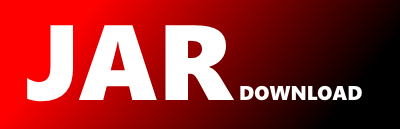
algoliasearch.api.IngestionClient.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-scala_3 Show documentation
Show all versions of algoliasearch-scala_3 Show documentation
Scala client for Algolia Search API
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on
* https://github.com/algolia/api-clients-automation. DO NOT EDIT.
*/
package algoliasearch.api
import algoliasearch.ingestion.ActionType._
import algoliasearch.ingestion.Authentication
import algoliasearch.ingestion.AuthenticationCreate
import algoliasearch.ingestion.AuthenticationCreateResponse
import algoliasearch.ingestion.AuthenticationSearch
import algoliasearch.ingestion.AuthenticationSortKeys._
import algoliasearch.ingestion.AuthenticationType._
import algoliasearch.ingestion.AuthenticationUpdate
import algoliasearch.ingestion.AuthenticationUpdateResponse
import algoliasearch.ingestion.DeleteResponse
import algoliasearch.ingestion.Destination
import algoliasearch.ingestion.DestinationCreate
import algoliasearch.ingestion.DestinationCreateResponse
import algoliasearch.ingestion.DestinationSearch
import algoliasearch.ingestion.DestinationSortKeys._
import algoliasearch.ingestion.DestinationType._
import algoliasearch.ingestion.DestinationUpdate
import algoliasearch.ingestion.DestinationUpdateResponse
import algoliasearch.ingestion.ErrorBase
import algoliasearch.ingestion.Event
import algoliasearch.ingestion.EventSortKeys._
import algoliasearch.ingestion.EventStatus._
import algoliasearch.ingestion.EventType._
import algoliasearch.ingestion.GenerateTransformationCodePayload
import algoliasearch.ingestion.GenerateTransformationCodeResponse
import algoliasearch.ingestion.ListAuthenticationsResponse
import algoliasearch.ingestion.ListDestinationsResponse
import algoliasearch.ingestion.ListEventsResponse
import algoliasearch.ingestion.ListSourcesResponse
import algoliasearch.ingestion.ListTasksResponse
import algoliasearch.ingestion.ListTasksResponseV1
import algoliasearch.ingestion.ListTransformationsResponse
import algoliasearch.ingestion.OrderKeys._
import algoliasearch.ingestion.PlatformWithNone
import algoliasearch.ingestion.PushTaskPayload
import algoliasearch.ingestion.Run
import algoliasearch.ingestion.RunListResponse
import algoliasearch.ingestion.RunResponse
import algoliasearch.ingestion.RunSortKeys._
import algoliasearch.ingestion.RunSourcePayload
import algoliasearch.ingestion.RunSourceResponse
import algoliasearch.ingestion.RunStatus._
import algoliasearch.ingestion.RunType._
import algoliasearch.ingestion.SortKeys._
import algoliasearch.ingestion.Source
import algoliasearch.ingestion.SourceCreate
import algoliasearch.ingestion.SourceCreateResponse
import algoliasearch.ingestion.SourceSearch
import algoliasearch.ingestion.SourceSortKeys._
import algoliasearch.ingestion.SourceType._
import algoliasearch.ingestion.SourceUpdate
import algoliasearch.ingestion.SourceUpdateResponse
import algoliasearch.ingestion.SourceWatchResponse
import algoliasearch.ingestion.Task
import algoliasearch.ingestion.TaskCreate
import algoliasearch.ingestion.TaskCreateResponse
import algoliasearch.ingestion.TaskCreateV1
import algoliasearch.ingestion.TaskSearch
import algoliasearch.ingestion.TaskSortKeys._
import algoliasearch.ingestion.TaskUpdate
import algoliasearch.ingestion.TaskUpdateResponse
import algoliasearch.ingestion.TaskUpdateV1
import algoliasearch.ingestion.TaskV1
import algoliasearch.ingestion.Transformation
import algoliasearch.ingestion.TransformationCreate
import algoliasearch.ingestion.TransformationCreateResponse
import algoliasearch.ingestion.TransformationModels
import algoliasearch.ingestion.TransformationSearch
import algoliasearch.ingestion.TransformationTry
import algoliasearch.ingestion.TransformationTryResponse
import algoliasearch.ingestion.TransformationUpdateResponse
import algoliasearch.ingestion.TriggerType._
import algoliasearch.ingestion._
import algoliasearch.ApiClient
import algoliasearch.api.IngestionClient.hosts
import algoliasearch.config._
import algoliasearch.internal.util._
import scala.concurrent.{ExecutionContext, Future}
import scala.util.Random
object IngestionClient {
/** Creates a new SearchApi instance using default hosts.
*
* @param appId
* application ID
* @param apiKey
* api key
* @param region
* region
* @param clientOptions
* client options
*/
def apply(
appId: String,
apiKey: String,
region: String,
clientOptions: ClientOptions = ClientOptions()
) = new IngestionClient(
appId = appId,
apiKey = apiKey,
region = region,
clientOptions = clientOptions
)
private def hosts(region: String): Seq[Host] = {
val allowedRegions = Seq("eu", "us")
if (!allowedRegions.contains(region)) {
throw new IllegalArgumentException(
s"`region` is required and must be one of the following: ${allowedRegions.mkString(", ")}"
)
}
val url = "data.{region}.algolia.com".replace("{region}", region)
Seq(Host(url = url, callTypes = Set(CallType.Read, CallType.Write)))
}
}
class IngestionClient(
appId: String,
apiKey: String,
region: String,
clientOptions: ClientOptions = ClientOptions()
) extends ApiClient(
appId = appId,
apiKey = apiKey,
clientName = "Ingestion",
defaultHosts = hosts(region),
formats = JsonSupport.format,
options = clientOptions
) {
/** Creates a new authentication resource.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param authenticationCreate
*/
def createAuthentication(authenticationCreate: AuthenticationCreate, requestOptions: Option[RequestOptions] = None)(
implicit ec: ExecutionContext
): Future[AuthenticationCreateResponse] = Future {
requireNotNull(
authenticationCreate,
"Parameter `authenticationCreate` is required when calling `createAuthentication`."
)
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/authentications")
.withBody(authenticationCreate)
.build()
execute[AuthenticationCreateResponse](request, requestOptions)
}
/** Creates a new destination.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param destinationCreate
*/
def createDestination(destinationCreate: DestinationCreate, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[DestinationCreateResponse] = Future {
requireNotNull(destinationCreate, "Parameter `destinationCreate` is required when calling `createDestination`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/destinations")
.withBody(destinationCreate)
.build()
execute[DestinationCreateResponse](request, requestOptions)
}
/** Creates a new source.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceCreate
*/
def createSource(sourceCreate: SourceCreate, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[SourceCreateResponse] = Future {
requireNotNull(sourceCreate, "Parameter `sourceCreate` is required when calling `createSource`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/sources")
.withBody(sourceCreate)
.build()
execute[SourceCreateResponse](request, requestOptions)
}
/** Creates a new task.
*
* @param taskCreate
* Request body for creating a task.
*/
def createTask(taskCreate: TaskCreate, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskCreateResponse] = Future {
requireNotNull(taskCreate, "Parameter `taskCreate` is required when calling `createTask`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/2/tasks")
.withBody(taskCreate)
.build()
execute[TaskCreateResponse](request, requestOptions)
}
/** Creates a new task using the v1 endpoint, please use `createTask` instead.
*
* @param taskCreate
* Request body for creating a task.
*/
def createTaskV1(taskCreate: TaskCreateV1, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskCreateResponse] = Future {
requireNotNull(taskCreate, "Parameter `taskCreate` is required when calling `createTaskV1`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/tasks")
.withBody(taskCreate)
.build()
execute[TaskCreateResponse](request, requestOptions)
}
/** Creates a new transformation.
*
* @param transformationCreate
* Request body for creating a transformation.
*/
def createTransformation(transformationCreate: TransformationCreate, requestOptions: Option[RequestOptions] = None)(
implicit ec: ExecutionContext
): Future[TransformationCreateResponse] = Future {
requireNotNull(
transformationCreate,
"Parameter `transformationCreate` is required when calling `createTransformation`."
)
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/transformations")
.withBody(transformationCreate)
.build()
execute[TransformationCreateResponse](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
*/
def customDelete[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customDelete`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/${path}")
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
*/
def customGet[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customGet`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/${path}")
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
* @param body
* Parameters to send with the custom request.
*/
def customPost[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
body: Option[Any] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customPost`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/${path}")
.withBody(body)
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
* @param body
* Parameters to send with the custom request.
*/
def customPut[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
body: Option[Any] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customPut`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/${path}")
.withBody(body)
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** Deletes an authentication resource. You can't delete authentication resources that are used by a source or a
* destination.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param authenticationID
* Unique identifier of an authentication resource.
*/
def deleteAuthentication(authenticationID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[DeleteResponse] = Future {
requireNotNull(authenticationID, "Parameter `authenticationID` is required when calling `deleteAuthentication`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/1/authentications/${escape(authenticationID)}")
.build()
execute[DeleteResponse](request, requestOptions)
}
/** Deletes a destination by its ID. You can't delete destinations that are referenced in tasks.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param destinationID
* Unique identifier of a destination.
*/
def deleteDestination(destinationID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[DeleteResponse] = Future {
requireNotNull(destinationID, "Parameter `destinationID` is required when calling `deleteDestination`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/1/destinations/${escape(destinationID)}")
.build()
execute[DeleteResponse](request, requestOptions)
}
/** Deletes a source by its ID. You can't delete sources that are referenced in tasks.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceID
* Unique identifier of a source.
*/
def deleteSource(sourceID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[DeleteResponse] = Future {
requireNotNull(sourceID, "Parameter `sourceID` is required when calling `deleteSource`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/1/sources/${escape(sourceID)}")
.build()
execute[DeleteResponse](request, requestOptions)
}
/** Deletes a task by its ID.
*
* @param taskID
* Unique identifier of a task.
*/
def deleteTask(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[DeleteResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `deleteTask`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/2/tasks/${escape(taskID)}")
.build()
execute[DeleteResponse](request, requestOptions)
}
/** Deletes a task by its ID using the v1 endpoint, please use `deleteTask` instead.
*
* @param taskID
* Unique identifier of a task.
*/
def deleteTaskV1(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[DeleteResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `deleteTaskV1`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/1/tasks/${escape(taskID)}")
.build()
execute[DeleteResponse](request, requestOptions)
}
/** Deletes a transformation by its ID.
*
* @param transformationID
* Unique identifier of a transformation.
*/
def deleteTransformation(transformationID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[DeleteResponse] = Future {
requireNotNull(transformationID, "Parameter `transformationID` is required when calling `deleteTransformation`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/1/transformations/${escape(transformationID)}")
.build()
execute[DeleteResponse](request, requestOptions)
}
/** Disables a task.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def disableTask(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskUpdateResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `disableTask`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/2/tasks/${escape(taskID)}/disable")
.build()
execute[TaskUpdateResponse](request, requestOptions)
}
/** Disables a task using the v1 endpoint, please use `disableTask` instead.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def disableTaskV1(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskUpdateResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `disableTaskV1`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/1/tasks/${escape(taskID)}/disable")
.build()
execute[TaskUpdateResponse](request, requestOptions)
}
/** Enables a task.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def enableTask(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskUpdateResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `enableTask`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/2/tasks/${escape(taskID)}/enable")
.build()
execute[TaskUpdateResponse](request, requestOptions)
}
/** Enables a task using the v1 endpoint, please use `enableTask` instead.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def enableTaskV1(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskUpdateResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `enableTaskV1`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/1/tasks/${escape(taskID)}/enable")
.build()
execute[TaskUpdateResponse](request, requestOptions)
}
/** Generates code for the selected model based on the given prompt.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def generateTransformationCode(
generateTransformationCodePayload: GenerateTransformationCodePayload,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GenerateTransformationCodeResponse] = Future {
requireNotNull(
generateTransformationCodePayload,
"Parameter `generateTransformationCodePayload` is required when calling `generateTransformationCode`."
)
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/transformations/models")
.withBody(generateTransformationCodePayload)
.build()
execute[GenerateTransformationCodeResponse](request, requestOptions)
}
/** Retrieves an authentication resource by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param authenticationID
* Unique identifier of an authentication resource.
*/
def getAuthentication(authenticationID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Authentication] = Future {
requireNotNull(authenticationID, "Parameter `authenticationID` is required when calling `getAuthentication`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/authentications/${escape(authenticationID)}")
.build()
execute[Authentication](request, requestOptions)
}
/** Retrieves a destination by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param destinationID
* Unique identifier of a destination.
*/
def getDestination(destinationID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Destination] = Future {
requireNotNull(destinationID, "Parameter `destinationID` is required when calling `getDestination`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/destinations/${escape(destinationID)}")
.build()
execute[Destination](request, requestOptions)
}
/** Retrieves a single task run event by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param runID
* Unique identifier of a task run.
* @param eventID
* Unique identifier of an event.
*/
def getEvent(runID: String, eventID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Event] = Future {
requireNotNull(runID, "Parameter `runID` is required when calling `getEvent`.")
requireNotNull(eventID, "Parameter `eventID` is required when calling `getEvent`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/runs/${escape(runID)}/events/${escape(eventID)}")
.build()
execute[Event](request, requestOptions)
}
/** Retrieve a single task run by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param runID
* Unique identifier of a task run.
*/
def getRun(runID: String, requestOptions: Option[RequestOptions] = None)(implicit ec: ExecutionContext): Future[Run] =
Future {
requireNotNull(runID, "Parameter `runID` is required when calling `getRun`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/runs/${escape(runID)}")
.build()
execute[Run](request, requestOptions)
}
/** Retrieve a source by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceID
* Unique identifier of a source.
*/
def getSource(sourceID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Source] = Future {
requireNotNull(sourceID, "Parameter `sourceID` is required when calling `getSource`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/sources/${escape(sourceID)}")
.build()
execute[Source](request, requestOptions)
}
/** Retrieves a task by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def getTask(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Task] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `getTask`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/tasks/${escape(taskID)}")
.build()
execute[Task](request, requestOptions)
}
/** Retrieves a task by its ID using the v1 endpoint, please use `getTask` instead.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def getTaskV1(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskV1] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `getTaskV1`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/tasks/${escape(taskID)}")
.build()
execute[TaskV1](request, requestOptions)
}
/** Retrieves a transformation by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param transformationID
* Unique identifier of a transformation.
*/
def getTransformation(transformationID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Transformation] = Future {
requireNotNull(transformationID, "Parameter `transformationID` is required when calling `getTransformation`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/transformations/${escape(transformationID)}")
.build()
execute[Transformation](request, requestOptions)
}
/** Retrieves a list of all authentication resources.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param `type`
* Type of authentication resource to retrieve.
* @param platform
* Ecommerce platform for which to retrieve authentication resources.
* @param sort
* Property by which to sort the list of authentication resources.
* @param order
* Sort order of the response, ascending or descending.
*/
def listAuthentications(
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
`type`: Option[Seq[AuthenticationType]] = None,
platform: Option[Seq[PlatformWithNone]] = None,
sort: Option[AuthenticationSortKeys] = None,
order: Option[OrderKeys] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[ListAuthenticationsResponse] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/authentications")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("type", `type`)
.withQueryParameter("platform", platform)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.build()
execute[ListAuthenticationsResponse](request, requestOptions)
}
/** Retrieves a list of destinations.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param `type`
* Destination type.
* @param authenticationID
* Authentication ID used by destinations.
* @param sort
* Property by which to sort the destinations.
* @param order
* Sort order of the response, ascending or descending.
*/
def listDestinations(
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
`type`: Option[Seq[DestinationType]] = None,
authenticationID: Option[Seq[String]] = None,
sort: Option[DestinationSortKeys] = None,
order: Option[OrderKeys] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[ListDestinationsResponse] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/destinations")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("type", `type`)
.withQueryParameter("authenticationID", authenticationID)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.build()
execute[ListDestinationsResponse](request, requestOptions)
}
/** Retrieves a list of events for a task run, identified by it's ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param runID
* Unique identifier of a task run.
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param status
* Event status for filtering the list of task runs.
* @param `type`
* Event type for filtering the list of task runs.
* @param sort
* Property by which to sort the list of task run events.
* @param order
* Sort order of the response, ascending or descending.
* @param startDate
* Date and time in RFC 3339 format for the earliest events to retrieve. By default, the current time minus three
* hours is used.
* @param endDate
* Date and time in RFC 3339 format for the latest events to retrieve. By default, the current time is used.
*/
def listEvents(
runID: String,
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
status: Option[Seq[EventStatus]] = None,
`type`: Option[Seq[EventType]] = None,
sort: Option[EventSortKeys] = None,
order: Option[OrderKeys] = None,
startDate: Option[String] = None,
endDate: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[ListEventsResponse] = Future {
requireNotNull(runID, "Parameter `runID` is required when calling `listEvents`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/runs/${escape(runID)}/events")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("status", status)
.withQueryParameter("type", `type`)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.build()
execute[ListEventsResponse](request, requestOptions)
}
/** Retrieve a list of task runs.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param status
* Run status for filtering the list of task runs.
* @param `type`
* Run type for filtering the list of task runs.
* @param taskID
* Task ID for filtering the list of task runs.
* @param sort
* Property by which to sort the list of task runs.
* @param order
* Sort order of the response, ascending or descending.
* @param startDate
* Date in RFC 3339 format for the earliest run to retrieve. By default, the current day minus seven days is used.
* @param endDate
* Date in RFC 3339 format for the latest run to retrieve. By default, the current day is used.
*/
def listRuns(
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
status: Option[Seq[RunStatus]] = None,
`type`: Option[Seq[RunType]] = None,
taskID: Option[String] = None,
sort: Option[RunSortKeys] = None,
order: Option[OrderKeys] = None,
startDate: Option[String] = None,
endDate: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[RunListResponse] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/runs")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("status", status)
.withQueryParameter("type", `type`)
.withQueryParameter("taskID", taskID)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.build()
execute[RunListResponse](request, requestOptions)
}
/** Retrieves a list of sources.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param `type`
* Source type. Some sources require authentication.
* @param authenticationID
* Authentication IDs of the sources to retrieve. 'none' returns sources that doesn't have an authentication
* resource.
* @param sort
* Property by which to sort the list of sources.
* @param order
* Sort order of the response, ascending or descending.
*/
def listSources(
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
`type`: Option[Seq[SourceType]] = None,
authenticationID: Option[Seq[String]] = None,
sort: Option[SourceSortKeys] = None,
order: Option[OrderKeys] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[ListSourcesResponse] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/sources")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("type", `type`)
.withQueryParameter("authenticationID", authenticationID)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.build()
execute[ListSourcesResponse](request, requestOptions)
}
/** Retrieves a list of tasks.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param action
* Actions for filtering the list of tasks.
* @param enabled
* Whether to filter the list of tasks by the `enabled` status.
* @param sourceID
* Source IDs for filtering the list of tasks.
* @param destinationID
* Destination IDs for filtering the list of tasks.
* @param triggerType
* Type of task trigger for filtering the list of tasks.
* @param sort
* Property by which to sort the list of tasks.
* @param order
* Sort order of the response, ascending or descending.
*/
def listTasks(
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
action: Option[Seq[ActionType]] = None,
enabled: Option[Boolean] = None,
sourceID: Option[Seq[String]] = None,
destinationID: Option[Seq[String]] = None,
triggerType: Option[Seq[TriggerType]] = None,
sort: Option[TaskSortKeys] = None,
order: Option[OrderKeys] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[ListTasksResponse] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/tasks")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("action", action)
.withQueryParameter("enabled", enabled)
.withQueryParameter("sourceID", sourceID)
.withQueryParameter("destinationID", destinationID)
.withQueryParameter("triggerType", triggerType)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.build()
execute[ListTasksResponse](request, requestOptions)
}
/** Retrieves a list of tasks using the v1 endpoint, please use `getTasks` instead.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param action
* Actions for filtering the list of tasks.
* @param enabled
* Whether to filter the list of tasks by the `enabled` status.
* @param sourceID
* Source IDs for filtering the list of tasks.
* @param destinationID
* Destination IDs for filtering the list of tasks.
* @param triggerType
* Type of task trigger for filtering the list of tasks.
* @param sort
* Property by which to sort the list of tasks.
* @param order
* Sort order of the response, ascending or descending.
*/
def listTasksV1(
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
action: Option[Seq[ActionType]] = None,
enabled: Option[Boolean] = None,
sourceID: Option[Seq[String]] = None,
destinationID: Option[Seq[String]] = None,
triggerType: Option[Seq[TriggerType]] = None,
sort: Option[TaskSortKeys] = None,
order: Option[OrderKeys] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[ListTasksResponseV1] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/tasks")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("action", action)
.withQueryParameter("enabled", enabled)
.withQueryParameter("sourceID", sourceID)
.withQueryParameter("destinationID", destinationID)
.withQueryParameter("triggerType", triggerType)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.build()
execute[ListTasksResponseV1](request, requestOptions)
}
/** Retrieves a list of existing LLM transformation helpers.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def listTransformationModels(
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[TransformationModels] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/transformations/models")
.build()
execute[TransformationModels](request, requestOptions)
}
/** Retrieves a list of transformations.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param itemsPerPage
* Number of items per page.
* @param page
* Page number of the paginated API response.
* @param sort
* Property by which to sort the list.
* @param order
* Sort order of the response, ascending or descending.
*/
def listTransformations(
itemsPerPage: Option[Int] = None,
page: Option[Int] = None,
sort: Option[SortKeys] = None,
order: Option[OrderKeys] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[ListTransformationsResponse] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/transformations")
.withQueryParameter("itemsPerPage", itemsPerPage)
.withQueryParameter("page", page)
.withQueryParameter("sort", sort)
.withQueryParameter("order", order)
.build()
execute[ListTransformationsResponse](request, requestOptions)
}
/** Push a `batch` request payload through the Pipeline. You can check the status of task pushes with the
* observability endpoints.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
* @param pushTaskPayload
* Request body of a Search API `batch` request that will be pushed in the Connectors pipeline.
*/
def pushTask(taskID: String, pushTaskPayload: PushTaskPayload, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[RunResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `pushTask`.")
requireNotNull(pushTaskPayload, "Parameter `pushTaskPayload` is required when calling `pushTask`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/2/tasks/${escape(taskID)}/push")
.withBody(pushTaskPayload)
.build()
execute[RunResponse](request, requestOptions)
}
/** Runs all tasks linked to a source, only available for Shopify sources. It will create 1 run per task.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceID
* Unique identifier of a source.
* @param runSourcePayload
*/
def runSource(
sourceID: String,
runSourcePayload: Option[RunSourcePayload] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[RunSourceResponse] = Future {
requireNotNull(sourceID, "Parameter `sourceID` is required when calling `runSource`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/sources/${escape(sourceID)}/run")
.withBody(runSourcePayload)
.build()
execute[RunSourceResponse](request, requestOptions)
}
/** Runs a task. You can check the status of task runs with the observability endpoints.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def runTask(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[RunResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `runTask`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/2/tasks/${escape(taskID)}/run")
.build()
execute[RunResponse](request, requestOptions)
}
/** Runs a task using the v1 endpoint, please use `runTask` instead. You can check the status of task runs with the
* observability endpoints.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param taskID
* Unique identifier of a task.
*/
def runTaskV1(taskID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[RunResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `runTaskV1`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/tasks/${escape(taskID)}/run")
.build()
execute[RunResponse](request, requestOptions)
}
/** Searches for authentication resources.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def searchAuthentications(authenticationSearch: AuthenticationSearch, requestOptions: Option[RequestOptions] = None)(
implicit ec: ExecutionContext
): Future[Seq[Authentication]] = Future {
requireNotNull(
authenticationSearch,
"Parameter `authenticationSearch` is required when calling `searchAuthentications`."
)
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/authentications/search")
.withBody(authenticationSearch)
.build()
execute[Seq[Authentication]](request, requestOptions)
}
/** Searches for destinations.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def searchDestinations(destinationSearch: DestinationSearch, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Seq[Destination]] = Future {
requireNotNull(destinationSearch, "Parameter `destinationSearch` is required when calling `searchDestinations`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/destinations/search")
.withBody(destinationSearch)
.build()
execute[Seq[Destination]](request, requestOptions)
}
/** Searches for sources.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def searchSources(sourceSearch: SourceSearch, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Seq[Source]] = Future {
requireNotNull(sourceSearch, "Parameter `sourceSearch` is required when calling `searchSources`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/sources/search")
.withBody(sourceSearch)
.build()
execute[Seq[Source]](request, requestOptions)
}
/** Searches for tasks.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def searchTasks(taskSearch: TaskSearch, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Seq[Task]] = Future {
requireNotNull(taskSearch, "Parameter `taskSearch` is required when calling `searchTasks`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/2/tasks/search")
.withBody(taskSearch)
.build()
execute[Seq[Task]](request, requestOptions)
}
/** Searches for tasks using the v1 endpoint, please use `searchTasks` instead.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def searchTasksV1(taskSearch: TaskSearch, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[Seq[TaskV1]] = Future {
requireNotNull(taskSearch, "Parameter `taskSearch` is required when calling `searchTasksV1`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/tasks/search")
.withBody(taskSearch)
.build()
execute[Seq[TaskV1]](request, requestOptions)
}
/** Searches for transformations.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def searchTransformations(transformationSearch: TransformationSearch, requestOptions: Option[RequestOptions] = None)(
implicit ec: ExecutionContext
): Future[Seq[Transformation]] = Future {
requireNotNull(
transformationSearch,
"Parameter `transformationSearch` is required when calling `searchTransformations`."
)
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/transformations/search")
.withBody(transformationSearch)
.build()
execute[Seq[Transformation]](request, requestOptions)
}
/** Triggers a stream-listing request for a source. Triggering stream-listing requests only works with sources with
* `type: docker` and `imageType: singer`.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceID
* Unique identifier of a source.
*/
def triggerDockerSourceDiscover(sourceID: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[SourceWatchResponse] = Future {
requireNotNull(sourceID, "Parameter `sourceID` is required when calling `triggerDockerSourceDiscover`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/sources/${escape(sourceID)}/discover")
.build()
execute[SourceWatchResponse](request, requestOptions)
}
/** Try a transformation before creating it.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*/
def tryTransformation(transformationTry: TransformationTry, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TransformationTryResponse] = Future {
requireNotNull(transformationTry, "Parameter `transformationTry` is required when calling `tryTransformation`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/transformations/try")
.withBody(transformationTry)
.build()
execute[TransformationTryResponse](request, requestOptions)
}
/** Try a transformation before updating it.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param transformationID
* Unique identifier of a transformation.
*/
def tryTransformationBeforeUpdate(
transformationID: String,
transformationTry: TransformationTry,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[TransformationTryResponse] = Future {
requireNotNull(
transformationID,
"Parameter `transformationID` is required when calling `tryTransformationBeforeUpdate`."
)
requireNotNull(
transformationTry,
"Parameter `transformationTry` is required when calling `tryTransformationBeforeUpdate`."
)
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/transformations/${escape(transformationID)}/try")
.withBody(transformationTry)
.build()
execute[TransformationTryResponse](request, requestOptions)
}
/** Updates an authentication resource.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param authenticationID
* Unique identifier of an authentication resource.
*/
def updateAuthentication(
authenticationID: String,
authenticationUpdate: AuthenticationUpdate,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[AuthenticationUpdateResponse] = Future {
requireNotNull(authenticationID, "Parameter `authenticationID` is required when calling `updateAuthentication`.")
requireNotNull(
authenticationUpdate,
"Parameter `authenticationUpdate` is required when calling `updateAuthentication`."
)
val request = HttpRequest
.builder()
.withMethod("PATCH")
.withPath(s"/1/authentications/${escape(authenticationID)}")
.withBody(authenticationUpdate)
.build()
execute[AuthenticationUpdateResponse](request, requestOptions)
}
/** Updates the destination by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param destinationID
* Unique identifier of a destination.
*/
def updateDestination(
destinationID: String,
destinationUpdate: DestinationUpdate,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[DestinationUpdateResponse] = Future {
requireNotNull(destinationID, "Parameter `destinationID` is required when calling `updateDestination`.")
requireNotNull(destinationUpdate, "Parameter `destinationUpdate` is required when calling `updateDestination`.")
val request = HttpRequest
.builder()
.withMethod("PATCH")
.withPath(s"/1/destinations/${escape(destinationID)}")
.withBody(destinationUpdate)
.build()
execute[DestinationUpdateResponse](request, requestOptions)
}
/** Updates a source by its ID.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceID
* Unique identifier of a source.
*/
def updateSource(sourceID: String, sourceUpdate: SourceUpdate, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[SourceUpdateResponse] = Future {
requireNotNull(sourceID, "Parameter `sourceID` is required when calling `updateSource`.")
requireNotNull(sourceUpdate, "Parameter `sourceUpdate` is required when calling `updateSource`.")
val request = HttpRequest
.builder()
.withMethod("PATCH")
.withPath(s"/1/sources/${escape(sourceID)}")
.withBody(sourceUpdate)
.build()
execute[SourceUpdateResponse](request, requestOptions)
}
/** Updates a task by its ID.
*
* @param taskID
* Unique identifier of a task.
*/
def updateTask(taskID: String, taskUpdate: TaskUpdate, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskUpdateResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `updateTask`.")
requireNotNull(taskUpdate, "Parameter `taskUpdate` is required when calling `updateTask`.")
val request = HttpRequest
.builder()
.withMethod("PATCH")
.withPath(s"/2/tasks/${escape(taskID)}")
.withBody(taskUpdate)
.build()
execute[TaskUpdateResponse](request, requestOptions)
}
/** Updates a task by its ID using the v1 endpoint, please use `updateTask` instead.
*
* @param taskID
* Unique identifier of a task.
*/
def updateTaskV1(taskID: String, taskUpdate: TaskUpdateV1, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[TaskUpdateResponse] = Future {
requireNotNull(taskID, "Parameter `taskID` is required when calling `updateTaskV1`.")
requireNotNull(taskUpdate, "Parameter `taskUpdate` is required when calling `updateTaskV1`.")
val request = HttpRequest
.builder()
.withMethod("PATCH")
.withPath(s"/1/tasks/${escape(taskID)}")
.withBody(taskUpdate)
.build()
execute[TaskUpdateResponse](request, requestOptions)
}
/** Updates a transformation by its ID.
*
* @param transformationID
* Unique identifier of a transformation.
*/
def updateTransformation(
transformationID: String,
transformationCreate: TransformationCreate,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[TransformationUpdateResponse] = Future {
requireNotNull(transformationID, "Parameter `transformationID` is required when calling `updateTransformation`.")
requireNotNull(
transformationCreate,
"Parameter `transformationCreate` is required when calling `updateTransformation`."
)
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/1/transformations/${escape(transformationID)}")
.withBody(transformationCreate)
.build()
execute[TransformationUpdateResponse](request, requestOptions)
}
/** Validates a source payload to ensure it can be created and that the data source can be reached by Algolia.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceCreate
*/
def validateSource(sourceCreate: Option[SourceCreate] = None, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[SourceWatchResponse] = Future {
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/sources/validate")
.withBody(sourceCreate)
.build()
execute[SourceWatchResponse](request, requestOptions)
}
/** Validates an update of a source payload to ensure it can be created and that the data source can be reached by
* Algolia.
*
* Required API Key ACLs:
* - addObject
* - deleteIndex
* - editSettings
*
* @param sourceID
* Unique identifier of a source.
*/
def validateSourceBeforeUpdate(
sourceID: String,
sourceUpdate: SourceUpdate,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[SourceWatchResponse] = Future {
requireNotNull(sourceID, "Parameter `sourceID` is required when calling `validateSourceBeforeUpdate`.")
requireNotNull(sourceUpdate, "Parameter `sourceUpdate` is required when calling `validateSourceBeforeUpdate`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/sources/${escape(sourceID)}/validate")
.withBody(sourceUpdate)
.build()
execute[SourceWatchResponse](request, requestOptions)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy