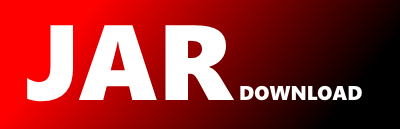
commonMain.com.algolia.client.api.RecommendClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
"Algolia is a powerful search-as-a-service solution, made easy to use with API clients, UI libraries, and pre-built integrations. Algolia API Client for Kotlin lets you easily use the Algolia Search REST API from your JVM project, such as Android or backend implementations."
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT. */
package com.algolia.client.api
import com.algolia.client.configuration.*
import com.algolia.client.exception.*
import com.algolia.client.extensions.internal.*
import com.algolia.client.model.recommend.*
import com.algolia.client.transport.*
import com.algolia.client.transport.internal.*
import kotlinx.serialization.json.*
public class RecommendClient(
override val appId: String,
override var apiKey: String,
override val options: ClientOptions = ClientOptions(),
) : ApiClient {
init {
require(appId.isNotBlank()) { "`appId` is missing." }
require(apiKey.isNotBlank()) { "`apiKey` is missing." }
}
override val requester: Requester = requesterOf(clientName = "Recommend", appId = appId, apiKey = apiKey, options = options) {
listOf(
Host("$appId-dsn.algolia.net", CallType.Read),
Host("$appId.algolia.net", CallType.Write),
) + mutableListOf(
Host("$appId-1.algolianet.com"),
Host("$appId-2.algolianet.com"),
Host("$appId-3.algolianet.com"),
).apply { shuffle() }
}
/**
* Create or update a batch of Recommend Rules Each Recommend Rule is created or updated, depending on whether a Recommend Rule with the same `objectID` already exists. You may also specify `true` for `clearExistingRules`, in which case the batch will atomically replace all the existing Recommend Rules. Recommend Rules are similar to Search Rules, except that the conditions and consequences apply to a [source item](/doc/guides/algolia-recommend/overview/#recommend-models) instead of a query. The main differences are the following: - Conditions `pattern` and `anchoring` are unavailable. - Condition `filters` triggers if the source item matches the specified filters. - Condition `filters` accepts numeric filters. - Consequence `params` only covers filtering parameters. - Consequence `automaticFacetFilters` doesn't require a facet value placeholder (it tries to match the data source item's attributes instead).
*
* Required API Key ACLs:
* - editSettings
* @param indexName Name of the index on which to perform the operation.
* @param model [Recommend model](https://www.algolia.com/doc/guides/algolia-recommend/overview/#recommend-models).
* @param recommendRule
* @param requestOptions additional request configuration.
*/
public suspend fun batchRecommendRules(indexName: String, model: RecommendModels, recommendRule: List? = null, requestOptions: RequestOptions? = null): RecommendUpdatedAtResponse {
require(indexName.isNotBlank()) { "Parameter `indexName` is required when calling `batchRecommendRules`." }
val requestConfig = RequestConfig(
method = RequestMethod.POST,
path = listOf("1", "indexes", "$indexName", "$model", "recommend", "rules", "batch"),
body = recommendRule,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param requestOptions additional request configuration.
*/
public suspend fun customDelete(path: String, parameters: Map? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customDelete`." }
val requestConfig = RequestConfig(
method = RequestMethod.DELETE,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param requestOptions additional request configuration.
*/
public suspend fun customGet(path: String, parameters: Map? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customGet`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param body Parameters to send with the custom request.
* @param requestOptions additional request configuration.
*/
public suspend fun customPost(path: String, parameters: Map? = null, body: JsonObject? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customPost`." }
val requestConfig = RequestConfig(
method = RequestMethod.POST,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
body = body,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param body Parameters to send with the custom request.
* @param requestOptions additional request configuration.
*/
public suspend fun customPut(path: String, parameters: Map? = null, body: JsonObject? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customPut`." }
val requestConfig = RequestConfig(
method = RequestMethod.PUT,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
body = body,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Deletes a Recommend rule from a recommendation scenario.
*
* Required API Key ACLs:
* - editSettings
* @param indexName Name of the index on which to perform the operation.
* @param model [Recommend model](https://www.algolia.com/doc/guides/algolia-recommend/overview/#recommend-models).
* @param objectID Unique record identifier.
* @param requestOptions additional request configuration.
*/
public suspend fun deleteRecommendRule(indexName: String, model: RecommendModels, objectID: String, requestOptions: RequestOptions? = null): DeletedAtResponse {
require(indexName.isNotBlank()) { "Parameter `indexName` is required when calling `deleteRecommendRule`." }
require(objectID.isNotBlank()) { "Parameter `objectID` is required when calling `deleteRecommendRule`." }
val requestConfig = RequestConfig(
method = RequestMethod.DELETE,
path = listOf("1", "indexes", "$indexName", "$model", "recommend", "rules", "$objectID"),
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves a Recommend rule that you previously created in the Algolia dashboard.
*
* Required API Key ACLs:
* - settings
* @param indexName Name of the index on which to perform the operation.
* @param model [Recommend model](https://www.algolia.com/doc/guides/algolia-recommend/overview/#recommend-models).
* @param objectID Unique record identifier.
* @param requestOptions additional request configuration.
*/
public suspend fun getRecommendRule(indexName: String, model: RecommendModels, objectID: String, requestOptions: RequestOptions? = null): RecommendRule {
require(indexName.isNotBlank()) { "Parameter `indexName` is required when calling `getRecommendRule`." }
require(objectID.isNotBlank()) { "Parameter `objectID` is required when calling `getRecommendRule`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("1", "indexes", "$indexName", "$model", "recommend", "rules", "$objectID"),
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Checks the status of a given task. Deleting a Recommend rule is asynchronous. When you delete a rule, a task is created on a queue and completed depending on the load on the server. The API response includes a task ID that you can use to check the status.
*
* Required API Key ACLs:
* - editSettings
* @param indexName Name of the index on which to perform the operation.
* @param model [Recommend model](https://www.algolia.com/doc/guides/algolia-recommend/overview/#recommend-models).
* @param taskID Unique task identifier.
* @param requestOptions additional request configuration.
*/
public suspend fun getRecommendStatus(indexName: String, model: RecommendModels, taskID: Long, requestOptions: RequestOptions? = null): GetRecommendTaskResponse {
require(indexName.isNotBlank()) { "Parameter `indexName` is required when calling `getRecommendStatus`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("1", "indexes", "$indexName", "$model", "task", "$taskID"),
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves recommendations from selected AI models.
*
* Required API Key ACLs:
* - search
* @param getRecommendationsParams
* @param requestOptions additional request configuration.
*/
public suspend fun getRecommendations(getRecommendationsParams: GetRecommendationsParams, requestOptions: RequestOptions? = null): GetRecommendationsResponse {
val requestConfig = RequestConfig(
method = RequestMethod.POST,
path = listOf("1", "indexes", "*", "recommendations"),
isRead = true,
body = getRecommendationsParams,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Searches for Recommend rules. Use an empty query to list all rules for this recommendation scenario.
*
* Required API Key ACLs:
* - settings
* @param indexName Name of the index on which to perform the operation.
* @param model [Recommend model](https://www.algolia.com/doc/guides/algolia-recommend/overview/#recommend-models).
* @param searchRecommendRulesParams
* @param requestOptions additional request configuration.
*/
public suspend fun searchRecommendRules(indexName: String, model: RecommendModels, searchRecommendRulesParams: SearchRecommendRulesParams? = null, requestOptions: RequestOptions? = null): SearchRecommendRulesResponse {
require(indexName.isNotBlank()) { "Parameter `indexName` is required when calling `searchRecommendRules`." }
val requestConfig = RequestConfig(
method = RequestMethod.POST,
path = listOf("1", "indexes", "$indexName", "$model", "recommend", "rules", "search"),
isRead = true,
body = searchRecommendRulesParams,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy