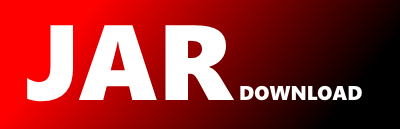
commonMain.com.algolia.client.model.ingestion.SourceInput.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
"Algolia is a powerful search-as-a-service solution, made easy to use with API clients, UI libraries, and pre-built integrations. Algolia API Client for Kotlin lets you easily use the Algolia Search REST API from your JVM project, such as Android or backend implementations."
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT. */
package com.algolia.client.model.ingestion
import com.algolia.client.exception.AlgoliaClientException
import com.algolia.client.extensions.internal.*
import kotlinx.serialization.*
import kotlinx.serialization.builtins.*
import kotlinx.serialization.descriptors.*
import kotlinx.serialization.encoding.*
import kotlinx.serialization.json.*
import kotlin.jvm.JvmInline
/**
* SourceInput
*
* Implementations:
* - [SourceBigCommerce]
* - [SourceBigQuery]
* - [SourceCSV]
* - [SourceCommercetools]
* - [SourceDocker]
* - [SourceGA4BigQueryExport]
* - [SourceJSON]
* - [SourceShopify]
*/
@Serializable(SourceInputSerializer::class)
public sealed interface SourceInput {
@Serializable
@JvmInline
public value class SourceDockerValue(public val value: SourceDocker) : SourceInput
@Serializable
@JvmInline
public value class SourceGA4BigQueryExportValue(public val value: SourceGA4BigQueryExport) : SourceInput
@Serializable
@JvmInline
public value class SourceCommercetoolsValue(public val value: SourceCommercetools) : SourceInput
@Serializable
@JvmInline
public value class SourceBigCommerceValue(public val value: SourceBigCommerce) : SourceInput
@Serializable
@JvmInline
public value class SourceBigQueryValue(public val value: SourceBigQuery) : SourceInput
@Serializable
@JvmInline
public value class SourceShopifyValue(public val value: SourceShopify) : SourceInput
@Serializable
@JvmInline
public value class SourceJSONValue(public val value: SourceJSON) : SourceInput
@Serializable
@JvmInline
public value class SourceCSVValue(public val value: SourceCSV) : SourceInput
public companion object {
public fun of(value: SourceDocker): SourceInput {
return SourceDockerValue(value)
}
public fun of(value: SourceGA4BigQueryExport): SourceInput {
return SourceGA4BigQueryExportValue(value)
}
public fun of(value: SourceCommercetools): SourceInput {
return SourceCommercetoolsValue(value)
}
public fun of(value: SourceBigCommerce): SourceInput {
return SourceBigCommerceValue(value)
}
public fun of(value: SourceBigQuery): SourceInput {
return SourceBigQueryValue(value)
}
public fun of(value: SourceShopify): SourceInput {
return SourceShopifyValue(value)
}
public fun of(value: SourceJSON): SourceInput {
return SourceJSONValue(value)
}
public fun of(value: SourceCSV): SourceInput {
return SourceCSVValue(value)
}
}
}
internal class SourceInputSerializer : JsonContentPolymorphicSerializer(SourceInput::class) {
override fun selectDeserializer(element: JsonElement): DeserializationStrategy {
return when {
element is JsonObject && element.containsKey("registry") && element.containsKey("image") && element.containsKey("imageType") && element.containsKey("configuration") -> SourceDocker.serializer()
element is JsonObject && element.containsKey("projectID") && element.containsKey("datasetID") && element.containsKey("tablePrefix") -> SourceGA4BigQueryExport.serializer()
element is JsonObject && element.containsKey("projectKey") -> SourceCommercetools.serializer()
element is JsonObject && element.containsKey("storeHash") -> SourceBigCommerce.serializer()
element is JsonObject && element.containsKey("projectID") -> SourceBigQuery.serializer()
element is JsonObject && element.containsKey("shopURL") -> SourceShopify.serializer()
element is JsonObject -> SourceJSON.serializer()
element is JsonObject -> SourceCSV.serializer()
else -> throw AlgoliaClientException("Failed to deserialize json element: $element")
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy