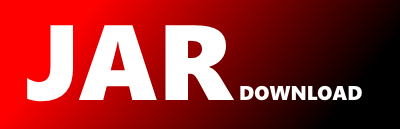
com.alachisoft.ncache.common.protobuf.DependencyProtocol Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Dependency.proto
package com.alachisoft.ncache.common.protobuf;
public final class DependencyProtocol {
private DependencyProtocol() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public static final class Dependency extends
com.google.protobuf.GeneratedMessage {
// Use Dependency.newBuilder() to construct.
private Dependency() {
initFields();
}
private Dependency(boolean noInit) {}
private static final Dependency defaultInstance;
public static Dependency getDefaultInstance() {
return defaultInstance;
}
public Dependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Dependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Dependency_fieldAccessorTable;
}
// repeated .com.alachisoft.ncache.common.protobuf.KeyDependency keyDep = 1;
public static final int KEYDEP_FIELD_NUMBER = 1;
private java.util.List keyDep_ =
java.util.Collections.emptyList();
public java.util.List getKeyDepList() {
return keyDep_;
}
public int getKeyDepCount() { return keyDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency getKeyDep(int index) {
return keyDep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.FileDependency fileDep = 2;
public static final int FILEDEP_FIELD_NUMBER = 2;
private java.util.List fileDep_ =
java.util.Collections.emptyList();
public java.util.List getFileDepList() {
return fileDep_;
}
public int getFileDepCount() { return fileDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency getFileDep(int index) {
return fileDep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.OracleDependency oracleDep = 3;
public static final int ORACLEDEP_FIELD_NUMBER = 3;
private java.util.List oracleDep_ =
java.util.Collections.emptyList();
public java.util.List getOracleDepList() {
return oracleDep_;
}
public int getOracleDepCount() { return oracleDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency getOracleDep(int index) {
return oracleDep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.YukonDependency yukonDep = 4;
public static final int YUKONDEP_FIELD_NUMBER = 4;
private java.util.List yukonDep_ =
java.util.Collections.emptyList();
public java.util.List getYukonDepList() {
return yukonDep_;
}
public int getYukonDepCount() { return yukonDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency getYukonDep(int index) {
return yukonDep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.Sql7Dependency sql7Dep = 5;
public static final int SQL7DEP_FIELD_NUMBER = 5;
private java.util.List sql7Dep_ =
java.util.Collections.emptyList();
public java.util.List getSql7DepList() {
return sql7Dep_;
}
public int getSql7DepCount() { return sql7Dep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency getSql7Dep(int index) {
return sql7Dep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.OleDbDependency oleDbDep = 6;
public static final int OLEDBDEP_FIELD_NUMBER = 6;
private java.util.List oleDbDep_ =
java.util.Collections.emptyList();
public java.util.List getOleDbDepList() {
return oleDbDep_;
}
public int getOleDbDepCount() { return oleDbDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency getOleDbDep(int index) {
return oleDbDep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.ExtensibleDependency xtDep = 7;
public static final int XTDEP_FIELD_NUMBER = 7;
private java.util.List xtDep_ =
java.util.Collections.emptyList();
public java.util.List getXtDepList() {
return xtDep_;
}
public int getXtDepCount() { return xtDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency getXtDep(int index) {
return xtDep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.NosDbDependency NosDep = 8;
public static final int NOSDEP_FIELD_NUMBER = 8;
private java.util.List nosDep_ =
java.util.Collections.emptyList();
public java.util.List getNosDepList() {
return nosDep_;
}
public int getNosDepCount() { return nosDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency getNosDep(int index) {
return nosDep_.get(index);
}
// repeated .com.alachisoft.ncache.common.protobuf.CustomDependency customDep = 9;
public static final int CUSTOMDEP_FIELD_NUMBER = 9;
private java.util.List customDep_ =
java.util.Collections.emptyList();
public java.util.List getCustomDepList() {
return customDep_;
}
public int getCustomDepCount() { return customDep_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency getCustomDep(int index) {
return customDep_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency element : getCustomDepList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency element : getKeyDepList()) {
output.writeMessage(1, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency element : getFileDepList()) {
output.writeMessage(2, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency element : getOracleDepList()) {
output.writeMessage(3, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency element : getYukonDepList()) {
output.writeMessage(4, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency element : getSql7DepList()) {
output.writeMessage(5, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency element : getOleDbDepList()) {
output.writeMessage(6, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency element : getXtDepList()) {
output.writeMessage(7, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency element : getNosDepList()) {
output.writeMessage(8, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency element : getCustomDepList()) {
output.writeMessage(9, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency element : getKeyDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency element : getFileDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency element : getOracleDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency element : getYukonDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency element : getSql7DepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency element : getOleDbDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency element : getXtDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency element : getNosDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, element);
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency element : getCustomDepList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.keyDep_ != java.util.Collections.EMPTY_LIST) {
result.keyDep_ =
java.util.Collections.unmodifiableList(result.keyDep_);
}
if (result.fileDep_ != java.util.Collections.EMPTY_LIST) {
result.fileDep_ =
java.util.Collections.unmodifiableList(result.fileDep_);
}
if (result.oracleDep_ != java.util.Collections.EMPTY_LIST) {
result.oracleDep_ =
java.util.Collections.unmodifiableList(result.oracleDep_);
}
if (result.yukonDep_ != java.util.Collections.EMPTY_LIST) {
result.yukonDep_ =
java.util.Collections.unmodifiableList(result.yukonDep_);
}
if (result.sql7Dep_ != java.util.Collections.EMPTY_LIST) {
result.sql7Dep_ =
java.util.Collections.unmodifiableList(result.sql7Dep_);
}
if (result.oleDbDep_ != java.util.Collections.EMPTY_LIST) {
result.oleDbDep_ =
java.util.Collections.unmodifiableList(result.oleDbDep_);
}
if (result.xtDep_ != java.util.Collections.EMPTY_LIST) {
result.xtDep_ =
java.util.Collections.unmodifiableList(result.xtDep_);
}
if (result.nosDep_ != java.util.Collections.EMPTY_LIST) {
result.nosDep_ =
java.util.Collections.unmodifiableList(result.nosDep_);
}
if (result.customDep_ != java.util.Collections.EMPTY_LIST) {
result.customDep_ =
java.util.Collections.unmodifiableList(result.customDep_);
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency.getDefaultInstance()) return this;
if (!other.keyDep_.isEmpty()) {
if (result.keyDep_.isEmpty()) {
result.keyDep_ = new java.util.ArrayList();
}
result.keyDep_.addAll(other.keyDep_);
}
if (!other.fileDep_.isEmpty()) {
if (result.fileDep_.isEmpty()) {
result.fileDep_ = new java.util.ArrayList();
}
result.fileDep_.addAll(other.fileDep_);
}
if (!other.oracleDep_.isEmpty()) {
if (result.oracleDep_.isEmpty()) {
result.oracleDep_ = new java.util.ArrayList();
}
result.oracleDep_.addAll(other.oracleDep_);
}
if (!other.yukonDep_.isEmpty()) {
if (result.yukonDep_.isEmpty()) {
result.yukonDep_ = new java.util.ArrayList();
}
result.yukonDep_.addAll(other.yukonDep_);
}
if (!other.sql7Dep_.isEmpty()) {
if (result.sql7Dep_.isEmpty()) {
result.sql7Dep_ = new java.util.ArrayList();
}
result.sql7Dep_.addAll(other.sql7Dep_);
}
if (!other.oleDbDep_.isEmpty()) {
if (result.oleDbDep_.isEmpty()) {
result.oleDbDep_ = new java.util.ArrayList();
}
result.oleDbDep_.addAll(other.oleDbDep_);
}
if (!other.xtDep_.isEmpty()) {
if (result.xtDep_.isEmpty()) {
result.xtDep_ = new java.util.ArrayList();
}
result.xtDep_.addAll(other.xtDep_);
}
if (!other.nosDep_.isEmpty()) {
if (result.nosDep_.isEmpty()) {
result.nosDep_ = new java.util.ArrayList();
}
result.nosDep_.addAll(other.nosDep_);
}
if (!other.customDep_.isEmpty()) {
if (result.customDep_.isEmpty()) {
result.customDep_ = new java.util.ArrayList();
}
result.customDep_.addAll(other.customDep_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addKeyDep(subBuilder.buildPartial());
break;
}
case 18: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addFileDep(subBuilder.buildPartial());
break;
}
case 26: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addOracleDep(subBuilder.buildPartial());
break;
}
case 34: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addYukonDep(subBuilder.buildPartial());
break;
}
case 42: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addSql7Dep(subBuilder.buildPartial());
break;
}
case 50: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addOleDbDep(subBuilder.buildPartial());
break;
}
case 58: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addXtDep(subBuilder.buildPartial());
break;
}
case 66: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addNosDep(subBuilder.buildPartial());
break;
}
case 74: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addCustomDep(subBuilder.buildPartial());
break;
}
}
}
}
// repeated .com.alachisoft.ncache.common.protobuf.KeyDependency keyDep = 1;
public java.util.List getKeyDepList() {
return java.util.Collections.unmodifiableList(result.keyDep_);
}
public int getKeyDepCount() {
return result.getKeyDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency getKeyDep(int index) {
return result.getKeyDep(index);
}
public Builder setKeyDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.keyDep_.set(index, value);
return this;
}
public Builder setKeyDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.Builder builderForValue) {
result.keyDep_.set(index, builderForValue.build());
return this;
}
public Builder addKeyDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.keyDep_.isEmpty()) {
result.keyDep_ = new java.util.ArrayList();
}
result.keyDep_.add(value);
return this;
}
public Builder addKeyDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.Builder builderForValue) {
if (result.keyDep_.isEmpty()) {
result.keyDep_ = new java.util.ArrayList();
}
result.keyDep_.add(builderForValue.build());
return this;
}
public Builder addAllKeyDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency> values) {
if (result.keyDep_.isEmpty()) {
result.keyDep_ = new java.util.ArrayList();
}
super.addAll(values, result.keyDep_);
return this;
}
public Builder clearKeyDep() {
result.keyDep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.FileDependency fileDep = 2;
public java.util.List getFileDepList() {
return java.util.Collections.unmodifiableList(result.fileDep_);
}
public int getFileDepCount() {
return result.getFileDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency getFileDep(int index) {
return result.getFileDep(index);
}
public Builder setFileDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.fileDep_.set(index, value);
return this;
}
public Builder setFileDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.Builder builderForValue) {
result.fileDep_.set(index, builderForValue.build());
return this;
}
public Builder addFileDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.fileDep_.isEmpty()) {
result.fileDep_ = new java.util.ArrayList();
}
result.fileDep_.add(value);
return this;
}
public Builder addFileDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.Builder builderForValue) {
if (result.fileDep_.isEmpty()) {
result.fileDep_ = new java.util.ArrayList();
}
result.fileDep_.add(builderForValue.build());
return this;
}
public Builder addAllFileDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency> values) {
if (result.fileDep_.isEmpty()) {
result.fileDep_ = new java.util.ArrayList();
}
super.addAll(values, result.fileDep_);
return this;
}
public Builder clearFileDep() {
result.fileDep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.OracleDependency oracleDep = 3;
public java.util.List getOracleDepList() {
return java.util.Collections.unmodifiableList(result.oracleDep_);
}
public int getOracleDepCount() {
return result.getOracleDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency getOracleDep(int index) {
return result.getOracleDep(index);
}
public Builder setOracleDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.oracleDep_.set(index, value);
return this;
}
public Builder setOracleDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.Builder builderForValue) {
result.oracleDep_.set(index, builderForValue.build());
return this;
}
public Builder addOracleDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.oracleDep_.isEmpty()) {
result.oracleDep_ = new java.util.ArrayList();
}
result.oracleDep_.add(value);
return this;
}
public Builder addOracleDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.Builder builderForValue) {
if (result.oracleDep_.isEmpty()) {
result.oracleDep_ = new java.util.ArrayList();
}
result.oracleDep_.add(builderForValue.build());
return this;
}
public Builder addAllOracleDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency> values) {
if (result.oracleDep_.isEmpty()) {
result.oracleDep_ = new java.util.ArrayList();
}
super.addAll(values, result.oracleDep_);
return this;
}
public Builder clearOracleDep() {
result.oracleDep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.YukonDependency yukonDep = 4;
public java.util.List getYukonDepList() {
return java.util.Collections.unmodifiableList(result.yukonDep_);
}
public int getYukonDepCount() {
return result.getYukonDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency getYukonDep(int index) {
return result.getYukonDep(index);
}
public Builder setYukonDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.yukonDep_.set(index, value);
return this;
}
public Builder setYukonDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.Builder builderForValue) {
result.yukonDep_.set(index, builderForValue.build());
return this;
}
public Builder addYukonDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.yukonDep_.isEmpty()) {
result.yukonDep_ = new java.util.ArrayList();
}
result.yukonDep_.add(value);
return this;
}
public Builder addYukonDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.Builder builderForValue) {
if (result.yukonDep_.isEmpty()) {
result.yukonDep_ = new java.util.ArrayList();
}
result.yukonDep_.add(builderForValue.build());
return this;
}
public Builder addAllYukonDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency> values) {
if (result.yukonDep_.isEmpty()) {
result.yukonDep_ = new java.util.ArrayList();
}
super.addAll(values, result.yukonDep_);
return this;
}
public Builder clearYukonDep() {
result.yukonDep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.Sql7Dependency sql7Dep = 5;
public java.util.List getSql7DepList() {
return java.util.Collections.unmodifiableList(result.sql7Dep_);
}
public int getSql7DepCount() {
return result.getSql7DepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency getSql7Dep(int index) {
return result.getSql7Dep(index);
}
public Builder setSql7Dep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency value) {
if (value == null) {
throw new NullPointerException();
}
result.sql7Dep_.set(index, value);
return this;
}
public Builder setSql7Dep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.Builder builderForValue) {
result.sql7Dep_.set(index, builderForValue.build());
return this;
}
public Builder addSql7Dep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.sql7Dep_.isEmpty()) {
result.sql7Dep_ = new java.util.ArrayList();
}
result.sql7Dep_.add(value);
return this;
}
public Builder addSql7Dep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.Builder builderForValue) {
if (result.sql7Dep_.isEmpty()) {
result.sql7Dep_ = new java.util.ArrayList();
}
result.sql7Dep_.add(builderForValue.build());
return this;
}
public Builder addAllSql7Dep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency> values) {
if (result.sql7Dep_.isEmpty()) {
result.sql7Dep_ = new java.util.ArrayList();
}
super.addAll(values, result.sql7Dep_);
return this;
}
public Builder clearSql7Dep() {
result.sql7Dep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.OleDbDependency oleDbDep = 6;
public java.util.List getOleDbDepList() {
return java.util.Collections.unmodifiableList(result.oleDbDep_);
}
public int getOleDbDepCount() {
return result.getOleDbDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency getOleDbDep(int index) {
return result.getOleDbDep(index);
}
public Builder setOleDbDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.oleDbDep_.set(index, value);
return this;
}
public Builder setOleDbDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.Builder builderForValue) {
result.oleDbDep_.set(index, builderForValue.build());
return this;
}
public Builder addOleDbDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.oleDbDep_.isEmpty()) {
result.oleDbDep_ = new java.util.ArrayList();
}
result.oleDbDep_.add(value);
return this;
}
public Builder addOleDbDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.Builder builderForValue) {
if (result.oleDbDep_.isEmpty()) {
result.oleDbDep_ = new java.util.ArrayList();
}
result.oleDbDep_.add(builderForValue.build());
return this;
}
public Builder addAllOleDbDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency> values) {
if (result.oleDbDep_.isEmpty()) {
result.oleDbDep_ = new java.util.ArrayList();
}
super.addAll(values, result.oleDbDep_);
return this;
}
public Builder clearOleDbDep() {
result.oleDbDep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.ExtensibleDependency xtDep = 7;
public java.util.List getXtDepList() {
return java.util.Collections.unmodifiableList(result.xtDep_);
}
public int getXtDepCount() {
return result.getXtDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency getXtDep(int index) {
return result.getXtDep(index);
}
public Builder setXtDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.xtDep_.set(index, value);
return this;
}
public Builder setXtDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.Builder builderForValue) {
result.xtDep_.set(index, builderForValue.build());
return this;
}
public Builder addXtDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.xtDep_.isEmpty()) {
result.xtDep_ = new java.util.ArrayList();
}
result.xtDep_.add(value);
return this;
}
public Builder addXtDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.Builder builderForValue) {
if (result.xtDep_.isEmpty()) {
result.xtDep_ = new java.util.ArrayList();
}
result.xtDep_.add(builderForValue.build());
return this;
}
public Builder addAllXtDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency> values) {
if (result.xtDep_.isEmpty()) {
result.xtDep_ = new java.util.ArrayList();
}
super.addAll(values, result.xtDep_);
return this;
}
public Builder clearXtDep() {
result.xtDep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.NosDbDependency NosDep = 8;
public java.util.List getNosDepList() {
return java.util.Collections.unmodifiableList(result.nosDep_);
}
public int getNosDepCount() {
return result.getNosDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency getNosDep(int index) {
return result.getNosDep(index);
}
public Builder setNosDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.nosDep_.set(index, value);
return this;
}
public Builder setNosDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.Builder builderForValue) {
result.nosDep_.set(index, builderForValue.build());
return this;
}
public Builder addNosDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.nosDep_.isEmpty()) {
result.nosDep_ = new java.util.ArrayList();
}
result.nosDep_.add(value);
return this;
}
public Builder addNosDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.Builder builderForValue) {
if (result.nosDep_.isEmpty()) {
result.nosDep_ = new java.util.ArrayList();
}
result.nosDep_.add(builderForValue.build());
return this;
}
public Builder addAllNosDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency> values) {
if (result.nosDep_.isEmpty()) {
result.nosDep_ = new java.util.ArrayList();
}
super.addAll(values, result.nosDep_);
return this;
}
public Builder clearNosDep() {
result.nosDep_ = java.util.Collections.emptyList();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.CustomDependency customDep = 9;
public java.util.List getCustomDepList() {
return java.util.Collections.unmodifiableList(result.customDep_);
}
public int getCustomDepCount() {
return result.getCustomDepCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency getCustomDep(int index) {
return result.getCustomDep(index);
}
public Builder setCustomDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency value) {
if (value == null) {
throw new NullPointerException();
}
result.customDep_.set(index, value);
return this;
}
public Builder setCustomDep(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.Builder builderForValue) {
result.customDep_.set(index, builderForValue.build());
return this;
}
public Builder addCustomDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency value) {
if (value == null) {
throw new NullPointerException();
}
if (result.customDep_.isEmpty()) {
result.customDep_ = new java.util.ArrayList();
}
result.customDep_.add(value);
return this;
}
public Builder addCustomDep(com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.Builder builderForValue) {
if (result.customDep_.isEmpty()) {
result.customDep_ = new java.util.ArrayList();
}
result.customDep_.add(builderForValue.build());
return this;
}
public Builder addAllCustomDep(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency> values) {
if (result.customDep_.isEmpty()) {
result.customDep_ = new java.util.ArrayList();
}
super.addAll(values, result.customDep_);
return this;
}
public Builder clearCustomDep() {
result.customDep_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.Dependency)
}
static {
defaultInstance = new Dependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.Dependency)
}
public static final class KeyDependency extends
com.google.protobuf.GeneratedMessage {
// Use KeyDependency.newBuilder() to construct.
private KeyDependency() {
initFields();
}
private KeyDependency(boolean noInit) {}
private static final KeyDependency defaultInstance;
public static KeyDependency getDefaultInstance() {
return defaultInstance;
}
public KeyDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_KeyDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_KeyDependency_fieldAccessorTable;
}
// repeated string keys = 1;
public static final int KEYS_FIELD_NUMBER = 1;
private java.util.List keys_ =
java.util.Collections.emptyList();
public java.util.List getKeysList() {
return keys_;
}
public int getKeysCount() { return keys_.size(); }
public java.lang.String getKeys(int index) {
return keys_.get(index);
}
// optional int64 startAfter = 2;
public static final int STARTAFTER_FIELD_NUMBER = 2;
private boolean hasStartAfter;
private long startAfter_ = 0L;
public boolean hasStartAfter() { return hasStartAfter; }
public long getStartAfter() { return startAfter_; }
// optional int32 keyDependencyType = 3;
public static final int KEYDEPENDENCYTYPE_FIELD_NUMBER = 3;
private boolean hasKeyDependencyType;
private int keyDependencyType_ = 0;
public boolean hasKeyDependencyType() { return hasKeyDependencyType; }
public int getKeyDependencyType() { return keyDependencyType_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (java.lang.String element : getKeysList()) {
output.writeString(1, element);
}
if (hasStartAfter()) {
output.writeInt64(2, getStartAfter());
}
if (hasKeyDependencyType()) {
output.writeInt32(3, getKeyDependencyType());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (java.lang.String element : getKeysList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeStringSizeNoTag(element);
}
size += dataSize;
size += 1 * getKeysList().size();
}
if (hasStartAfter()) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, getStartAfter());
}
if (hasKeyDependencyType()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, getKeyDependencyType());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.keys_ != java.util.Collections.EMPTY_LIST) {
result.keys_ =
java.util.Collections.unmodifiableList(result.keys_);
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.getDefaultInstance()) return this;
if (!other.keys_.isEmpty()) {
if (result.keys_.isEmpty()) {
result.keys_ = new java.util.ArrayList();
}
result.keys_.addAll(other.keys_);
}
if (other.hasStartAfter()) {
setStartAfter(other.getStartAfter());
}
if (other.hasKeyDependencyType()) {
setKeyDependencyType(other.getKeyDependencyType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
addKeys(input.readString());
break;
}
case 16: {
setStartAfter(input.readInt64());
break;
}
case 24: {
setKeyDependencyType(input.readInt32());
break;
}
}
}
}
// repeated string keys = 1;
public java.util.List getKeysList() {
return java.util.Collections.unmodifiableList(result.keys_);
}
public int getKeysCount() {
return result.getKeysCount();
}
public java.lang.String getKeys(int index) {
return result.getKeys(index);
}
public Builder setKeys(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.keys_.set(index, value);
return this;
}
public Builder addKeys(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
if (result.keys_.isEmpty()) {
result.keys_ = new java.util.ArrayList();
}
result.keys_.add(value);
return this;
}
public Builder addAllKeys(
java.lang.Iterable extends java.lang.String> values) {
if (result.keys_.isEmpty()) {
result.keys_ = new java.util.ArrayList();
}
super.addAll(values, result.keys_);
return this;
}
public Builder clearKeys() {
result.keys_ = java.util.Collections.emptyList();
return this;
}
// optional int64 startAfter = 2;
public boolean hasStartAfter() {
return result.hasStartAfter();
}
public long getStartAfter() {
return result.getStartAfter();
}
public Builder setStartAfter(long value) {
result.hasStartAfter = true;
result.startAfter_ = value;
return this;
}
public Builder clearStartAfter() {
result.hasStartAfter = false;
result.startAfter_ = 0L;
return this;
}
// optional int32 keyDependencyType = 3;
public boolean hasKeyDependencyType() {
return result.hasKeyDependencyType();
}
public int getKeyDependencyType() {
return result.getKeyDependencyType();
}
public Builder setKeyDependencyType(int value) {
result.hasKeyDependencyType = true;
result.keyDependencyType_ = value;
return this;
}
public Builder clearKeyDependencyType() {
result.hasKeyDependencyType = false;
result.keyDependencyType_ = 0;
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.KeyDependency)
}
static {
defaultInstance = new KeyDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.KeyDependency)
}
public static final class FileDependency extends
com.google.protobuf.GeneratedMessage {
// Use FileDependency.newBuilder() to construct.
private FileDependency() {
initFields();
}
private FileDependency(boolean noInit) {}
private static final FileDependency defaultInstance;
public static FileDependency getDefaultInstance() {
return defaultInstance;
}
public FileDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_FileDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_FileDependency_fieldAccessorTable;
}
// repeated string filePaths = 1;
public static final int FILEPATHS_FIELD_NUMBER = 1;
private java.util.List filePaths_ =
java.util.Collections.emptyList();
public java.util.List getFilePathsList() {
return filePaths_;
}
public int getFilePathsCount() { return filePaths_.size(); }
public java.lang.String getFilePaths(int index) {
return filePaths_.get(index);
}
// optional int64 startAfter = 2;
public static final int STARTAFTER_FIELD_NUMBER = 2;
private boolean hasStartAfter;
private long startAfter_ = 0L;
public boolean hasStartAfter() { return hasStartAfter; }
public long getStartAfter() { return startAfter_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (java.lang.String element : getFilePathsList()) {
output.writeString(1, element);
}
if (hasStartAfter()) {
output.writeInt64(2, getStartAfter());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (java.lang.String element : getFilePathsList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeStringSizeNoTag(element);
}
size += dataSize;
size += 1 * getFilePathsList().size();
}
if (hasStartAfter()) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, getStartAfter());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.filePaths_ != java.util.Collections.EMPTY_LIST) {
result.filePaths_ =
java.util.Collections.unmodifiableList(result.filePaths_);
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.getDefaultInstance()) return this;
if (!other.filePaths_.isEmpty()) {
if (result.filePaths_.isEmpty()) {
result.filePaths_ = new java.util.ArrayList();
}
result.filePaths_.addAll(other.filePaths_);
}
if (other.hasStartAfter()) {
setStartAfter(other.getStartAfter());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
addFilePaths(input.readString());
break;
}
case 16: {
setStartAfter(input.readInt64());
break;
}
}
}
}
// repeated string filePaths = 1;
public java.util.List getFilePathsList() {
return java.util.Collections.unmodifiableList(result.filePaths_);
}
public int getFilePathsCount() {
return result.getFilePathsCount();
}
public java.lang.String getFilePaths(int index) {
return result.getFilePaths(index);
}
public Builder setFilePaths(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.filePaths_.set(index, value);
return this;
}
public Builder addFilePaths(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
if (result.filePaths_.isEmpty()) {
result.filePaths_ = new java.util.ArrayList();
}
result.filePaths_.add(value);
return this;
}
public Builder addAllFilePaths(
java.lang.Iterable extends java.lang.String> values) {
if (result.filePaths_.isEmpty()) {
result.filePaths_ = new java.util.ArrayList();
}
super.addAll(values, result.filePaths_);
return this;
}
public Builder clearFilePaths() {
result.filePaths_ = java.util.Collections.emptyList();
return this;
}
// optional int64 startAfter = 2;
public boolean hasStartAfter() {
return result.hasStartAfter();
}
public long getStartAfter() {
return result.getStartAfter();
}
public Builder setStartAfter(long value) {
result.hasStartAfter = true;
result.startAfter_ = value;
return this;
}
public Builder clearStartAfter() {
result.hasStartAfter = false;
result.startAfter_ = 0L;
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.FileDependency)
}
static {
defaultInstance = new FileDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.FileDependency)
}
public static final class OracleDependency extends
com.google.protobuf.GeneratedMessage {
// Use OracleDependency.newBuilder() to construct.
private OracleDependency() {
initFields();
}
private OracleDependency(boolean noInit) {}
private static final OracleDependency defaultInstance;
public static OracleDependency getDefaultInstance() {
return defaultInstance;
}
public OracleDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OracleDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OracleDependency_fieldAccessorTable;
}
// optional string connectionString = 1;
public static final int CONNECTIONSTRING_FIELD_NUMBER = 1;
private boolean hasConnectionString;
private java.lang.String connectionString_ = "";
public boolean hasConnectionString() { return hasConnectionString; }
public java.lang.String getConnectionString() { return connectionString_; }
// optional string query = 2;
public static final int QUERY_FIELD_NUMBER = 2;
private boolean hasQuery;
private java.lang.String query_ = "";
public boolean hasQuery() { return hasQuery; }
public java.lang.String getQuery() { return query_; }
// optional int32 commandType = 3;
public static final int COMMANDTYPE_FIELD_NUMBER = 3;
private boolean hasCommandType;
private int commandType_ = 0;
public boolean hasCommandType() { return hasCommandType; }
public int getCommandType() { return commandType_; }
// repeated .com.alachisoft.ncache.common.protobuf.OracleParam param = 4;
public static final int PARAM_FIELD_NUMBER = 4;
private java.util.List param_ =
java.util.Collections.emptyList();
public java.util.List getParamList() {
return param_;
}
public int getParamCount() { return param_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam getParam(int index) {
return param_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasConnectionString()) {
output.writeString(1, getConnectionString());
}
if (hasQuery()) {
output.writeString(2, getQuery());
}
if (hasCommandType()) {
output.writeInt32(3, getCommandType());
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam element : getParamList()) {
output.writeMessage(4, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasConnectionString()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getConnectionString());
}
if (hasQuery()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getQuery());
}
if (hasCommandType()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, getCommandType());
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam element : getParamList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.param_ != java.util.Collections.EMPTY_LIST) {
result.param_ =
java.util.Collections.unmodifiableList(result.param_);
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.getDefaultInstance()) return this;
if (other.hasConnectionString()) {
setConnectionString(other.getConnectionString());
}
if (other.hasQuery()) {
setQuery(other.getQuery());
}
if (other.hasCommandType()) {
setCommandType(other.getCommandType());
}
if (!other.param_.isEmpty()) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.addAll(other.param_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setConnectionString(input.readString());
break;
}
case 18: {
setQuery(input.readString());
break;
}
case 24: {
setCommandType(input.readInt32());
break;
}
case 34: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addParam(subBuilder.buildPartial());
break;
}
}
}
}
// optional string connectionString = 1;
public boolean hasConnectionString() {
return result.hasConnectionString();
}
public java.lang.String getConnectionString() {
return result.getConnectionString();
}
public Builder setConnectionString(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasConnectionString = true;
result.connectionString_ = value;
return this;
}
public Builder clearConnectionString() {
result.hasConnectionString = false;
result.connectionString_ = getDefaultInstance().getConnectionString();
return this;
}
// optional string query = 2;
public boolean hasQuery() {
return result.hasQuery();
}
public java.lang.String getQuery() {
return result.getQuery();
}
public Builder setQuery(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasQuery = true;
result.query_ = value;
return this;
}
public Builder clearQuery() {
result.hasQuery = false;
result.query_ = getDefaultInstance().getQuery();
return this;
}
// optional int32 commandType = 3;
public boolean hasCommandType() {
return result.hasCommandType();
}
public int getCommandType() {
return result.getCommandType();
}
public Builder setCommandType(int value) {
result.hasCommandType = true;
result.commandType_ = value;
return this;
}
public Builder clearCommandType() {
result.hasCommandType = false;
result.commandType_ = 0;
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.OracleParam param = 4;
public java.util.List getParamList() {
return java.util.Collections.unmodifiableList(result.param_);
}
public int getParamCount() {
return result.getParamCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam getParam(int index) {
return result.getParam(index);
}
public Builder setParam(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam value) {
if (value == null) {
throw new NullPointerException();
}
result.param_.set(index, value);
return this;
}
public Builder setParam(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.Builder builderForValue) {
result.param_.set(index, builderForValue.build());
return this;
}
public Builder addParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam value) {
if (value == null) {
throw new NullPointerException();
}
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.add(value);
return this;
}
public Builder addParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.Builder builderForValue) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.add(builderForValue.build());
return this;
}
public Builder addAllParam(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam> values) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
super.addAll(values, result.param_);
return this;
}
public Builder clearParam() {
result.param_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.OracleDependency)
}
static {
defaultInstance = new OracleDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.OracleDependency)
}
public static final class OracleParam extends
com.google.protobuf.GeneratedMessage {
// Use OracleParam.newBuilder() to construct.
private OracleParam() {
initFields();
}
private OracleParam(boolean noInit) {}
private static final OracleParam defaultInstance;
public static OracleParam getDefaultInstance() {
return defaultInstance;
}
public OracleParam getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OracleParam_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OracleParam_fieldAccessorTable;
}
// optional string key = 1;
public static final int KEY_FIELD_NUMBER = 1;
private boolean hasKey;
private java.lang.String key_ = "";
public boolean hasKey() { return hasKey; }
public java.lang.String getKey() { return key_; }
// optional .com.alachisoft.ncache.common.protobuf.OracleCommandParam cmdParam = 2;
public static final int CMDPARAM_FIELD_NUMBER = 2;
private boolean hasCmdParam;
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam cmdParam_;
public boolean hasCmdParam() { return hasCmdParam; }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam getCmdParam() { return cmdParam_; }
private void initFields() {
cmdParam_ = com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.getDefaultInstance();
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasKey()) {
output.writeString(1, getKey());
}
if (hasCmdParam()) {
output.writeMessage(2, getCmdParam());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasKey()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getKey());
}
if (hasCmdParam()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCmdParam());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasCmdParam()) {
mergeCmdParam(other.getCmdParam());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setKey(input.readString());
break;
}
case 18: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.newBuilder();
if (hasCmdParam()) {
subBuilder.mergeFrom(getCmdParam());
}
input.readMessage(subBuilder, extensionRegistry);
setCmdParam(subBuilder.buildPartial());
break;
}
}
}
}
// optional string key = 1;
public boolean hasKey() {
return result.hasKey();
}
public java.lang.String getKey() {
return result.getKey();
}
public Builder setKey(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasKey = true;
result.key_ = value;
return this;
}
public Builder clearKey() {
result.hasKey = false;
result.key_ = getDefaultInstance().getKey();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.OracleCommandParam cmdParam = 2;
public boolean hasCmdParam() {
return result.hasCmdParam();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam getCmdParam() {
return result.getCmdParam();
}
public Builder setCmdParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam value) {
if (value == null) {
throw new NullPointerException();
}
result.hasCmdParam = true;
result.cmdParam_ = value;
return this;
}
public Builder setCmdParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.Builder builderForValue) {
result.hasCmdParam = true;
result.cmdParam_ = builderForValue.build();
return this;
}
public Builder mergeCmdParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam value) {
if (result.hasCmdParam() &&
result.cmdParam_ != com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.getDefaultInstance()) {
result.cmdParam_ =
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.newBuilder(result.cmdParam_).mergeFrom(value).buildPartial();
} else {
result.cmdParam_ = value;
}
result.hasCmdParam = true;
return this;
}
public Builder clearCmdParam() {
result.hasCmdParam = false;
result.cmdParam_ = com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.getDefaultInstance();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.OracleParam)
}
static {
defaultInstance = new OracleParam(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.OracleParam)
}
public static final class OracleCommandParam extends
com.google.protobuf.GeneratedMessage {
// Use OracleCommandParam.newBuilder() to construct.
private OracleCommandParam() {
initFields();
}
private OracleCommandParam(boolean noInit) {}
private static final OracleCommandParam defaultInstance;
public static OracleCommandParam getDefaultInstance() {
return defaultInstance;
}
public OracleCommandParam getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OracleCommandParam_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OracleCommandParam_fieldAccessorTable;
}
// optional int32 dbType = 1;
public static final int DBTYPE_FIELD_NUMBER = 1;
private boolean hasDbType;
private int dbType_ = 0;
public boolean hasDbType() { return hasDbType; }
public int getDbType() { return dbType_; }
// optional int32 direction = 2;
public static final int DIRECTION_FIELD_NUMBER = 2;
private boolean hasDirection;
private int direction_ = 0;
public boolean hasDirection() { return hasDirection; }
public int getDirection() { return direction_; }
// optional string value = 3;
public static final int VALUE_FIELD_NUMBER = 3;
private boolean hasValue;
private java.lang.String value_ = "";
public boolean hasValue() { return hasValue; }
public java.lang.String getValue() { return value_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasDbType()) {
output.writeInt32(1, getDbType());
}
if (hasDirection()) {
output.writeInt32(2, getDirection());
}
if (hasValue()) {
output.writeString(3, getValue());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasDbType()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, getDbType());
}
if (hasDirection()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, getDirection());
}
if (hasValue()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(3, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.getDefaultInstance()) return this;
if (other.hasDbType()) {
setDbType(other.getDbType());
}
if (other.hasDirection()) {
setDirection(other.getDirection());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 8: {
setDbType(input.readInt32());
break;
}
case 16: {
setDirection(input.readInt32());
break;
}
case 26: {
setValue(input.readString());
break;
}
}
}
}
// optional int32 dbType = 1;
public boolean hasDbType() {
return result.hasDbType();
}
public int getDbType() {
return result.getDbType();
}
public Builder setDbType(int value) {
result.hasDbType = true;
result.dbType_ = value;
return this;
}
public Builder clearDbType() {
result.hasDbType = false;
result.dbType_ = 0;
return this;
}
// optional int32 direction = 2;
public boolean hasDirection() {
return result.hasDirection();
}
public int getDirection() {
return result.getDirection();
}
public Builder setDirection(int value) {
result.hasDirection = true;
result.direction_ = value;
return this;
}
public Builder clearDirection() {
result.hasDirection = false;
result.direction_ = 0;
return this;
}
// optional string value = 3;
public boolean hasValue() {
return result.hasValue();
}
public java.lang.String getValue() {
return result.getValue();
}
public Builder setValue(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasValue = true;
result.value_ = value;
return this;
}
public Builder clearValue() {
result.hasValue = false;
result.value_ = getDefaultInstance().getValue();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.OracleCommandParam)
}
static {
defaultInstance = new OracleCommandParam(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.OracleCommandParam)
}
public static final class YukonDependency extends
com.google.protobuf.GeneratedMessage {
// Use YukonDependency.newBuilder() to construct.
private YukonDependency() {
initFields();
}
private YukonDependency(boolean noInit) {}
private static final YukonDependency defaultInstance;
public static YukonDependency getDefaultInstance() {
return defaultInstance;
}
public YukonDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_YukonDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_YukonDependency_fieldAccessorTable;
}
// optional string connectionString = 1;
public static final int CONNECTIONSTRING_FIELD_NUMBER = 1;
private boolean hasConnectionString;
private java.lang.String connectionString_ = "";
public boolean hasConnectionString() { return hasConnectionString; }
public java.lang.String getConnectionString() { return connectionString_; }
// optional string query = 2;
public static final int QUERY_FIELD_NUMBER = 2;
private boolean hasQuery;
private java.lang.String query_ = "";
public boolean hasQuery() { return hasQuery; }
public java.lang.String getQuery() { return query_; }
// optional int32 commandType = 3;
public static final int COMMANDTYPE_FIELD_NUMBER = 3;
private boolean hasCommandType;
private int commandType_ = 0;
public boolean hasCommandType() { return hasCommandType; }
public int getCommandType() { return commandType_; }
// repeated .com.alachisoft.ncache.common.protobuf.YukonParam param = 4;
public static final int PARAM_FIELD_NUMBER = 4;
private java.util.List param_ =
java.util.Collections.emptyList();
public java.util.List getParamList() {
return param_;
}
public int getParamCount() { return param_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam getParam(int index) {
return param_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasConnectionString()) {
output.writeString(1, getConnectionString());
}
if (hasQuery()) {
output.writeString(2, getQuery());
}
if (hasCommandType()) {
output.writeInt32(3, getCommandType());
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam element : getParamList()) {
output.writeMessage(4, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasConnectionString()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getConnectionString());
}
if (hasQuery()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getQuery());
}
if (hasCommandType()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, getCommandType());
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam element : getParamList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.param_ != java.util.Collections.EMPTY_LIST) {
result.param_ =
java.util.Collections.unmodifiableList(result.param_);
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.getDefaultInstance()) return this;
if (other.hasConnectionString()) {
setConnectionString(other.getConnectionString());
}
if (other.hasQuery()) {
setQuery(other.getQuery());
}
if (other.hasCommandType()) {
setCommandType(other.getCommandType());
}
if (!other.param_.isEmpty()) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.addAll(other.param_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setConnectionString(input.readString());
break;
}
case 18: {
setQuery(input.readString());
break;
}
case 24: {
setCommandType(input.readInt32());
break;
}
case 34: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addParam(subBuilder.buildPartial());
break;
}
}
}
}
// optional string connectionString = 1;
public boolean hasConnectionString() {
return result.hasConnectionString();
}
public java.lang.String getConnectionString() {
return result.getConnectionString();
}
public Builder setConnectionString(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasConnectionString = true;
result.connectionString_ = value;
return this;
}
public Builder clearConnectionString() {
result.hasConnectionString = false;
result.connectionString_ = getDefaultInstance().getConnectionString();
return this;
}
// optional string query = 2;
public boolean hasQuery() {
return result.hasQuery();
}
public java.lang.String getQuery() {
return result.getQuery();
}
public Builder setQuery(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasQuery = true;
result.query_ = value;
return this;
}
public Builder clearQuery() {
result.hasQuery = false;
result.query_ = getDefaultInstance().getQuery();
return this;
}
// optional int32 commandType = 3;
public boolean hasCommandType() {
return result.hasCommandType();
}
public int getCommandType() {
return result.getCommandType();
}
public Builder setCommandType(int value) {
result.hasCommandType = true;
result.commandType_ = value;
return this;
}
public Builder clearCommandType() {
result.hasCommandType = false;
result.commandType_ = 0;
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.YukonParam param = 4;
public java.util.List getParamList() {
return java.util.Collections.unmodifiableList(result.param_);
}
public int getParamCount() {
return result.getParamCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam getParam(int index) {
return result.getParam(index);
}
public Builder setParam(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam value) {
if (value == null) {
throw new NullPointerException();
}
result.param_.set(index, value);
return this;
}
public Builder setParam(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.Builder builderForValue) {
result.param_.set(index, builderForValue.build());
return this;
}
public Builder addParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam value) {
if (value == null) {
throw new NullPointerException();
}
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.add(value);
return this;
}
public Builder addParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.Builder builderForValue) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.add(builderForValue.build());
return this;
}
public Builder addAllParam(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam> values) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
super.addAll(values, result.param_);
return this;
}
public Builder clearParam() {
result.param_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.YukonDependency)
}
static {
defaultInstance = new YukonDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.YukonDependency)
}
public static final class YukonParam extends
com.google.protobuf.GeneratedMessage {
// Use YukonParam.newBuilder() to construct.
private YukonParam() {
initFields();
}
private YukonParam(boolean noInit) {}
private static final YukonParam defaultInstance;
public static YukonParam getDefaultInstance() {
return defaultInstance;
}
public YukonParam getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_YukonParam_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_YukonParam_fieldAccessorTable;
}
// optional string key = 1;
public static final int KEY_FIELD_NUMBER = 1;
private boolean hasKey;
private java.lang.String key_ = "";
public boolean hasKey() { return hasKey; }
public java.lang.String getKey() { return key_; }
// optional .com.alachisoft.ncache.common.protobuf.YukonCommandParam cmdParam = 2;
public static final int CMDPARAM_FIELD_NUMBER = 2;
private boolean hasCmdParam;
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam cmdParam_;
public boolean hasCmdParam() { return hasCmdParam; }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam getCmdParam() { return cmdParam_; }
private void initFields() {
cmdParam_ = com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.getDefaultInstance();
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasKey()) {
output.writeString(1, getKey());
}
if (hasCmdParam()) {
output.writeMessage(2, getCmdParam());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasKey()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getKey());
}
if (hasCmdParam()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCmdParam());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasCmdParam()) {
mergeCmdParam(other.getCmdParam());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setKey(input.readString());
break;
}
case 18: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.newBuilder();
if (hasCmdParam()) {
subBuilder.mergeFrom(getCmdParam());
}
input.readMessage(subBuilder, extensionRegistry);
setCmdParam(subBuilder.buildPartial());
break;
}
}
}
}
// optional string key = 1;
public boolean hasKey() {
return result.hasKey();
}
public java.lang.String getKey() {
return result.getKey();
}
public Builder setKey(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasKey = true;
result.key_ = value;
return this;
}
public Builder clearKey() {
result.hasKey = false;
result.key_ = getDefaultInstance().getKey();
return this;
}
// optional .com.alachisoft.ncache.common.protobuf.YukonCommandParam cmdParam = 2;
public boolean hasCmdParam() {
return result.hasCmdParam();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam getCmdParam() {
return result.getCmdParam();
}
public Builder setCmdParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam value) {
if (value == null) {
throw new NullPointerException();
}
result.hasCmdParam = true;
result.cmdParam_ = value;
return this;
}
public Builder setCmdParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.Builder builderForValue) {
result.hasCmdParam = true;
result.cmdParam_ = builderForValue.build();
return this;
}
public Builder mergeCmdParam(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam value) {
if (result.hasCmdParam() &&
result.cmdParam_ != com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.getDefaultInstance()) {
result.cmdParam_ =
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.newBuilder(result.cmdParam_).mergeFrom(value).buildPartial();
} else {
result.cmdParam_ = value;
}
result.hasCmdParam = true;
return this;
}
public Builder clearCmdParam() {
result.hasCmdParam = false;
result.cmdParam_ = com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.getDefaultInstance();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.YukonParam)
}
static {
defaultInstance = new YukonParam(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.YukonParam)
}
public static final class YukonCommandParam extends
com.google.protobuf.GeneratedMessage {
// Use YukonCommandParam.newBuilder() to construct.
private YukonCommandParam() {
initFields();
}
private YukonCommandParam(boolean noInit) {}
private static final YukonCommandParam defaultInstance;
public static YukonCommandParam getDefaultInstance() {
return defaultInstance;
}
public YukonCommandParam getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_YukonCommandParam_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_YukonCommandParam_fieldAccessorTable;
}
// optional int32 typeId = 1;
public static final int TYPEID_FIELD_NUMBER = 1;
private boolean hasTypeId;
private int typeId_ = 0;
public boolean hasTypeId() { return hasTypeId; }
public int getTypeId() { return typeId_; }
// optional int32 direction = 2;
public static final int DIRECTION_FIELD_NUMBER = 2;
private boolean hasDirection;
private int direction_ = 0;
public boolean hasDirection() { return hasDirection; }
public int getDirection() { return direction_; }
// optional int32 dbType = 3;
public static final int DBTYPE_FIELD_NUMBER = 3;
private boolean hasDbType;
private int dbType_ = 0;
public boolean hasDbType() { return hasDbType; }
public int getDbType() { return dbType_; }
// optional int32 cmpOptions = 4;
public static final int CMPOPTIONS_FIELD_NUMBER = 4;
private boolean hasCmpOptions;
private int cmpOptions_ = 0;
public boolean hasCmpOptions() { return hasCmpOptions; }
public int getCmpOptions() { return cmpOptions_; }
// optional int32 version = 5;
public static final int VERSION_FIELD_NUMBER = 5;
private boolean hasVersion;
private int version_ = 0;
public boolean hasVersion() { return hasVersion; }
public int getVersion() { return version_; }
// optional string value = 6;
public static final int VALUE_FIELD_NUMBER = 6;
private boolean hasValue;
private java.lang.String value_ = "";
public boolean hasValue() { return hasValue; }
public java.lang.String getValue() { return value_; }
// optional bool isNullable = 7;
public static final int ISNULLABLE_FIELD_NUMBER = 7;
private boolean hasIsNullable;
private boolean isNullable_ = false;
public boolean hasIsNullable() { return hasIsNullable; }
public boolean getIsNullable() { return isNullable_; }
// optional int32 localeId = 8;
public static final int LOCALEID_FIELD_NUMBER = 8;
private boolean hasLocaleId;
private int localeId_ = 0;
public boolean hasLocaleId() { return hasLocaleId; }
public int getLocaleId() { return localeId_; }
// optional int32 offset = 9;
public static final int OFFSET_FIELD_NUMBER = 9;
private boolean hasOffset;
private int offset_ = 0;
public boolean hasOffset() { return hasOffset; }
public int getOffset() { return offset_; }
// optional int32 precision = 10;
public static final int PRECISION_FIELD_NUMBER = 10;
private boolean hasPrecision;
private int precision_ = 0;
public boolean hasPrecision() { return hasPrecision; }
public int getPrecision() { return precision_; }
// optional int32 scale = 11;
public static final int SCALE_FIELD_NUMBER = 11;
private boolean hasScale;
private int scale_ = 0;
public boolean hasScale() { return hasScale; }
public int getScale() { return scale_; }
// optional int32 size = 12;
public static final int SIZE_FIELD_NUMBER = 12;
private boolean hasSize;
private int size_ = 0;
public boolean hasSize() { return hasSize; }
public int getSize() { return size_; }
// optional string sourceColumn = 13;
public static final int SOURCECOLUMN_FIELD_NUMBER = 13;
private boolean hasSourceColumn;
private java.lang.String sourceColumn_ = "";
public boolean hasSourceColumn() { return hasSourceColumn; }
public java.lang.String getSourceColumn() { return sourceColumn_; }
// optional bool sourceColumnNull = 14;
public static final int SOURCECOLUMNNULL_FIELD_NUMBER = 14;
private boolean hasSourceColumnNull;
private boolean sourceColumnNull_ = false;
public boolean hasSourceColumnNull() { return hasSourceColumnNull; }
public boolean getSourceColumnNull() { return sourceColumnNull_; }
// optional string sqlValue = 15;
public static final int SQLVALUE_FIELD_NUMBER = 15;
private boolean hasSqlValue;
private java.lang.String sqlValue_ = "";
public boolean hasSqlValue() { return hasSqlValue; }
public java.lang.String getSqlValue() { return sqlValue_; }
// optional string typeName = 16;
public static final int TYPENAME_FIELD_NUMBER = 16;
private boolean hasTypeName;
private java.lang.String typeName_ = "";
public boolean hasTypeName() { return hasTypeName; }
public java.lang.String getTypeName() { return typeName_; }
// optional string udtTypeName = 17;
public static final int UDTTYPENAME_FIELD_NUMBER = 17;
private boolean hasUdtTypeName;
private java.lang.String udtTypeName_ = "";
public boolean hasUdtTypeName() { return hasUdtTypeName; }
public java.lang.String getUdtTypeName() { return udtTypeName_; }
// optional bool nullValueProvided = 18;
public static final int NULLVALUEPROVIDED_FIELD_NUMBER = 18;
private boolean hasNullValueProvided;
private boolean nullValueProvided_ = false;
public boolean hasNullValueProvided() { return hasNullValueProvided; }
public boolean getNullValueProvided() { return nullValueProvided_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasTypeId()) {
output.writeInt32(1, getTypeId());
}
if (hasDirection()) {
output.writeInt32(2, getDirection());
}
if (hasDbType()) {
output.writeInt32(3, getDbType());
}
if (hasCmpOptions()) {
output.writeInt32(4, getCmpOptions());
}
if (hasVersion()) {
output.writeInt32(5, getVersion());
}
if (hasValue()) {
output.writeString(6, getValue());
}
if (hasIsNullable()) {
output.writeBool(7, getIsNullable());
}
if (hasLocaleId()) {
output.writeInt32(8, getLocaleId());
}
if (hasOffset()) {
output.writeInt32(9, getOffset());
}
if (hasPrecision()) {
output.writeInt32(10, getPrecision());
}
if (hasScale()) {
output.writeInt32(11, getScale());
}
if (hasSize()) {
output.writeInt32(12, getSize());
}
if (hasSourceColumn()) {
output.writeString(13, getSourceColumn());
}
if (hasSourceColumnNull()) {
output.writeBool(14, getSourceColumnNull());
}
if (hasSqlValue()) {
output.writeString(15, getSqlValue());
}
if (hasTypeName()) {
output.writeString(16, getTypeName());
}
if (hasUdtTypeName()) {
output.writeString(17, getUdtTypeName());
}
if (hasNullValueProvided()) {
output.writeBool(18, getNullValueProvided());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasTypeId()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, getTypeId());
}
if (hasDirection()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, getDirection());
}
if (hasDbType()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, getDbType());
}
if (hasCmpOptions()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, getCmpOptions());
}
if (hasVersion()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, getVersion());
}
if (hasValue()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(6, getValue());
}
if (hasIsNullable()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, getIsNullable());
}
if (hasLocaleId()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, getLocaleId());
}
if (hasOffset()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(9, getOffset());
}
if (hasPrecision()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, getPrecision());
}
if (hasScale()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, getScale());
}
if (hasSize()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(12, getSize());
}
if (hasSourceColumn()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(13, getSourceColumn());
}
if (hasSourceColumnNull()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(14, getSourceColumnNull());
}
if (hasSqlValue()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(15, getSqlValue());
}
if (hasTypeName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(16, getTypeName());
}
if (hasUdtTypeName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(17, getUdtTypeName());
}
if (hasNullValueProvided()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(18, getNullValueProvided());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.getDefaultInstance()) return this;
if (other.hasTypeId()) {
setTypeId(other.getTypeId());
}
if (other.hasDirection()) {
setDirection(other.getDirection());
}
if (other.hasDbType()) {
setDbType(other.getDbType());
}
if (other.hasCmpOptions()) {
setCmpOptions(other.getCmpOptions());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
if (other.hasValue()) {
setValue(other.getValue());
}
if (other.hasIsNullable()) {
setIsNullable(other.getIsNullable());
}
if (other.hasLocaleId()) {
setLocaleId(other.getLocaleId());
}
if (other.hasOffset()) {
setOffset(other.getOffset());
}
if (other.hasPrecision()) {
setPrecision(other.getPrecision());
}
if (other.hasScale()) {
setScale(other.getScale());
}
if (other.hasSize()) {
setSize(other.getSize());
}
if (other.hasSourceColumn()) {
setSourceColumn(other.getSourceColumn());
}
if (other.hasSourceColumnNull()) {
setSourceColumnNull(other.getSourceColumnNull());
}
if (other.hasSqlValue()) {
setSqlValue(other.getSqlValue());
}
if (other.hasTypeName()) {
setTypeName(other.getTypeName());
}
if (other.hasUdtTypeName()) {
setUdtTypeName(other.getUdtTypeName());
}
if (other.hasNullValueProvided()) {
setNullValueProvided(other.getNullValueProvided());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 8: {
setTypeId(input.readInt32());
break;
}
case 16: {
setDirection(input.readInt32());
break;
}
case 24: {
setDbType(input.readInt32());
break;
}
case 32: {
setCmpOptions(input.readInt32());
break;
}
case 40: {
setVersion(input.readInt32());
break;
}
case 50: {
setValue(input.readString());
break;
}
case 56: {
setIsNullable(input.readBool());
break;
}
case 64: {
setLocaleId(input.readInt32());
break;
}
case 72: {
setOffset(input.readInt32());
break;
}
case 80: {
setPrecision(input.readInt32());
break;
}
case 88: {
setScale(input.readInt32());
break;
}
case 96: {
setSize(input.readInt32());
break;
}
case 106: {
setSourceColumn(input.readString());
break;
}
case 112: {
setSourceColumnNull(input.readBool());
break;
}
case 122: {
setSqlValue(input.readString());
break;
}
case 130: {
setTypeName(input.readString());
break;
}
case 138: {
setUdtTypeName(input.readString());
break;
}
case 144: {
setNullValueProvided(input.readBool());
break;
}
}
}
}
// optional int32 typeId = 1;
public boolean hasTypeId() {
return result.hasTypeId();
}
public int getTypeId() {
return result.getTypeId();
}
public Builder setTypeId(int value) {
result.hasTypeId = true;
result.typeId_ = value;
return this;
}
public Builder clearTypeId() {
result.hasTypeId = false;
result.typeId_ = 0;
return this;
}
// optional int32 direction = 2;
public boolean hasDirection() {
return result.hasDirection();
}
public int getDirection() {
return result.getDirection();
}
public Builder setDirection(int value) {
result.hasDirection = true;
result.direction_ = value;
return this;
}
public Builder clearDirection() {
result.hasDirection = false;
result.direction_ = 0;
return this;
}
// optional int32 dbType = 3;
public boolean hasDbType() {
return result.hasDbType();
}
public int getDbType() {
return result.getDbType();
}
public Builder setDbType(int value) {
result.hasDbType = true;
result.dbType_ = value;
return this;
}
public Builder clearDbType() {
result.hasDbType = false;
result.dbType_ = 0;
return this;
}
// optional int32 cmpOptions = 4;
public boolean hasCmpOptions() {
return result.hasCmpOptions();
}
public int getCmpOptions() {
return result.getCmpOptions();
}
public Builder setCmpOptions(int value) {
result.hasCmpOptions = true;
result.cmpOptions_ = value;
return this;
}
public Builder clearCmpOptions() {
result.hasCmpOptions = false;
result.cmpOptions_ = 0;
return this;
}
// optional int32 version = 5;
public boolean hasVersion() {
return result.hasVersion();
}
public int getVersion() {
return result.getVersion();
}
public Builder setVersion(int value) {
result.hasVersion = true;
result.version_ = value;
return this;
}
public Builder clearVersion() {
result.hasVersion = false;
result.version_ = 0;
return this;
}
// optional string value = 6;
public boolean hasValue() {
return result.hasValue();
}
public java.lang.String getValue() {
return result.getValue();
}
public Builder setValue(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasValue = true;
result.value_ = value;
return this;
}
public Builder clearValue() {
result.hasValue = false;
result.value_ = getDefaultInstance().getValue();
return this;
}
// optional bool isNullable = 7;
public boolean hasIsNullable() {
return result.hasIsNullable();
}
public boolean getIsNullable() {
return result.getIsNullable();
}
public Builder setIsNullable(boolean value) {
result.hasIsNullable = true;
result.isNullable_ = value;
return this;
}
public Builder clearIsNullable() {
result.hasIsNullable = false;
result.isNullable_ = false;
return this;
}
// optional int32 localeId = 8;
public boolean hasLocaleId() {
return result.hasLocaleId();
}
public int getLocaleId() {
return result.getLocaleId();
}
public Builder setLocaleId(int value) {
result.hasLocaleId = true;
result.localeId_ = value;
return this;
}
public Builder clearLocaleId() {
result.hasLocaleId = false;
result.localeId_ = 0;
return this;
}
// optional int32 offset = 9;
public boolean hasOffset() {
return result.hasOffset();
}
public int getOffset() {
return result.getOffset();
}
public Builder setOffset(int value) {
result.hasOffset = true;
result.offset_ = value;
return this;
}
public Builder clearOffset() {
result.hasOffset = false;
result.offset_ = 0;
return this;
}
// optional int32 precision = 10;
public boolean hasPrecision() {
return result.hasPrecision();
}
public int getPrecision() {
return result.getPrecision();
}
public Builder setPrecision(int value) {
result.hasPrecision = true;
result.precision_ = value;
return this;
}
public Builder clearPrecision() {
result.hasPrecision = false;
result.precision_ = 0;
return this;
}
// optional int32 scale = 11;
public boolean hasScale() {
return result.hasScale();
}
public int getScale() {
return result.getScale();
}
public Builder setScale(int value) {
result.hasScale = true;
result.scale_ = value;
return this;
}
public Builder clearScale() {
result.hasScale = false;
result.scale_ = 0;
return this;
}
// optional int32 size = 12;
public boolean hasSize() {
return result.hasSize();
}
public int getSize() {
return result.getSize();
}
public Builder setSize(int value) {
result.hasSize = true;
result.size_ = value;
return this;
}
public Builder clearSize() {
result.hasSize = false;
result.size_ = 0;
return this;
}
// optional string sourceColumn = 13;
public boolean hasSourceColumn() {
return result.hasSourceColumn();
}
public java.lang.String getSourceColumn() {
return result.getSourceColumn();
}
public Builder setSourceColumn(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSourceColumn = true;
result.sourceColumn_ = value;
return this;
}
public Builder clearSourceColumn() {
result.hasSourceColumn = false;
result.sourceColumn_ = getDefaultInstance().getSourceColumn();
return this;
}
// optional bool sourceColumnNull = 14;
public boolean hasSourceColumnNull() {
return result.hasSourceColumnNull();
}
public boolean getSourceColumnNull() {
return result.getSourceColumnNull();
}
public Builder setSourceColumnNull(boolean value) {
result.hasSourceColumnNull = true;
result.sourceColumnNull_ = value;
return this;
}
public Builder clearSourceColumnNull() {
result.hasSourceColumnNull = false;
result.sourceColumnNull_ = false;
return this;
}
// optional string sqlValue = 15;
public boolean hasSqlValue() {
return result.hasSqlValue();
}
public java.lang.String getSqlValue() {
return result.getSqlValue();
}
public Builder setSqlValue(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSqlValue = true;
result.sqlValue_ = value;
return this;
}
public Builder clearSqlValue() {
result.hasSqlValue = false;
result.sqlValue_ = getDefaultInstance().getSqlValue();
return this;
}
// optional string typeName = 16;
public boolean hasTypeName() {
return result.hasTypeName();
}
public java.lang.String getTypeName() {
return result.getTypeName();
}
public Builder setTypeName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTypeName = true;
result.typeName_ = value;
return this;
}
public Builder clearTypeName() {
result.hasTypeName = false;
result.typeName_ = getDefaultInstance().getTypeName();
return this;
}
// optional string udtTypeName = 17;
public boolean hasUdtTypeName() {
return result.hasUdtTypeName();
}
public java.lang.String getUdtTypeName() {
return result.getUdtTypeName();
}
public Builder setUdtTypeName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasUdtTypeName = true;
result.udtTypeName_ = value;
return this;
}
public Builder clearUdtTypeName() {
result.hasUdtTypeName = false;
result.udtTypeName_ = getDefaultInstance().getUdtTypeName();
return this;
}
// optional bool nullValueProvided = 18;
public boolean hasNullValueProvided() {
return result.hasNullValueProvided();
}
public boolean getNullValueProvided() {
return result.getNullValueProvided();
}
public Builder setNullValueProvided(boolean value) {
result.hasNullValueProvided = true;
result.nullValueProvided_ = value;
return this;
}
public Builder clearNullValueProvided() {
result.hasNullValueProvided = false;
result.nullValueProvided_ = false;
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.YukonCommandParam)
}
static {
defaultInstance = new YukonCommandParam(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.YukonCommandParam)
}
public static final class Sql7Dependency extends
com.google.protobuf.GeneratedMessage {
// Use Sql7Dependency.newBuilder() to construct.
private Sql7Dependency() {
initFields();
}
private Sql7Dependency(boolean noInit) {}
private static final Sql7Dependency defaultInstance;
public static Sql7Dependency getDefaultInstance() {
return defaultInstance;
}
public Sql7Dependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Sql7Dependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Sql7Dependency_fieldAccessorTable;
}
// optional string connectionString = 1;
public static final int CONNECTIONSTRING_FIELD_NUMBER = 1;
private boolean hasConnectionString;
private java.lang.String connectionString_ = "";
public boolean hasConnectionString() { return hasConnectionString; }
public java.lang.String getConnectionString() { return connectionString_; }
// optional string dbCacheKey = 2;
public static final int DBCACHEKEY_FIELD_NUMBER = 2;
private boolean hasDbCacheKey;
private java.lang.String dbCacheKey_ = "";
public boolean hasDbCacheKey() { return hasDbCacheKey; }
public java.lang.String getDbCacheKey() { return dbCacheKey_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasConnectionString()) {
output.writeString(1, getConnectionString());
}
if (hasDbCacheKey()) {
output.writeString(2, getDbCacheKey());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasConnectionString()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getConnectionString());
}
if (hasDbCacheKey()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getDbCacheKey());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.getDefaultInstance()) return this;
if (other.hasConnectionString()) {
setConnectionString(other.getConnectionString());
}
if (other.hasDbCacheKey()) {
setDbCacheKey(other.getDbCacheKey());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setConnectionString(input.readString());
break;
}
case 18: {
setDbCacheKey(input.readString());
break;
}
}
}
}
// optional string connectionString = 1;
public boolean hasConnectionString() {
return result.hasConnectionString();
}
public java.lang.String getConnectionString() {
return result.getConnectionString();
}
public Builder setConnectionString(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasConnectionString = true;
result.connectionString_ = value;
return this;
}
public Builder clearConnectionString() {
result.hasConnectionString = false;
result.connectionString_ = getDefaultInstance().getConnectionString();
return this;
}
// optional string dbCacheKey = 2;
public boolean hasDbCacheKey() {
return result.hasDbCacheKey();
}
public java.lang.String getDbCacheKey() {
return result.getDbCacheKey();
}
public Builder setDbCacheKey(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDbCacheKey = true;
result.dbCacheKey_ = value;
return this;
}
public Builder clearDbCacheKey() {
result.hasDbCacheKey = false;
result.dbCacheKey_ = getDefaultInstance().getDbCacheKey();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.Sql7Dependency)
}
static {
defaultInstance = new Sql7Dependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.Sql7Dependency)
}
public static final class OleDbDependency extends
com.google.protobuf.GeneratedMessage {
// Use OleDbDependency.newBuilder() to construct.
private OleDbDependency() {
initFields();
}
private OleDbDependency(boolean noInit) {}
private static final OleDbDependency defaultInstance;
public static OleDbDependency getDefaultInstance() {
return defaultInstance;
}
public OleDbDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OleDbDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_OleDbDependency_fieldAccessorTable;
}
// optional string connectionString = 1;
public static final int CONNECTIONSTRING_FIELD_NUMBER = 1;
private boolean hasConnectionString;
private java.lang.String connectionString_ = "";
public boolean hasConnectionString() { return hasConnectionString; }
public java.lang.String getConnectionString() { return connectionString_; }
// optional string dbCacheKey = 2;
public static final int DBCACHEKEY_FIELD_NUMBER = 2;
private boolean hasDbCacheKey;
private java.lang.String dbCacheKey_ = "";
public boolean hasDbCacheKey() { return hasDbCacheKey; }
public java.lang.String getDbCacheKey() { return dbCacheKey_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasConnectionString()) {
output.writeString(1, getConnectionString());
}
if (hasDbCacheKey()) {
output.writeString(2, getDbCacheKey());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasConnectionString()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getConnectionString());
}
if (hasDbCacheKey()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getDbCacheKey());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.getDefaultInstance()) return this;
if (other.hasConnectionString()) {
setConnectionString(other.getConnectionString());
}
if (other.hasDbCacheKey()) {
setDbCacheKey(other.getDbCacheKey());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setConnectionString(input.readString());
break;
}
case 18: {
setDbCacheKey(input.readString());
break;
}
}
}
}
// optional string connectionString = 1;
public boolean hasConnectionString() {
return result.hasConnectionString();
}
public java.lang.String getConnectionString() {
return result.getConnectionString();
}
public Builder setConnectionString(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasConnectionString = true;
result.connectionString_ = value;
return this;
}
public Builder clearConnectionString() {
result.hasConnectionString = false;
result.connectionString_ = getDefaultInstance().getConnectionString();
return this;
}
// optional string dbCacheKey = 2;
public boolean hasDbCacheKey() {
return result.hasDbCacheKey();
}
public java.lang.String getDbCacheKey() {
return result.getDbCacheKey();
}
public Builder setDbCacheKey(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDbCacheKey = true;
result.dbCacheKey_ = value;
return this;
}
public Builder clearDbCacheKey() {
result.hasDbCacheKey = false;
result.dbCacheKey_ = getDefaultInstance().getDbCacheKey();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.OleDbDependency)
}
static {
defaultInstance = new OleDbDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.OleDbDependency)
}
public static final class ExtensibleDependency extends
com.google.protobuf.GeneratedMessage {
// Use ExtensibleDependency.newBuilder() to construct.
private ExtensibleDependency() {
initFields();
}
private ExtensibleDependency(boolean noInit) {}
private static final ExtensibleDependency defaultInstance;
public static ExtensibleDependency getDefaultInstance() {
return defaultInstance;
}
public ExtensibleDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_ExtensibleDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_ExtensibleDependency_fieldAccessorTable;
}
// optional bytes data = 1;
public static final int DATA_FIELD_NUMBER = 1;
private boolean hasData;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasData() { return hasData; }
public com.google.protobuf.ByteString getData() { return data_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasData()) {
output.writeBytes(1, getData());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasData()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getData());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.getDefaultInstance()) return this;
if (other.hasData()) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setData(input.readBytes());
break;
}
}
}
}
// optional bytes data = 1;
public boolean hasData() {
return result.hasData();
}
public com.google.protobuf.ByteString getData() {
return result.getData();
}
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
result.hasData = true;
result.data_ = value;
return this;
}
public Builder clearData() {
result.hasData = false;
result.data_ = getDefaultInstance().getData();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.ExtensibleDependency)
}
static {
defaultInstance = new ExtensibleDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.ExtensibleDependency)
}
public static final class NosDbDependency extends
com.google.protobuf.GeneratedMessage {
// Use NosDbDependency.newBuilder() to construct.
private NosDbDependency() {
initFields();
}
private NosDbDependency(boolean noInit) {}
private static final NosDbDependency defaultInstance;
public static NosDbDependency getDefaultInstance() {
return defaultInstance;
}
public NosDbDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_NosDbDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_NosDbDependency_fieldAccessorTable;
}
// optional string connectionString = 1;
public static final int CONNECTIONSTRING_FIELD_NUMBER = 1;
private boolean hasConnectionString;
private java.lang.String connectionString_ = "";
public boolean hasConnectionString() { return hasConnectionString; }
public java.lang.String getConnectionString() { return connectionString_; }
// optional string query = 2;
public static final int QUERY_FIELD_NUMBER = 2;
private boolean hasQuery;
private java.lang.String query_ = "";
public boolean hasQuery() { return hasQuery; }
public java.lang.String getQuery() { return query_; }
// optional int32 timeout = 3;
public static final int TIMEOUT_FIELD_NUMBER = 3;
private boolean hasTimeout;
private int timeout_ = 0;
public boolean hasTimeout() { return hasTimeout; }
public int getTimeout() { return timeout_; }
// repeated .com.alachisoft.ncache.common.protobuf.KeyValue param = 4;
public static final int PARAM_FIELD_NUMBER = 4;
private java.util.List param_ =
java.util.Collections.emptyList();
public java.util.List getParamList() {
return param_;
}
public int getParamCount() { return param_.size(); }
public com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue getParam(int index) {
return param_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasConnectionString()) {
output.writeString(1, getConnectionString());
}
if (hasQuery()) {
output.writeString(2, getQuery());
}
if (hasTimeout()) {
output.writeInt32(3, getTimeout());
}
for (com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue element : getParamList()) {
output.writeMessage(4, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasConnectionString()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getConnectionString());
}
if (hasQuery()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getQuery());
}
if (hasTimeout()) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, getTimeout());
}
for (com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue element : getParamList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.param_ != java.util.Collections.EMPTY_LIST) {
result.param_ =
java.util.Collections.unmodifiableList(result.param_);
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.getDefaultInstance()) return this;
if (other.hasConnectionString()) {
setConnectionString(other.getConnectionString());
}
if (other.hasQuery()) {
setQuery(other.getQuery());
}
if (other.hasTimeout()) {
setTimeout(other.getTimeout());
}
if (!other.param_.isEmpty()) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.addAll(other.param_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setConnectionString(input.readString());
break;
}
case 18: {
setQuery(input.readString());
break;
}
case 24: {
setTimeout(input.readInt32());
break;
}
case 34: {
com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue.Builder subBuilder = com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addParam(subBuilder.buildPartial());
break;
}
}
}
}
// optional string connectionString = 1;
public boolean hasConnectionString() {
return result.hasConnectionString();
}
public java.lang.String getConnectionString() {
return result.getConnectionString();
}
public Builder setConnectionString(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasConnectionString = true;
result.connectionString_ = value;
return this;
}
public Builder clearConnectionString() {
result.hasConnectionString = false;
result.connectionString_ = getDefaultInstance().getConnectionString();
return this;
}
// optional string query = 2;
public boolean hasQuery() {
return result.hasQuery();
}
public java.lang.String getQuery() {
return result.getQuery();
}
public Builder setQuery(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasQuery = true;
result.query_ = value;
return this;
}
public Builder clearQuery() {
result.hasQuery = false;
result.query_ = getDefaultInstance().getQuery();
return this;
}
// optional int32 timeout = 3;
public boolean hasTimeout() {
return result.hasTimeout();
}
public int getTimeout() {
return result.getTimeout();
}
public Builder setTimeout(int value) {
result.hasTimeout = true;
result.timeout_ = value;
return this;
}
public Builder clearTimeout() {
result.hasTimeout = false;
result.timeout_ = 0;
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.KeyValue param = 4;
public java.util.List getParamList() {
return java.util.Collections.unmodifiableList(result.param_);
}
public int getParamCount() {
return result.getParamCount();
}
public com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue getParam(int index) {
return result.getParam(index);
}
public Builder setParam(int index, com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue value) {
if (value == null) {
throw new NullPointerException();
}
result.param_.set(index, value);
return this;
}
public Builder setParam(int index, com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue.Builder builderForValue) {
result.param_.set(index, builderForValue.build());
return this;
}
public Builder addParam(com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue value) {
if (value == null) {
throw new NullPointerException();
}
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.add(value);
return this;
}
public Builder addParam(com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue.Builder builderForValue) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
result.param_.add(builderForValue.build());
return this;
}
public Builder addAllParam(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.KeyValueProtocol.KeyValue> values) {
if (result.param_.isEmpty()) {
result.param_ = new java.util.ArrayList();
}
super.addAll(values, result.param_);
return this;
}
public Builder clearParam() {
result.param_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.NosDbDependency)
}
static {
defaultInstance = new NosDbDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.NosDbDependency)
}
public static final class Dictionary extends
com.google.protobuf.GeneratedMessage {
// Use Dictionary.newBuilder() to construct.
private Dictionary() {
initFields();
}
private Dictionary(boolean noInit) {}
private static final Dictionary defaultInstance;
public static Dictionary getDefaultInstance() {
return defaultInstance;
}
public Dictionary getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Dictionary_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_Dictionary_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private boolean hasValue;
private java.lang.String value_ = "";
public boolean hasValue() { return hasValue; }
public java.lang.String getValue() { return value_; }
private void initFields() {
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasValue) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasValue()) {
output.writeString(2, getValue());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasValue()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 18: {
setValue(input.readString());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// required string value = 2;
public boolean hasValue() {
return result.hasValue();
}
public java.lang.String getValue() {
return result.getValue();
}
public Builder setValue(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasValue = true;
result.value_ = value;
return this;
}
public Builder clearValue() {
result.hasValue = false;
result.value_ = getDefaultInstance().getValue();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.Dictionary)
}
static {
defaultInstance = new Dictionary(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.Dictionary)
}
public static final class CustomDependency extends
com.google.protobuf.GeneratedMessage {
// Use CustomDependency.newBuilder() to construct.
private CustomDependency() {
initFields();
}
private CustomDependency(boolean noInit) {}
private static final CustomDependency defaultInstance;
public static CustomDependency getDefaultInstance() {
return defaultInstance;
}
public CustomDependency getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_CustomDependency_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.internal_static_com_alachisoft_ncache_common_protobuf_CustomDependency_fieldAccessorTable;
}
// required string providerName = 1;
public static final int PROVIDERNAME_FIELD_NUMBER = 1;
private boolean hasProviderName;
private java.lang.String providerName_ = "";
public boolean hasProviderName() { return hasProviderName; }
public java.lang.String getProviderName() { return providerName_; }
// repeated .com.alachisoft.ncache.common.protobuf.Dictionary parameters = 2;
public static final int PARAMETERS_FIELD_NUMBER = 2;
private java.util.List parameters_ =
java.util.Collections.emptyList();
public java.util.List getParametersList() {
return parameters_;
}
public int getParametersCount() { return parameters_.size(); }
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary getParameters(int index) {
return parameters_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
if (!hasProviderName) return false;
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary element : getParametersList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasProviderName()) {
output.writeString(1, getProviderName());
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary element : getParametersList()) {
output.writeMessage(2, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasProviderName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getProviderName());
}
for (com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary element : getParametersList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency result;
// Construct using com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency();
return builder;
}
protected com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.getDescriptor();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency getDefaultInstanceForType() {
return com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.parameters_ != java.util.Collections.EMPTY_LIST) {
result.parameters_ =
java.util.Collections.unmodifiableList(result.parameters_);
}
com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency) {
return mergeFrom((com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency other) {
if (other == com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.getDefaultInstance()) return this;
if (other.hasProviderName()) {
setProviderName(other.getProviderName());
}
if (!other.parameters_.isEmpty()) {
if (result.parameters_.isEmpty()) {
result.parameters_ = new java.util.ArrayList();
}
result.parameters_.addAll(other.parameters_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setProviderName(input.readString());
break;
}
case 18: {
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.Builder subBuilder = com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addParameters(subBuilder.buildPartial());
break;
}
}
}
}
// required string providerName = 1;
public boolean hasProviderName() {
return result.hasProviderName();
}
public java.lang.String getProviderName() {
return result.getProviderName();
}
public Builder setProviderName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasProviderName = true;
result.providerName_ = value;
return this;
}
public Builder clearProviderName() {
result.hasProviderName = false;
result.providerName_ = getDefaultInstance().getProviderName();
return this;
}
// repeated .com.alachisoft.ncache.common.protobuf.Dictionary parameters = 2;
public java.util.List getParametersList() {
return java.util.Collections.unmodifiableList(result.parameters_);
}
public int getParametersCount() {
return result.getParametersCount();
}
public com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary getParameters(int index) {
return result.getParameters(index);
}
public Builder setParameters(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary value) {
if (value == null) {
throw new NullPointerException();
}
result.parameters_.set(index, value);
return this;
}
public Builder setParameters(int index, com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.Builder builderForValue) {
result.parameters_.set(index, builderForValue.build());
return this;
}
public Builder addParameters(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary value) {
if (value == null) {
throw new NullPointerException();
}
if (result.parameters_.isEmpty()) {
result.parameters_ = new java.util.ArrayList();
}
result.parameters_.add(value);
return this;
}
public Builder addParameters(com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.Builder builderForValue) {
if (result.parameters_.isEmpty()) {
result.parameters_ = new java.util.ArrayList();
}
result.parameters_.add(builderForValue.build());
return this;
}
public Builder addAllParameters(
java.lang.Iterable extends com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary> values) {
if (result.parameters_.isEmpty()) {
result.parameters_ = new java.util.ArrayList();
}
super.addAll(values, result.parameters_);
return this;
}
public Builder clearParameters() {
result.parameters_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:com.alachisoft.ncache.common.protobuf.CustomDependency)
}
static {
defaultInstance = new CustomDependency(true);
com.alachisoft.ncache.common.protobuf.DependencyProtocol.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.alachisoft.ncache.common.protobuf.CustomDependency)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_Dependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_Dependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_KeyDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_KeyDependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_FileDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_FileDependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_OracleDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_OracleDependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_OracleParam_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_OracleParam_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_OracleCommandParam_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_OracleCommandParam_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_YukonDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_YukonDependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_YukonParam_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_YukonParam_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_YukonCommandParam_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_YukonCommandParam_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_Sql7Dependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_Sql7Dependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_OleDbDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_OleDbDependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_ExtensibleDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_ExtensibleDependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_NosDbDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_NosDbDependency_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_Dictionary_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_Dictionary_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_alachisoft_ncache_common_protobuf_CustomDependency_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_alachisoft_ncache_common_protobuf_CustomDependency_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\020Dependency.proto\022%com.alachisoft.ncach" +
"e.common.protobuf\032\016KeyValue.proto\"\242\005\n\nDe" +
"pendency\022D\n\006keyDep\030\001 \003(\01324.com.alachisof" +
"t.ncache.common.protobuf.KeyDependency\022F" +
"\n\007fileDep\030\002 \003(\01325.com.alachisoft.ncache." +
"common.protobuf.FileDependency\022J\n\toracle" +
"Dep\030\003 \003(\01327.com.alachisoft.ncache.common" +
".protobuf.OracleDependency\022H\n\010yukonDep\030\004" +
" \003(\01326.com.alachisoft.ncache.common.prot" +
"obuf.YukonDependency\022F\n\007sql7Dep\030\005 \003(\01325.",
"com.alachisoft.ncache.common.protobuf.Sq" +
"l7Dependency\022H\n\010oleDbDep\030\006 \003(\01326.com.ala" +
"chisoft.ncache.common.protobuf.OleDbDepe" +
"ndency\022J\n\005xtDep\030\007 \003(\0132;.com.alachisoft.n" +
"cache.common.protobuf.ExtensibleDependen" +
"cy\022F\n\006NosDep\030\010 \003(\01326.com.alachisoft.ncac" +
"he.common.protobuf.NosDbDependency\022J\n\tcu" +
"stomDep\030\t \003(\01327.com.alachisoft.ncache.co" +
"mmon.protobuf.CustomDependency\"L\n\rKeyDep" +
"endency\022\014\n\004keys\030\001 \003(\t\022\022\n\nstartAfter\030\002 \001(",
"\003\022\031\n\021keyDependencyType\030\003 \001(\005\"7\n\016FileDepe" +
"ndency\022\021\n\tfilePaths\030\001 \003(\t\022\022\n\nstartAfter\030" +
"\002 \001(\003\"\223\001\n\020OracleDependency\022\030\n\020connection" +
"String\030\001 \001(\t\022\r\n\005query\030\002 \001(\t\022\023\n\013commandTy" +
"pe\030\003 \001(\005\022A\n\005param\030\004 \003(\01322.com.alachisoft" +
".ncache.common.protobuf.OracleParam\"g\n\013O" +
"racleParam\022\013\n\003key\030\001 \001(\t\022K\n\010cmdParam\030\002 \001(" +
"\01329.com.alachisoft.ncache.common.protobu" +
"f.OracleCommandParam\"F\n\022OracleCommandPar" +
"am\022\016\n\006dbType\030\001 \001(\005\022\021\n\tdirection\030\002 \001(\005\022\r\n",
"\005value\030\003 \001(\t\"\221\001\n\017YukonDependency\022\030\n\020conn" +
"ectionString\030\001 \001(\t\022\r\n\005query\030\002 \001(\t\022\023\n\013com" +
"mandType\030\003 \001(\005\022@\n\005param\030\004 \003(\01321.com.alac" +
"hisoft.ncache.common.protobuf.YukonParam" +
"\"e\n\nYukonParam\022\013\n\003key\030\001 \001(\t\022J\n\010cmdParam\030" +
"\002 \001(\01328.com.alachisoft.ncache.common.pro" +
"tobuf.YukonCommandParam\"\344\002\n\021YukonCommand" +
"Param\022\016\n\006typeId\030\001 \001(\005\022\021\n\tdirection\030\002 \001(\005" +
"\022\016\n\006dbType\030\003 \001(\005\022\022\n\ncmpOptions\030\004 \001(\005\022\017\n\007" +
"version\030\005 \001(\005\022\r\n\005value\030\006 \001(\t\022\022\n\nisNullab",
"le\030\007 \001(\010\022\020\n\010localeId\030\010 \001(\005\022\016\n\006offset\030\t \001" +
"(\005\022\021\n\tprecision\030\n \001(\005\022\r\n\005scale\030\013 \001(\005\022\014\n\004" +
"size\030\014 \001(\005\022\024\n\014sourceColumn\030\r \001(\t\022\030\n\020sour" +
"ceColumnNull\030\016 \001(\010\022\020\n\010sqlValue\030\017 \001(\t\022\020\n\010" +
"typeName\030\020 \001(\t\022\023\n\013udtTypeName\030\021 \001(\t\022\031\n\021n" +
"ullValueProvided\030\022 \001(\010\">\n\016Sql7Dependency" +
"\022\030\n\020connectionString\030\001 \001(\t\022\022\n\ndbCacheKey" +
"\030\002 \001(\t\"?\n\017OleDbDependency\022\030\n\020connectionS" +
"tring\030\001 \001(\t\022\022\n\ndbCacheKey\030\002 \001(\t\"$\n\024Exten" +
"sibleDependency\022\014\n\004data\030\001 \001(\014\"\213\001\n\017NosDbD",
"ependency\022\030\n\020connectionString\030\001 \001(\t\022\r\n\005q" +
"uery\030\002 \001(\t\022\017\n\007timeout\030\003 \001(\005\022>\n\005param\030\004 \003" +
"(\0132/.com.alachisoft.ncache.common.protob" +
"uf.KeyValue\")\n\nDictionary\022\014\n\004name\030\001 \002(\t\022" +
"\r\n\005value\030\002 \002(\t\"o\n\020CustomDependency\022\024\n\014pr" +
"oviderName\030\001 \002(\t\022E\n\nparameters\030\002 \003(\01321.c" +
"om.alachisoft.ncache.common.protobuf.Dic" +
"tionaryB\024B\022DependencyProtocol"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_com_alachisoft_ncache_common_protobuf_Dependency_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_com_alachisoft_ncache_common_protobuf_Dependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_Dependency_descriptor,
new java.lang.String[] { "KeyDep", "FileDep", "OracleDep", "YukonDep", "Sql7Dep", "OleDbDep", "XtDep", "NosDep", "CustomDep", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_KeyDependency_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_com_alachisoft_ncache_common_protobuf_KeyDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_KeyDependency_descriptor,
new java.lang.String[] { "Keys", "StartAfter", "KeyDependencyType", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.KeyDependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_FileDependency_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_com_alachisoft_ncache_common_protobuf_FileDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_FileDependency_descriptor,
new java.lang.String[] { "FilePaths", "StartAfter", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.FileDependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_OracleDependency_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_com_alachisoft_ncache_common_protobuf_OracleDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_OracleDependency_descriptor,
new java.lang.String[] { "ConnectionString", "Query", "CommandType", "Param", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleDependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_OracleParam_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_com_alachisoft_ncache_common_protobuf_OracleParam_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_OracleParam_descriptor,
new java.lang.String[] { "Key", "CmdParam", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleParam.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_OracleCommandParam_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_com_alachisoft_ncache_common_protobuf_OracleCommandParam_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_OracleCommandParam_descriptor,
new java.lang.String[] { "DbType", "Direction", "Value", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OracleCommandParam.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_YukonDependency_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_com_alachisoft_ncache_common_protobuf_YukonDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_YukonDependency_descriptor,
new java.lang.String[] { "ConnectionString", "Query", "CommandType", "Param", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonDependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_YukonParam_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_com_alachisoft_ncache_common_protobuf_YukonParam_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_YukonParam_descriptor,
new java.lang.String[] { "Key", "CmdParam", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonParam.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_YukonCommandParam_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_com_alachisoft_ncache_common_protobuf_YukonCommandParam_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_YukonCommandParam_descriptor,
new java.lang.String[] { "TypeId", "Direction", "DbType", "CmpOptions", "Version", "Value", "IsNullable", "LocaleId", "Offset", "Precision", "Scale", "Size", "SourceColumn", "SourceColumnNull", "SqlValue", "TypeName", "UdtTypeName", "NullValueProvided", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.YukonCommandParam.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_Sql7Dependency_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_com_alachisoft_ncache_common_protobuf_Sql7Dependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_Sql7Dependency_descriptor,
new java.lang.String[] { "ConnectionString", "DbCacheKey", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Sql7Dependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_OleDbDependency_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_com_alachisoft_ncache_common_protobuf_OleDbDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_OleDbDependency_descriptor,
new java.lang.String[] { "ConnectionString", "DbCacheKey", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.OleDbDependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_ExtensibleDependency_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_com_alachisoft_ncache_common_protobuf_ExtensibleDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_ExtensibleDependency_descriptor,
new java.lang.String[] { "Data", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.ExtensibleDependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_NosDbDependency_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_com_alachisoft_ncache_common_protobuf_NosDbDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_NosDbDependency_descriptor,
new java.lang.String[] { "ConnectionString", "Query", "Timeout", "Param", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.NosDbDependency.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_Dictionary_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_com_alachisoft_ncache_common_protobuf_Dictionary_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_Dictionary_descriptor,
new java.lang.String[] { "Name", "Value", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.Dictionary.Builder.class);
internal_static_com_alachisoft_ncache_common_protobuf_CustomDependency_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_com_alachisoft_ncache_common_protobuf_CustomDependency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_alachisoft_ncache_common_protobuf_CustomDependency_descriptor,
new java.lang.String[] { "ProviderName", "Parameters", },
com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.class,
com.alachisoft.ncache.common.protobuf.DependencyProtocol.CustomDependency.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.alachisoft.ncache.common.protobuf.KeyValueProtocol.getDescriptor(),
}, assigner);
}
public static void internalForceInit() {}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy