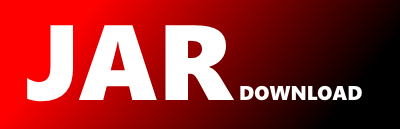
com.alextherapeutics.diga.model.generatedxml.billingreport.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.06.03 at 07:50:14 AM UTC
//
package com.alextherapeutics.diga.model.generatedxml.billingreport;
import javax.xml.namespace.QName;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
import jakarta.xml.bind.annotation.adapters.NormalizedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.alextherapeutics.diga.model.generatedxml.billingreport package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _Scenario_QNAME = new QName("http://www.xoev.de/de/validator/framework/1/scenarios", "scenario");
private final static QName _Resource_QNAME = new QName("http://www.xoev.de/de/validator/framework/1/scenarios", "resource");
private final static QName _DescriptionTypeP_QNAME = new QName("http://www.xoev.de/de/validator/framework/1/scenarios", "p");
private final static QName _DescriptionTypeOl_QNAME = new QName("http://www.xoev.de/de/validator/framework/1/scenarios", "ol");
private final static QName _DescriptionTypeUl_QNAME = new QName("http://www.xoev.de/de/validator/framework/1/scenarios", "ul");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.alextherapeutics.diga.model.generatedxml.billingreport
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Report }
*
*/
public Report createReport() {
return new Report();
}
/**
* Create an instance of {@link RecommendationType }
*
*/
public RecommendationType createRecommendationType() {
return new RecommendationType();
}
/**
* Create an instance of {@link DocumentIdentificationType }
*
*/
public DocumentIdentificationType createDocumentIdentificationType() {
return new DocumentIdentificationType();
}
/**
* Create an instance of {@link EngineType }
*
*/
public EngineType createEngineType() {
return new EngineType();
}
/**
* Create an instance of {@link DescriptionType }
*
*/
public DescriptionType createDescriptionType() {
return new DescriptionType();
}
/**
* Create an instance of {@link Scenarios }
*
*/
public Scenarios createScenarios() {
return new Scenarios();
}
/**
* Create an instance of {@link ScenarioType }
*
*/
public ScenarioType createScenarioType() {
return new ScenarioType();
}
/**
* Create an instance of {@link NoScenarioReportType }
*
*/
public NoScenarioReportType createNoScenarioReportType() {
return new NoScenarioReportType();
}
/**
* Create an instance of {@link ResourceType }
*
*/
public ResourceType createResourceType() {
return new ResourceType();
}
/**
* Create an instance of {@link NamespaceType }
*
*/
public NamespaceType createNamespaceType() {
return new NamespaceType();
}
/**
* Create an instance of {@link ValidateWithXmlSchema }
*
*/
public ValidateWithXmlSchema createValidateWithXmlSchema() {
return new ValidateWithXmlSchema();
}
/**
* Create an instance of {@link ValidateWithSchematron }
*
*/
public ValidateWithSchematron createValidateWithSchematron() {
return new ValidateWithSchematron();
}
/**
* Create an instance of {@link CreateReportType }
*
*/
public CreateReportType createCreateReportType() {
return new CreateReportType();
}
/**
* Create an instance of {@link CustomErrorLevel }
*
*/
public CustomErrorLevel createCustomErrorLevel() {
return new CustomErrorLevel();
}
/**
* Create an instance of {@link Report.ScenarioMatched }
*
*/
public Report.ScenarioMatched createReportScenarioMatched() {
return new Report.ScenarioMatched();
}
/**
* Create an instance of {@link Report.NoScenarioMatched }
*
*/
public Report.NoScenarioMatched createReportNoScenarioMatched() {
return new Report.NoScenarioMatched();
}
/**
* Create an instance of {@link AssessmentType }
*
*/
public AssessmentType createAssessmentType() {
return new AssessmentType();
}
/**
* Create an instance of {@link MessageType }
*
*/
public MessageType createMessageType() {
return new MessageType();
}
/**
* Create an instance of {@link ValidationStepResultType }
*
*/
public ValidationStepResultType createValidationStepResultType() {
return new ValidationStepResultType();
}
/**
* Create an instance of {@link DocumentDataType }
*
*/
public DocumentDataType createDocumentDataType() {
return new DocumentDataType();
}
/**
* Create an instance of {@link RecommendationType.Explanation }
*
*/
public RecommendationType.Explanation createRecommendationTypeExplanation() {
return new RecommendationType.Explanation();
}
/**
* Create an instance of {@link DocumentIdentificationType.DocumentHash }
*
*/
public DocumentIdentificationType.DocumentHash createDocumentIdentificationTypeDocumentHash() {
return new DocumentIdentificationType.DocumentHash();
}
/**
* Create an instance of {@link EngineType.Info }
*
*/
public EngineType.Info createEngineTypeInfo() {
return new EngineType.Info();
}
/**
* Create an instance of {@link DescriptionType.Ol }
*
*/
public DescriptionType.Ol createDescriptionTypeOl() {
return new DescriptionType.Ol();
}
/**
* Create an instance of {@link DescriptionType.Ul }
*
*/
public DescriptionType.Ul createDescriptionTypeUl() {
return new DescriptionType.Ul();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ScenarioType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ScenarioType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.xoev.de/de/validator/framework/1/scenarios", name = "scenario")
public JAXBElement createScenario(ScenarioType value) {
return new JAXBElement(_Scenario_QNAME, ScenarioType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ResourceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ResourceType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.xoev.de/de/validator/framework/1/scenarios", name = "resource")
public JAXBElement createResource(ResourceType value) {
return new JAXBElement(_Resource_QNAME, ResourceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://www.xoev.de/de/validator/framework/1/scenarios", name = "p", scope = DescriptionType.class)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
public JAXBElement createDescriptionTypeP(String value) {
return new JAXBElement(_DescriptionTypeP_QNAME, String.class, DescriptionType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DescriptionType.Ol }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DescriptionType.Ol }{@code >}
*/
@XmlElementDecl(namespace = "http://www.xoev.de/de/validator/framework/1/scenarios", name = "ol", scope = DescriptionType.class)
public JAXBElement createDescriptionTypeOl(DescriptionType.Ol value) {
return new JAXBElement(_DescriptionTypeOl_QNAME, DescriptionType.Ol.class, DescriptionType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DescriptionType.Ul }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DescriptionType.Ul }{@code >}
*/
@XmlElementDecl(namespace = "http://www.xoev.de/de/validator/framework/1/scenarios", name = "ul", scope = DescriptionType.class)
public JAXBElement createDescriptionTypeUl(DescriptionType.Ul value) {
return new JAXBElement(_DescriptionTypeUl_QNAME, DescriptionType.Ul.class, DescriptionType.class, value);
}
}