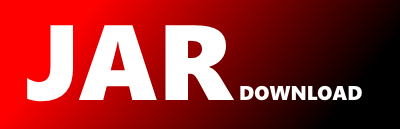
algoliasearch.api.QuerySuggestionsClient.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-scala_3 Show documentation
Show all versions of algoliasearch-scala_3 Show documentation
Scala client for Algolia Search API
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on
* https://github.com/algolia/api-clients-automation. DO NOT EDIT.
*/
package algoliasearch.api
import algoliasearch.querysuggestions.BaseResponse
import algoliasearch.querysuggestions.ConfigStatus
import algoliasearch.querysuggestions.Configuration
import algoliasearch.querysuggestions.ConfigurationResponse
import algoliasearch.querysuggestions.ConfigurationWithIndex
import algoliasearch.querysuggestions.ErrorBase
import algoliasearch.querysuggestions.LogFile
import algoliasearch.querysuggestions._
import algoliasearch.ApiClient
import algoliasearch.api.QuerySuggestionsClient.hosts
import algoliasearch.config._
import algoliasearch.internal.util._
import scala.concurrent.{ExecutionContext, Future}
import scala.util.Random
object QuerySuggestionsClient {
/** Creates a new SearchApi instance using default hosts.
*
* @param appId
* application ID
* @param apiKey
* api key
* @param region
* region
* @param clientOptions
* client options
*/
def apply(
appId: String,
apiKey: String,
region: String,
clientOptions: ClientOptions = ClientOptions()
) = new QuerySuggestionsClient(
appId = appId,
apiKey = apiKey,
region = region,
clientOptions = clientOptions
)
private def hosts(region: String): Seq[Host] = {
val allowedRegions = Seq("eu", "us")
if (!allowedRegions.contains(region)) {
throw new IllegalArgumentException(
s"`region` is required and must be one of the following: ${allowedRegions.mkString(", ")}"
)
}
val url = "query-suggestions.{region}.algolia.com".replace("{region}", region)
Seq(Host(url = url, callTypes = Set(CallType.Read, CallType.Write)))
}
}
class QuerySuggestionsClient(
appId: String,
apiKey: String,
region: String,
clientOptions: ClientOptions = ClientOptions()
) extends ApiClient(
appId = appId,
apiKey = apiKey,
clientName = "QuerySuggestions",
defaultHosts = hosts(region),
formats = JsonSupport.format,
options = clientOptions
) {
/** Creates a new Query Suggestions configuration. You can have up to 100 configurations per Algolia application.
*
* Required API Key ACLs:
* - editSettings
*/
def createConfig(configurationWithIndex: ConfigurationWithIndex, requestOptions: Option[RequestOptions] = None)(
implicit ec: ExecutionContext
): Future[BaseResponse] = Future {
requireNotNull(
configurationWithIndex,
"Parameter `configurationWithIndex` is required when calling `createConfig`."
)
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/1/configs")
.withBody(configurationWithIndex)
.build()
execute[BaseResponse](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
*/
def customDelete[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customDelete`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/${path}")
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
*/
def customGet[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customGet`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/${path}")
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
* @param body
* Parameters to send with the custom request.
*/
def customPost[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
body: Option[Any] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customPost`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/${path}")
.withBody(body)
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
* @param body
* Parameters to send with the custom request.
*/
def customPut[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
body: Option[Any] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customPut`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/${path}")
.withBody(body)
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** Deletes a Query Suggestions configuration. Deleting only removes the configuration and stops updates to the Query
* Suggestions index. To delete the Query Suggestions index itself, use the Search API and the [Delete an
* index](/specs/search#tag/Indices/operation/deleteIndex) operation.
*
* Required API Key ACLs:
* - editSettings
*
* @param indexName
* Query Suggestions index name.
*/
def deleteConfig(indexName: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[BaseResponse] = Future {
requireNotNull(indexName, "Parameter `indexName` is required when calling `deleteConfig`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/1/configs/${escape(indexName)}")
.build()
execute[BaseResponse](request, requestOptions)
}
/** Retrieves all Query Suggestions configurations of your Algolia application.
*
* Required API Key ACLs:
* - settings
*/
def getAllConfigs(
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[Seq[ConfigurationResponse]] = Future {
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/configs")
.build()
execute[Seq[ConfigurationResponse]](request, requestOptions)
}
/** Retrieves a single Query Suggestions configuration by its index name.
*
* Required API Key ACLs:
* - settings
*
* @param indexName
* Query Suggestions index name.
*/
def getConfig(indexName: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[ConfigurationResponse] = Future {
requireNotNull(indexName, "Parameter `indexName` is required when calling `getConfig`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/configs/${escape(indexName)}")
.build()
execute[ConfigurationResponse](request, requestOptions)
}
/** Reports the status of a Query Suggestions index.
*
* Required API Key ACLs:
* - settings
*
* @param indexName
* Query Suggestions index name.
*/
def getConfigStatus(indexName: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[ConfigStatus] = Future {
requireNotNull(indexName, "Parameter `indexName` is required when calling `getConfigStatus`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/configs/${escape(indexName)}/status")
.build()
execute[ConfigStatus](request, requestOptions)
}
/** Retrieves the logs for a single Query Suggestions index.
*
* Required API Key ACLs:
* - settings
*
* @param indexName
* Query Suggestions index name.
*/
def getLogFile(indexName: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[LogFile] = Future {
requireNotNull(indexName, "Parameter `indexName` is required when calling `getLogFile`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/1/logs/${escape(indexName)}")
.build()
execute[LogFile](request, requestOptions)
}
/** Updates a QuerySuggestions configuration.
*
* Required API Key ACLs:
* - editSettings
*
* @param indexName
* Query Suggestions index name.
*/
def updateConfig(indexName: String, configuration: Configuration, requestOptions: Option[RequestOptions] = None)(
implicit ec: ExecutionContext
): Future[BaseResponse] = Future {
requireNotNull(indexName, "Parameter `indexName` is required when calling `updateConfig`.")
requireNotNull(configuration, "Parameter `configuration` is required when calling `updateConfig`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/1/configs/${escape(indexName)}")
.withBody(configuration)
.build()
execute[BaseResponse](request, requestOptions)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy