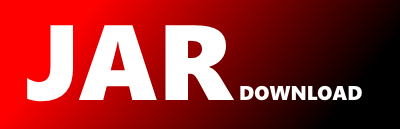
com.algolia.search.util.QueryStringUtils Maven / Gradle / Ivy
package com.algolia.search.util;
import com.algolia.search.Defaults;
import com.algolia.search.models.apikeys.SecuredApiKeyRestriction;
import com.algolia.search.models.indexing.SearchParameters;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.*;
import java.util.stream.Collectors;
public class QueryStringUtils {
/**
* Encode the given string
*
* @param s The string to encode
* @return URL encoded string
*/
public static String urlEncodeUTF8(String s) {
try {
return URLEncoder.encode(s, "UTF-8");
} catch (UnsupportedEncodingException e) {
throw new UnsupportedOperationException(e);
}
}
/**
* Build a query string from its input
*
* @param map The map to convert to a query string
* @param withoutLeadingMark Tells whether or not the leading interrogation mark should be used as
* a prefix of the query string.
*/
public static String buildQueryString(Map map, boolean withoutLeadingMark) {
return withoutLeadingMark ? buildString(map).orElse("") : buildQueryString(map);
}
/**
* Build a query string from its input
*
* @param map The map to convert to a query string
*/
public static String buildQueryString(Map map) {
return buildString(map).map(s -> "?" + s).orElse("");
}
@SuppressWarnings("unchecked")
public static String buildQueryAsQueryParams(SearchParameters query) {
// This could be improved
// We need to create a Map to keep track of the List>
Map map =
Defaults.getObjectMapper().convertValue(query, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy