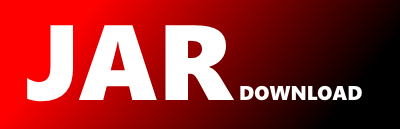
commonMain.com.algolia.client.model.search.SearchResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
Show all versions of algoliasearch-client-kotlin-jvm Show documentation
"Algolia is a powerful search-as-a-service solution, made easy to use with API clients, UI libraries, and pre-built integrations. Algolia API Client for Kotlin lets you easily use the Algolia Search REST API from your JVM project, such as Android or backend implementations."
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT. */
package com.algolia.client.model.search
import com.algolia.client.extensions.internal.*
import kotlinx.serialization.*
import kotlinx.serialization.descriptors.*
import kotlinx.serialization.encoding.*
import kotlinx.serialization.json.*
/**
* SearchResponse
*
* @param processingTimeMS Time the server took to process the request, in milliseconds.
* @param hits Search results (hits). Hits are records from your index that match the search criteria, augmented with additional attributes, such as, for highlighting.
* @param query Search query.
* @param params URL-encoded string of all search parameters.
* @param abTestID A/B test ID. This is only included in the response for indices that are part of an A/B test.
* @param abTestVariantID Variant ID. This is only included in the response for indices that are part of an A/B test.
* @param aroundLatLng Computed geographical location.
* @param automaticRadius Distance from a central coordinate provided by `aroundLatLng`.
* @param exhaustive
* @param appliedRules Rules applied to the query.
* @param exhaustiveFacetsCount See the `facetsCount` field of the `exhaustive` object in the response.
* @param exhaustiveNbHits See the `nbHits` field of the `exhaustive` object in the response.
* @param exhaustiveTypo See the `typo` field of the `exhaustive` object in the response.
* @param facets Facet counts.
* @param facetsStats Statistics for numerical facets.
* @param index Index name used for the query.
* @param indexUsed Index name used for the query. During A/B testing, the targeted index isn't always the index used by the query.
* @param message Warnings about the query.
* @param nbSortedHits Number of hits selected and sorted by the relevant sort algorithm.
* @param parsedQuery Post-[normalization](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/#what-does-normalization-mean) query string that will be searched.
* @param processingTimingsMS Experimental. List of processing steps and their times, in milliseconds. You can use this list to investigate performance issues.
* @param queryAfterRemoval Markup text indicating which parts of the original query have been removed to retrieve a non-empty result set.
* @param redirect
* @param renderingContent
* @param serverTimeMS Time the server took to process the request, in milliseconds.
* @param serverUsed Host name of the server that processed the request.
* @param userData An object with custom data. You can store up to 32kB as custom data.
* @param queryID Unique identifier for the query. This is used for [click analytics](https://www.algolia.com/doc/guides/analytics/click-analytics/).
* @param automaticInsights Whether automatic events collection is enabled for the application.
* @param page Page of search results to retrieve.
* @param nbHits Number of results (hits).
* @param nbPages Number of pages of results.
* @param hitsPerPage Number of hits per page.
*/
@Serializable(SearchResponseSerializer::class)
public data class SearchResponse(
/** Time the server took to process the request, in milliseconds. */
val processingTimeMS: Int,
/** Search results (hits). Hits are records from your index that match the search criteria, augmented with additional attributes, such as, for highlighting. */
val hits: List,
/** Search query. */
val query: String,
/** URL-encoded string of all search parameters. */
val params: String,
/** A/B test ID. This is only included in the response for indices that are part of an A/B test. */
val abTestID: Int? = null,
/** Variant ID. This is only included in the response for indices that are part of an A/B test. */
val abTestVariantID: Int? = null,
/** Computed geographical location. */
val aroundLatLng: String? = null,
/** Distance from a central coordinate provided by `aroundLatLng`. */
val automaticRadius: String? = null,
val exhaustive: Exhaustive? = null,
/** Rules applied to the query. */
val appliedRules: List? = null,
/** See the `facetsCount` field of the `exhaustive` object in the response. */
@Deprecated(message = "This property is deprecated.")
val exhaustiveFacetsCount: Boolean? = null,
/** See the `nbHits` field of the `exhaustive` object in the response. */
@Deprecated(message = "This property is deprecated.")
val exhaustiveNbHits: Boolean? = null,
/** See the `typo` field of the `exhaustive` object in the response. */
@Deprecated(message = "This property is deprecated.")
val exhaustiveTypo: Boolean? = null,
/** Facet counts. */
val facets: Map>? = null,
/** Statistics for numerical facets. */
val facetsStats: Map? = null,
/** Index name used for the query. */
val index: String? = null,
/** Index name used for the query. During A/B testing, the targeted index isn't always the index used by the query. */
val indexUsed: String? = null,
/** Warnings about the query. */
val message: String? = null,
/** Number of hits selected and sorted by the relevant sort algorithm. */
val nbSortedHits: Int? = null,
/** Post-[normalization](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/#what-does-normalization-mean) query string that will be searched. */
val parsedQuery: String? = null,
/** Experimental. List of processing steps and their times, in milliseconds. You can use this list to investigate performance issues. */
val processingTimingsMS: JsonObject? = null,
/** Markup text indicating which parts of the original query have been removed to retrieve a non-empty result set. */
val queryAfterRemoval: String? = null,
val redirect: Redirect? = null,
val renderingContent: RenderingContent? = null,
/** Time the server took to process the request, in milliseconds. */
val serverTimeMS: Int? = null,
/** Host name of the server that processed the request. */
val serverUsed: String? = null,
/** An object with custom data. You can store up to 32kB as custom data. */
val userData: JsonObject? = null,
/** Unique identifier for the query. This is used for [click analytics](https://www.algolia.com/doc/guides/analytics/click-analytics/). */
val queryID: String? = null,
/** Whether automatic events collection is enabled for the application. */
val automaticInsights: Boolean? = null,
/** Page of search results to retrieve. */
val page: Int? = null,
/** Number of results (hits). */
val nbHits: Int? = null,
/** Number of pages of results. */
val nbPages: Int? = null,
/** Number of hits per page. */
val hitsPerPage: Int? = null,
val additionalProperties: Map? = null,
) : SearchResult
internal object SearchResponseSerializer : KSerializer {
override val descriptor: SerialDescriptor = buildClassSerialDescriptor("SearchResponse") {
element("processingTimeMS")
element>("hits")
element("query")
element("params")
element("abTestID", isOptional = true)
element("abTestVariantID", isOptional = true)
element("aroundLatLng", isOptional = true)
element("automaticRadius", isOptional = true)
element("exhaustive", isOptional = true)
element>("appliedRules", isOptional = true)
element("exhaustiveFacetsCount", isOptional = true)
element("exhaustiveNbHits", isOptional = true)
element("exhaustiveTypo", isOptional = true)
element
© 2015 - 2024 Weber Informatics LLC | Privacy Policy