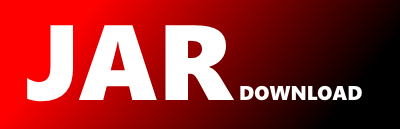
jdash.client.response.impl.GDSerializedSourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdash-client Show documentation
Show all versions of jdash-client Show documentation
The HTTP client used to pull data from Geometry Dash servers
The newest version!
package jdash.client.response.impl;
import jdash.client.exception.GDClientException;
import jdash.client.exception.GDResponseException;
import jdash.client.exception.ResponseDeserializationException;
import jdash.client.request.GDRequest;
import jdash.client.response.GDResponse;
import reactor.core.publisher.Mono;
import java.util.function.Consumer;
import java.util.function.Function;
public final class GDSerializedSourceResponse implements GDResponse {
private final GDRequest request;
private final Mono serializedSource;
private final Consumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy