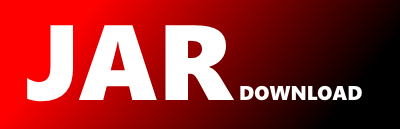
commonMain.com.algolia.client.api.AnalyticsClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
"Algolia is a powerful search-as-a-service solution, made easy to use with API clients, UI libraries, and pre-built integrations. Algolia API Client for Kotlin lets you easily use the Algolia Search REST API from your JVM project, such as Android or backend implementations."
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT. */
package com.algolia.client.api
import com.algolia.client.configuration.*
import com.algolia.client.exception.*
import com.algolia.client.extensions.internal.*
import com.algolia.client.model.analytics.*
import com.algolia.client.transport.*
import com.algolia.client.transport.internal.*
import kotlinx.serialization.json.*
public class AnalyticsClient(
override val appId: String,
override var apiKey: String,
public val region: String? = null,
override val options: ClientOptions = ClientOptions(),
) : ApiClient {
init {
require(appId.isNotBlank()) { "`appId` is missing." }
require(apiKey.isNotBlank()) { "`apiKey` is missing." }
}
override val requester: Requester = requesterOf(clientName = "Analytics", appId = appId, apiKey = apiKey, options = options) {
val allowedRegions = listOf("de", "us")
require(region == null || region in allowedRegions) { "`region` must be one of the following: ${allowedRegions.joinToString()}" }
val url = if (region == null) "analytics.algolia.com" else "analytics.$region.algolia.com"
listOf(Host(url))
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param requestOptions additional request configuration.
*/
public suspend fun customDelete(path: String, parameters: Map? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customDelete`." }
val requestConfig = RequestConfig(
method = RequestMethod.DELETE,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param requestOptions additional request configuration.
*/
public suspend fun customGet(path: String, parameters: Map? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customGet`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param body Parameters to send with the custom request.
* @param requestOptions additional request configuration.
*/
public suspend fun customPost(path: String, parameters: Map? = null, body: JsonObject? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customPost`." }
val requestConfig = RequestConfig(
method = RequestMethod.POST,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
body = body,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param body Parameters to send with the custom request.
* @param requestOptions additional request configuration.
*/
public suspend fun customPut(path: String, parameters: Map? = null, body: JsonObject? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customPut`." }
val requestConfig = RequestConfig(
method = RequestMethod.PUT,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
body = body,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the add-to-cart rate for all of your searches with at least one add-to-cart event, including a daily breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getAddToCartRate(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetAddToCartRateResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getAddToCartRate`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "conversions", "addToCartRate"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the average click position of your search results, including a daily breakdown. The average click position is the average of all clicked search results' positions. For example, if users only ever click on the first result for any search, the average click position is 1. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getAverageClickPosition(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetAverageClickPositionResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getAverageClickPosition`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "clicks", "averageClickPosition"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the positions in the search results and their associated number of clicks. This lets you check how many clicks the first, second, or tenth search results receive.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getClickPositions(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetClickPositionsResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getClickPositions`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "clicks", "positions"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the click-through rate for all of your searches with at least one click event, including a daily breakdown By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getClickThroughRate(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetClickThroughRateResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getClickThroughRate`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "clicks", "clickThroughRate"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the conversion rate for all of your searches with at least one conversion event, including a daily breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getConversionRate(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetConversionRateResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getConversionRate`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "conversions", "conversionRate"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the fraction of searches that didn't lead to any click within a time range, including a daily breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getNoClickRate(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetNoClickRateResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getNoClickRate`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "searches", "noClickRate"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the fraction of searches that didn't return any results within a time range, including a daily breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getNoResultsRate(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetNoResultsRateResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getNoResultsRate`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "searches", "noResultRate"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the purchase rate for all of your searches with at least one purchase event, including a daily breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getPurchaseRate(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetPurchaseRateResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getPurchaseRate`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "conversions", "purchaseRate"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves revenue-related metrics, such as the total revenue or the average order value. To retrieve revenue-related metrics, sent purchase events. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getRevenue(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetRevenue {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getRevenue`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "conversions", "revenue"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the number of searches within a time range, including a daily breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getSearchesCount(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetSearchesCountResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getSearchesCount`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "searches", "count"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the most popular searches that didn't lead to any clicks, from the 1,000 most frequent searches.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getSearchesNoClicks(index: String, startDate: String? = null, endDate: String? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetSearchesNoClicksResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getSearchesNoClicks`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "searches", "noClicks"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the most popular searches that didn't return any results.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getSearchesNoResults(index: String, startDate: String? = null, endDate: String? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetSearchesNoResultsResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getSearchesNoResults`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "searches", "noResults"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the time when the Analytics data for the specified index was last updated. The Analytics data is updated every 5 minutes.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param requestOptions additional request configuration.
*/
public suspend fun getStatus(index: String, requestOptions: RequestOptions? = null): GetStatusResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getStatus`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "status"),
query = buildMap {
put("index", index)
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the countries with the most searches to your index.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getTopCountries(index: String, startDate: String? = null, endDate: String? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetTopCountriesResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getTopCountries`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "countries"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the most frequently used filter attributes. These are attributes of your records that you included in the `attributesForFaceting` setting.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param search Search query.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getTopFilterAttributes(index: String, search: String? = null, startDate: String? = null, endDate: String? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetTopFilterAttributesResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getTopFilterAttributes`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "filters"),
query = buildMap {
put("index", index)
search?.let { put("search", it) }
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the most frequent filter (facet) values for a filter attribute. These are attributes of your records that you included in the `attributesForFaceting` setting.
*
* Required API Key ACLs:
* - analytics
* @param attribute Attribute name.
* @param index Index name.
* @param search Search query.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getTopFilterForAttribute(attribute: String, index: String, search: String? = null, startDate: String? = null, endDate: String? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetTopFilterForAttributeResponse {
require(attribute.isNotBlank()) { "Parameter `attribute` is required when calling `getTopFilterForAttribute`." }
require(index.isNotBlank()) { "Parameter `index` is required when calling `getTopFilterForAttribute`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "filters", "$attribute"),
query = buildMap {
put("index", index)
search?.let { put("search", it) }
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the most frequently used filters for a search that didn't return any results. To get the most frequent searches without results, use the [Retrieve searches without results](#tag/search/operation/getSearchesNoResults) operation.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param search Search query.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getTopFiltersNoResults(index: String, search: String? = null, startDate: String? = null, endDate: String? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetTopFiltersNoResultsResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getTopFiltersNoResults`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "filters", "noResults"),
query = buildMap {
put("index", index)
search?.let { put("search", it) }
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the object IDs of the most frequent search results.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param search Search query.
* @param clickAnalytics Whether to include metrics related to click and conversion events in the response. (default to false)
* @param revenueAnalytics Whether to include revenue-related metrics in the response. If true, metrics related to click and conversion events are also included in the response. (default to false)
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getTopHits(index: String, search: String? = null, clickAnalytics: Boolean? = null, revenueAnalytics: Boolean? = null, startDate: String? = null, endDate: String? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetTopHitsResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getTopHits`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "hits"),
query = buildMap {
put("index", index)
search?.let { put("search", it) }
clickAnalytics?.let { put("clickAnalytics", it) }
revenueAnalytics?.let { put("revenueAnalytics", it) }
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Returns the most popular search terms.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param clickAnalytics Whether to include metrics related to click and conversion events in the response. (default to false)
* @param revenueAnalytics Whether to include revenue-related metrics in the response. If true, metrics related to click and conversion events are also included in the response. (default to false)
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param orderBy Attribute by which to order the response items. If the `clickAnalytics` parameter is false, only `searchCount` is available. (default to searchCount)
* @param direction Sorting direction of the results: ascending or descending. (default to asc)
* @param limit Number of items to return. (default to 10)
* @param offset Position of the first item to return. (default to 0)
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getTopSearches(index: String, clickAnalytics: Boolean? = null, revenueAnalytics: Boolean? = null, startDate: String? = null, endDate: String? = null, orderBy: OrderBy? = null, direction: Direction? = null, limit: Int? = null, offset: Int? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetTopSearchesResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getTopSearches`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "searches"),
query = buildMap {
put("index", index)
clickAnalytics?.let { put("clickAnalytics", it) }
revenueAnalytics?.let { put("revenueAnalytics", it) }
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
orderBy?.let { put("orderBy", it) }
direction?.let { put("direction", it) }
limit?.let { put("limit", it) }
offset?.let { put("offset", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the number of unique users within a time range, including a daily breakdown. Since this endpoint returns the number of unique users, the sum of the daily values might be different from the total number. By default, Algolia distinguishes search users by their IP address, _unless_ you include a pseudonymous user identifier in your search requests with the `userToken` API parameter or `x-algolia-usertoken` request header. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
* @param index Index name.
* @param startDate Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be URL-encoded. For more information, see [Segment your analytics data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
* @param requestOptions additional request configuration.
*/
public suspend fun getUsersCount(index: String, startDate: String? = null, endDate: String? = null, tags: String? = null, requestOptions: RequestOptions? = null): GetUsersCountResponse {
require(index.isNotBlank()) { "Parameter `index` is required when calling `getUsersCount`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("2", "users", "count"),
query = buildMap {
put("index", index)
startDate?.let { put("startDate", it) }
endDate?.let { put("endDate", it) }
tags?.let { put("tags", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy