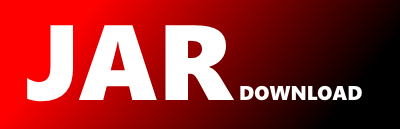
com.algolia.search.models.settings.IndexSettings Maven / Gradle / Ivy
The newest version!
package com.algolia.search.models.settings;
import com.algolia.search.models.rules.RenderingContent;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import java.io.Serializable;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Here is the list of parameters you can use with the set settings method. Parameters that can be
* overridden at search time also have the search scope. More information:
*
* @see Algolia.com
*/
@SuppressWarnings({"unused", "WeakerAccess"})
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class IndexSettings implements Serializable {
/* advanced */
private String attributeForDistinct;
private Distinct distinct;
private Boolean replaceSynonymsInHighlight;
private Integer minProximity;
private Boolean attributeCriteriaComputedByMinProximity;
private List responseFields;
private Integer maxFacetHits;
private String keepDiacriticsOnCharacters;
private List queryLanguages;
private List indexLanguages;
private Map> customNormalization;
private Boolean enablePersonalization;
private List searchableAttributes;
private List attributesForFaceting;
private List unretrievableAttributes;
private List attributesToRetrieve;
private List camelCaseAttributes;
private Map> decompoundedAttributes;
private String primary;
private Map userData;
private List attributesToTransliterate;
private Boolean decompoundQuery;
/* filtering-faceting */
private Long maxValuesPerFacet;
private String sortFacetValuesBy;
/* geo-search */
// nothing on Index Settings
/* highlighting-snippeting */
private List attributesToHighlight;
private List attributesToSnippet;
private String highlightPreTag;
private String highlightPostTag;
private String snippetEllipsisText;
private Boolean restrictHighlightAndSnippetArrays;
/* pagination */
private Long hitsPerPage;
private Long paginationLimitedTo;
private List numericAttributesForFiltering;
private Boolean allowCompressionOfIntegerArray;
/* query rules */
private Boolean enableRules;
/* query strategy */
private String queryType;
private String removeWordsIfNoResults;
private Boolean advancedSyntax;
private List advancedSyntaxFeatures;
private List optionalWords;
private RemoveStopWords removeStopWords;
private List disablePrefixOnAttributes;
private List disableExactOnAttributes;
private String exactOnSingleWordQuery;
private List alternativesAsExact;
/* ranking */
private List ranking;
private List customRanking;
private List replicas;
/* search */
// nothing on Index Settings
/* typos */
private Integer minWordSizefor1Typo;
private Integer minWordSizefor2Typos;
private TypoTolerance typoTolerance;
private Boolean allowTyposOnNumericTokens;
private IgnorePlurals ignorePlurals;
private List disableTypoToleranceOnAttributes;
private List disableTypoToleranceOnWords;
private String separatorsToIndex;
/* custom */
private Map customSettings = new HashMap<>();
/* Virtual Indices */
protected Integer relevancyStrictness;
/* Facets Ordering */
private RenderingContent renderingContent;
public List getAttributesForFaceting() {
return attributesForFaceting;
}
public IndexSettings setAttributesForFaceting(List attributesForFaceting) {
this.attributesForFaceting = attributesForFaceting;
return this;
}
public String getAttributeForDistinct() {
return attributeForDistinct;
}
public IndexSettings setAttributeForDistinct(String attributeForDistinct) {
this.attributeForDistinct = attributeForDistinct;
return this;
}
public List getRanking() {
return ranking;
}
public IndexSettings setRanking(List ranking) {
this.ranking = ranking;
return this;
}
public List getCustomRanking() {
return customRanking;
}
public IndexSettings setCustomRanking(List customRanking) {
this.customRanking = customRanking;
return this;
}
public String getSeparatorsToIndex() {
return separatorsToIndex;
}
public IndexSettings setSeparatorsToIndex(String separatorsToIndex) {
this.separatorsToIndex = separatorsToIndex;
return this;
}
public List getUnretrievableAttributes() {
return unretrievableAttributes;
}
public IndexSettings setUnretrievableAttributes(List unretrievableAttributes) {
this.unretrievableAttributes = unretrievableAttributes;
return this;
}
public Boolean getAllowCompressionOfIntegerArray() {
return allowCompressionOfIntegerArray;
}
public IndexSettings setAllowCompressionOfIntegerArray(Boolean allowCompressionOfIntegerArray) {
this.allowCompressionOfIntegerArray = allowCompressionOfIntegerArray;
return this;
}
public List getDisableTypoToleranceOnWords() {
return disableTypoToleranceOnWords;
}
public IndexSettings setDisableTypoToleranceOnWords(List disableTypoToleranceOnWords) {
this.disableTypoToleranceOnWords = disableTypoToleranceOnWords;
return this;
}
public List getDisableTypoToleranceOnAttributes() {
return disableTypoToleranceOnAttributes;
}
public IndexSettings setDisableTypoToleranceOnAttributes(
List disableTypoToleranceOnAttributes) {
this.disableTypoToleranceOnAttributes = disableTypoToleranceOnAttributes;
return this;
}
public Integer getMinWordSizefor1Typo() {
return minWordSizefor1Typo;
}
public IndexSettings setMinWordSizefor1Typo(Integer minWordSizefor1Typo) {
this.minWordSizefor1Typo = minWordSizefor1Typo;
return this;
}
public Integer getMinWordSizefor2Typos() {
return minWordSizefor2Typos;
}
public IndexSettings setMinWordSizefor2Typos(Integer minWordSizefor2Typos) {
this.minWordSizefor2Typos = minWordSizefor2Typos;
return this;
}
public Long getHitsPerPage() {
return hitsPerPage;
}
public IndexSettings setHitsPerPage(Integer hitsPerPage) {
return this.setHitsPerPage(hitsPerPage.longValue());
}
@JsonSetter
public IndexSettings setHitsPerPage(Long hitsPerPage) {
this.hitsPerPage = hitsPerPage;
return this;
}
public List getAttributesToRetrieve() {
return attributesToRetrieve;
}
public IndexSettings setAttributesToRetrieve(List attributesToRetrieve) {
this.attributesToRetrieve = attributesToRetrieve;
return this;
}
public List getCamelCaseAttributes() {
return camelCaseAttributes;
}
public IndexSettings setCamelCaseAttributes(List camelCaseAttributes) {
this.camelCaseAttributes = camelCaseAttributes;
return this;
}
public Map> getDecompoundedAttributes() {
return decompoundedAttributes;
}
public IndexSettings setDecompoundedAttributes(Map> decompoundedAttributes) {
this.decompoundedAttributes = decompoundedAttributes;
return this;
}
public String getPrimary() {
return primary;
}
public Map getUserData() {
return userData;
}
public IndexSettings setUserData(Map userData) {
this.userData = userData;
return this;
}
public List getAttributesToHighlight() {
return attributesToHighlight;
}
public IndexSettings setAttributesToHighlight(List attributesToHighlight) {
this.attributesToHighlight = attributesToHighlight;
return this;
}
public List getAttributesToSnippet() {
return attributesToSnippet;
}
public IndexSettings setAttributesToSnippet(List attributesToSnippet) {
this.attributesToSnippet = attributesToSnippet;
return this;
}
public String getQueryType() {
return queryType;
}
public IndexSettings setQueryType(String queryType) {
this.queryType = queryType;
return this;
}
public Integer getMinProximity() {
return minProximity;
}
public IndexSettings setMinProximity(Integer minProximity) {
this.minProximity = minProximity;
return this;
}
public Boolean getAttributeCriteriaComputedByMinProximity() {
return attributeCriteriaComputedByMinProximity;
}
public IndexSettings setAttributeCriteriaComputedByMinProximity(
Boolean attributeCriteriaComputedByMinProximity) {
this.attributeCriteriaComputedByMinProximity = attributeCriteriaComputedByMinProximity;
return this;
}
public String getHighlightPreTag() {
return highlightPreTag;
}
public IndexSettings setHighlightPreTag(String highlightPreTag) {
this.highlightPreTag = highlightPreTag;
return this;
}
public String getHighlightPostTag() {
return highlightPostTag;
}
public IndexSettings setHighlightPostTag(String highlightPostTag) {
this.highlightPostTag = highlightPostTag;
return this;
}
public List getOptionalWords() {
return optionalWords;
}
public IndexSettings setOptionalWords(List optionalWords) {
this.optionalWords = optionalWords;
return this;
}
public Boolean getAllowTyposOnNumericTokens() {
return allowTyposOnNumericTokens;
}
public IndexSettings setAllowTyposOnNumericTokens(Boolean allowTyposOnNumericTokens) {
this.allowTyposOnNumericTokens = allowTyposOnNumericTokens;
return this;
}
public IgnorePlurals getIgnorePlurals() {
return ignorePlurals;
}
@JsonIgnore
public IndexSettings setIgnorePlurals(List ignorePlurals) {
return this.setIgnorePlurals(IgnorePlurals.of(ignorePlurals));
}
@JsonProperty
public IndexSettings setIgnorePlurals(IgnorePlurals ignorePlurals) {
this.ignorePlurals = ignorePlurals;
return this;
}
@JsonIgnore
public IndexSettings setIgnorePlurals(Boolean ignorePlurals) {
return this.setIgnorePlurals(IgnorePlurals.of(ignorePlurals));
}
public Boolean getAdvancedSyntax() {
return advancedSyntax;
}
public IndexSettings setAdvancedSyntax(Boolean advancedSyntax) {
this.advancedSyntax = advancedSyntax;
return this;
}
public List getAdvancedSyntaxFeatures() {
return advancedSyntaxFeatures;
}
public IndexSettings setAdvancedSyntaxFeatures(List advancedSyntaxFeatures) {
this.advancedSyntaxFeatures = advancedSyntaxFeatures;
return this;
}
public Boolean getReplaceSynonymsInHighlight() {
return replaceSynonymsInHighlight;
}
public IndexSettings setReplaceSynonymsInHighlight(Boolean replaceSynonymsInHighlight) {
this.replaceSynonymsInHighlight = replaceSynonymsInHighlight;
return this;
}
public Long getMaxValuesPerFacet() {
return maxValuesPerFacet;
}
public IndexSettings setMaxValuesPerFacet(Integer maxValuesPerFacet) {
return this.setMaxValuesPerFacet(maxValuesPerFacet.longValue());
}
@JsonSetter
public IndexSettings setMaxValuesPerFacet(Long maxValuesPerFacet) {
this.maxValuesPerFacet = maxValuesPerFacet;
return this;
}
public Distinct getDistinct() {
return distinct;
}
@JsonIgnore
public IndexSettings setDistinct(Boolean distinct) {
return this.setDistinct(Distinct.of(distinct));
}
@JsonProperty
public IndexSettings setDistinct(Distinct distinct) {
this.distinct = distinct;
return this;
}
@JsonIgnore
public IndexSettings setDistinct(Integer distinct) {
return this.setDistinct(Distinct.of(distinct));
}
public TypoTolerance getTypoTolerance() {
return typoTolerance;
}
public IndexSettings setTypoTolerance(TypoTolerance typoTolerance) {
this.typoTolerance = typoTolerance;
return this;
}
public RemoveStopWords getRemoveStopWords() {
return removeStopWords;
}
@JsonIgnore
public IndexSettings setRemoveStopWords(List removeStopWords) {
return this.setRemoveStopWords(RemoveStopWords.of(removeStopWords));
}
@JsonProperty
public IndexSettings setRemoveStopWords(RemoveStopWords removeStopWords) {
this.removeStopWords = removeStopWords;
return this;
}
@JsonIgnore
public IndexSettings setRemoveStopWords(Boolean removeStopWords) {
return this.setRemoveStopWords(RemoveStopWords.of(removeStopWords));
}
public String getSnippetEllipsisText() {
return snippetEllipsisText;
}
public IndexSettings setSnippetEllipsisText(String snippetEllipsisText) {
this.snippetEllipsisText = snippetEllipsisText;
return this;
}
public String getRemoveWordsIfNoResults() {
return removeWordsIfNoResults;
}
public IndexSettings setRemoveWordsIfNoResults(String removeWordsIfNoResults) {
this.removeWordsIfNoResults = removeWordsIfNoResults;
return this;
}
public List getReplicas() {
return replicas;
}
public IndexSettings setReplicas(List replicas) {
this.replicas = replicas;
return this;
}
// Here for legacy purposes
@JsonSetter("slaves")
public IndexSettings setSlaves(List slaves) {
if (this.replicas == null && slaves != null) {
setReplicas(slaves);
}
return this;
}
public List getSearchableAttributes() {
return searchableAttributes;
}
public IndexSettings setSearchableAttributes(List searchableAttributes) {
this.searchableAttributes = searchableAttributes;
return this;
}
// Here for legacy purposes
@JsonSetter("attributesToIndex")
public IndexSettings setAttributesToIndex(List attributesToIndex) {
if (this.searchableAttributes == null && attributesToIndex != null) {
setSearchableAttributes(attributesToIndex);
}
return this;
}
public List getResponseFields() {
return responseFields;
}
public IndexSettings setResponseFields(List responseFields) {
this.responseFields = responseFields;
return this;
}
public Integer getMaxFacetHits() {
return maxFacetHits;
}
public IndexSettings setMaxFacetHits(Integer maxFacetHits) {
this.maxFacetHits = maxFacetHits;
return this;
}
public Boolean getRestrictHighlightAndSnippetArrays() {
return restrictHighlightAndSnippetArrays;
}
public IndexSettings setRestrictHighlightAndSnippetArrays(
Boolean restrictHighlightAndSnippetArrays) {
this.restrictHighlightAndSnippetArrays = restrictHighlightAndSnippetArrays;
return this;
}
public Long getPaginationLimitedTo() {
return paginationLimitedTo;
}
public IndexSettings setPaginationLimitedTo(Integer paginationLimitedTo) {
return this.setPaginationLimitedTo(paginationLimitedTo.longValue());
}
@JsonSetter
public IndexSettings setPaginationLimitedTo(Long paginationLimitedTo) {
this.paginationLimitedTo = paginationLimitedTo;
return this;
}
public List getNumericAttributesForFiltering() {
return numericAttributesForFiltering;
}
public IndexSettings setNumericAttributesForFiltering(
List numericAttributesForFiltering) {
this.numericAttributesForFiltering = numericAttributesForFiltering;
return this;
}
// Here for legacy purposes
@JsonSetter("numericAttributesToIndex")
public IndexSettings setNumericAttributesToIndex(List numericAttributesToIndex) {
if (this.numericAttributesForFiltering == null && numericAttributesToIndex != null) {
setNumericAttributesForFiltering(numericAttributesToIndex);
}
return this;
}
public List getDisablePrefixOnAttributes() {
return disablePrefixOnAttributes;
}
public IndexSettings setDisablePrefixOnAttributes(List disablePrefixOnAttributes) {
this.disablePrefixOnAttributes = disablePrefixOnAttributes;
return this;
}
public List getDisableExactOnAttributes() {
return disableExactOnAttributes;
}
public IndexSettings setDisableExactOnAttributes(List disableExactOnAttributes) {
this.disableExactOnAttributes = disableExactOnAttributes;
return this;
}
public String getExactOnSingleWordQuery() {
return exactOnSingleWordQuery;
}
public IndexSettings setExactOnSingleWordQuery(String exactOnSingleWordQuery) {
this.exactOnSingleWordQuery = exactOnSingleWordQuery;
return this;
}
public List getAlternativesAsExact() {
return alternativesAsExact;
}
public IndexSettings setAlternativesAsExact(List alternativesAsExact) {
this.alternativesAsExact = alternativesAsExact;
return this;
}
public Boolean getEnableRules() {
return enableRules;
}
public IndexSettings setEnableRules(Boolean enableRules) {
this.enableRules = enableRules;
return this;
}
public String getSortFacetValuesBy() {
return sortFacetValuesBy;
}
public IndexSettings setSortFacetValuesBy(String sortFacetValuesBy) {
this.sortFacetValuesBy = sortFacetValuesBy;
return this;
}
public String getKeepDiacriticsOnCharacters() {
return keepDiacriticsOnCharacters;
}
public IndexSettings setKeepDiacriticsOnCharacters(String keepDiacriticsOnCharacters) {
this.keepDiacriticsOnCharacters = keepDiacriticsOnCharacters;
return this;
}
public List getQueryLanguages() {
return queryLanguages;
}
public IndexSettings setQueryLanguages(List queryLanguages) {
this.queryLanguages = queryLanguages;
return this;
}
public Map> getCustomNormalization() {
return customNormalization;
}
public IndexSettings setCustomNormalization(
Map> customNormalization) {
this.customNormalization = customNormalization;
return this;
}
public List getIndexLanguages() {
return indexLanguages;
}
public IndexSettings setIndexLanguages(List indexLanguages) {
this.indexLanguages = indexLanguages;
return this;
}
@JsonAnySetter
public IndexSettings setCustomSetting(String key, Object value) {
this.customSettings.put(key, value);
return this;
}
@JsonAnyGetter
public Map getCustomSettings() {
return customSettings;
}
@JsonAnySetter
public IndexSettings setCustomSettings(Map customSettings) {
this.customSettings = customSettings;
return this;
}
public Boolean getEnablePersonalization() {
return enablePersonalization;
}
public IndexSettings setEnablePersonalization(Boolean enablePersonalization) {
this.enablePersonalization = enablePersonalization;
return this;
}
public List getAttributesToTransliterate() {
return attributesToTransliterate;
}
public IndexSettings setAttributesToTransliterate(List attributesToTransliterate) {
this.attributesToTransliterate = attributesToTransliterate;
return this;
}
public Boolean getDecompoundQuery() {
return decompoundQuery;
}
public IndexSettings setDecompoundQuery(Boolean decompoundQuery) {
this.decompoundQuery = decompoundQuery;
return this;
}
public Integer getRelevancyStrictness() {
return relevancyStrictness;
}
public IndexSettings setRelevancyStrictness(Integer relevancyStrictness) {
this.relevancyStrictness = relevancyStrictness;
return this;
}
public RenderingContent getRenderingContent() {
return renderingContent;
}
public IndexSettings setRenderingContent(RenderingContent renderingContent) {
this.renderingContent = renderingContent;
return this;
}
@Override
public String toString() {
return "IndexSettings{"
+ "attributeForDistinct='"
+ attributeForDistinct
+ '\''
+ ", distinct="
+ distinct
+ ", replaceSynonymsInHighlight="
+ replaceSynonymsInHighlight
+ ", minProximity="
+ minProximity
+ ", responseFields="
+ responseFields
+ ", maxFacetHits="
+ maxFacetHits
+ ", searchableAttributes="
+ searchableAttributes
+ ", attributesForFaceting="
+ attributesForFaceting
+ ", unretrievableAttributes="
+ unretrievableAttributes
+ ", attributesToRetrieve="
+ attributesToRetrieve
+ ", camelCaseAttributes="
+ camelCaseAttributes
+ ", decompoundedAttributes="
+ decompoundedAttributes
+ ", maxValuesPerFacet="
+ maxValuesPerFacet
+ ", sortFacetValuesBy="
+ sortFacetValuesBy
+ ", attributesToHighlight="
+ attributesToHighlight
+ ", attributesToSnippet="
+ attributesToSnippet
+ ", highlightPreTag='"
+ highlightPreTag
+ '\''
+ ", highlightPostTag='"
+ highlightPostTag
+ '\''
+ ", snippetEllipsisText='"
+ snippetEllipsisText
+ '\''
+ ", restrictHighlightAndSnippetArrays="
+ restrictHighlightAndSnippetArrays
+ ", hitsPerPage="
+ hitsPerPage
+ ", paginationLimitedTo="
+ paginationLimitedTo
+ ", numericAttributesForFiltering="
+ numericAttributesForFiltering
+ ", allowCompressionOfIntegerArray="
+ allowCompressionOfIntegerArray
+ ", enableRules="
+ enableRules
+ ", queryType='"
+ queryType
+ '\''
+ ", removeWordsIfNoResults='"
+ removeWordsIfNoResults
+ '\''
+ ", advancedSyntax="
+ advancedSyntax
+ ", advancedSyntaxFeatures="
+ advancedSyntaxFeatures
+ ", optionalWords="
+ optionalWords
+ ", removeStopWords="
+ removeStopWords
+ ", disablePrefixOnAttributes="
+ disablePrefixOnAttributes
+ ", disableExactOnAttributes="
+ disableExactOnAttributes
+ ", exactOnSingleWordQuery='"
+ exactOnSingleWordQuery
+ '\''
+ ", alternativesAsExact="
+ alternativesAsExact
+ ", ranking="
+ ranking
+ ", customRanking="
+ customRanking
+ ", replicas="
+ replicas
+ ", minWordSizefor1Typo="
+ minWordSizefor1Typo
+ ", minWordSizefor2Typos="
+ minWordSizefor2Typos
+ ", typoTolerance="
+ typoTolerance
+ ", allowTyposOnNumericTokens="
+ allowTyposOnNumericTokens
+ ", ignorePlurals="
+ ignorePlurals
+ ", disableTypoToleranceOnAttributes="
+ disableTypoToleranceOnAttributes
+ ", disableTypoToleranceOnWords="
+ disableTypoToleranceOnWords
+ ", separatorsToIndex='"
+ separatorsToIndex
+ '\''
+ ", keepDiacriticsOnCharacters='"
+ keepDiacriticsOnCharacters
+ '\''
+ ", queryLanguages="
+ queryLanguages
+ '\''
+ ", attributesToTransliterate="
+ attributesToTransliterate
+ '\''
+ ", decompoundQuery="
+ decompoundQuery
+ '\''
+ ", renderingContent="
+ renderingContent
+ '}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy