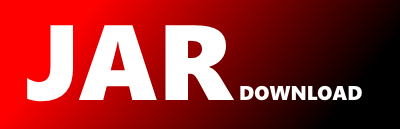
commonMain.com.algolia.search.endpoint.internal.EndpointInsightsUser.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
"Algolia is a powerful search-as-a-service solution, made easy to use with API clients, UI libraries, and pre-built integrations. Algolia API Client for Kotlin lets you easily use the Algolia Search REST API from your JVM project, such as Android or backend implementations."
@file:Suppress("FunctionName")
package com.algolia.search.endpoint.internal
import com.algolia.search.endpoint.EndpointInsights
import com.algolia.search.endpoint.EndpointInsightsUser
import com.algolia.search.model.IndexName
import com.algolia.search.model.ObjectID
import com.algolia.search.model.QueryID
import com.algolia.search.model.filter.Filter
import com.algolia.search.model.insights.EventName
import com.algolia.search.model.insights.InsightsEvent
import com.algolia.search.model.insights.UserToken
import io.ktor.client.statement.HttpResponse
internal class EndpointInsightsUserImpl(
private val insights: EndpointInsights,
private val userToken: UserToken,
) : EndpointInsightsUser {
override suspend fun viewedFilters(
indexName: IndexName,
eventName: EventName,
filters: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.View(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.Filters(filters),
timestamp = timestamp,
userToken = userToken
)
)
}
override suspend fun viewedObjectIDs(
indexName: IndexName,
eventName: EventName,
objectIDs: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.View(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.ObjectIDs(objectIDs),
timestamp = timestamp,
userToken = userToken
)
)
}
override suspend fun clickedFilters(
indexName: IndexName,
eventName: EventName,
filters: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.Click(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.Filters(filters),
timestamp = timestamp,
userToken = userToken
)
)
}
override suspend fun clickedObjectIDs(
indexName: IndexName,
eventName: EventName,
objectIDs: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.Click(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.ObjectIDs(objectIDs),
timestamp = timestamp,
userToken = userToken
)
)
}
override suspend fun clickedObjectIDsAfterSearch(
indexName: IndexName,
eventName: EventName,
queryID: QueryID,
objectIDs: List,
positions: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.Click(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.ObjectIDs(objectIDs),
timestamp = timestamp,
userToken = userToken,
positions = positions,
queryID = queryID
)
)
}
override suspend fun convertedFilters(
indexName: IndexName,
eventName: EventName,
filters: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.Conversion(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.Filters(filters),
timestamp = timestamp,
userToken = userToken
)
)
}
override suspend fun convertedObjectIDs(
indexName: IndexName,
eventName: EventName,
objectIDs: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.Conversion(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.ObjectIDs(objectIDs),
timestamp = timestamp,
userToken = userToken
)
)
}
override suspend fun convertedObjectIDsAfterSearch(
indexName: IndexName,
eventName: EventName,
queryID: QueryID,
objectIDs: List,
timestamp: Long?,
): HttpResponse {
return insights.sendEvent(
InsightsEvent.Conversion(
indexName = indexName,
eventName = eventName,
resources = InsightsEvent.Resources.ObjectIDs(objectIDs),
timestamp = timestamp,
userToken = userToken,
queryID = queryID
)
)
}
}
/**
* Create an [EndpointInsightsUser] instance.
*/
internal fun EndpointInsightsUser(
insights: EndpointInsights,
userToken: UserToken,
): EndpointInsightsUser = EndpointInsightsUserImpl(insights, userToken)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy