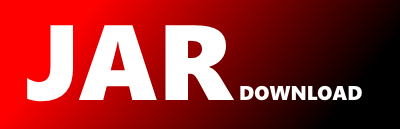
com.alexkasko.springjdbc.parallel.accessor.RoundRobinAccessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parallel-queries Show documentation
Show all versions of parallel-queries Show documentation
Processes given SQL query in parallel in multiple data sources (assuming that all data source
contain the same data). Combined results from multiple queries are exposed to application as
java.util.Iterator. Worker thread use spring's JdbcTemplate with named parameters support.
package com.alexkasko.springjdbc.parallel.accessor;
import org.springframework.jdbc.core.namedparam.NamedParameterJdbcOperations;
import org.springframework.jdbc.core.namedparam.SqlParameterSource;
import java.util.Collection;
import java.util.concurrent.atomic.AtomicInteger;
/**
* Round-robin collection accessor. Copies provided collection into inner immutable one on creation.
* Element is chosen in circular order ignoring provided parameter.
* Thread-safe.
*
* @author alexkasko
* Date: 6/11/12
* @see DataSourceAccessor
*/
public class RoundRobinAccessor extends ImmutableAccessor {
private AtomicInteger index = new AtomicInteger(0);
/**
* Protected constructors
*
* @param target collection to wrap
*/
public RoundRobinAccessor(Collection target) {
super(target);
}
/**
* Generic friendly factory method
*
* @param target collection to wrap
* @param JdbcOperations implementation
* @return new accessor instance
*/
public static RoundRobinAccessor of(Collection target) {
return new RoundRobinAccessor(target);
}
/**
* {@inheritDoc}
*/
@Override
public T get(SqlParameterSource params) {
return delegate.get(incrementAndGet());
}
/**
* Returns current index value
*
* @return current index
*/
public int getIndex() {
return index.get();
}
/**
* Atomically increments index using target list size modulus
*
* @return incremented index
*/
private int incrementAndGet() {
for (;;) {
int current = index.get();
int next = (current < delegate.size() - 1) ? current + 1 : 0;
if (index.compareAndSet(current, next)) return current;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy