alachisoft.ncache.common.protobuf.LockCommandProtocol.LockCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.UnlockCommand unlockCommand = 33;
public boolean hasUnlockCommand() {
return result.hasUnlockCommand();
}
public com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand getUnlockCommand() {
return result.getUnlockCommand();
}
public Builder setUnlockCommand(com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasUnlockCommand = true;
result.unlockCommand_ = value;
return this;
}
public Builder setUnlockCommand(com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand.Builder builderForValue) {
result.hasUnlockCommand = true;
result.unlockCommand_ = builderForValue.build();
return this;
}
public Builder mergeUnlockCommand(com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand value) {
if (result.hasUnlockCommand() &&
result.unlockCommand_ != com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand.getDefaultInstance()) {
result.unlockCommand_ =
com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand.newBuilder(result.unlockCommand_).mergeFrom(value).buildPartial();
} else {
result.unlockCommand_ = value;
}
result.hasUnlockCommand = true;
return this;
}
public Builder clearUnlockCommand() {
result.hasUnlockCommand = false;
result.unlockCommand_ = com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.UnlockCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.IsLockedCommand isLockedCommand = 34;
public boolean hasIsLockedCommand() {
return result.hasIsLockedCommand();
}
public com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand getIsLockedCommand() {
return result.getIsLockedCommand();
}
public Builder setIsLockedCommand(com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasIsLockedCommand = true;
result.isLockedCommand_ = value;
return this;
}
public Builder setIsLockedCommand(com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand.Builder builderForValue) {
result.hasIsLockedCommand = true;
result.isLockedCommand_ = builderForValue.build();
return this;
}
public Builder mergeIsLockedCommand(com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand value) {
if (result.hasIsLockedCommand() &&
result.isLockedCommand_ != com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand.getDefaultInstance()) {
result.isLockedCommand_ =
com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand.newBuilder(result.isLockedCommand_).mergeFrom(value).buildPartial();
} else {
result.isLockedCommand_ = value;
}
result.hasIsLockedCommand = true;
return this;
}
public Builder clearIsLockedCommand() {
result.hasIsLockedCommand = false;
result.isLockedCommand_ = com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.IsLockedCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.LockVerifyCommand lockVerifyCommand = 35;
public boolean hasLockVerifyCommand() {
return result.hasLockVerifyCommand();
}
public com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand getLockVerifyCommand() {
return result.getLockVerifyCommand();
}
public Builder setLockVerifyCommand(com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasLockVerifyCommand = true;
result.lockVerifyCommand_ = value;
return this;
}
public Builder setLockVerifyCommand(com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand.Builder builderForValue) {
result.hasLockVerifyCommand = true;
result.lockVerifyCommand_ = builderForValue.build();
return this;
}
public Builder mergeLockVerifyCommand(com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand value) {
if (result.hasLockVerifyCommand() &&
result.lockVerifyCommand_ != com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand.getDefaultInstance()) {
result.lockVerifyCommand_ =
com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand.newBuilder(result.lockVerifyCommand_).mergeFrom(value).buildPartial();
} else {
result.lockVerifyCommand_ = value;
}
result.hasLockVerifyCommand = true;
return this;
}
public Builder clearLockVerifyCommand() {
result.hasLockVerifyCommand = false;
result.lockVerifyCommand_ = com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.LockVerifyCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommand unRegisterKeyNotifCommand = 36;
public boolean hasUnRegisterKeyNotifCommand() {
return result.hasUnRegisterKeyNotifCommand();
}
public com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand getUnRegisterKeyNotifCommand() {
return result.getUnRegisterKeyNotifCommand();
}
public Builder setUnRegisterKeyNotifCommand(com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasUnRegisterKeyNotifCommand = true;
result.unRegisterKeyNotifCommand_ = value;
return this;
}
public Builder setUnRegisterKeyNotifCommand(com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand.Builder builderForValue) {
result.hasUnRegisterKeyNotifCommand = true;
result.unRegisterKeyNotifCommand_ = builderForValue.build();
return this;
}
public Builder mergeUnRegisterKeyNotifCommand(com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand value) {
if (result.hasUnRegisterKeyNotifCommand() &&
result.unRegisterKeyNotifCommand_ != com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand.getDefaultInstance()) {
result.unRegisterKeyNotifCommand_ =
com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand.newBuilder(result.unRegisterKeyNotifCommand_).mergeFrom(value).buildPartial();
} else {
result.unRegisterKeyNotifCommand_ = value;
}
result.hasUnRegisterKeyNotifCommand = true;
return this;
}
public Builder clearUnRegisterKeyNotifCommand() {
result.hasUnRegisterKeyNotifCommand = false;
result.unRegisterKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.UnRegisterKeyNotifCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommand unRegisterBulkKeyNotifCommand = 37;
public boolean hasUnRegisterBulkKeyNotifCommand() {
return result.hasUnRegisterBulkKeyNotifCommand();
}
public com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand getUnRegisterBulkKeyNotifCommand() {
return result.getUnRegisterBulkKeyNotifCommand();
}
public Builder setUnRegisterBulkKeyNotifCommand(com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasUnRegisterBulkKeyNotifCommand = true;
result.unRegisterBulkKeyNotifCommand_ = value;
return this;
}
public Builder setUnRegisterBulkKeyNotifCommand(com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand.Builder builderForValue) {
result.hasUnRegisterBulkKeyNotifCommand = true;
result.unRegisterBulkKeyNotifCommand_ = builderForValue.build();
return this;
}
public Builder mergeUnRegisterBulkKeyNotifCommand(com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand value) {
if (result.hasUnRegisterBulkKeyNotifCommand() &&
result.unRegisterBulkKeyNotifCommand_ != com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand.getDefaultInstance()) {
result.unRegisterBulkKeyNotifCommand_ =
com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand.newBuilder(result.unRegisterBulkKeyNotifCommand_).mergeFrom(value).buildPartial();
} else {
result.unRegisterBulkKeyNotifCommand_ = value;
}
result.hasUnRegisterBulkKeyNotifCommand = true;
return this;
}
public Builder clearUnRegisterBulkKeyNotifCommand() {
result.hasUnRegisterBulkKeyNotifCommand = false;
result.unRegisterBulkKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.UnRegisterBulkKeyNotifCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommand registerBulkKeyNotifCommand = 38;
public boolean hasRegisterBulkKeyNotifCommand() {
return result.hasRegisterBulkKeyNotifCommand();
}
public com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand getRegisterBulkKeyNotifCommand() {
return result.getRegisterBulkKeyNotifCommand();
}
public Builder setRegisterBulkKeyNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRegisterBulkKeyNotifCommand = true;
result.registerBulkKeyNotifCommand_ = value;
return this;
}
public Builder setRegisterBulkKeyNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand.Builder builderForValue) {
result.hasRegisterBulkKeyNotifCommand = true;
result.registerBulkKeyNotifCommand_ = builderForValue.build();
return this;
}
public Builder mergeRegisterBulkKeyNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand value) {
if (result.hasRegisterBulkKeyNotifCommand() &&
result.registerBulkKeyNotifCommand_ != com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand.getDefaultInstance()) {
result.registerBulkKeyNotifCommand_ =
com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand.newBuilder(result.registerBulkKeyNotifCommand_).mergeFrom(value).buildPartial();
} else {
result.registerBulkKeyNotifCommand_ = value;
}
result.hasRegisterBulkKeyNotifCommand = true;
return this;
}
public Builder clearRegisterBulkKeyNotifCommand() {
result.hasRegisterBulkKeyNotifCommand = false;
result.registerBulkKeyNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.RegisterBulkKeyNotifCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.HybridBulkCommand hybridBulkCommand = 39;
public boolean hasHybridBulkCommand() {
return result.hasHybridBulkCommand();
}
public com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand getHybridBulkCommand() {
return result.getHybridBulkCommand();
}
public Builder setHybridBulkCommand(com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasHybridBulkCommand = true;
result.hybridBulkCommand_ = value;
return this;
}
public Builder setHybridBulkCommand(com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand.Builder builderForValue) {
result.hasHybridBulkCommand = true;
result.hybridBulkCommand_ = builderForValue.build();
return this;
}
public Builder mergeHybridBulkCommand(com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand value) {
if (result.hasHybridBulkCommand() &&
result.hybridBulkCommand_ != com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand.getDefaultInstance()) {
result.hybridBulkCommand_ =
com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand.newBuilder(result.hybridBulkCommand_).mergeFrom(value).buildPartial();
} else {
result.hybridBulkCommand_ = value;
}
result.hasHybridBulkCommand = true;
return this;
}
public Builder clearHybridBulkCommand() {
result.hasHybridBulkCommand = false;
result.hybridBulkCommand_ = com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.HybridBulkCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommand getLoggingInfoCommand = 40;
public boolean hasGetLoggingInfoCommand() {
return result.hasGetLoggingInfoCommand();
}
public com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand getGetLoggingInfoCommand() {
return result.getGetLoggingInfoCommand();
}
public Builder setGetLoggingInfoCommand(com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetLoggingInfoCommand = true;
result.getLoggingInfoCommand_ = value;
return this;
}
public Builder setGetLoggingInfoCommand(com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand.Builder builderForValue) {
result.hasGetLoggingInfoCommand = true;
result.getLoggingInfoCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetLoggingInfoCommand(com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand value) {
if (result.hasGetLoggingInfoCommand() &&
result.getLoggingInfoCommand_ != com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand.getDefaultInstance()) {
result.getLoggingInfoCommand_ =
com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand.newBuilder(result.getLoggingInfoCommand_).mergeFrom(value).buildPartial();
} else {
result.getLoggingInfoCommand_ = value;
}
result.hasGetLoggingInfoCommand = true;
return this;
}
public Builder clearGetLoggingInfoCommand() {
result.hasGetLoggingInfoCommand = false;
result.getLoggingInfoCommand_ = com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.GetLoggingInfoCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.CloseStreamCommand closeStreamCommand = 41;
public boolean hasCloseStreamCommand() {
return result.hasCloseStreamCommand();
}
public com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand getCloseStreamCommand() {
return result.getCloseStreamCommand();
}
public Builder setCloseStreamCommand(com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasCloseStreamCommand = true;
result.closeStreamCommand_ = value;
return this;
}
public Builder setCloseStreamCommand(com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand.Builder builderForValue) {
result.hasCloseStreamCommand = true;
result.closeStreamCommand_ = builderForValue.build();
return this;
}
public Builder mergeCloseStreamCommand(com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand value) {
if (result.hasCloseStreamCommand() &&
result.closeStreamCommand_ != com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand.getDefaultInstance()) {
result.closeStreamCommand_ =
com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand.newBuilder(result.closeStreamCommand_).mergeFrom(value).buildPartial();
} else {
result.closeStreamCommand_ = value;
}
result.hasCloseStreamCommand = true;
return this;
}
public Builder clearCloseStreamCommand() {
result.hasCloseStreamCommand = false;
result.closeStreamCommand_ = com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.CloseStreamCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetStreamLengthCommand getStreamLengthCommand = 42;
public boolean hasGetStreamLengthCommand() {
return result.hasGetStreamLengthCommand();
}
public com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand getGetStreamLengthCommand() {
return result.getGetStreamLengthCommand();
}
public Builder setGetStreamLengthCommand(com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetStreamLengthCommand = true;
result.getStreamLengthCommand_ = value;
return this;
}
public Builder setGetStreamLengthCommand(com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand.Builder builderForValue) {
result.hasGetStreamLengthCommand = true;
result.getStreamLengthCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetStreamLengthCommand(com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand value) {
if (result.hasGetStreamLengthCommand() &&
result.getStreamLengthCommand_ != com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand.getDefaultInstance()) {
result.getStreamLengthCommand_ =
com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand.newBuilder(result.getStreamLengthCommand_).mergeFrom(value).buildPartial();
} else {
result.getStreamLengthCommand_ = value;
}
result.hasGetStreamLengthCommand = true;
return this;
}
public Builder clearGetStreamLengthCommand() {
result.hasGetStreamLengthCommand = false;
result.getStreamLengthCommand_ = com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.GetStreamLengthCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.OpenStreamCommand openStreamCommand = 43;
public boolean hasOpenStreamCommand() {
return result.hasOpenStreamCommand();
}
public com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand getOpenStreamCommand() {
return result.getOpenStreamCommand();
}
public Builder setOpenStreamCommand(com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasOpenStreamCommand = true;
result.openStreamCommand_ = value;
return this;
}
public Builder setOpenStreamCommand(com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand.Builder builderForValue) {
result.hasOpenStreamCommand = true;
result.openStreamCommand_ = builderForValue.build();
return this;
}
public Builder mergeOpenStreamCommand(com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand value) {
if (result.hasOpenStreamCommand() &&
result.openStreamCommand_ != com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand.getDefaultInstance()) {
result.openStreamCommand_ =
com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand.newBuilder(result.openStreamCommand_).mergeFrom(value).buildPartial();
} else {
result.openStreamCommand_ = value;
}
result.hasOpenStreamCommand = true;
return this;
}
public Builder clearOpenStreamCommand() {
result.hasOpenStreamCommand = false;
result.openStreamCommand_ = com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.WriteToStreamCommand writeToStreamCommand = 44;
public boolean hasWriteToStreamCommand() {
return result.hasWriteToStreamCommand();
}
public com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand getWriteToStreamCommand() {
return result.getWriteToStreamCommand();
}
public Builder setWriteToStreamCommand(com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasWriteToStreamCommand = true;
result.writeToStreamCommand_ = value;
return this;
}
public Builder setWriteToStreamCommand(com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand.Builder builderForValue) {
result.hasWriteToStreamCommand = true;
result.writeToStreamCommand_ = builderForValue.build();
return this;
}
public Builder mergeWriteToStreamCommand(com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand value) {
if (result.hasWriteToStreamCommand() &&
result.writeToStreamCommand_ != com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand.getDefaultInstance()) {
result.writeToStreamCommand_ =
com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand.newBuilder(result.writeToStreamCommand_).mergeFrom(value).buildPartial();
} else {
result.writeToStreamCommand_ = value;
}
result.hasWriteToStreamCommand = true;
return this;
}
public Builder clearWriteToStreamCommand() {
result.hasWriteToStreamCommand = false;
result.writeToStreamCommand_ = com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.WriteToStreamCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.ReadFromStreamCommand readFromStreamCommand = 45;
public boolean hasReadFromStreamCommand() {
return result.hasReadFromStreamCommand();
}
public com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand getReadFromStreamCommand() {
return result.getReadFromStreamCommand();
}
public Builder setReadFromStreamCommand(com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasReadFromStreamCommand = true;
result.readFromStreamCommand_ = value;
return this;
}
public Builder setReadFromStreamCommand(com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand.Builder builderForValue) {
result.hasReadFromStreamCommand = true;
result.readFromStreamCommand_ = builderForValue.build();
return this;
}
public Builder mergeReadFromStreamCommand(com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand value) {
if (result.hasReadFromStreamCommand() &&
result.readFromStreamCommand_ != com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand.getDefaultInstance()) {
result.readFromStreamCommand_ =
com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand.newBuilder(result.readFromStreamCommand_).mergeFrom(value).buildPartial();
} else {
result.readFromStreamCommand_ = value;
}
result.hasReadFromStreamCommand = true;
return this;
}
public Builder clearReadFromStreamCommand() {
result.hasReadFromStreamCommand = false;
result.readFromStreamCommand_ = com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.ReadFromStreamCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommand bridgeGetStateTransferInfoCommand = 46;
public boolean hasBridgeGetStateTransferInfoCommand() {
return result.hasBridgeGetStateTransferInfoCommand();
}
public com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand getBridgeGetStateTransferInfoCommand() {
return result.getBridgeGetStateTransferInfoCommand();
}
public Builder setBridgeGetStateTransferInfoCommand(com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeGetStateTransferInfoCommand = true;
result.bridgeGetStateTransferInfoCommand_ = value;
return this;
}
public Builder setBridgeGetStateTransferInfoCommand(com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand.Builder builderForValue) {
result.hasBridgeGetStateTransferInfoCommand = true;
result.bridgeGetStateTransferInfoCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeGetStateTransferInfoCommand(com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand value) {
if (result.hasBridgeGetStateTransferInfoCommand() &&
result.bridgeGetStateTransferInfoCommand_ != com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand.getDefaultInstance()) {
result.bridgeGetStateTransferInfoCommand_ =
com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand.newBuilder(result.bridgeGetStateTransferInfoCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeGetStateTransferInfoCommand_ = value;
}
result.hasBridgeGetStateTransferInfoCommand = true;
return this;
}
public Builder clearBridgeGetStateTransferInfoCommand() {
result.hasBridgeGetStateTransferInfoCommand = false;
result.bridgeGetStateTransferInfoCommand_ = com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.GetStateTransferInfoCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommand bridgeGetTransferableKeyListCommand = 47;
public boolean hasBridgeGetTransferableKeyListCommand() {
return result.hasBridgeGetTransferableKeyListCommand();
}
public com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand getBridgeGetTransferableKeyListCommand() {
return result.getBridgeGetTransferableKeyListCommand();
}
public Builder setBridgeGetTransferableKeyListCommand(com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeGetTransferableKeyListCommand = true;
result.bridgeGetTransferableKeyListCommand_ = value;
return this;
}
public Builder setBridgeGetTransferableKeyListCommand(com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand.Builder builderForValue) {
result.hasBridgeGetTransferableKeyListCommand = true;
result.bridgeGetTransferableKeyListCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeGetTransferableKeyListCommand(com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand value) {
if (result.hasBridgeGetTransferableKeyListCommand() &&
result.bridgeGetTransferableKeyListCommand_ != com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand.getDefaultInstance()) {
result.bridgeGetTransferableKeyListCommand_ =
com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand.newBuilder(result.bridgeGetTransferableKeyListCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeGetTransferableKeyListCommand_ = value;
}
result.hasBridgeGetTransferableKeyListCommand = true;
return this;
}
public Builder clearBridgeGetTransferableKeyListCommand() {
result.hasBridgeGetTransferableKeyListCommand = false;
result.bridgeGetTransferableKeyListCommand_ = com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.GetTransferableKeyListCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommand bridgeHybridBulkCommand = 48;
public boolean hasBridgeHybridBulkCommand() {
return result.hasBridgeHybridBulkCommand();
}
public com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand getBridgeHybridBulkCommand() {
return result.getBridgeHybridBulkCommand();
}
public Builder setBridgeHybridBulkCommand(com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeHybridBulkCommand = true;
result.bridgeHybridBulkCommand_ = value;
return this;
}
public Builder setBridgeHybridBulkCommand(com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand.Builder builderForValue) {
result.hasBridgeHybridBulkCommand = true;
result.bridgeHybridBulkCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeHybridBulkCommand(com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand value) {
if (result.hasBridgeHybridBulkCommand() &&
result.bridgeHybridBulkCommand_ != com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand.getDefaultInstance()) {
result.bridgeHybridBulkCommand_ =
com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand.newBuilder(result.bridgeHybridBulkCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeHybridBulkCommand_ = value;
}
result.hasBridgeHybridBulkCommand = true;
return this;
}
public Builder clearBridgeHybridBulkCommand() {
result.hasBridgeHybridBulkCommand = false;
result.bridgeHybridBulkCommand_ = com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.BridgeHybridBulkCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BridgeInitCommand bridgeInitCommand = 49;
public boolean hasBridgeInitCommand() {
return result.hasBridgeInitCommand();
}
public com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand getBridgeInitCommand() {
return result.getBridgeInitCommand();
}
public Builder setBridgeInitCommand(com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeInitCommand = true;
result.bridgeInitCommand_ = value;
return this;
}
public Builder setBridgeInitCommand(com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand.Builder builderForValue) {
result.hasBridgeInitCommand = true;
result.bridgeInitCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeInitCommand(com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand value) {
if (result.hasBridgeInitCommand() &&
result.bridgeInitCommand_ != com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand.getDefaultInstance()) {
result.bridgeInitCommand_ =
com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand.newBuilder(result.bridgeInitCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeInitCommand_ = value;
}
result.hasBridgeInitCommand = true;
return this;
}
public Builder clearBridgeInitCommand() {
result.hasBridgeInitCommand = false;
result.bridgeInitCommand_ = com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.BridgeInitCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommand bridgeSetTransferableKeyListCommand = 50;
public boolean hasBridgeSetTransferableKeyListCommand() {
return result.hasBridgeSetTransferableKeyListCommand();
}
public com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand getBridgeSetTransferableKeyListCommand() {
return result.getBridgeSetTransferableKeyListCommand();
}
public Builder setBridgeSetTransferableKeyListCommand(com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeSetTransferableKeyListCommand = true;
result.bridgeSetTransferableKeyListCommand_ = value;
return this;
}
public Builder setBridgeSetTransferableKeyListCommand(com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand.Builder builderForValue) {
result.hasBridgeSetTransferableKeyListCommand = true;
result.bridgeSetTransferableKeyListCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeSetTransferableKeyListCommand(com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand value) {
if (result.hasBridgeSetTransferableKeyListCommand() &&
result.bridgeSetTransferableKeyListCommand_ != com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand.getDefaultInstance()) {
result.bridgeSetTransferableKeyListCommand_ =
com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand.newBuilder(result.bridgeSetTransferableKeyListCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeSetTransferableKeyListCommand_ = value;
}
result.hasBridgeSetTransferableKeyListCommand = true;
return this;
}
public Builder clearBridgeSetTransferableKeyListCommand() {
result.hasBridgeSetTransferableKeyListCommand = false;
result.bridgeSetTransferableKeyListCommand_ = com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.SetTransferableKeyListCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommand bridgeSignlEndOfStateTransferCommand = 51;
public boolean hasBridgeSignlEndOfStateTransferCommand() {
return result.hasBridgeSignlEndOfStateTransferCommand();
}
public com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand getBridgeSignlEndOfStateTransferCommand() {
return result.getBridgeSignlEndOfStateTransferCommand();
}
public Builder setBridgeSignlEndOfStateTransferCommand(com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeSignlEndOfStateTransferCommand = true;
result.bridgeSignlEndOfStateTransferCommand_ = value;
return this;
}
public Builder setBridgeSignlEndOfStateTransferCommand(com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand.Builder builderForValue) {
result.hasBridgeSignlEndOfStateTransferCommand = true;
result.bridgeSignlEndOfStateTransferCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeSignlEndOfStateTransferCommand(com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand value) {
if (result.hasBridgeSignlEndOfStateTransferCommand() &&
result.bridgeSignlEndOfStateTransferCommand_ != com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand.getDefaultInstance()) {
result.bridgeSignlEndOfStateTransferCommand_ =
com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand.newBuilder(result.bridgeSignlEndOfStateTransferCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeSignlEndOfStateTransferCommand_ = value;
}
result.hasBridgeSignlEndOfStateTransferCommand = true;
return this;
}
public Builder clearBridgeSignlEndOfStateTransferCommand() {
result.hasBridgeSignlEndOfStateTransferCommand = false;
result.bridgeSignlEndOfStateTransferCommand_ = com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.SignlEndOfStateTransferCommand.getDefaultInstance();
return this;
}
// optional int64 requestID = 52;
public boolean hasRequestID() {
return result.hasRequestID();
}
public long getRequestID() {
return result.getRequestID();
}
public Builder setRequestID(long value) {
result.hasRequestID = true;
result.requestID_ = value;
return this;
}
public Builder clearRequestID() {
result.hasRequestID = false;
result.requestID_ = 0L;
return this;
}
// optional int32 commandVersion = 53 [default = 0];
public boolean hasCommandVersion() {
return result.hasCommandVersion();
}
public int getCommandVersion() {
return result.getCommandVersion();
}
public Builder setCommandVersion(int value) {
result.hasCommandVersion = true;
result.commandVersion_ = value;
return this;
}
public Builder clearCommandVersion() {
result.hasCommandVersion = false;
result.commandVersion_ = 0;
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BridgeSyncTimeCommand bridgeSyncTimeCommand = 54;
public boolean hasBridgeSyncTimeCommand() {
return result.hasBridgeSyncTimeCommand();
}
public com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand getBridgeSyncTimeCommand() {
return result.getBridgeSyncTimeCommand();
}
public Builder setBridgeSyncTimeCommand(com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeSyncTimeCommand = true;
result.bridgeSyncTimeCommand_ = value;
return this;
}
public Builder setBridgeSyncTimeCommand(com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand.Builder builderForValue) {
result.hasBridgeSyncTimeCommand = true;
result.bridgeSyncTimeCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeSyncTimeCommand(com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand value) {
if (result.hasBridgeSyncTimeCommand() &&
result.bridgeSyncTimeCommand_ != com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand.getDefaultInstance()) {
result.bridgeSyncTimeCommand_ =
com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand.newBuilder(result.bridgeSyncTimeCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeSyncTimeCommand_ = value;
}
result.hasBridgeSyncTimeCommand = true;
return this;
}
public Builder clearBridgeSyncTimeCommand() {
result.hasBridgeSyncTimeCommand = false;
result.bridgeSyncTimeCommand_ = com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.BridgeSyncTimeCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedCommand bridgeHeartBeatReceivedCommand = 55;
public boolean hasBridgeHeartBeatReceivedCommand() {
return result.hasBridgeHeartBeatReceivedCommand();
}
public com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand getBridgeHeartBeatReceivedCommand() {
return result.getBridgeHeartBeatReceivedCommand();
}
public Builder setBridgeHeartBeatReceivedCommand(com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeHeartBeatReceivedCommand = true;
result.bridgeHeartBeatReceivedCommand_ = value;
return this;
}
public Builder setBridgeHeartBeatReceivedCommand(com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand.Builder builderForValue) {
result.hasBridgeHeartBeatReceivedCommand = true;
result.bridgeHeartBeatReceivedCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeHeartBeatReceivedCommand(com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand value) {
if (result.hasBridgeHeartBeatReceivedCommand() &&
result.bridgeHeartBeatReceivedCommand_ != com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand.getDefaultInstance()) {
result.bridgeHeartBeatReceivedCommand_ =
com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand.newBuilder(result.bridgeHeartBeatReceivedCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeHeartBeatReceivedCommand_ = value;
}
result.hasBridgeHeartBeatReceivedCommand = true;
return this;
}
public Builder clearBridgeHeartBeatReceivedCommand() {
result.hasBridgeHeartBeatReceivedCommand = false;
result.bridgeHeartBeatReceivedCommand_ = com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.BridgeHeartBeatReceivedCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RemoveByTagCommand removeByTagCommand = 56;
public boolean hasRemoveByTagCommand() {
return result.hasRemoveByTagCommand();
}
public com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand getRemoveByTagCommand() {
return result.getRemoveByTagCommand();
}
public Builder setRemoveByTagCommand(com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRemoveByTagCommand = true;
result.removeByTagCommand_ = value;
return this;
}
public Builder setRemoveByTagCommand(com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand.Builder builderForValue) {
result.hasRemoveByTagCommand = true;
result.removeByTagCommand_ = builderForValue.build();
return this;
}
public Builder mergeRemoveByTagCommand(com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand value) {
if (result.hasRemoveByTagCommand() &&
result.removeByTagCommand_ != com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand.getDefaultInstance()) {
result.removeByTagCommand_ =
com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand.newBuilder(result.removeByTagCommand_).mergeFrom(value).buildPartial();
} else {
result.removeByTagCommand_ = value;
}
result.hasRemoveByTagCommand = true;
return this;
}
public Builder clearRemoveByTagCommand() {
result.hasRemoveByTagCommand = false;
result.removeByTagCommand_ = com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.RemoveByTagCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommand bridgeMakeTargetCacheActivePassiveCommand = 57;
public boolean hasBridgeMakeTargetCacheActivePassiveCommand() {
return result.hasBridgeMakeTargetCacheActivePassiveCommand();
}
public com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand getBridgeMakeTargetCacheActivePassiveCommand() {
return result.getBridgeMakeTargetCacheActivePassiveCommand();
}
public Builder setBridgeMakeTargetCacheActivePassiveCommand(com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeMakeTargetCacheActivePassiveCommand = true;
result.bridgeMakeTargetCacheActivePassiveCommand_ = value;
return this;
}
public Builder setBridgeMakeTargetCacheActivePassiveCommand(com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand.Builder builderForValue) {
result.hasBridgeMakeTargetCacheActivePassiveCommand = true;
result.bridgeMakeTargetCacheActivePassiveCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeMakeTargetCacheActivePassiveCommand(com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand value) {
if (result.hasBridgeMakeTargetCacheActivePassiveCommand() &&
result.bridgeMakeTargetCacheActivePassiveCommand_ != com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand.getDefaultInstance()) {
result.bridgeMakeTargetCacheActivePassiveCommand_ =
com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand.newBuilder(result.bridgeMakeTargetCacheActivePassiveCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeMakeTargetCacheActivePassiveCommand_ = value;
}
result.hasBridgeMakeTargetCacheActivePassiveCommand = true;
return this;
}
public Builder clearBridgeMakeTargetCacheActivePassiveCommand() {
result.hasBridgeMakeTargetCacheActivePassiveCommand = false;
result.bridgeMakeTargetCacheActivePassiveCommand_ = com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.BridgeMakeTargetCacheActivePassiveCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.UnRegisterCQCommand unRegisterCQCommand = 58;
public boolean hasUnRegisterCQCommand() {
return result.hasUnRegisterCQCommand();
}
public com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand getUnRegisterCQCommand() {
return result.getUnRegisterCQCommand();
}
public Builder setUnRegisterCQCommand(com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasUnRegisterCQCommand = true;
result.unRegisterCQCommand_ = value;
return this;
}
public Builder setUnRegisterCQCommand(com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand.Builder builderForValue) {
result.hasUnRegisterCQCommand = true;
result.unRegisterCQCommand_ = builderForValue.build();
return this;
}
public Builder mergeUnRegisterCQCommand(com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand value) {
if (result.hasUnRegisterCQCommand() &&
result.unRegisterCQCommand_ != com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand.getDefaultInstance()) {
result.unRegisterCQCommand_ =
com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand.newBuilder(result.unRegisterCQCommand_).mergeFrom(value).buildPartial();
} else {
result.unRegisterCQCommand_ = value;
}
result.hasUnRegisterCQCommand = true;
return this;
}
public Builder clearUnRegisterCQCommand() {
result.hasUnRegisterCQCommand = false;
result.unRegisterCQCommand_ = com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.UnRegisterCQCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SearchCQCommand searchCQCommand = 59;
public boolean hasSearchCQCommand() {
return result.hasSearchCQCommand();
}
public com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand getSearchCQCommand() {
return result.getSearchCQCommand();
}
public Builder setSearchCQCommand(com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSearchCQCommand = true;
result.searchCQCommand_ = value;
return this;
}
public Builder setSearchCQCommand(com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand.Builder builderForValue) {
result.hasSearchCQCommand = true;
result.searchCQCommand_ = builderForValue.build();
return this;
}
public Builder mergeSearchCQCommand(com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand value) {
if (result.hasSearchCQCommand() &&
result.searchCQCommand_ != com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand.getDefaultInstance()) {
result.searchCQCommand_ =
com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand.newBuilder(result.searchCQCommand_).mergeFrom(value).buildPartial();
} else {
result.searchCQCommand_ = value;
}
result.hasSearchCQCommand = true;
return this;
}
public Builder clearSearchCQCommand() {
result.hasSearchCQCommand = false;
result.searchCQCommand_ = com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RegisterCQCommand registerCQCommand = 60;
public boolean hasRegisterCQCommand() {
return result.hasRegisterCQCommand();
}
public com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand getRegisterCQCommand() {
return result.getRegisterCQCommand();
}
public Builder setRegisterCQCommand(com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRegisterCQCommand = true;
result.registerCQCommand_ = value;
return this;
}
public Builder setRegisterCQCommand(com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand.Builder builderForValue) {
result.hasRegisterCQCommand = true;
result.registerCQCommand_ = builderForValue.build();
return this;
}
public Builder mergeRegisterCQCommand(com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand value) {
if (result.hasRegisterCQCommand() &&
result.registerCQCommand_ != com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand.getDefaultInstance()) {
result.registerCQCommand_ =
com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand.newBuilder(result.registerCQCommand_).mergeFrom(value).buildPartial();
} else {
result.registerCQCommand_ = value;
}
result.hasRegisterCQCommand = true;
return this;
}
public Builder clearRegisterCQCommand() {
result.hasRegisterCQCommand = false;
result.registerCQCommand_ = com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.RegisterCQCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetKeysByTagCommand getKeysByTagCommand = 61;
public boolean hasGetKeysByTagCommand() {
return result.hasGetKeysByTagCommand();
}
public com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand getGetKeysByTagCommand() {
return result.getGetKeysByTagCommand();
}
public Builder setGetKeysByTagCommand(com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetKeysByTagCommand = true;
result.getKeysByTagCommand_ = value;
return this;
}
public Builder setGetKeysByTagCommand(com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand.Builder builderForValue) {
result.hasGetKeysByTagCommand = true;
result.getKeysByTagCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetKeysByTagCommand(com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand value) {
if (result.hasGetKeysByTagCommand() &&
result.getKeysByTagCommand_ != com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand.getDefaultInstance()) {
result.getKeysByTagCommand_ =
com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand.newBuilder(result.getKeysByTagCommand_).mergeFrom(value).buildPartial();
} else {
result.getKeysByTagCommand_ = value;
}
result.hasGetKeysByTagCommand = true;
return this;
}
public Builder clearGetKeysByTagCommand() {
result.hasGetKeysByTagCommand = false;
result.getKeysByTagCommand_ = com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.GetKeysByTagCommand.getDefaultInstance();
return this;
}
// optional int64 clientLastViewId = 62 [default = -1];
public boolean hasClientLastViewId() {
return result.hasClientLastViewId();
}
public long getClientLastViewId() {
return result.getClientLastViewId();
}
public Builder setClientLastViewId(long value) {
result.hasClientLastViewId = true;
result.clientLastViewId_ = value;
return this;
}
public Builder clearClientLastViewId() {
result.hasClientLastViewId = false;
result.clientLastViewId_ = -1L;
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BulkDeleteCommand bulkDeleteCommand = 63;
public boolean hasBulkDeleteCommand() {
return result.hasBulkDeleteCommand();
}
public com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand getBulkDeleteCommand() {
return result.getBulkDeleteCommand();
}
public Builder setBulkDeleteCommand(com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBulkDeleteCommand = true;
result.bulkDeleteCommand_ = value;
return this;
}
public Builder setBulkDeleteCommand(com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand.Builder builderForValue) {
result.hasBulkDeleteCommand = true;
result.bulkDeleteCommand_ = builderForValue.build();
return this;
}
public Builder mergeBulkDeleteCommand(com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand value) {
if (result.hasBulkDeleteCommand() &&
result.bulkDeleteCommand_ != com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand.getDefaultInstance()) {
result.bulkDeleteCommand_ =
com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand.newBuilder(result.bulkDeleteCommand_).mergeFrom(value).buildPartial();
} else {
result.bulkDeleteCommand_ = value;
}
result.hasBulkDeleteCommand = true;
return this;
}
public Builder clearBulkDeleteCommand() {
result.hasBulkDeleteCommand = false;
result.bulkDeleteCommand_ = com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.BulkDeleteCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.DeleteCommand deleteCommand = 64;
public boolean hasDeleteCommand() {
return result.hasDeleteCommand();
}
public com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand getDeleteCommand() {
return result.getDeleteCommand();
}
public Builder setDeleteCommand(com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDeleteCommand = true;
result.deleteCommand_ = value;
return this;
}
public Builder setDeleteCommand(com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand.Builder builderForValue) {
result.hasDeleteCommand = true;
result.deleteCommand_ = builderForValue.build();
return this;
}
public Builder mergeDeleteCommand(com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand value) {
if (result.hasDeleteCommand() &&
result.deleteCommand_ != com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand.getDefaultInstance()) {
result.deleteCommand_ =
com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand.newBuilder(result.deleteCommand_).mergeFrom(value).buildPartial();
} else {
result.deleteCommand_ = value;
}
result.hasDeleteCommand = true;
return this;
}
public Builder clearDeleteCommand() {
result.hasDeleteCommand = false;
result.deleteCommand_ = com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.DeleteCommand.getDefaultInstance();
return this;
}
// optional string intendedRecipient = 65 [default = ""];
public boolean hasIntendedRecipient() {
return result.hasIntendedRecipient();
}
public java.lang.String getIntendedRecipient() {
return result.getIntendedRecipient();
}
public Builder setIntendedRecipient(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasIntendedRecipient = true;
result.intendedRecipient_ = value;
return this;
}
public Builder clearIntendedRecipient() {
result.hasIntendedRecipient = false;
result.intendedRecipient_ = getDefaultInstance().getIntendedRecipient();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetNextChunkCommand getNextChunkCommand = 66;
public boolean hasGetNextChunkCommand() {
return result.hasGetNextChunkCommand();
}
public com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand getGetNextChunkCommand() {
return result.getGetNextChunkCommand();
}
public Builder setGetNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetNextChunkCommand = true;
result.getNextChunkCommand_ = value;
return this;
}
public Builder setGetNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand.Builder builderForValue) {
result.hasGetNextChunkCommand = true;
result.getNextChunkCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand value) {
if (result.hasGetNextChunkCommand() &&
result.getNextChunkCommand_ != com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand.getDefaultInstance()) {
result.getNextChunkCommand_ =
com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand.newBuilder(result.getNextChunkCommand_).mergeFrom(value).buildPartial();
} else {
result.getNextChunkCommand_ = value;
}
result.hasGetNextChunkCommand = true;
return this;
}
public Builder clearGetNextChunkCommand() {
result.hasGetNextChunkCommand = false;
result.getNextChunkCommand_ = com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.GetNextChunkCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommand getGroupNextChunkCommand = 67;
public boolean hasGetGroupNextChunkCommand() {
return result.hasGetGroupNextChunkCommand();
}
public com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand getGetGroupNextChunkCommand() {
return result.getGetGroupNextChunkCommand();
}
public Builder setGetGroupNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetGroupNextChunkCommand = true;
result.getGroupNextChunkCommand_ = value;
return this;
}
public Builder setGetGroupNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand.Builder builderForValue) {
result.hasGetGroupNextChunkCommand = true;
result.getGroupNextChunkCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetGroupNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand value) {
if (result.hasGetGroupNextChunkCommand() &&
result.getGroupNextChunkCommand_ != com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand.getDefaultInstance()) {
result.getGroupNextChunkCommand_ =
com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand.newBuilder(result.getGroupNextChunkCommand_).mergeFrom(value).buildPartial();
} else {
result.getGroupNextChunkCommand_ = value;
}
result.hasGetGroupNextChunkCommand = true;
return this;
}
public Builder clearGetGroupNextChunkCommand() {
result.hasGetGroupNextChunkCommand = false;
result.getGroupNextChunkCommand_ = com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.GetGroupNextChunkCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.AddAttributeCommand addAttributeCommand = 68;
public boolean hasAddAttributeCommand() {
return result.hasAddAttributeCommand();
}
public com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand getAddAttributeCommand() {
return result.getAddAttributeCommand();
}
public Builder setAddAttributeCommand(com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasAddAttributeCommand = true;
result.addAttributeCommand_ = value;
return this;
}
public Builder setAddAttributeCommand(com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand.Builder builderForValue) {
result.hasAddAttributeCommand = true;
result.addAttributeCommand_ = builderForValue.build();
return this;
}
public Builder mergeAddAttributeCommand(com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand value) {
if (result.hasAddAttributeCommand() &&
result.addAttributeCommand_ != com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand.getDefaultInstance()) {
result.addAttributeCommand_ =
com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand.newBuilder(result.addAttributeCommand_).mergeFrom(value).buildPartial();
} else {
result.addAttributeCommand_ = value;
}
result.hasAddAttributeCommand = true;
return this;
}
public Builder clearAddAttributeCommand() {
result.hasAddAttributeCommand = false;
result.addAttributeCommand_ = com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.AddAttributeCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetEncryptionCommand getEncryptionCommand = 69;
public boolean hasGetEncryptionCommand() {
return result.hasGetEncryptionCommand();
}
public com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand getGetEncryptionCommand() {
return result.getGetEncryptionCommand();
}
public Builder setGetEncryptionCommand(com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetEncryptionCommand = true;
result.getEncryptionCommand_ = value;
return this;
}
public Builder setGetEncryptionCommand(com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand.Builder builderForValue) {
result.hasGetEncryptionCommand = true;
result.getEncryptionCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetEncryptionCommand(com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand value) {
if (result.hasGetEncryptionCommand() &&
result.getEncryptionCommand_ != com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand.getDefaultInstance()) {
result.getEncryptionCommand_ =
com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand.newBuilder(result.getEncryptionCommand_).mergeFrom(value).buildPartial();
} else {
result.getEncryptionCommand_ = value;
}
result.hasGetEncryptionCommand = true;
return this;
}
public Builder clearGetEncryptionCommand() {
result.hasGetEncryptionCommand = false;
result.getEncryptionCommand_ = com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.GetEncryptionCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetRunningServersCommand getRunningServersCommand = 70;
public boolean hasGetRunningServersCommand() {
return result.hasGetRunningServersCommand();
}
public com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand getGetRunningServersCommand() {
return result.getGetRunningServersCommand();
}
public Builder setGetRunningServersCommand(com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetRunningServersCommand = true;
result.getRunningServersCommand_ = value;
return this;
}
public Builder setGetRunningServersCommand(com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand.Builder builderForValue) {
result.hasGetRunningServersCommand = true;
result.getRunningServersCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetRunningServersCommand(com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand value) {
if (result.hasGetRunningServersCommand() &&
result.getRunningServersCommand_ != com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand.getDefaultInstance()) {
result.getRunningServersCommand_ =
com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand.newBuilder(result.getRunningServersCommand_).mergeFrom(value).buildPartial();
} else {
result.getRunningServersCommand_ = value;
}
result.hasGetRunningServersCommand = true;
return this;
}
public Builder clearGetRunningServersCommand() {
result.hasGetRunningServersCommand = false;
result.getRunningServersCommand_ = com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.GetRunningServersCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SyncEventsCommand syncEventsCommand = 71;
public boolean hasSyncEventsCommand() {
return result.hasSyncEventsCommand();
}
public com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand getSyncEventsCommand() {
return result.getSyncEventsCommand();
}
public Builder setSyncEventsCommand(com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSyncEventsCommand = true;
result.syncEventsCommand_ = value;
return this;
}
public Builder setSyncEventsCommand(com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand.Builder builderForValue) {
result.hasSyncEventsCommand = true;
result.syncEventsCommand_ = builderForValue.build();
return this;
}
public Builder mergeSyncEventsCommand(com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand value) {
if (result.hasSyncEventsCommand() &&
result.syncEventsCommand_ != com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand.getDefaultInstance()) {
result.syncEventsCommand_ =
com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand.newBuilder(result.syncEventsCommand_).mergeFrom(value).buildPartial();
} else {
result.syncEventsCommand_ = value;
}
result.hasSyncEventsCommand = true;
return this;
}
public Builder clearSyncEventsCommand() {
result.hasSyncEventsCommand = false;
result.syncEventsCommand_ = com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.SyncEventsCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.DeleteQueryCommand deleteQueryCommand = 72;
public boolean hasDeleteQueryCommand() {
return result.hasDeleteQueryCommand();
}
public com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand getDeleteQueryCommand() {
return result.getDeleteQueryCommand();
}
public Builder setDeleteQueryCommand(com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDeleteQueryCommand = true;
result.deleteQueryCommand_ = value;
return this;
}
public Builder setDeleteQueryCommand(com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand.Builder builderForValue) {
result.hasDeleteQueryCommand = true;
result.deleteQueryCommand_ = builderForValue.build();
return this;
}
public Builder mergeDeleteQueryCommand(com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand value) {
if (result.hasDeleteQueryCommand() &&
result.deleteQueryCommand_ != com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand.getDefaultInstance()) {
result.deleteQueryCommand_ =
com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand.newBuilder(result.deleteQueryCommand_).mergeFrom(value).buildPartial();
} else {
result.deleteQueryCommand_ = value;
}
result.hasDeleteQueryCommand = true;
return this;
}
public Builder clearDeleteQueryCommand() {
result.hasDeleteQueryCommand = false;
result.deleteQueryCommand_ = com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetProductVersionCommand getProductVersionCommand = 73;
public boolean hasGetProductVersionCommand() {
return result.hasGetProductVersionCommand();
}
public com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand getGetProductVersionCommand() {
return result.getGetProductVersionCommand();
}
public Builder setGetProductVersionCommand(com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetProductVersionCommand = true;
result.getProductVersionCommand_ = value;
return this;
}
public Builder setGetProductVersionCommand(com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand.Builder builderForValue) {
result.hasGetProductVersionCommand = true;
result.getProductVersionCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetProductVersionCommand(com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand value) {
if (result.hasGetProductVersionCommand() &&
result.getProductVersionCommand_ != com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand.getDefaultInstance()) {
result.getProductVersionCommand_ =
com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand.newBuilder(result.getProductVersionCommand_).mergeFrom(value).buildPartial();
} else {
result.getProductVersionCommand_ = value;
}
result.hasGetProductVersionCommand = true;
return this;
}
public Builder clearGetProductVersionCommand() {
result.hasGetProductVersionCommand = false;
result.getProductVersionCommand_ = com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.GetProductVersionCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommand bridgeGetReplicatorStatusInfoCommand = 74;
public boolean hasBridgeGetReplicatorStatusInfoCommand() {
return result.hasBridgeGetReplicatorStatusInfoCommand();
}
public com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand getBridgeGetReplicatorStatusInfoCommand() {
return result.getBridgeGetReplicatorStatusInfoCommand();
}
public Builder setBridgeGetReplicatorStatusInfoCommand(com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeGetReplicatorStatusInfoCommand = true;
result.bridgeGetReplicatorStatusInfoCommand_ = value;
return this;
}
public Builder setBridgeGetReplicatorStatusInfoCommand(com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand.Builder builderForValue) {
result.hasBridgeGetReplicatorStatusInfoCommand = true;
result.bridgeGetReplicatorStatusInfoCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeGetReplicatorStatusInfoCommand(com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand value) {
if (result.hasBridgeGetReplicatorStatusInfoCommand() &&
result.bridgeGetReplicatorStatusInfoCommand_ != com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand.getDefaultInstance()) {
result.bridgeGetReplicatorStatusInfoCommand_ =
com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand.newBuilder(result.bridgeGetReplicatorStatusInfoCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeGetReplicatorStatusInfoCommand_ = value;
}
result.hasBridgeGetReplicatorStatusInfoCommand = true;
return this;
}
public Builder clearBridgeGetReplicatorStatusInfoCommand() {
result.hasBridgeGetReplicatorStatusInfoCommand = false;
result.bridgeGetReplicatorStatusInfoCommand_ = com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.GetReplicatorStatusInfoCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RequestStateTransferCommand bridgeRequestStateTransferCommand = 75;
public boolean hasBridgeRequestStateTransferCommand() {
return result.hasBridgeRequestStateTransferCommand();
}
public com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand getBridgeRequestStateTransferCommand() {
return result.getBridgeRequestStateTransferCommand();
}
public Builder setBridgeRequestStateTransferCommand(com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgeRequestStateTransferCommand = true;
result.bridgeRequestStateTransferCommand_ = value;
return this;
}
public Builder setBridgeRequestStateTransferCommand(com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand.Builder builderForValue) {
result.hasBridgeRequestStateTransferCommand = true;
result.bridgeRequestStateTransferCommand_ = builderForValue.build();
return this;
}
public Builder mergeBridgeRequestStateTransferCommand(com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand value) {
if (result.hasBridgeRequestStateTransferCommand() &&
result.bridgeRequestStateTransferCommand_ != com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand.getDefaultInstance()) {
result.bridgeRequestStateTransferCommand_ =
com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand.newBuilder(result.bridgeRequestStateTransferCommand_).mergeFrom(value).buildPartial();
} else {
result.bridgeRequestStateTransferCommand_ = value;
}
result.hasBridgeRequestStateTransferCommand = true;
return this;
}
public Builder clearBridgeRequestStateTransferCommand() {
result.hasBridgeRequestStateTransferCommand = false;
result.bridgeRequestStateTransferCommand_ = com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.RequestStateTransferCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetServerMappingCommand getServerMappingCommand = 76;
public boolean hasGetServerMappingCommand() {
return result.hasGetServerMappingCommand();
}
public com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand getGetServerMappingCommand() {
return result.getGetServerMappingCommand();
}
public Builder setGetServerMappingCommand(com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetServerMappingCommand = true;
result.getServerMappingCommand_ = value;
return this;
}
public Builder setGetServerMappingCommand(com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand.Builder builderForValue) {
result.hasGetServerMappingCommand = true;
result.getServerMappingCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetServerMappingCommand(com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand value) {
if (result.hasGetServerMappingCommand() &&
result.getServerMappingCommand_ != com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand.getDefaultInstance()) {
result.getServerMappingCommand_ =
com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand.newBuilder(result.getServerMappingCommand_).mergeFrom(value).buildPartial();
} else {
result.getServerMappingCommand_ = value;
}
result.hasGetServerMappingCommand = true;
return this;
}
public Builder clearGetServerMappingCommand() {
result.hasGetServerMappingCommand = false;
result.getServerMappingCommand_ = com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.GetServerMappingCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.InquiryRequestCommand inquiryRequestCommand = 77;
public boolean hasInquiryRequestCommand() {
return result.hasInquiryRequestCommand();
}
public com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand getInquiryRequestCommand() {
return result.getInquiryRequestCommand();
}
public Builder setInquiryRequestCommand(com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasInquiryRequestCommand = true;
result.inquiryRequestCommand_ = value;
return this;
}
public Builder setInquiryRequestCommand(com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand.Builder builderForValue) {
result.hasInquiryRequestCommand = true;
result.inquiryRequestCommand_ = builderForValue.build();
return this;
}
public Builder mergeInquiryRequestCommand(com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand value) {
if (result.hasInquiryRequestCommand() &&
result.inquiryRequestCommand_ != com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand.getDefaultInstance()) {
result.inquiryRequestCommand_ =
com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand.newBuilder(result.inquiryRequestCommand_).mergeFrom(value).buildPartial();
} else {
result.inquiryRequestCommand_ = value;
}
result.hasInquiryRequestCommand = true;
return this;
}
public Builder clearInquiryRequestCommand() {
result.hasInquiryRequestCommand = false;
result.inquiryRequestCommand_ = com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.InquiryRequestCommand.getDefaultInstance();
return this;
}
// optional bool isRetryCommand = 78 [default = false];
public boolean hasIsRetryCommand() {
return result.hasIsRetryCommand();
}
public boolean getIsRetryCommand() {
return result.getIsRetryCommand();
}
public Builder setIsRetryCommand(boolean value) {
result.hasIsRetryCommand = true;
result.isRetryCommand_ = value;
return this;
}
public Builder clearIsRetryCommand() {
result.hasIsRetryCommand = false;
result.isRetryCommand_ = false;
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.MapReduceTaskCommand mapReduceTaskCommand = 79;
public boolean hasMapReduceTaskCommand() {
return result.hasMapReduceTaskCommand();
}
public com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand getMapReduceTaskCommand() {
return result.getMapReduceTaskCommand();
}
public Builder setMapReduceTaskCommand(com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasMapReduceTaskCommand = true;
result.mapReduceTaskCommand_ = value;
return this;
}
public Builder setMapReduceTaskCommand(com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand.Builder builderForValue) {
result.hasMapReduceTaskCommand = true;
result.mapReduceTaskCommand_ = builderForValue.build();
return this;
}
public Builder mergeMapReduceTaskCommand(com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand value) {
if (result.hasMapReduceTaskCommand() &&
result.mapReduceTaskCommand_ != com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand.getDefaultInstance()) {
result.mapReduceTaskCommand_ =
com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand.newBuilder(result.mapReduceTaskCommand_).mergeFrom(value).buildPartial();
} else {
result.mapReduceTaskCommand_ = value;
}
result.hasMapReduceTaskCommand = true;
return this;
}
public Builder clearMapReduceTaskCommand() {
result.hasMapReduceTaskCommand = false;
result.mapReduceTaskCommand_ = com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.MapReduceTaskCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.TaskCallbackCommand TaskCallbackCommand = 80;
public boolean hasTaskCallbackCommand() {
return result.hasTaskCallbackCommand();
}
public com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand getTaskCallbackCommand() {
return result.getTaskCallbackCommand();
}
public Builder setTaskCallbackCommand(com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTaskCallbackCommand = true;
result.taskCallbackCommand_ = value;
return this;
}
public Builder setTaskCallbackCommand(com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand.Builder builderForValue) {
result.hasTaskCallbackCommand = true;
result.taskCallbackCommand_ = builderForValue.build();
return this;
}
public Builder mergeTaskCallbackCommand(com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand value) {
if (result.hasTaskCallbackCommand() &&
result.taskCallbackCommand_ != com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand.getDefaultInstance()) {
result.taskCallbackCommand_ =
com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand.newBuilder(result.taskCallbackCommand_).mergeFrom(value).buildPartial();
} else {
result.taskCallbackCommand_ = value;
}
result.hasTaskCallbackCommand = true;
return this;
}
public Builder clearTaskCallbackCommand() {
result.hasTaskCallbackCommand = false;
result.taskCallbackCommand_ = com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.TaskCallbackCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetRunningTasksCommand RunningTasksCommand = 81;
public boolean hasRunningTasksCommand() {
return result.hasRunningTasksCommand();
}
public com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand getRunningTasksCommand() {
return result.getRunningTasksCommand();
}
public Builder setRunningTasksCommand(com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRunningTasksCommand = true;
result.runningTasksCommand_ = value;
return this;
}
public Builder setRunningTasksCommand(com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand.Builder builderForValue) {
result.hasRunningTasksCommand = true;
result.runningTasksCommand_ = builderForValue.build();
return this;
}
public Builder mergeRunningTasksCommand(com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand value) {
if (result.hasRunningTasksCommand() &&
result.runningTasksCommand_ != com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand.getDefaultInstance()) {
result.runningTasksCommand_ =
com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand.newBuilder(result.runningTasksCommand_).mergeFrom(value).buildPartial();
} else {
result.runningTasksCommand_ = value;
}
result.hasRunningTasksCommand = true;
return this;
}
public Builder clearRunningTasksCommand() {
result.hasRunningTasksCommand = false;
result.runningTasksCommand_ = com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.GetRunningTasksCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.TaskCancelCommand TaskCancelCommand = 82;
public boolean hasTaskCancelCommand() {
return result.hasTaskCancelCommand();
}
public com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand getTaskCancelCommand() {
return result.getTaskCancelCommand();
}
public Builder setTaskCancelCommand(com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTaskCancelCommand = true;
result.taskCancelCommand_ = value;
return this;
}
public Builder setTaskCancelCommand(com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand.Builder builderForValue) {
result.hasTaskCancelCommand = true;
result.taskCancelCommand_ = builderForValue.build();
return this;
}
public Builder mergeTaskCancelCommand(com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand value) {
if (result.hasTaskCancelCommand() &&
result.taskCancelCommand_ != com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand.getDefaultInstance()) {
result.taskCancelCommand_ =
com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand.newBuilder(result.taskCancelCommand_).mergeFrom(value).buildPartial();
} else {
result.taskCancelCommand_ = value;
}
result.hasTaskCancelCommand = true;
return this;
}
public Builder clearTaskCancelCommand() {
result.hasTaskCancelCommand = false;
result.taskCancelCommand_ = com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.TaskCancelCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.TaskProgressCommand TaskProgressCommand = 83;
public boolean hasTaskProgressCommand() {
return result.hasTaskProgressCommand();
}
public com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand getTaskProgressCommand() {
return result.getTaskProgressCommand();
}
public Builder setTaskProgressCommand(com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTaskProgressCommand = true;
result.taskProgressCommand_ = value;
return this;
}
public Builder setTaskProgressCommand(com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand.Builder builderForValue) {
result.hasTaskProgressCommand = true;
result.taskProgressCommand_ = builderForValue.build();
return this;
}
public Builder mergeTaskProgressCommand(com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand value) {
if (result.hasTaskProgressCommand() &&
result.taskProgressCommand_ != com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand.getDefaultInstance()) {
result.taskProgressCommand_ =
com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand.newBuilder(result.taskProgressCommand_).mergeFrom(value).buildPartial();
} else {
result.taskProgressCommand_ = value;
}
result.hasTaskProgressCommand = true;
return this;
}
public Builder clearTaskProgressCommand() {
result.hasTaskProgressCommand = false;
result.taskProgressCommand_ = com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.TaskProgressCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetNextRecordCommand NextRecordCommand = 84;
public boolean hasNextRecordCommand() {
return result.hasNextRecordCommand();
}
public com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand getNextRecordCommand() {
return result.getNextRecordCommand();
}
public Builder setNextRecordCommand(com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasNextRecordCommand = true;
result.nextRecordCommand_ = value;
return this;
}
public Builder setNextRecordCommand(com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand.Builder builderForValue) {
result.hasNextRecordCommand = true;
result.nextRecordCommand_ = builderForValue.build();
return this;
}
public Builder mergeNextRecordCommand(com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand value) {
if (result.hasNextRecordCommand() &&
result.nextRecordCommand_ != com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand.getDefaultInstance()) {
result.nextRecordCommand_ =
com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand.newBuilder(result.nextRecordCommand_).mergeFrom(value).buildPartial();
} else {
result.nextRecordCommand_ = value;
}
result.hasNextRecordCommand = true;
return this;
}
public Builder clearNextRecordCommand() {
result.hasNextRecordCommand = false;
result.nextRecordCommand_ = com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.GetNextRecordCommand.getDefaultInstance();
return this;
}
// optional int32 commandID = 85 [default = -1];
public boolean hasCommandID() {
return result.hasCommandID();
}
public int getCommandID() {
return result.getCommandID();
}
public Builder setCommandID(int value) {
result.hasCommandID = true;
result.commandID_ = value;
return this;
}
public Builder clearCommandID() {
result.hasCommandID = false;
result.commandID_ = -1;
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetCacheBindingCommand getCacheBindingCommand = 86;
public boolean hasGetCacheBindingCommand() {
return result.hasGetCacheBindingCommand();
}
public com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand getGetCacheBindingCommand() {
return result.getGetCacheBindingCommand();
}
public Builder setGetCacheBindingCommand(com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetCacheBindingCommand = true;
result.getCacheBindingCommand_ = value;
return this;
}
public Builder setGetCacheBindingCommand(com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand.Builder builderForValue) {
result.hasGetCacheBindingCommand = true;
result.getCacheBindingCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetCacheBindingCommand(com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand value) {
if (result.hasGetCacheBindingCommand() &&
result.getCacheBindingCommand_ != com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand.getDefaultInstance()) {
result.getCacheBindingCommand_ =
com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand.newBuilder(result.getCacheBindingCommand_).mergeFrom(value).buildPartial();
} else {
result.getCacheBindingCommand_ = value;
}
result.hasGetCacheBindingCommand = true;
return this;
}
public Builder clearGetCacheBindingCommand() {
result.hasGetCacheBindingCommand = false;
result.getCacheBindingCommand_ = com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.GetCacheBindingCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommand TaskEnumeratorCommand = 87;
public boolean hasTaskEnumeratorCommand() {
return result.hasTaskEnumeratorCommand();
}
public com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand getTaskEnumeratorCommand() {
return result.getTaskEnumeratorCommand();
}
public Builder setTaskEnumeratorCommand(com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTaskEnumeratorCommand = true;
result.taskEnumeratorCommand_ = value;
return this;
}
public Builder setTaskEnumeratorCommand(com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand.Builder builderForValue) {
result.hasTaskEnumeratorCommand = true;
result.taskEnumeratorCommand_ = builderForValue.build();
return this;
}
public Builder mergeTaskEnumeratorCommand(com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand value) {
if (result.hasTaskEnumeratorCommand() &&
result.taskEnumeratorCommand_ != com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand.getDefaultInstance()) {
result.taskEnumeratorCommand_ =
com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand.newBuilder(result.taskEnumeratorCommand_).mergeFrom(value).buildPartial();
} else {
result.taskEnumeratorCommand_ = value;
}
result.hasTaskEnumeratorCommand = true;
return this;
}
public Builder clearTaskEnumeratorCommand() {
result.hasTaskEnumeratorCommand = false;
result.taskEnumeratorCommand_ = com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.TaskEnumeratorCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorCommand invokeEntryProcessorCommand = 88;
public boolean hasInvokeEntryProcessorCommand() {
return result.hasInvokeEntryProcessorCommand();
}
public com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand getInvokeEntryProcessorCommand() {
return result.getInvokeEntryProcessorCommand();
}
public Builder setInvokeEntryProcessorCommand(com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasInvokeEntryProcessorCommand = true;
result.invokeEntryProcessorCommand_ = value;
return this;
}
public Builder setInvokeEntryProcessorCommand(com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand.Builder builderForValue) {
result.hasInvokeEntryProcessorCommand = true;
result.invokeEntryProcessorCommand_ = builderForValue.build();
return this;
}
public Builder mergeInvokeEntryProcessorCommand(com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand value) {
if (result.hasInvokeEntryProcessorCommand() &&
result.invokeEntryProcessorCommand_ != com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand.getDefaultInstance()) {
result.invokeEntryProcessorCommand_ =
com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand.newBuilder(result.invokeEntryProcessorCommand_).mergeFrom(value).buildPartial();
} else {
result.invokeEntryProcessorCommand_ = value;
}
result.hasInvokeEntryProcessorCommand = true;
return this;
}
public Builder clearInvokeEntryProcessorCommand() {
result.hasInvokeEntryProcessorCommand = false;
result.invokeEntryProcessorCommand_ = com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.InvokeEntryProcessorCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.ExecuteReaderCommand executeReaderCommand = 89;
public boolean hasExecuteReaderCommand() {
return result.hasExecuteReaderCommand();
}
public com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand getExecuteReaderCommand() {
return result.getExecuteReaderCommand();
}
public Builder setExecuteReaderCommand(com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasExecuteReaderCommand = true;
result.executeReaderCommand_ = value;
return this;
}
public Builder setExecuteReaderCommand(com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand.Builder builderForValue) {
result.hasExecuteReaderCommand = true;
result.executeReaderCommand_ = builderForValue.build();
return this;
}
public Builder mergeExecuteReaderCommand(com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand value) {
if (result.hasExecuteReaderCommand() &&
result.executeReaderCommand_ != com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand.getDefaultInstance()) {
result.executeReaderCommand_ =
com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand.newBuilder(result.executeReaderCommand_).mergeFrom(value).buildPartial();
} else {
result.executeReaderCommand_ = value;
}
result.hasExecuteReaderCommand = true;
return this;
}
public Builder clearExecuteReaderCommand() {
result.hasExecuteReaderCommand = false;
result.executeReaderCommand_ = com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.ExecuteReaderCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommand getReaderNextChunkCommand = 90;
public boolean hasGetReaderNextChunkCommand() {
return result.hasGetReaderNextChunkCommand();
}
public com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand getGetReaderNextChunkCommand() {
return result.getGetReaderNextChunkCommand();
}
public Builder setGetReaderNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetReaderNextChunkCommand = true;
result.getReaderNextChunkCommand_ = value;
return this;
}
public Builder setGetReaderNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand.Builder builderForValue) {
result.hasGetReaderNextChunkCommand = true;
result.getReaderNextChunkCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetReaderNextChunkCommand(com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand value) {
if (result.hasGetReaderNextChunkCommand() &&
result.getReaderNextChunkCommand_ != com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand.getDefaultInstance()) {
result.getReaderNextChunkCommand_ =
com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand.newBuilder(result.getReaderNextChunkCommand_).mergeFrom(value).buildPartial();
} else {
result.getReaderNextChunkCommand_ = value;
}
result.hasGetReaderNextChunkCommand = true;
return this;
}
public Builder clearGetReaderNextChunkCommand() {
result.hasGetReaderNextChunkCommand = false;
result.getReaderNextChunkCommand_ = com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.GetReaderNextChunkCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.DisposeReaderCommand disposeReaderCommand = 91;
public boolean hasDisposeReaderCommand() {
return result.hasDisposeReaderCommand();
}
public com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand getDisposeReaderCommand() {
return result.getDisposeReaderCommand();
}
public Builder setDisposeReaderCommand(com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDisposeReaderCommand = true;
result.disposeReaderCommand_ = value;
return this;
}
public Builder setDisposeReaderCommand(com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand.Builder builderForValue) {
result.hasDisposeReaderCommand = true;
result.disposeReaderCommand_ = builderForValue.build();
return this;
}
public Builder mergeDisposeReaderCommand(com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand value) {
if (result.hasDisposeReaderCommand() &&
result.disposeReaderCommand_ != com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand.getDefaultInstance()) {
result.disposeReaderCommand_ =
com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand.newBuilder(result.disposeReaderCommand_).mergeFrom(value).buildPartial();
} else {
result.disposeReaderCommand_ = value;
}
result.hasDisposeReaderCommand = true;
return this;
}
public Builder clearDisposeReaderCommand() {
result.hasDisposeReaderCommand = false;
result.disposeReaderCommand_ = com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.DisposeReaderCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommand executeReaderCQCommand = 92;
public boolean hasExecuteReaderCQCommand() {
return result.hasExecuteReaderCQCommand();
}
public com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand getExecuteReaderCQCommand() {
return result.getExecuteReaderCQCommand();
}
public Builder setExecuteReaderCQCommand(com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasExecuteReaderCQCommand = true;
result.executeReaderCQCommand_ = value;
return this;
}
public Builder setExecuteReaderCQCommand(com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand.Builder builderForValue) {
result.hasExecuteReaderCQCommand = true;
result.executeReaderCQCommand_ = builderForValue.build();
return this;
}
public Builder mergeExecuteReaderCQCommand(com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand value) {
if (result.hasExecuteReaderCQCommand() &&
result.executeReaderCQCommand_ != com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand.getDefaultInstance()) {
result.executeReaderCQCommand_ =
com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand.newBuilder(result.executeReaderCQCommand_).mergeFrom(value).buildPartial();
} else {
result.executeReaderCQCommand_ = value;
}
result.hasExecuteReaderCQCommand = true;
return this;
}
public Builder clearExecuteReaderCQCommand() {
result.hasExecuteReaderCQCommand = false;
result.executeReaderCQCommand_ = com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.ExecuteReaderCQCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetExpirationCommand getExpirationCommand = 93;
public boolean hasGetExpirationCommand() {
return result.hasGetExpirationCommand();
}
public com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand getGetExpirationCommand() {
return result.getGetExpirationCommand();
}
public Builder setGetExpirationCommand(com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetExpirationCommand = true;
result.getExpirationCommand_ = value;
return this;
}
public Builder setGetExpirationCommand(com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand.Builder builderForValue) {
result.hasGetExpirationCommand = true;
result.getExpirationCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetExpirationCommand(com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand value) {
if (result.hasGetExpirationCommand() &&
result.getExpirationCommand_ != com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand.getDefaultInstance()) {
result.getExpirationCommand_ =
com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand.newBuilder(result.getExpirationCommand_).mergeFrom(value).buildPartial();
} else {
result.getExpirationCommand_ = value;
}
result.hasGetExpirationCommand = true;
return this;
}
public Builder clearGetExpirationCommand() {
result.hasGetExpirationCommand = false;
result.getExpirationCommand_ = com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.GetExpirationCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetLCCommand getLCCommand = 94;
public boolean hasGetLCCommand() {
return result.hasGetLCCommand();
}
public com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand getGetLCCommand() {
return result.getGetLCCommand();
}
public Builder setGetLCCommand(com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetLCCommand = true;
result.getLCCommand_ = value;
return this;
}
public Builder setGetLCCommand(com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand.Builder builderForValue) {
result.hasGetLCCommand = true;
result.getLCCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetLCCommand(com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand value) {
if (result.hasGetLCCommand() &&
result.getLCCommand_ != com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand.getDefaultInstance()) {
result.getLCCommand_ =
com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand.newBuilder(result.getLCCommand_).mergeFrom(value).buildPartial();
} else {
result.getLCCommand_ = value;
}
result.hasGetLCCommand = true;
return this;
}
public Builder clearGetLCCommand() {
result.hasGetLCCommand = false;
result.getLCCommand_ = com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.GetLCCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommand cacheSecurityAuthorizationCommand = 95;
public boolean hasCacheSecurityAuthorizationCommand() {
return result.hasCacheSecurityAuthorizationCommand();
}
public com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand getCacheSecurityAuthorizationCommand() {
return result.getCacheSecurityAuthorizationCommand();
}
public Builder setCacheSecurityAuthorizationCommand(com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasCacheSecurityAuthorizationCommand = true;
result.cacheSecurityAuthorizationCommand_ = value;
return this;
}
public Builder setCacheSecurityAuthorizationCommand(com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand.Builder builderForValue) {
result.hasCacheSecurityAuthorizationCommand = true;
result.cacheSecurityAuthorizationCommand_ = builderForValue.build();
return this;
}
public Builder mergeCacheSecurityAuthorizationCommand(com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand value) {
if (result.hasCacheSecurityAuthorizationCommand() &&
result.cacheSecurityAuthorizationCommand_ != com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand.getDefaultInstance()) {
result.cacheSecurityAuthorizationCommand_ =
com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand.newBuilder(result.cacheSecurityAuthorizationCommand_).mergeFrom(value).buildPartial();
} else {
result.cacheSecurityAuthorizationCommand_ = value;
}
result.hasCacheSecurityAuthorizationCommand = true;
return this;
}
public Builder clearCacheSecurityAuthorizationCommand() {
result.hasCacheSecurityAuthorizationCommand = false;
result.cacheSecurityAuthorizationCommand_ = com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.CacheSecurityAuthorizationCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.PollCommand pollCommand = 96;
public boolean hasPollCommand() {
return result.hasPollCommand();
}
public com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand getPollCommand() {
return result.getPollCommand();
}
public Builder setPollCommand(com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasPollCommand = true;
result.pollCommand_ = value;
return this;
}
public Builder setPollCommand(com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand.Builder builderForValue) {
result.hasPollCommand = true;
result.pollCommand_ = builderForValue.build();
return this;
}
public Builder mergePollCommand(com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand value) {
if (result.hasPollCommand() &&
result.pollCommand_ != com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand.getDefaultInstance()) {
result.pollCommand_ =
com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand.newBuilder(result.pollCommand_).mergeFrom(value).buildPartial();
} else {
result.pollCommand_ = value;
}
result.hasPollCommand = true;
return this;
}
public Builder clearPollCommand() {
result.hasPollCommand = false;
result.pollCommand_ = com.alachisoft.ncache.common.protobuf.PollCommandProtocol.PollCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommand registerPollNotifCommand = 97;
public boolean hasRegisterPollNotifCommand() {
return result.hasRegisterPollNotifCommand();
}
public com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand getRegisterPollNotifCommand() {
return result.getRegisterPollNotifCommand();
}
public Builder setRegisterPollNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRegisterPollNotifCommand = true;
result.registerPollNotifCommand_ = value;
return this;
}
public Builder setRegisterPollNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand.Builder builderForValue) {
result.hasRegisterPollNotifCommand = true;
result.registerPollNotifCommand_ = builderForValue.build();
return this;
}
public Builder mergeRegisterPollNotifCommand(com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand value) {
if (result.hasRegisterPollNotifCommand() &&
result.registerPollNotifCommand_ != com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand.getDefaultInstance()) {
result.registerPollNotifCommand_ =
com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand.newBuilder(result.registerPollNotifCommand_).mergeFrom(value).buildPartial();
} else {
result.registerPollNotifCommand_ = value;
}
result.hasRegisterPollNotifCommand = true;
return this;
}
public Builder clearRegisterPollNotifCommand() {
result.hasRegisterPollNotifCommand = false;
result.registerPollNotifCommand_ = com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.RegisterPollingNotificationCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommand getConnectedClientsCommand = 98;
public boolean hasGetConnectedClientsCommand() {
return result.hasGetConnectedClientsCommand();
}
public com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand getGetConnectedClientsCommand() {
return result.getGetConnectedClientsCommand();
}
public Builder setGetConnectedClientsCommand(com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetConnectedClientsCommand = true;
result.getConnectedClientsCommand_ = value;
return this;
}
public Builder setGetConnectedClientsCommand(com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand.Builder builderForValue) {
result.hasGetConnectedClientsCommand = true;
result.getConnectedClientsCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetConnectedClientsCommand(com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand value) {
if (result.hasGetConnectedClientsCommand() &&
result.getConnectedClientsCommand_ != com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand.getDefaultInstance()) {
result.getConnectedClientsCommand_ =
com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand.newBuilder(result.getConnectedClientsCommand_).mergeFrom(value).buildPartial();
} else {
result.getConnectedClientsCommand_ = value;
}
result.hasGetConnectedClientsCommand = true;
return this;
}
public Builder clearGetConnectedClientsCommand() {
result.hasGetConnectedClientsCommand = false;
result.getConnectedClientsCommand_ = com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.GetConnectedClientsCommand.getDefaultInstance();
return this;
}
// optional int32 MethodOverload = 99 [default = 0];
public boolean hasMethodOverload() {
return result.hasMethodOverload();
}
public int getMethodOverload() {
return result.getMethodOverload();
}
public Builder setMethodOverload(int value) {
result.hasMethodOverload = true;
result.methodOverload_ = value;
return this;
}
public Builder clearMethodOverload() {
result.hasMethodOverload = false;
result.methodOverload_ = 0;
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.TouchCommand touchCommand = 100;
public boolean hasTouchCommand() {
return result.hasTouchCommand();
}
public com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand getTouchCommand() {
return result.getTouchCommand();
}
public Builder setTouchCommand(com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTouchCommand = true;
result.touchCommand_ = value;
return this;
}
public Builder setTouchCommand(com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand.Builder builderForValue) {
result.hasTouchCommand = true;
result.touchCommand_ = builderForValue.build();
return this;
}
public Builder mergeTouchCommand(com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand value) {
if (result.hasTouchCommand() &&
result.touchCommand_ != com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand.getDefaultInstance()) {
result.touchCommand_ =
com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand.newBuilder(result.touchCommand_).mergeFrom(value).buildPartial();
} else {
result.touchCommand_ = value;
}
result.hasTouchCommand = true;
return this;
}
public Builder clearTouchCommand() {
result.hasTouchCommand = false;
result.touchCommand_ = com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.TouchCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommand getCacheManagementPortCommand = 101;
public boolean hasGetCacheManagementPortCommand() {
return result.hasGetCacheManagementPortCommand();
}
public com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand getGetCacheManagementPortCommand() {
return result.getGetCacheManagementPortCommand();
}
public Builder setGetCacheManagementPortCommand(com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetCacheManagementPortCommand = true;
result.getCacheManagementPortCommand_ = value;
return this;
}
public Builder setGetCacheManagementPortCommand(com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand.Builder builderForValue) {
result.hasGetCacheManagementPortCommand = true;
result.getCacheManagementPortCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetCacheManagementPortCommand(com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand value) {
if (result.hasGetCacheManagementPortCommand() &&
result.getCacheManagementPortCommand_ != com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand.getDefaultInstance()) {
result.getCacheManagementPortCommand_ =
com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand.newBuilder(result.getCacheManagementPortCommand_).mergeFrom(value).buildPartial();
} else {
result.getCacheManagementPortCommand_ = value;
}
result.hasGetCacheManagementPortCommand = true;
return this;
}
public Builder clearGetCacheManagementPortCommand() {
result.hasGetCacheManagementPortCommand = false;
result.getCacheManagementPortCommand_ = com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.GetCacheManagementPortCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetTopicCommand getTopicCommand = 102;
public boolean hasGetTopicCommand() {
return result.hasGetTopicCommand();
}
public com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand getGetTopicCommand() {
return result.getGetTopicCommand();
}
public Builder setGetTopicCommand(com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetTopicCommand = true;
result.getTopicCommand_ = value;
return this;
}
public Builder setGetTopicCommand(com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand.Builder builderForValue) {
result.hasGetTopicCommand = true;
result.getTopicCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetTopicCommand(com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand value) {
if (result.hasGetTopicCommand() &&
result.getTopicCommand_ != com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand.getDefaultInstance()) {
result.getTopicCommand_ =
com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand.newBuilder(result.getTopicCommand_).mergeFrom(value).buildPartial();
} else {
result.getTopicCommand_ = value;
}
result.hasGetTopicCommand = true;
return this;
}
public Builder clearGetTopicCommand() {
result.hasGetTopicCommand = false;
result.getTopicCommand_ = com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.GetTopicCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SubscribeTopicCommand subscribeTopicCommand = 103;
public boolean hasSubscribeTopicCommand() {
return result.hasSubscribeTopicCommand();
}
public com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand getSubscribeTopicCommand() {
return result.getSubscribeTopicCommand();
}
public Builder setSubscribeTopicCommand(com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSubscribeTopicCommand = true;
result.subscribeTopicCommand_ = value;
return this;
}
public Builder setSubscribeTopicCommand(com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand.Builder builderForValue) {
result.hasSubscribeTopicCommand = true;
result.subscribeTopicCommand_ = builderForValue.build();
return this;
}
public Builder mergeSubscribeTopicCommand(com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand value) {
if (result.hasSubscribeTopicCommand() &&
result.subscribeTopicCommand_ != com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand.getDefaultInstance()) {
result.subscribeTopicCommand_ =
com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand.newBuilder(result.subscribeTopicCommand_).mergeFrom(value).buildPartial();
} else {
result.subscribeTopicCommand_ = value;
}
result.hasSubscribeTopicCommand = true;
return this;
}
public Builder clearSubscribeTopicCommand() {
result.hasSubscribeTopicCommand = false;
result.subscribeTopicCommand_ = com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.SubscribeTopicCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.RemoveTopicCommand removeTopicCommand = 104;
public boolean hasRemoveTopicCommand() {
return result.hasRemoveTopicCommand();
}
public com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand getRemoveTopicCommand() {
return result.getRemoveTopicCommand();
}
public Builder setRemoveTopicCommand(com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRemoveTopicCommand = true;
result.removeTopicCommand_ = value;
return this;
}
public Builder setRemoveTopicCommand(com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand.Builder builderForValue) {
result.hasRemoveTopicCommand = true;
result.removeTopicCommand_ = builderForValue.build();
return this;
}
public Builder mergeRemoveTopicCommand(com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand value) {
if (result.hasRemoveTopicCommand() &&
result.removeTopicCommand_ != com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand.getDefaultInstance()) {
result.removeTopicCommand_ =
com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand.newBuilder(result.removeTopicCommand_).mergeFrom(value).buildPartial();
} else {
result.removeTopicCommand_ = value;
}
result.hasRemoveTopicCommand = true;
return this;
}
public Builder clearRemoveTopicCommand() {
result.hasRemoveTopicCommand = false;
result.removeTopicCommand_ = com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.RemoveTopicCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommand unSubscribeTopicCommand = 105;
public boolean hasUnSubscribeTopicCommand() {
return result.hasUnSubscribeTopicCommand();
}
public com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand getUnSubscribeTopicCommand() {
return result.getUnSubscribeTopicCommand();
}
public Builder setUnSubscribeTopicCommand(com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasUnSubscribeTopicCommand = true;
result.unSubscribeTopicCommand_ = value;
return this;
}
public Builder setUnSubscribeTopicCommand(com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand.Builder builderForValue) {
result.hasUnSubscribeTopicCommand = true;
result.unSubscribeTopicCommand_ = builderForValue.build();
return this;
}
public Builder mergeUnSubscribeTopicCommand(com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand value) {
if (result.hasUnSubscribeTopicCommand() &&
result.unSubscribeTopicCommand_ != com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand.getDefaultInstance()) {
result.unSubscribeTopicCommand_ =
com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand.newBuilder(result.unSubscribeTopicCommand_).mergeFrom(value).buildPartial();
} else {
result.unSubscribeTopicCommand_ = value;
}
result.hasUnSubscribeTopicCommand = true;
return this;
}
public Builder clearUnSubscribeTopicCommand() {
result.hasUnSubscribeTopicCommand = false;
result.unSubscribeTopicCommand_ = com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.UnSubscribeTopicCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.MessagePublishCommand messagePublishCommand = 106;
public boolean hasMessagePublishCommand() {
return result.hasMessagePublishCommand();
}
public com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand getMessagePublishCommand() {
return result.getMessagePublishCommand();
}
public Builder setMessagePublishCommand(com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasMessagePublishCommand = true;
result.messagePublishCommand_ = value;
return this;
}
public Builder setMessagePublishCommand(com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand.Builder builderForValue) {
result.hasMessagePublishCommand = true;
result.messagePublishCommand_ = builderForValue.build();
return this;
}
public Builder mergeMessagePublishCommand(com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand value) {
if (result.hasMessagePublishCommand() &&
result.messagePublishCommand_ != com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand.getDefaultInstance()) {
result.messagePublishCommand_ =
com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand.newBuilder(result.messagePublishCommand_).mergeFrom(value).buildPartial();
} else {
result.messagePublishCommand_ = value;
}
result.hasMessagePublishCommand = true;
return this;
}
public Builder clearMessagePublishCommand() {
result.hasMessagePublishCommand = false;
result.messagePublishCommand_ = com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetMessageCommand getMessageCommand = 107;
public boolean hasGetMessageCommand() {
return result.hasGetMessageCommand();
}
public com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand getGetMessageCommand() {
return result.getGetMessageCommand();
}
public Builder setGetMessageCommand(com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetMessageCommand = true;
result.getMessageCommand_ = value;
return this;
}
public Builder setGetMessageCommand(com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand.Builder builderForValue) {
result.hasGetMessageCommand = true;
result.getMessageCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetMessageCommand(com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand value) {
if (result.hasGetMessageCommand() &&
result.getMessageCommand_ != com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand.getDefaultInstance()) {
result.getMessageCommand_ =
com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand.newBuilder(result.getMessageCommand_).mergeFrom(value).buildPartial();
} else {
result.getMessageCommand_ = value;
}
result.hasGetMessageCommand = true;
return this;
}
public Builder clearGetMessageCommand() {
result.hasGetMessageCommand = false;
result.getMessageCommand_ = com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.GetMessageCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommand mesasgeAcknowledgmentCommand = 108;
public boolean hasMesasgeAcknowledgmentCommand() {
return result.hasMesasgeAcknowledgmentCommand();
}
public com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand getMesasgeAcknowledgmentCommand() {
return result.getMesasgeAcknowledgmentCommand();
}
public Builder setMesasgeAcknowledgmentCommand(com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasMesasgeAcknowledgmentCommand = true;
result.mesasgeAcknowledgmentCommand_ = value;
return this;
}
public Builder setMesasgeAcknowledgmentCommand(com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand.Builder builderForValue) {
result.hasMesasgeAcknowledgmentCommand = true;
result.mesasgeAcknowledgmentCommand_ = builderForValue.build();
return this;
}
public Builder mergeMesasgeAcknowledgmentCommand(com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand value) {
if (result.hasMesasgeAcknowledgmentCommand() &&
result.mesasgeAcknowledgmentCommand_ != com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand.getDefaultInstance()) {
result.mesasgeAcknowledgmentCommand_ =
com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand.newBuilder(result.mesasgeAcknowledgmentCommand_).mergeFrom(value).buildPartial();
} else {
result.mesasgeAcknowledgmentCommand_ = value;
}
result.hasMesasgeAcknowledgmentCommand = true;
return this;
}
public Builder clearMesasgeAcknowledgmentCommand() {
result.hasMesasgeAcknowledgmentCommand = false;
result.mesasgeAcknowledgmentCommand_ = com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.MesasgeAcknowledgmentCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.PingCommand pingCommand = 109;
public boolean hasPingCommand() {
return result.hasPingCommand();
}
public com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand getPingCommand() {
return result.getPingCommand();
}
public Builder setPingCommand(com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasPingCommand = true;
result.pingCommand_ = value;
return this;
}
public Builder setPingCommand(com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand.Builder builderForValue) {
result.hasPingCommand = true;
result.pingCommand_ = builderForValue.build();
return this;
}
public Builder mergePingCommand(com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand value) {
if (result.hasPingCommand() &&
result.pingCommand_ != com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand.getDefaultInstance()) {
result.pingCommand_ =
com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand.newBuilder(result.pingCommand_).mergeFrom(value).buildPartial();
} else {
result.pingCommand_ = value;
}
result.hasPingCommand = true;
return this;
}
public Builder clearPingCommand() {
result.hasPingCommand = false;
result.pingCommand_ = com.alachisoft.ncache.common.protobuf.PingCommandProtocol.PingCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.MessageCountCommand messageCountCommand = 110;
public boolean hasMessageCountCommand() {
return result.hasMessageCountCommand();
}
public com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand getMessageCountCommand() {
return result.getMessageCountCommand();
}
public Builder setMessageCountCommand(com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasMessageCountCommand = true;
result.messageCountCommand_ = value;
return this;
}
public Builder setMessageCountCommand(com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand.Builder builderForValue) {
result.hasMessageCountCommand = true;
result.messageCountCommand_ = builderForValue.build();
return this;
}
public Builder mergeMessageCountCommand(com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand value) {
if (result.hasMessageCountCommand() &&
result.messageCountCommand_ != com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand.getDefaultInstance()) {
result.messageCountCommand_ =
com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand.newBuilder(result.messageCountCommand_).mergeFrom(value).buildPartial();
} else {
result.messageCountCommand_ = value;
}
result.hasMessageCountCommand = true;
return this;
}
public Builder clearMessageCountCommand() {
result.hasMessageCountCommand = false;
result.messageCountCommand_ = com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.MessageCountCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.DataTypes dataTypes = 111;
public boolean hasDataTypes() {
return result.hasDataTypes();
}
public com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes getDataTypes() {
return result.getDataTypes();
}
public Builder setDataTypes(com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDataTypes = true;
result.dataTypes_ = value;
return this;
}
public Builder setDataTypes(com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes.Builder builderForValue) {
result.hasDataTypes = true;
result.dataTypes_ = builderForValue.build();
return this;
}
public Builder mergeDataTypes(com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes value) {
if (result.hasDataTypes() &&
result.dataTypes_ != com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes.getDefaultInstance()) {
result.dataTypes_ =
com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes.newBuilder(result.dataTypes_).mergeFrom(value).buildPartial();
} else {
result.dataTypes_ = value;
}
result.hasDataTypes = true;
return this;
}
public Builder clearDataTypes() {
result.hasDataTypes = false;
result.dataTypes_ = com.alachisoft.ncache.common.protobuf.DataTypesProtocol.DataTypes.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommand getSerializationFormatCommand = 112;
public boolean hasGetSerializationFormatCommand() {
return result.hasGetSerializationFormatCommand();
}
public com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand getGetSerializationFormatCommand() {
return result.getGetSerializationFormatCommand();
}
public Builder setGetSerializationFormatCommand(com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetSerializationFormatCommand = true;
result.getSerializationFormatCommand_ = value;
return this;
}
public Builder setGetSerializationFormatCommand(com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand.Builder builderForValue) {
result.hasGetSerializationFormatCommand = true;
result.getSerializationFormatCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetSerializationFormatCommand(com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand value) {
if (result.hasGetSerializationFormatCommand() &&
result.getSerializationFormatCommand_ != com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand.getDefaultInstance()) {
result.getSerializationFormatCommand_ =
com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand.newBuilder(result.getSerializationFormatCommand_).mergeFrom(value).buildPartial();
} else {
result.getSerializationFormatCommand_ = value;
}
result.hasGetSerializationFormatCommand = true;
return this;
}
public Builder clearGetSerializationFormatCommand() {
result.hasGetSerializationFormatCommand = false;
result.getSerializationFormatCommand_ = com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.GetSerializationFormatCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommand bulkGetCacheItemCommand = 113;
public boolean hasBulkGetCacheItemCommand() {
return result.hasBulkGetCacheItemCommand();
}
public com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand getBulkGetCacheItemCommand() {
return result.getBulkGetCacheItemCommand();
}
public Builder setBulkGetCacheItemCommand(com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBulkGetCacheItemCommand = true;
result.bulkGetCacheItemCommand_ = value;
return this;
}
public Builder setBulkGetCacheItemCommand(com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand.Builder builderForValue) {
result.hasBulkGetCacheItemCommand = true;
result.bulkGetCacheItemCommand_ = builderForValue.build();
return this;
}
public Builder mergeBulkGetCacheItemCommand(com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand value) {
if (result.hasBulkGetCacheItemCommand() &&
result.bulkGetCacheItemCommand_ != com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand.getDefaultInstance()) {
result.bulkGetCacheItemCommand_ =
com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand.newBuilder(result.bulkGetCacheItemCommand_).mergeFrom(value).buildPartial();
} else {
result.bulkGetCacheItemCommand_ = value;
}
result.hasBulkGetCacheItemCommand = true;
return this;
}
public Builder clearBulkGetCacheItemCommand() {
result.hasBulkGetCacheItemCommand = false;
result.bulkGetCacheItemCommand_ = com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.BulkGetCacheItemCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.ContainsBulkCommand containsBulkCommand = 114;
public boolean hasContainsBulkCommand() {
return result.hasContainsBulkCommand();
}
public com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand getContainsBulkCommand() {
return result.getContainsBulkCommand();
}
public Builder setContainsBulkCommand(com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasContainsBulkCommand = true;
result.containsBulkCommand_ = value;
return this;
}
public Builder setContainsBulkCommand(com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand.Builder builderForValue) {
result.hasContainsBulkCommand = true;
result.containsBulkCommand_ = builderForValue.build();
return this;
}
public Builder mergeContainsBulkCommand(com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand value) {
if (result.hasContainsBulkCommand() &&
result.containsBulkCommand_ != com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand.getDefaultInstance()) {
result.containsBulkCommand_ =
com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand.newBuilder(result.containsBulkCommand_).mergeFrom(value).buildPartial();
} else {
result.containsBulkCommand_ = value;
}
result.hasContainsBulkCommand = true;
return this;
}
public Builder clearContainsBulkCommand() {
result.hasContainsBulkCommand = false;
result.containsBulkCommand_ = com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.ModuleCommand moduleCommand = 115;
public boolean hasModuleCommand() {
return result.hasModuleCommand();
}
public com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand getModuleCommand() {
return result.getModuleCommand();
}
public Builder setModuleCommand(com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasModuleCommand = true;
result.moduleCommand_ = value;
return this;
}
public Builder setModuleCommand(com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand.Builder builderForValue) {
result.hasModuleCommand = true;
result.moduleCommand_ = builderForValue.build();
return this;
}
public Builder mergeModuleCommand(com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand value) {
if (result.hasModuleCommand() &&
result.moduleCommand_ != com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand.getDefaultInstance()) {
result.moduleCommand_ =
com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand.newBuilder(result.moduleCommand_).mergeFrom(value).buildPartial();
} else {
result.moduleCommand_ = value;
}
result.hasModuleCommand = true;
return this;
}
public Builder clearModuleCommand() {
result.hasModuleCommand = false;
result.moduleCommand_ = com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.ModuleCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SurrogateCommand surrogateCommand = 116;
public boolean hasSurrogateCommand() {
return result.hasSurrogateCommand();
}
public com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand getSurrogateCommand() {
return result.getSurrogateCommand();
}
public Builder setSurrogateCommand(com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSurrogateCommand = true;
result.surrogateCommand_ = value;
return this;
}
public Builder setSurrogateCommand(com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand.Builder builderForValue) {
result.hasSurrogateCommand = true;
result.surrogateCommand_ = builderForValue.build();
return this;
}
public Builder mergeSurrogateCommand(com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand value) {
if (result.hasSurrogateCommand() &&
result.surrogateCommand_ != com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand.getDefaultInstance()) {
result.surrogateCommand_ =
com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand.newBuilder(result.surrogateCommand_).mergeFrom(value).buildPartial();
} else {
result.surrogateCommand_ = value;
}
result.hasSurrogateCommand = true;
return this;
}
public Builder clearSurrogateCommand() {
result.hasSurrogateCommand = false;
result.surrogateCommand_ = com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.SurrogateCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommand messagePublishBulkCommand = 117;
public boolean hasMessagePublishBulkCommand() {
return result.hasMessagePublishBulkCommand();
}
public com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand getMessagePublishBulkCommand() {
return result.getMessagePublishBulkCommand();
}
public Builder setMessagePublishBulkCommand(com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasMessagePublishBulkCommand = true;
result.messagePublishBulkCommand_ = value;
return this;
}
public Builder setMessagePublishBulkCommand(com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.Builder builderForValue) {
result.hasMessagePublishBulkCommand = true;
result.messagePublishBulkCommand_ = builderForValue.build();
return this;
}
public Builder mergeMessagePublishBulkCommand(com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand value) {
if (result.hasMessagePublishBulkCommand() &&
result.messagePublishBulkCommand_ != com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.getDefaultInstance()) {
result.messagePublishBulkCommand_ =
com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.newBuilder(result.messagePublishBulkCommand_).mergeFrom(value).buildPartial();
} else {
result.messagePublishBulkCommand_ = value;
}
result.hasMessagePublishBulkCommand = true;
return this;
}
public Builder clearMessagePublishBulkCommand() {
result.hasMessagePublishBulkCommand = false;
result.messagePublishBulkCommand_ = com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplication bridgePollForResumingReplication = 118;
public boolean hasBridgePollForResumingReplication() {
return result.hasBridgePollForResumingReplication();
}
public com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication getBridgePollForResumingReplication() {
return result.getBridgePollForResumingReplication();
}
public Builder setBridgePollForResumingReplication(com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication value) {
if (value == null) {
throw new NullPointerException();
}
result.hasBridgePollForResumingReplication = true;
result.bridgePollForResumingReplication_ = value;
return this;
}
public Builder setBridgePollForResumingReplication(com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication.Builder builderForValue) {
result.hasBridgePollForResumingReplication = true;
result.bridgePollForResumingReplication_ = builderForValue.build();
return this;
}
public Builder mergeBridgePollForResumingReplication(com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication value) {
if (result.hasBridgePollForResumingReplication() &&
result.bridgePollForResumingReplication_ != com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication.getDefaultInstance()) {
result.bridgePollForResumingReplication_ =
com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication.newBuilder(result.bridgePollForResumingReplication_).mergeFrom(value).buildPartial();
} else {
result.bridgePollForResumingReplication_ = value;
}
result.hasBridgePollForResumingReplication = true;
return this;
}
public Builder clearBridgePollForResumingReplication() {
result.hasBridgePollForResumingReplication = false;
result.bridgePollForResumingReplication_ = com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.BridgePollForResumingReplication.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.GetModuleStateCommand getModuleStateCommand = 119;
public boolean hasGetModuleStateCommand() {
return result.hasGetModuleStateCommand();
}
public com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand getGetModuleStateCommand() {
return result.getGetModuleStateCommand();
}
public Builder setGetModuleStateCommand(com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasGetModuleStateCommand = true;
result.getModuleStateCommand_ = value;
return this;
}
public Builder setGetModuleStateCommand(com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand.Builder builderForValue) {
result.hasGetModuleStateCommand = true;
result.getModuleStateCommand_ = builderForValue.build();
return this;
}
public Builder mergeGetModuleStateCommand(com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand value) {
if (result.hasGetModuleStateCommand() &&
result.getModuleStateCommand_ != com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand.getDefaultInstance()) {
result.getModuleStateCommand_ =
com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand.newBuilder(result.getModuleStateCommand_).mergeFrom(value).buildPartial();
} else {
result.getModuleStateCommand_ = value;
}
result.hasGetModuleStateCommand = true;
return this;
}
public Builder clearGetModuleStateCommand() {
result.hasGetModuleStateCommand = false;
result.getModuleStateCommand_ = com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.GetModuleStateCommand.getDefaultInstance();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.SetModuleStateCommand setModuleStateCommand = 120;
public boolean hasSetModuleStateCommand() {
return result.hasSetModuleStateCommand();
}
public com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand getSetModuleStateCommand() {
return result.getSetModuleStateCommand();
}
public Builder setSetModuleStateCommand(com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSetModuleStateCommand = true;
result.setModuleStateCommand_ = value;
return this;
}
public Builder setSetModuleStateCommand(com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand.Builder builderForValue) {
result.hasSetModuleStateCommand = true;
result.setModuleStateCommand_ = builderForValue.build();
return this;
}
public Builder mergeSetModuleStateCommand(com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand value) {
if (result.hasSetModuleStateCommand() &&
result.setModuleStateCommand_ != com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand.getDefaultInstance()) {
result.setModuleStateCommand_ =
com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand.newBuilder(result.setModuleStateCommand_).mergeFrom(value).buildPartial();
} else {
result.setModuleStateCommand_ = value;
}
result.hasSetModuleStateCommand = true;
return this;
}
public Builder clearSetModuleStateCommand() {
result.hasSetModuleStateCommand = false;
result.setModuleStateCommand_ = com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.SetModuleStateCommand.getDefaultInstance();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.Command)
}
static {
defaultInstance = new Command(true);
com.alachisoft.ncache.common.protobuf.CommandProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.Command)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_Command_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_Command_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\rCommand.proto\022%com.alachisoft.ncache.c" +
"ommon.protobuf\032\020AddCommand.proto\032\032AddDep" +
"endencyCommand.proto\032\036AddSyncDependencyC" +
"ommand.proto\032\024BulkAddCommand.proto\032\024Bulk" +
"GetCommand.proto\032\027BulkInsertCommand.prot" +
"o\032\027BulkRemoveCommand.proto\032\022ClearCommand" +
".proto\032\025ContainsCommand.proto\032\022CountComm" +
"and.proto\032\024DisposeCommand.proto\032\031GetCach" +
"eItemCommand.proto\032\020GetCommand.proto\032\034Ge" +
"tCompactTypesCommand.proto\032\032GetEnumerato",
"rCommand.proto\032\025GetGroupCommand.proto\032\027G" +
"etHashmapCommand.proto\032\035GetOptimalServer" +
"Command.proto\032\035GetThresholdSizeCommand.p" +
"roto\032\033GetTypeInfoMapCommand.proto\032\021InitC" +
"ommand.proto\032\023InsertCommand.proto\032\035Raise" +
"CustomEventCommand.proto\032\035RegisterKeyNot" +
"ifCommand.proto\032!RegisterBulkKeyNotifCom" +
"mand.proto\032\032RegisterNotifCommand.proto\032\023" +
"RemoveCommand.proto\032\030RemoveGroupCommand." +
"proto\032\023SearchCommand.proto\032\023GetTagComman",
"d.proto\032\021LockCommand.proto\032\025IsLockedComm" +
"and.proto\032\027LockVerifyCommand.proto\032\023Unlo" +
"ckCommand.proto\032\037UnRegisterKeyNotifComma" +
"nd.proto\032#UnRegisterBulkKeyNotifCommand." +
"proto\032\027HybridBulkCommand.proto\032\033GetLoggi" +
"ngInfoCommand.proto\032\030CloseStreamCommand." +
"proto\032\034GetStreamLengthCommand.proto\032\027Ope" +
"nStreamCommand.proto\032\032WriteToStreamComma" +
"nd.proto\032\033ReadFromStreamCommand.proto\032!G" +
"etStateTransferInfoCommand.proto\032#GetTra",
"nsferableKeyListCommand.proto\032\035BridgeHyb" +
"ridBulkCommand.proto\032\027BridgeInitCommand." +
"proto\032#SetTransferableKeyListCommand.pro" +
"to\032$SignlEndOfStateTransferCommand.proto" +
"\032\033BridgeSyncTimeCommand.proto\032$BridgeHea" +
"rtBeatReceivedCommand.proto\032\030RemoveByTag" +
"Command.proto\032/BridgeMakeTargetCacheActi" +
"vePassiveCommand.proto\032\031UnRegisterCQComm" +
"and.proto\032\025SearchCQCommand.proto\032\027Regist" +
"erCQCommand.proto\032\031GetKeysByTagCommand.p",
"roto\032\027BulkDeleteCommand.proto\032\023DeleteCom" +
"mand.proto\032\031GetNextChunkCommand.proto\032\036G" +
"etGroupNextChunkCommand.proto\032\031AddAttrib" +
"uteCommand.proto\032\032GetEncryptionCommand.p" +
"roto\032\022GetLCCommand.proto\032\036GetRunningServ" +
"ersCommand.proto\032\027SyncEventsCommand.prot" +
"o\032\030DeleteQueryCommand.proto\032\036GetProductV" +
"ersionCommand.proto\032$GetReplicatorStatus" +
"InfoCommand.proto\032\035GetServerMappingComma" +
"nd.proto\032!RequestStateTransferCommand.pr",
"oto\032\034GetCacheBindingCommand.proto\032\032MapRe" +
"duceTaskCommand.proto\032\031TaskCallbackComma" +
"nd.proto\032\027TaskCancelCommand.proto\032\031TaskP" +
"rogressCommand.proto\032\034GetRunningTasksCom" +
"mand.proto\032\032GetNextRecordCommand.proto\032\033" +
"TaskEnumeratorCommand.proto\032!InvokeEntry" +
"ProcessorCommand.proto\032\033InquiryRequestCo" +
"mmand.proto\032\032ExecuteReaderCommand.proto\032" +
"\037GetReaderNextChunkCommand.proto\032\032Dispos" +
"eReaderCommand.proto\032\034ExecuteReaderCQCom",
"mand.proto\032\032GetExpirationCommand.proto\032\021" +
"PollCommand.proto\032(RegisterPollingNotifi" +
"cationCommand.proto\032\'CacheSecurityAuthor" +
"izationCommand.proto\032 GetConnectedClient" +
"sCommand.proto\032\022TouchCommand.proto\032\025GetT" +
"opicCommand.proto\032\033SubscribeTopicCommand" +
".proto\032\030RemoveTopicCommand.proto\032\035UnSubs" +
"cribeTopicCommand.proto\032\033MessagePublishC" +
"ommand.proto\032\027GetMessageCommand.proto\032\"M" +
"esasgeAcknowledgmentCommand.proto\032#GetCa",
"cheManagementPortCommand.proto\032\021PingComm" +
"and.proto\032\031MessageCountCommand.proto\032\017Da" +
"taTypes.proto\032#GetSerializationFormatCom" +
"mand.proto\032\035BulkGetCacheItemCommand.prot" +
"o\032\031ContainsBulkCommand.proto\032\026SurrogateC" +
"ommand.proto\032\023ModuleCommand.proto\032\037Messa" +
"gePublishBulkCommand.proto\032&BridgePollFo" +
"rResumingReplication.proto\032\033GetModuleSta" +
"teCommand.proto\032\033SetModuleStateCommand.p" +
"roto\"\360d\n\007Command\022A\n\004type\030\001 \001(\01623.com.ala",
"chisoft.ncache.common.protobuf.Command.T" +
"ype\022\017\n\007version\030\002 \001(\t\022E\n\naddCommand\030\003 \001(\013" +
"21.com.alachisoft.ncache.common.protobuf" +
".AddCommand\022Y\n\024addDependencyCommand\030\004 \001(" +
"\0132;.com.alachisoft.ncache.common.protobu" +
"f.AddDependencyCommand\022a\n\030addSyncDepende" +
"ncyCommand\030\005 \001(\0132?.com.alachisoft.ncache" +
".common.protobuf.AddSyncDependencyComman" +
"d\022M\n\016bulkAddCommand\030\006 \001(\01325.com.alachiso" +
"ft.ncache.common.protobuf.BulkAddCommand",
"\022M\n\016bulkGetCommand\030\007 \001(\01325.com.alachisof" +
"t.ncache.common.protobuf.BulkGetCommand\022" +
"S\n\021bulkInsertCommand\030\010 \001(\01328.com.alachis" +
"oft.ncache.common.protobuf.BulkInsertCom" +
"mand\022S\n\021bulkRemoveCommand\030\t \001(\01328.com.al" +
"achisoft.ncache.common.protobuf.BulkRemo" +
"veCommand\022I\n\014clearCommand\030\n \001(\01323.com.al" +
"achisoft.ncache.common.protobuf.ClearCom" +
"mand\022O\n\017containsCommand\030\013 \001(\01326.com.alac" +
"hisoft.ncache.common.protobuf.ContainsCo",
"mmand\022I\n\014countCommand\030\014 \001(\01323.com.alachi" +
"soft.ncache.common.protobuf.CountCommand" +
"\022M\n\016disposeCommand\030\r \001(\01325.com.alachisof" +
"t.ncache.common.protobuf.DisposeCommand\022" +
"W\n\023getCacheItemCommand\030\016 \001(\0132:.com.alach" +
"isoft.ncache.common.protobuf.GetCacheIte" +
"mCommand\022E\n\ngetCommand\030\017 \001(\01321.com.alach" +
"isoft.ncache.common.protobuf.GetCommand\022" +
"]\n\026getCompactTypesCommand\030\020 \001(\0132=.com.al" +
"achisoft.ncache.common.protobuf.GetCompa",
"ctTypesCommand\022Y\n\024getEnumeratorCommand\030\021" +
" \001(\0132;.com.alachisoft.ncache.common.prot" +
"obuf.GetEnumeratorCommand\022O\n\017getGroupCom" +
"mand\030\022 \001(\01326.com.alachisoft.ncache.commo" +
"n.protobuf.GetGroupCommand\022S\n\021getHashmap" +
"Command\030\023 \001(\01328.com.alachisoft.ncache.co" +
"mmon.protobuf.GetHashmapCommand\022_\n\027getOp" +
"timalServerCommand\030\024 \001(\0132>.com.alachisof" +
"t.ncache.common.protobuf.GetOptimalServe" +
"rCommand\022_\n\027getThresholdSizeCommand\030\025 \001(",
"\0132>.com.alachisoft.ncache.common.protobu" +
"f.GetThresholdSizeCommand\022[\n\025getTypeInfo" +
"MapCommand\030\026 \001(\0132<.com.alachisoft.ncache" +
".common.protobuf.GetTypeInfoMapCommand\022G" +
"\n\013initCommand\030\027 \001(\01322.com.alachisoft.nca" +
"che.common.protobuf.InitCommand\022K\n\rinser" +
"tCommand\030\030 \001(\01324.com.alachisoft.ncache.c" +
"ommon.protobuf.InsertCommand\022_\n\027raiseCus" +
"tomEventCommand\030\031 \001(\0132>.com.alachisoft.n" +
"cache.common.protobuf.RaiseCustomEventCo",
"mmand\022_\n\027registerKeyNotifCommand\030\032 \001(\0132>" +
".com.alachisoft.ncache.common.protobuf.R" +
"egisterKeyNotifCommand\022Y\n\024registerNotifC" +
"ommand\030\033 \001(\0132;.com.alachisoft.ncache.com" +
"mon.protobuf.RegisterNotifCommand\022K\n\rrem" +
"oveCommand\030\034 \001(\01324.com.alachisoft.ncache" +
".common.protobuf.RemoveCommand\022U\n\022remove" +
"GroupCommand\030\035 \001(\01329.com.alachisoft.ncac" +
"he.common.protobuf.RemoveGroupCommand\022K\n" +
"\rsearchCommand\030\036 \001(\01324.com.alachisoft.nc",
"ache.common.protobuf.SearchCommand\022K\n\rge" +
"tTagCommand\030\037 \001(\01324.com.alachisoft.ncach" +
"e.common.protobuf.GetTagCommand\022G\n\013lockC" +
"ommand\030 \001(\01322.com.alachisoft.ncache.com" +
"mon.protobuf.LockCommand\022K\n\runlockComman" +
"d\030! \001(\01324.com.alachisoft.ncache.common.p" +
"rotobuf.UnlockCommand\022O\n\017isLockedCommand" +
"\030\" \001(\01326.com.alachisoft.ncache.common.pr" +
"otobuf.IsLockedCommand\022S\n\021lockVerifyComm" +
"and\030# \001(\01328.com.alachisoft.ncache.common",
".protobuf.LockVerifyCommand\022c\n\031unRegiste" +
"rKeyNotifCommand\030$ \001(\[email protected]." +
"ncache.common.protobuf.UnRegisterKeyNoti" +
"fCommand\022k\n\035unRegisterBulkKeyNotifComman" +
"d\030% \001(\0132D.com.alachisoft.ncache.common.p" +
"rotobuf.UnRegisterBulkKeyNotifCommand\022g\n" +
"\033registerBulkKeyNotifCommand\030& \001(\0132B.com" +
".alachisoft.ncache.common.protobuf.Regis" +
"terBulkKeyNotifCommand\022S\n\021hybridBulkComm" +
"and\030\' \001(\01328.com.alachisoft.ncache.common",
".protobuf.HybridBulkCommand\022[\n\025getLoggin" +
"gInfoCommand\030( \001(\0132<.com.alachisoft.ncac" +
"he.common.protobuf.GetLoggingInfoCommand" +
"\022U\n\022closeStreamCommand\030) \001(\01329.com.alach" +
"isoft.ncache.common.protobuf.CloseStream" +
"Command\022]\n\026getStreamLengthCommand\030* \001(\0132" +
"=.com.alachisoft.ncache.common.protobuf." +
"GetStreamLengthCommand\022S\n\021openStreamComm" +
"and\030+ \001(\01328.com.alachisoft.ncache.common" +
".protobuf.OpenStreamCommand\022Y\n\024writeToSt",
"reamCommand\030, \001(\0132;.com.alachisoft.ncach" +
"e.common.protobuf.WriteToStreamCommand\022[" +
"\n\025readFromStreamCommand\030- \001(\0132<.com.alac" +
"hisoft.ncache.common.protobuf.ReadFromSt" +
"reamCommand\022m\n!bridgeGetStateTransferInf" +
"oCommand\030. \001(\0132B.com.alachisoft.ncache.c" +
"ommon.protobuf.GetStateTransferInfoComma" +
"nd\022q\n#bridgeGetTransferableKeyListComman" +
"d\030/ \001(\0132D.com.alachisoft.ncache.common.p" +
"rotobuf.GetTransferableKeyListCommand\022_\n",
"\027bridgeHybridBulkCommand\0300 \001(\0132>.com.ala" +
"chisoft.ncache.common.protobuf.BridgeHyb" +
"ridBulkCommand\022S\n\021bridgeInitCommand\0301 \001(" +
"\01328.com.alachisoft.ncache.common.protobu" +
"f.BridgeInitCommand\022q\n#bridgeSetTransfer" +
"ableKeyListCommand\0302 \001(\0132D.com.alachisof" +
"t.ncache.common.protobuf.SetTransferable" +
"KeyListCommand\022s\n$bridgeSignlEndOfStateT" +
"ransferCommand\0303 \001(\0132E.com.alachisoft.nc" +
"ache.common.protobuf.SignlEndOfStateTran",
"sferCommand\022\021\n\trequestID\0304 \001(\003\022\031\n\016comman" +
"dVersion\0305 \001(\005:\0010\022[\n\025bridgeSyncTimeComma" +
"nd\0306 \001(\0132<.com.alachisoft.ncache.common." +
"protobuf.BridgeSyncTimeCommand\022m\n\036bridge" +
"HeartBeatReceivedCommand\0307 \001(\0132E.com.ala" +
"chisoft.ncache.common.protobuf.BridgeHea" +
"rtBeatReceivedCommand\022U\n\022removeByTagComm" +
"and\0308 \001(\01329.com.alachisoft.ncache.common" +
".protobuf.RemoveByTagCommand\022\203\001\n)bridgeM" +
"akeTargetCacheActivePassiveCommand\0309 \001(\013",
"2P.com.alachisoft.ncache.common.protobuf" +
".BridgeMakeTargetCacheActivePassiveComma" +
"nd\022W\n\023unRegisterCQCommand\030: \001(\0132:.com.al" +
"achisoft.ncache.common.protobuf.UnRegist" +
"erCQCommand\022O\n\017searchCQCommand\030; \001(\01326.c" +
"om.alachisoft.ncache.common.protobuf.Sea" +
"rchCQCommand\022S\n\021registerCQCommand\030< \001(\0132" +
"8.com.alachisoft.ncache.common.protobuf." +
"RegisterCQCommand\022W\n\023getKeysByTagCommand" +
"\030= \001(\0132:.com.alachisoft.ncache.common.pr",
"otobuf.GetKeysByTagCommand\022\034\n\020clientLast" +
"ViewId\030> \001(\003:\002-1\022S\n\021bulkDeleteCommand\030? " +
"\001(\01328.com.alachisoft.ncache.common.proto" +
"buf.BulkDeleteCommand\022K\n\rdeleteCommand\030@" +
" \001(\01324.com.alachisoft.ncache.common.prot" +
"obuf.DeleteCommand\022\033\n\021intendedRecipient\030" +
"A \001(\t:\000\022W\n\023getNextChunkCommand\030B \001(\0132:.c" +
"om.alachisoft.ncache.common.protobuf.Get" +
"NextChunkCommand\022a\n\030getGroupNextChunkCom" +
"mand\030C \001(\0132?.com.alachisoft.ncache.commo",
"n.protobuf.GetGroupNextChunkCommand\022W\n\023a" +
"ddAttributeCommand\030D \001(\0132:.com.alachisof" +
"t.ncache.common.protobuf.AddAttributeCom" +
"mand\022Y\n\024getEncryptionCommand\030E \001(\0132;.com" +
".alachisoft.ncache.common.protobuf.GetEn" +
"cryptionCommand\022a\n\030getRunningServersComm" +
"and\030F \001(\0132?.com.alachisoft.ncache.common" +
".protobuf.GetRunningServersCommand\022S\n\021sy" +
"ncEventsCommand\030G \001(\01328.com.alachisoft.n" +
"cache.common.protobuf.SyncEventsCommand\022",
"U\n\022deleteQueryCommand\030H \001(\01329.com.alachi" +
"soft.ncache.common.protobuf.DeleteQueryC" +
"ommand\022a\n\030getProductVersionCommand\030I \001(\013" +
"2?.com.alachisoft.ncache.common.protobuf" +
".GetProductVersionCommand\022s\n$bridgeGetRe" +
"plicatorStatusInfoCommand\030J \001(\0132E.com.al" +
"achisoft.ncache.common.protobuf.GetRepli" +
"catorStatusInfoCommand\022m\n!bridgeRequestS" +
"tateTransferCommand\030K \001(\0132B.com.alachiso" +
"ft.ncache.common.protobuf.RequestStateTr",
"ansferCommand\022_\n\027getServerMappingCommand" +
"\030L \001(\0132>.com.alachisoft.ncache.common.pr" +
"otobuf.GetServerMappingCommand\022[\n\025inquir" +
"yRequestCommand\030M \001(\0132<.com.alachisoft.n" +
"cache.common.protobuf.InquiryRequestComm" +
"and\022\035\n\016isRetryCommand\030N \001(\010:\005false\022Y\n\024ma" +
"pReduceTaskCommand\030O \001(\0132;.com.alachisof" +
"t.ncache.common.protobuf.MapReduceTaskCo" +
"mmand\022W\n\023TaskCallbackCommand\030P \001(\0132:.com" +
".alachisoft.ncache.common.protobuf.TaskC",
"allbackCommand\022Z\n\023RunningTasksCommand\030Q " +
"\001(\0132=.com.alachisoft.ncache.common.proto" +
"buf.GetRunningTasksCommand\022S\n\021TaskCancel" +
"Command\030R \001(\01328.com.alachisoft.ncache.co" +
"mmon.protobuf.TaskCancelCommand\022W\n\023TaskP" +
"rogressCommand\030S \001(\0132:.com.alachisoft.nc" +
"ache.common.protobuf.TaskProgressCommand" +
"\022V\n\021NextRecordCommand\030T \001(\0132;.com.alachi" +
"soft.ncache.common.protobuf.GetNextRecor" +
"dCommand\022\025\n\tcommandID\030U \001(\005:\002-1\022]\n\026getCa",
"cheBindingCommand\030V \001(\0132=.com.alachisoft" +
".ncache.common.protobuf.GetCacheBindingC" +
"ommand\022[\n\025TaskEnumeratorCommand\030W \001(\0132<." +
"com.alachisoft.ncache.common.protobuf.Ta" +
"skEnumeratorCommand\022g\n\033invokeEntryProces" +
"sorCommand\030X \001(\0132B.com.alachisoft.ncache" +
".common.protobuf.InvokeEntryProcessorCom" +
"mand\022Y\n\024executeReaderCommand\030Y \001(\0132;.com" +
".alachisoft.ncache.common.protobuf.Execu" +
"teReaderCommand\022c\n\031getReaderNextChunkCom",
"mand\030Z \001(\[email protected]" +
"n.protobuf.GetReaderNextChunkCommand\022Y\n\024" +
"disposeReaderCommand\030[ \001(\0132;.com.alachis" +
"oft.ncache.common.protobuf.DisposeReader" +
"Command\022]\n\026executeReaderCQCommand\030\\ \001(\0132" +
"=.com.alachisoft.ncache.common.protobuf." +
"ExecuteReaderCQCommand\022Y\n\024getExpirationC" +
"ommand\030] \001(\0132;.com.alachisoft.ncache.com" +
"mon.protobuf.GetExpirationCommand\022I\n\014get" +
"LCCommand\030^ \001(\01323.com.alachisoft.ncache.",
"common.protobuf.GetLCCommand\022s\n!cacheSec" +
"urityAuthorizationCommand\030_ \001(\0132H.com.al" +
"achisoft.ncache.common.protobuf.CacheSec" +
"urityAuthorizationCommand\022G\n\013pollCommand" +
"\030` \001(\01322.com.alachisoft.ncache.common.pr" +
"otobuf.PollCommand\022k\n\030registerPollNotifC" +
"ommand\030a \001(\0132I.com.alachisoft.ncache.com" +
"mon.protobuf.RegisterPollingNotification" +
"Command\022e\n\032getConnectedClientsCommand\030b " +
"\001(\0132A.com.alachisoft.ncache.common.proto",
"buf.GetConnectedClientsCommand\022\031\n\016Method" +
"Overload\030c \001(\005:\0010\022I\n\014touchCommand\030d \001(\0132" +
"3.com.alachisoft.ncache.common.protobuf." +
"TouchCommand\022k\n\035getCacheManagementPortCo" +
"mmand\030e \001(\0132D.com.alachisoft.ncache.comm" +
"on.protobuf.GetCacheManagementPortComman" +
"d\022O\n\017getTopicCommand\030f \001(\01326.com.alachis" +
"oft.ncache.common.protobuf.GetTopicComma" +
"nd\022[\n\025subscribeTopicCommand\030g \001(\0132<.com." +
"alachisoft.ncache.common.protobuf.Subscr",
"ibeTopicCommand\022U\n\022removeTopicCommand\030h " +
"\001(\01329.com.alachisoft.ncache.common.proto" +
"buf.RemoveTopicCommand\022_\n\027unSubscribeTop" +
"icCommand\030i \001(\0132>.com.alachisoft.ncache." +
"common.protobuf.UnSubscribeTopicCommand\022" +
"[\n\025messagePublishCommand\030j \001(\0132<.com.ala" +
"chisoft.ncache.common.protobuf.MessagePu" +
"blishCommand\022S\n\021getMessageCommand\030k \001(\0132" +
"8.com.alachisoft.ncache.common.protobuf." +
"GetMessageCommand\022i\n\034mesasgeAcknowledgme",
"ntCommand\030l \001(\0132C.com.alachisoft.ncache." +
"common.protobuf.MesasgeAcknowledgmentCom" +
"mand\022G\n\013pingCommand\030m \001(\01322.com.alachiso" +
"ft.ncache.common.protobuf.PingCommand\022W\n" +
"\023messageCountCommand\030n \001(\0132:.com.alachis" +
"oft.ncache.common.protobuf.MessageCountC" +
"ommand\022C\n\tdataTypes\030o \001(\01320.com.alachiso" +
"ft.ncache.common.protobuf.DataTypes\022k\n\035g" +
"etSerializationFormatCommand\030p \001(\0132D.com" +
".alachisoft.ncache.common.protobuf.GetSe",
"rializationFormatCommand\022_\n\027bulkGetCache" +
"ItemCommand\030q \001(\0132>.com.alachisoft.ncach" +
"e.common.protobuf.BulkGetCacheItemComman" +
"d\022W\n\023containsBulkCommand\030r \001(\0132:.com.ala" +
"chisoft.ncache.common.protobuf.ContainsB" +
"ulkCommand\022K\n\rmoduleCommand\030s \001(\01324.com." +
"alachisoft.ncache.common.protobuf.Module" +
"Command\022Q\n\020surrogateCommand\030t \001(\01327.com." +
"alachisoft.ncache.common.protobuf.Surrog" +
"ateCommand\022c\n\031messagePublishBulkCommand\030",
"u \001(\[email protected]" +
"tobuf.MessagePublishBulkCommand\022q\n bridg" +
"ePollForResumingReplication\030v \001(\0132G.com." +
"alachisoft.ncache.common.protobuf.Bridge" +
"PollForResumingReplication\022[\n\025getModuleS" +
"tateCommand\030w \001(\0132<.com.alachisoft.ncach" +
"e.common.protobuf.GetModuleStateCommand\022" +
"[\n\025setModuleStateCommand\030x \001(\0132<.com.ala" +
"chisoft.ncache.common.protobuf.SetModule" +
"StateCommand\"\310\023\n\004Type\022\007\n\003ADD\020\001\022\022\n\016ADD_DE",
"PENDENCY\020\002\022\027\n\023ADD_SYNC_DEPENDENCY\020\003\022\014\n\010A" +
"DD_BULK\020\004\022\014\n\010GET_BULK\020\005\022\017\n\013INSERT_BULK\020\006" +
"\022\017\n\013REMOVE_BULK\020\007\022\t\n\005CLEAR\020\010\022\014\n\010CONTAINS" +
"\020\t\022\t\n\005COUNT\020\n\022\013\n\007DISPOSE\020\013\022\022\n\016GET_CACHE_" +
"ITEM\020\014\022\007\n\003GET\020\r\022\025\n\021GET_COMPACT_TYPES\020\016\022\022" +
"\n\016GET_ENUMERATOR\020\017\022\r\n\tGET_GROUP\020\020\022\017\n\013GET" +
"_HASHMAP\020\021\022\026\n\022GET_OPTIMAL_SERVER\020\022\022\026\n\022GE" +
"T_THRESHOLD_SIZE\020\023\022\024\n\020GET_TYPEINFO_MAP\020\024" +
"\022\010\n\004INIT\020\025\022\n\n\006INSERT\020\026\022\026\n\022RAISE_CUSTOM_E" +
"VENT\020\027\022\026\n\022REGISTER_KEY_NOTIF\020\030\022\022\n\016REGIST",
"ER_NOTIF\020\031\022\n\n\006REMOVE\020\032\022\020\n\014REMOVE_GROUP\020\033" +
"\022\n\n\006SEARCH\020\034\022\013\n\007GET_TAG\020\035\022\010\n\004LOCK\020\036\022\n\n\006U" +
"NLOCK\020\037\022\014\n\010ISLOCKED\020 \022\017\n\013LOCK_VERIFY\020!\022\030" +
"\n\024UNREGISTER_KEY_NOTIF\020\"\022\035\n\031UNREGISTER_B" +
"ULK_KEY_NOTIF\020#\022\033\n\027REGISTER_BULK_KEY_NOT" +
"IF\020$\022\017\n\013HYBRID_BULK\020%\022\024\n\020GET_LOGGING_INF" +
"O\020&\022\020\n\014CLOSE_STREAM\020\'\022\025\n\021GET_STREAM_LENG" +
"TH\020(\022\017\n\013OPEN_STREAM\020)\022\023\n\017WRITE_TO_STREAM" +
"\020*\022\024\n\020READ_FROM_STREAM\020+\022\"\n\036BRIDGE_GET_S" +
"TATE_TRANSFER_INFO\020,\022$\n BRIDGE_GET_TRANS",
"FERABLE_KEY_LIST\020-\022\026\n\022BRIDGE_HYBRID_BULK" +
"\020.\022\017\n\013BRIDGE_INIT\020/\022$\n BRIDGE_SET_TRANSF" +
"ERABLE_KEY_LIST\0200\022\'\n#BRIDGE_SIGNAL_END_O" +
"F_STATE_TRANSFER\0201\022\024\n\020BRIDGE_SYNC_TIME\0202" +
"\022\036\n\032BRIDGE_HEART_BEAT_RECEIVED\0203\022\021\n\rREMO" +
"VE_BY_TAG\0204\022+\n\'BRIDGE_MAKE_TARGET_CACHE_" +
"ACTIVE_PASSIVE\0205\022\021\n\rUNREGISTER_CQ\0206\022\r\n\tS" +
"EARCH_CQ\0207\022\017\n\013REGISTER_CQ\0208\022\020\n\014GET_KEYS_" +
"TAG\0209\022\017\n\013DELETE_BULK\020:\022\n\n\006DELETE\020;\022\022\n\016GE" +
"T_NEXT_CHUNK\020<\022\030\n\024GET_GROUP_NEXT_CHUNK\020=",
"\022\021\n\rADD_ATTRIBUTE\020>\022\022\n\016GET_ENCRYPTION\020?\022" +
"\027\n\023GET_RUNNING_SERVERS\020@\022\017\n\013SYNC_EVENTS\020" +
"A\022\017\n\013DELETEQUERY\020B\022\027\n\023GET_PRODUCT_VERSIO" +
"N\020C\022%\n!BRIDGE_GET_REPLICATOR_STATUS_INFO" +
"\020D\022!\n\035BRIDGE_REQUEST_STATE_TRANSFER\020E\022\026\n" +
"\022GET_SERVER_MAPPING\020F\022\023\n\017INQUIRY_REQUEST" +
"\020G\022\025\n\021GET_CACHE_BINDING\020H\022\023\n\017MAP_REDUCE_" +
"TASK\020J\022\021\n\rTASK_CALLBACK\020K\022\021\n\rRUNNING_TAS" +
"KS\020L\022\021\n\rTASK_PROGRESS\020M\022\017\n\013CANCEL_TASK\020N" +
"\022\024\n\020TASK_NEXT_RECORD\020O\022\023\n\017TASK_ENUMERATO",
"R\020P\022\032\n\026INVOKE_ENTRY_PROCESSOR\020Q\022\022\n\016EXECU" +
"TE_READER\020R\022\024\n\020GET_READER_CHUNK\020S\022\022\n\016DIS" +
"POSE_READER\020T\022\025\n\021EXECUTE_READER_CQ\020U\022\022\n\016" +
"GET_EXPIRATION\020V\022\017\n\013GET_LC_DATA\020W\022\010\n\004POL" +
"L\020X\022!\n\035REGISTER_POLLING_NOTIFICATION\020Y\022\032" +
"\n\026SECURITY_AUTHORIZATION\020Z\022\031\n\025GET_CONNEC" +
"TED_CLIENTS\020[\022\t\n\005TOUCH\020\\\022\035\n\031GET_CACHE_MA" +
"NAGEMENT_PORT\020^\022\r\n\tGET_TOPIC\020_\022\023\n\017SUBSCR" +
"IBE_TOPIC\020a\022\020\n\014REMOVE_TOPIC\020b\022\025\n\021UNSUBSC" +
"RIBE_TOPIC\020c\022\023\n\017MESSAGE_PUBLISH\020d\022\017\n\013GET",
"_MESSAGE\020e\022\032\n\026MESSAGE_ACKNOWLEDGMENT\020f\022\010" +
"\n\004PING\020g\022\021\n\rMESSAGE_COUNT\020h\022\025\n\021DATATYPES" +
"_COMMAND\020i\022\034\n\030GET_SERIALIZATION_FORMAT\020j" +
"\022\026\n\022GET_BULK_CACHEITEM\020k\022\021\n\rCONTAINS_BUL" +
"K\020l\022\027\n\023DATATYPE_MANAGEMENT\020m\022\020\n\014LIST_COM" +
"MAND\020n\022\026\n\022DICTIONARY_COMMAND\020o\022\023\n\017HASHSE" +
"T_COMMAND\020p\022\021\n\rQUEUE_COMMAND\020q\022\026\n\022COLLEC" +
"TION_COMMAND\020r\022\033\n\027COLLECTION_NOTIFICATIO" +
"N\020s\022\023\n\017COUNTER_COMMAND\020t\022\n\n\006MODULE\020u\022\r\n\t" +
"SURROGATE\020v\022\030\n\024MESSAGE_PUBLISH_BULK\020w\022(\n",
"$BRIDGE_POLL_FOR_RESUMING_REPLICATION\020x\022" +
"\022\n\016GETMODULESTATE\020y\022\022\n\016SETMODULESTATE\020zB" +
"\021B\017CommandProtocol"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_com_alachisoft_ncache_common_protobuf_Command_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_com_alachisoft_ncache_common_protobuf_Command_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_Command_descriptor,
new java.lang.String[] { "Type", "Version", "AddCommand", "AddDependencyCommand", "AddSyncDependencyCommand", "BulkAddCommand", "BulkGetCommand", "BulkInsertCommand", "BulkRemoveCommand", "ClearCommand", "ContainsCommand", "CountCommand", "DisposeCommand", "GetCacheItemCommand", "GetCommand", "GetCompactTypesCommand", "GetEnumeratorCommand", "GetGroupCommand", "GetHashmapCommand", "GetOptimalServerCommand", "GetThresholdSizeCommand", "GetTypeInfoMapCommand", "InitCommand", "InsertCommand", "RaiseCustomEventCommand", "RegisterKeyNotifCommand", "RegisterNotifCommand", "RemoveCommand", "RemoveGroupCommand", "SearchCommand", "GetTagCommand", "LockCommand", "UnlockCommand", "IsLockedCommand", "LockVerifyCommand", "UnRegisterKeyNotifCommand", "UnRegisterBulkKeyNotifCommand", "RegisterBulkKeyNotifCommand", "HybridBulkCommand", "GetLoggingInfoCommand", "CloseStreamCommand", "GetStreamLengthCommand", "OpenStreamCommand", "WriteToStreamCommand", "ReadFromStreamCommand", "BridgeGetStateTransferInfoCommand", "BridgeGetTransferableKeyListCommand", "BridgeHybridBulkCommand", "BridgeInitCommand", "BridgeSetTransferableKeyListCommand", "BridgeSignlEndOfStateTransferCommand", "RequestID", "CommandVersion", "BridgeSyncTimeCommand", "BridgeHeartBeatReceivedCommand", "RemoveByTagCommand", "BridgeMakeTargetCacheActivePassiveCommand", "UnRegisterCQCommand", "SearchCQCommand", "RegisterCQCommand", "GetKeysByTagCommand", "ClientLastViewId", "BulkDeleteCommand", "DeleteCommand", "IntendedRecipient", "GetNextChunkCommand", "GetGroupNextChunkCommand", "AddAttributeCommand", "GetEncryptionCommand", "GetRunningServersCommand", "SyncEventsCommand", "DeleteQueryCommand", "GetProductVersionCommand", "BridgeGetReplicatorStatusInfoCommand", "BridgeRequestStateTransferCommand", "GetServerMappingCommand", "InquiryRequestCommand", "IsRetryCommand", "MapReduceTaskCommand", "TaskCallbackCommand", "RunningTasksCommand", "TaskCancelCommand", "TaskProgressCommand", "NextRecordCommand", "CommandID", "GetCacheBindingCommand", "TaskEnumeratorCommand", "InvokeEntryProcessorCommand", "ExecuteReaderCommand", "GetReaderNextChunkCommand", "DisposeReaderCommand", "ExecuteReaderCQCommand", "GetExpirationCommand", "GetLCCommand", "CacheSecurityAuthorizationCommand", "PollCommand", "RegisterPollNotifCommand", "GetConnectedClientsCommand", "MethodOverload", "TouchCommand", "GetCacheManagementPortCommand", "GetTopicCommand", "SubscribeTopicCommand", "RemoveTopicCommand", "UnSubscribeTopicCommand", "MessagePublishCommand", "GetMessageCommand", "MesasgeAcknowledgmentCommand", "PingCommand", "MessageCountCommand", "DataTypes", "GetSerializationFormatCommand", "BulkGetCacheItemCommand", "ContainsBulkCommand", "ModuleCommand", "SurrogateCommand", "MessagePublishBulkCommand", "BridgePollForResumingReplication", "GetModuleStateCommand", "SetModuleStateCommand", },
com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.class,
com.alachisoft.ncache.common.protobuf.CommandProtocol.Command.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.alachisoft.ncache.common.protobuf.AddCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.AddDependencyCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.AddSyncDependencyCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BulkAddCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BulkGetCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BulkInsertCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BulkRemoveCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.ClearCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.CountCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.DisposeCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetCacheItemCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetCompactTypesCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetEnumeratorCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetGroupCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetHashmapCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetOptimalServerCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetThresholdSizeCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetTypeInfoMapCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.InitCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.InsertCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RaiseCustomEventCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RegisterKeyNotifCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RegisterBulkKeyNotifCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RegisterNotifCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RemoveCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RemoveGroupCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SearchCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetTagCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.LockCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.IsLockedCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.LockVerifyCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.UnRegisterKeyNotifCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.UnRegisterBulkKeyNotifCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetLoggingInfoCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.CloseStreamCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetStreamLengthCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.WriteToStreamCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.ReadFromStreamCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetStateTransferInfoCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetTransferableKeyListCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BridgeHybridBulkCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BridgeInitCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SetTransferableKeyListCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SignlEndOfStateTransferCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BridgeSyncTimeProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BridgeHeartBeatReceivedProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RemoveByTagCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BridgeMakeTargetCacheActivePassiveCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.UnRegisterCQCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RegisterCQCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetKeysByTagCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BulkDeleteCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.DeleteCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetNextChunkCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetGroupNextChunkCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.AddAttributeProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetEncryptionCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetLCCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetRunningServersCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SyncEventsCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetProductVersionCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetReplicatorStatusInfoCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetServerMappingCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RequestStateTransferCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetCacheBindingCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.MapReduceTaskCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.TaskCallbackCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.TaskCancelCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.TaskProgressCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetRunningTasksCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetNextRecordCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.TaskEnumeratorCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.InvokeEntryProcessorProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.InquiryRequestCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.ExecuteReaderCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetReaderNextChunkCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.DisposeReaderCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.ExecuteReaderCQCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetExpirationCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.PollCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RegisterPollingNotificationCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.CacheSecurityAuthorizationCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetConnectedClientsCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.TouchCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetTopicCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SubscribeTopicCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.RemoveTopicCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.UnSubscribeTopicCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetMessageCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.MesasgeAcknowledgmentCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetCacheManagementPortCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.PingCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.MessageCountCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.DataTypesProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetSerializationFormatCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BulkGetCacheItemCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SurrogateCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.ModuleCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.BridgePollForResumingReplicationProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.GetModuleStateCommandProtocol.getDescriptor(),
com.alachisoft.ncache.common.protobuf.SetModuleStateCommandProtocol.getDescriptor(),
}, assigner);
}
public static void internalForceInit() {}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy