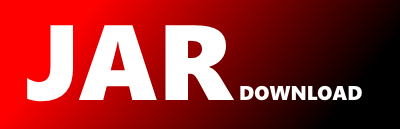
commonMain.com.algolia.client.api.UsageClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-client-kotlin Show documentation
Show all versions of algoliasearch-client-kotlin Show documentation
"Algolia is a powerful search-as-a-service solution, made easy to use with API clients, UI libraries, and pre-built integrations. Algolia API Client for Kotlin lets you easily use the Algolia Search REST API from your JVM project, such as Android or backend implementations."
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT. */
package com.algolia.client.api
import com.algolia.client.configuration.*
import com.algolia.client.exception.*
import com.algolia.client.extensions.internal.*
import com.algolia.client.model.usage.*
import com.algolia.client.transport.*
import com.algolia.client.transport.internal.*
import kotlinx.serialization.json.*
public class UsageClient(
override val appId: String,
override var apiKey: String,
override val options: ClientOptions = ClientOptions(),
) : ApiClient {
init {
require(appId.isNotBlank()) { "`appId` is missing." }
require(apiKey.isNotBlank()) { "`apiKey` is missing." }
}
override val requester: Requester = requesterOf(clientName = "Usage", appId = appId, apiKey = apiKey, options = options) {
listOf(
Host("usage.algolia.com"),
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param requestOptions additional request configuration.
*/
public suspend fun customDelete(path: String, parameters: Map? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customDelete`." }
val requestConfig = RequestConfig(
method = RequestMethod.DELETE,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param requestOptions additional request configuration.
*/
public suspend fun customGet(path: String, parameters: Map? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customGet`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param body Parameters to send with the custom request.
* @param requestOptions additional request configuration.
*/
public suspend fun customPost(path: String, parameters: Map? = null, body: JsonObject? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customPost`." }
val requestConfig = RequestConfig(
method = RequestMethod.POST,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
body = body,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* This method allow you to send requests to the Algolia REST API.
* @param path Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters Query parameters to apply to the current query.
* @param body Parameters to send with the custom request.
* @param requestOptions additional request configuration.
*/
public suspend fun customPut(path: String, parameters: Map? = null, body: JsonObject? = null, requestOptions: RequestOptions? = null): JsonObject {
require(path.isNotBlank()) { "Parameter `path` is required when calling `customPut`." }
val requestConfig = RequestConfig(
method = RequestMethod.PUT,
path = "/{path}".replace("{path}", path),
query = buildMap {
parameters?.let { putAll(it) }
},
body = body,
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves the selected usage statistics for one index.
* @param statistic Usage statistics to retrieve. Use `*` to retrieve all usage metrics, otherwise add one or more of the following metrics, separated by a comma. **Search operations** - `search_operations`. All search operations. - `total_search_operations`: Sum of all search operations. - `total_search_requests`: Sum of all [search requests](https://support.algolia.com/hc/en-us/articles/4406981829777-How-does-Algolia-count-records-and-operations-). The number of billed search requests is equal to this value minus `querysuggestions_total_search_requests`. - `queries_operations`. Number of [single index search](/specs/search#tag/Search/operation/searchSingleIndex) operations. - `multi_queries_operations`. Number of [multi-index search](/specs/search#tag/Search/operation/search) operations. **ACL operations** - `acl_operations`. All ACL operations. - `total_acl_operations`. Sum of all ACL operations. - `get_api_keys_operations`. Number of [list API keys](/specs/search#tag/Api-Keys/operation/listApiKeys) operations. - `get_api_key_operations`. Number of [get API key permission](/specs/search#tag/Api-Keys/operation/getApiKey) operations. - `add_api_key_operations`. Number of [create API key](/specs/search#tag/Api-Keys/operation/addApiKey) operations. - `update_api_key_operations`. Number of [update API key](/specs/search#tag/Api-Keys/operation/updateApiKey) operations. - `delete_api_key_operations`. Number of [delete API key](/specs/search#tag/Api-Keys/operation/deleteApiKey) operations. - `list_api_key_operations`. Number of list index API keys operations. **Indexing operations** - `indexing_operations`. All indexing operations. - `total_indexing_operations`. Sum of all indexing operations. - `browse_operations`. Number of [browse index](/specs/search#tag/Search/operation/browse) operations. - `clear_index_operations`. Number of [clear records](/specs/search#tag/Records/operation/clearObjects) operations. - `copy_move_operations`. Number of [copy or move index](/specs/search#tag/Indices/operation/operationIndex) operations. - `delete_index_operations`. Number of [delete index](/specs/search#tag/Indices/operation/deleteIndex) operations. - `get_log_operations`. Number of [get logs](/specs/search#tag/Advanced/operation/getLogs) operations. - `get_settings_operations`. Number of [get settings](/specs/search#operation/getIndexUsage) operations. - `set_settings_operations`. Number of [set settings](/specs/search#tag/Indices/operation/setSettings) operations. - `list_indices_operations`. Number of [list indices](/specs/search#tag/Indices/operation/listIndices) operations. - `wait_task_operations`. Number of [wait](/specs/search#tag/Indices/operation/getTask) operations. **Record operations** - `record_operations`. All record operations. - `total_records_operations`. Sum of all record operations. - `add_record_operations`. Number of [add or replace record](/specs/search#tag/Records/operation/saveObject) operations. - `batch_operations`. Number of [batch indexing](/specs/search#tag/Records/operation/multipleBatch) operations. - `delete_by_query_operations`. Number of [delete by query](/specs/search#tag/Records/operation/deleteBy) operations. - `delete_record_operations`. Number of [delete record](/specs/search#tag/Records/operation/deleteObject) operations. - `get_record_operations`. Number of [get record](/specs/search#tag/Records/operation/getObject) operations. - `partial_update_record_operations`. Number of [partially update records](/specs/search#tag/Records/operation/partialUpdateObject) operations. - `update_record_operations`. Number of [add or replace record by objectID](/specs/search#tag/Records/operation/addOrUpdateObject) operations. **Synonym operations** - `synonym_operations`. All synonym operations. - `total_synonym_operations`. Sum of all synonym operations. - `batch_synonym_operations`. Number of [save all synonyms](/specs/search#tag/Synonyms/operation/saveSynonyms) operations. - `clear_synonym_operations`. Number of [clear synonyms](/specs/search#tag/Synonyms/operation/clearSynonyms) operations. - `delete_synonym_operations`. Number of [delete synonym](/specs/search#tag/Synonyms/operation/deleteSynonym) operations. - `get_synonym_operations`. Number of [get synonym](/specs/search#tag/Synonyms/operation/getSynonym) operations. - `query_synonym_operations`. Number of [search synonyms](/specs/search#tag/Synonyms/operation/searchSynonyms) operations. - `update_synonym_operations`. Number of [save a synonym](/specs/search#tag/Synonyms/operation/saveSynonym) operations. **Rule operations** - `rule_operations`. All rule operations. - `total_rules_operations`. Sum of all rule operations. - `batch_rules_operations`. Number of [batch rules](/specs/search#tag/Rules/operation/saveRules) operations. - `clear_rules_operations`. Number of [delete rule](/specs/search#tag/Rules/operation/deleteRule) operations. - `delete_rules_operations`. Number of [clear rules](/specs/search#tag/Rules/operation/clearRules) operations. - `get_rules_operations`. Number of [get rule](/specs/search#tag/Rules/operation/getRule) operations. - `save_rules_operations`. Number of [save rule](/specs/search#operation/getIndexUsage) operations. - `search_rules_operations`. Number of [search rules](/specs/search#tag/Rules/operation/searchRules) operations. **Total operations** - `total_recommend_requests`. Number of [Recommend requests](https://www.algolia.com/doc/guides/algolia-ai/recommend/) - `total_write_operations`. Number of Write operations - `total_read_operations`. Number of read operations - `total_operations`. Sum of all operations **Total Query Suggestions operations** Query Suggestions operations are a subset of `total_search_operations`. - `querysuggestions_total_search_operations`. Number of Query Suggestions search operations. - `querysuggestions_total_search_requests`. Number of Query Suggestions [search requests](https://support.algolia.com/hc/en-us/articles/4406981829777-How-does-Algolia-count-records-and-operations-). - `querysuggestions_total_acl_operations`. Sum of all Query Suggestions [ACL operations](#acl-operations). - `querysuggestions_total_indexing_operations`. Number of Query Suggestions [indexing operations](#indexing-operations). - `querysuggestions_total_records_operations`. Number of Query Suggestions [record operations](#record-operations). - `querysuggestions_total_synonym_operations`. Number of Query Suggestions [synonym operations](#synonym-operations). - `querysuggestions_total_rules_operations`. Number of Query Suggestions [Rule operations](#rule-operations). - `querysuggestions_total_write_operations`. Number of Query Suggestions Write operations. - `querysuggestions_total_read_operations`. Number of Query Suggestions Read operations. - `querysuggestions_total_operations`. Sum of all Query Suggestions operations. **Processing time** - `avg_processing_time`. Average processing time (in milliseconds). - `90p_processing_time`. 90th percentile of processing time (in milliseconds). - `99p_processing_time`. 99th percentile of processing time (in milliseconds). - `queries_above_last_ms_processing_time`. Number of queries that take one or more seconds to process. **Indices** - `records`. Number of records. - `data_size`. The size of the records (in bytes). - `file_size`. The size of the records _and_ index metadata (in bytes). **Maximum queries per second** - `max_qps`. [Maximum queries per second](https://support.algolia.com/hc/en-us/articles/4406975224721) per server. - `region_max_qps`. Maximum queries per second per region. - `total_max_qps`. Maximum queries per second across all servers. **Used search capacity** The following capacities are reported in percent: - `used_search_capacity`. Maximum search capacity used per server. - `avg_used_search_capacity`. Average search capacity used per server. - `region_used_search_capacity`. Maximum search capacity used per region. - `region_avg_used_search_capacity`. Average search capacity used per region. - `total_used_search_capacity`. Maximum search capacity used for all servers. - `total_avg_used_search_capacity`. Average used search capacity for all servers. **Degraded queries** Check the impact of [degraded queries](https://support.algolia.com/hc/en-us/articles/4406981934481). - `degraded_queries_ssd_used_queries_impacted`. Percentage of degraded queries due to the Algolia search engine having to read from the server's SSD. - `degraded_queries_ssd_used_seconds_impacted`. Percentage of seconds affected by `ssd_used` degraded queries. - `degraded_queries_max_capacity_queries_impacted`. Percentage of degraded queries due to all search threads being used. - `degraded_queries_max_capacity_seconds_impacted`. Percentage of seconds affected by `max_capacity` degraded queries.
* @param indexName Name of the index on which to perform the operation.
* @param startDate Start date of the period to analyze, in RFC 3339 format.
* @param endDate End date of the period to analyze, in RFC 3339 format.
* @param granularity Granularity of the aggregated metrics. - `hourly`: the maximum time range for hourly metrics is 7 days. - `daily`: the maximum time range for daily metrics is 365 days. (default to daily)
* @param requestOptions additional request configuration.
*/
public suspend fun getIndexUsage(statistic: Statistic, indexName: String, startDate: String, endDate: String, granularity: Granularity? = null, requestOptions: RequestOptions? = null): IndexUsage {
require(indexName.isNotBlank()) { "Parameter `indexName` is required when calling `getIndexUsage`." }
require(startDate.isNotBlank()) { "Parameter `startDate` is required when calling `getIndexUsage`." }
require(endDate.isNotBlank()) { "Parameter `endDate` is required when calling `getIndexUsage`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("1", "usage", "$statistic", "$indexName"),
query = buildMap {
put("startDate", startDate)
put("endDate", endDate)
granularity?.let { put("granularity", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
/**
* Retrieves usage statistics evaluated over a specified period.
* @param statistic Usage statistics to retrieve. Use `*` to retrieve all usage metrics, otherwise add one or more of the following metrics, separated by a comma. **Search operations** - `search_operations`. All search operations. - `total_search_operations`: Sum of all search operations. - `total_search_requests`: Sum of all [search requests](https://support.algolia.com/hc/en-us/articles/4406981829777-How-does-Algolia-count-records-and-operations-). The number of billed search requests is equal to this value minus `querysuggestions_total_search_requests`. - `queries_operations`. Number of [single index search](/specs/search#tag/Search/operation/searchSingleIndex) operations. - `multi_queries_operations`. Number of [multi-index search](/specs/search#tag/Search/operation/search) operations. **ACL operations** - `acl_operations`. All ACL operations. - `total_acl_operations`. Sum of all ACL operations. - `get_api_keys_operations`. Number of [list API keys](/specs/search#tag/Api-Keys/operation/listApiKeys) operations. - `get_api_key_operations`. Number of [get API key permission](/specs/search#tag/Api-Keys/operation/getApiKey) operations. - `add_api_key_operations`. Number of [create API key](/specs/search#tag/Api-Keys/operation/addApiKey) operations. - `update_api_key_operations`. Number of [update API key](/specs/search#tag/Api-Keys/operation/updateApiKey) operations. - `delete_api_key_operations`. Number of [delete API key](/specs/search#tag/Api-Keys/operation/deleteApiKey) operations. - `list_api_key_operations`. Number of list index API keys operations. **Indexing operations** - `indexing_operations`. All indexing operations. - `total_indexing_operations`. Sum of all indexing operations. - `browse_operations`. Number of [browse index](/specs/search#tag/Search/operation/browse) operations. - `clear_index_operations`. Number of [clear records](/specs/search#tag/Records/operation/clearObjects) operations. - `copy_move_operations`. Number of [copy or move index](/specs/search#tag/Indices/operation/operationIndex) operations. - `delete_index_operations`. Number of [delete index](/specs/search#tag/Indices/operation/deleteIndex) operations. - `get_log_operations`. Number of [get logs](/specs/search#tag/Advanced/operation/getLogs) operations. - `get_settings_operations`. Number of [get settings](/specs/search#operation/getIndexUsage) operations. - `set_settings_operations`. Number of [set settings](/specs/search#tag/Indices/operation/setSettings) operations. - `list_indices_operations`. Number of [list indices](/specs/search#tag/Indices/operation/listIndices) operations. - `wait_task_operations`. Number of [wait](/specs/search#tag/Indices/operation/getTask) operations. **Record operations** - `record_operations`. All record operations. - `total_records_operations`. Sum of all record operations. - `add_record_operations`. Number of [add or replace record](/specs/search#tag/Records/operation/saveObject) operations. - `batch_operations`. Number of [batch indexing](/specs/search#tag/Records/operation/multipleBatch) operations. - `delete_by_query_operations`. Number of [delete by query](/specs/search#tag/Records/operation/deleteBy) operations. - `delete_record_operations`. Number of [delete record](/specs/search#tag/Records/operation/deleteObject) operations. - `get_record_operations`. Number of [get record](/specs/search#tag/Records/operation/getObject) operations. - `partial_update_record_operations`. Number of [partially update records](/specs/search#tag/Records/operation/partialUpdateObject) operations. - `update_record_operations`. Number of [add or replace record by objectID](/specs/search#tag/Records/operation/addOrUpdateObject) operations. **Synonym operations** - `synonym_operations`. All synonym operations. - `total_synonym_operations`. Sum of all synonym operations. - `batch_synonym_operations`. Number of [save all synonyms](/specs/search#tag/Synonyms/operation/saveSynonyms) operations. - `clear_synonym_operations`. Number of [clear synonyms](/specs/search#tag/Synonyms/operation/clearSynonyms) operations. - `delete_synonym_operations`. Number of [delete synonym](/specs/search#tag/Synonyms/operation/deleteSynonym) operations. - `get_synonym_operations`. Number of [get synonym](/specs/search#tag/Synonyms/operation/getSynonym) operations. - `query_synonym_operations`. Number of [search synonyms](/specs/search#tag/Synonyms/operation/searchSynonyms) operations. - `update_synonym_operations`. Number of [save a synonym](/specs/search#tag/Synonyms/operation/saveSynonym) operations. **Rule operations** - `rule_operations`. All rule operations. - `total_rules_operations`. Sum of all rule operations. - `batch_rules_operations`. Number of [batch rules](/specs/search#tag/Rules/operation/saveRules) operations. - `clear_rules_operations`. Number of [delete rule](/specs/search#tag/Rules/operation/deleteRule) operations. - `delete_rules_operations`. Number of [clear rules](/specs/search#tag/Rules/operation/clearRules) operations. - `get_rules_operations`. Number of [get rule](/specs/search#tag/Rules/operation/getRule) operations. - `save_rules_operations`. Number of [save rule](/specs/search#operation/getIndexUsage) operations. - `search_rules_operations`. Number of [search rules](/specs/search#tag/Rules/operation/searchRules) operations. **Total operations** - `total_recommend_requests`. Number of [Recommend requests](https://www.algolia.com/doc/guides/algolia-ai/recommend/) - `total_write_operations`. Number of Write operations - `total_read_operations`. Number of read operations - `total_operations`. Sum of all operations **Total Query Suggestions operations** Query Suggestions operations are a subset of `total_search_operations`. - `querysuggestions_total_search_operations`. Number of Query Suggestions search operations. - `querysuggestions_total_search_requests`. Number of Query Suggestions [search requests](https://support.algolia.com/hc/en-us/articles/4406981829777-How-does-Algolia-count-records-and-operations-). - `querysuggestions_total_acl_operations`. Sum of all Query Suggestions [ACL operations](#acl-operations). - `querysuggestions_total_indexing_operations`. Number of Query Suggestions [indexing operations](#indexing-operations). - `querysuggestions_total_records_operations`. Number of Query Suggestions [record operations](#record-operations). - `querysuggestions_total_synonym_operations`. Number of Query Suggestions [synonym operations](#synonym-operations). - `querysuggestions_total_rules_operations`. Number of Query Suggestions [Rule operations](#rule-operations). - `querysuggestions_total_write_operations`. Number of Query Suggestions Write operations. - `querysuggestions_total_read_operations`. Number of Query Suggestions Read operations. - `querysuggestions_total_operations`. Sum of all Query Suggestions operations. **Processing time** - `avg_processing_time`. Average processing time (in milliseconds). - `90p_processing_time`. 90th percentile of processing time (in milliseconds). - `99p_processing_time`. 99th percentile of processing time (in milliseconds). - `queries_above_last_ms_processing_time`. Number of queries that take one or more seconds to process. **Indices** - `records`. Number of records. - `data_size`. The size of the records (in bytes). - `file_size`. The size of the records _and_ index metadata (in bytes). **Maximum queries per second** - `max_qps`. [Maximum queries per second](https://support.algolia.com/hc/en-us/articles/4406975224721) per server. - `region_max_qps`. Maximum queries per second per region. - `total_max_qps`. Maximum queries per second across all servers. **Used search capacity** The following capacities are reported in percent: - `used_search_capacity`. Maximum search capacity used per server. - `avg_used_search_capacity`. Average search capacity used per server. - `region_used_search_capacity`. Maximum search capacity used per region. - `region_avg_used_search_capacity`. Average search capacity used per region. - `total_used_search_capacity`. Maximum search capacity used for all servers. - `total_avg_used_search_capacity`. Average used search capacity for all servers. **Degraded queries** Check the impact of [degraded queries](https://support.algolia.com/hc/en-us/articles/4406981934481). - `degraded_queries_ssd_used_queries_impacted`. Percentage of degraded queries due to the Algolia search engine having to read from the server's SSD. - `degraded_queries_ssd_used_seconds_impacted`. Percentage of seconds affected by `ssd_used` degraded queries. - `degraded_queries_max_capacity_queries_impacted`. Percentage of degraded queries due to all search threads being used. - `degraded_queries_max_capacity_seconds_impacted`. Percentage of seconds affected by `max_capacity` degraded queries.
* @param startDate Start date of the period to analyze, in RFC 3339 format.
* @param endDate End date of the period to analyze, in RFC 3339 format.
* @param granularity Granularity of the aggregated metrics. - `hourly`: the maximum time range for hourly metrics is 7 days. - `daily`: the maximum time range for daily metrics is 365 days. (default to daily)
* @param requestOptions additional request configuration.
*/
public suspend fun getUsage(statistic: Statistic, startDate: String, endDate: String, granularity: Granularity? = null, requestOptions: RequestOptions? = null): IndexUsage {
require(startDate.isNotBlank()) { "Parameter `startDate` is required when calling `getUsage`." }
require(endDate.isNotBlank()) { "Parameter `endDate` is required when calling `getUsage`." }
val requestConfig = RequestConfig(
method = RequestMethod.GET,
path = listOf("1", "usage", "$statistic"),
query = buildMap {
put("startDate", startDate)
put("endDate", endDate)
granularity?.let { put("granularity", it) }
},
)
return requester.execute(
requestConfig = requestConfig,
requestOptions = requestOptions,
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy