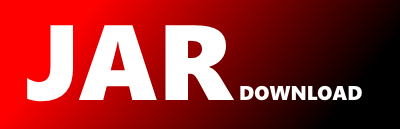
algoliasearch.api.AnalyticsClient.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch-scala_3 Show documentation
Show all versions of algoliasearch-scala_3 Show documentation
Scala client for Algolia Search API
/** Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost - read more on
* https://github.com/algolia/api-clients-automation. DO NOT EDIT.
*/
package algoliasearch.api
import algoliasearch.analytics.Direction._
import algoliasearch.analytics.ErrorBase
import algoliasearch.analytics.GetAddToCartRateResponse
import algoliasearch.analytics.GetAverageClickPositionResponse
import algoliasearch.analytics.GetClickPositionsResponse
import algoliasearch.analytics.GetClickThroughRateResponse
import algoliasearch.analytics.GetConversionRateResponse
import algoliasearch.analytics.GetNoClickRateResponse
import algoliasearch.analytics.GetNoResultsRateResponse
import algoliasearch.analytics.GetPurchaseRateResponse
import algoliasearch.analytics.GetRevenue
import algoliasearch.analytics.GetSearchesCountResponse
import algoliasearch.analytics.GetSearchesNoClicksResponse
import algoliasearch.analytics.GetSearchesNoResultsResponse
import algoliasearch.analytics.GetStatusResponse
import algoliasearch.analytics.GetTopCountriesResponse
import algoliasearch.analytics.GetTopFilterAttributesResponse
import algoliasearch.analytics.GetTopFilterForAttributeResponse
import algoliasearch.analytics.GetTopFiltersNoResultsResponse
import algoliasearch.analytics.GetTopHitsResponse
import algoliasearch.analytics.GetTopSearchesResponse
import algoliasearch.analytics.GetUsersCountResponse
import algoliasearch.analytics.OrderBy._
import algoliasearch.analytics._
import algoliasearch.ApiClient
import algoliasearch.api.AnalyticsClient.hosts
import algoliasearch.config._
import algoliasearch.internal.util._
import scala.concurrent.{ExecutionContext, Future}
import scala.util.Random
object AnalyticsClient {
/** Creates a new SearchApi instance using default hosts.
*
* @param appId
* application ID
* @param apiKey
* api key
* @param region
* region
* @param clientOptions
* client options
*/
def apply(
appId: String,
apiKey: String,
region: Option[String] = None,
clientOptions: ClientOptions = ClientOptions()
) = new AnalyticsClient(
appId = appId,
apiKey = apiKey,
region = region,
clientOptions = clientOptions
)
private def hosts(region: Option[String] = None): Seq[Host] = {
val allowedRegions = Seq("de", "us")
if (region.isDefined && !allowedRegions.contains(region.get)) {
throw new IllegalArgumentException(s"`region` must be one of the following: ${allowedRegions.mkString(", ")}")
}
val url =
if (region.isEmpty) "analytics.algolia.com" else "analytics.{region}.algolia.com".replace("{region}", region.get)
Seq(Host(url = url, callTypes = Set(CallType.Read, CallType.Write)))
}
}
class AnalyticsClient(
appId: String,
apiKey: String,
region: Option[String] = None,
clientOptions: ClientOptions = ClientOptions()
) extends ApiClient(
appId = appId,
apiKey = apiKey,
clientName = "Analytics",
defaultHosts = hosts(region),
formats = JsonSupport.format,
options = clientOptions
) {
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
*/
def customDelete[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customDelete`.")
val request = HttpRequest
.builder()
.withMethod("DELETE")
.withPath(s"/${path}")
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
*/
def customGet[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customGet`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/${path}")
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
* @param body
* Parameters to send with the custom request.
*/
def customPost[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
body: Option[Any] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customPost`.")
val request = HttpRequest
.builder()
.withMethod("POST")
.withPath(s"/${path}")
.withBody(body)
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** This method allow you to send requests to the Algolia REST API.
*
* @param path
* Path of the endpoint, anything after \"/1\" must be specified.
* @param parameters
* Query parameters to apply to the current query.
* @param body
* Parameters to send with the custom request.
*/
def customPut[T: Manifest](
path: String,
parameters: Option[Map[String, Any]] = None,
body: Option[Any] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[T] = Future {
requireNotNull(path, "Parameter `path` is required when calling `customPut`.")
val request = HttpRequest
.builder()
.withMethod("PUT")
.withPath(s"/${path}")
.withBody(body)
.withQueryParameters(parameters)
.build()
execute[T](request, requestOptions)
}
/** Retrieves the add-to-cart rate for all of your searches with at least one add-to-cart event, including a daily
* breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getAddToCartRate(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetAddToCartRateResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getAddToCartRate`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/conversions/addToCartRate")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetAddToCartRateResponse](request, requestOptions)
}
/** Retrieves the average click position of your search results, including a daily breakdown. The average click
* position is the average of all clicked search results' positions. For example, if users only ever click on the
* first result for any search, the average click position is 1. By default, the analyzed period includes the last
* eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getAverageClickPosition(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetAverageClickPositionResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getAverageClickPosition`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/clicks/averageClickPosition")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetAverageClickPositionResponse](request, requestOptions)
}
/** Retrieves the positions in the search results and their associated number of clicks. This lets you check how many
* clicks the first, second, or tenth search results receive.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getClickPositions(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetClickPositionsResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getClickPositions`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/clicks/positions")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetClickPositionsResponse](request, requestOptions)
}
/** Retrieves the click-through rate for all of your searches with at least one click event, including a daily
* breakdown By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getClickThroughRate(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetClickThroughRateResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getClickThroughRate`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/clicks/clickThroughRate")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetClickThroughRateResponse](request, requestOptions)
}
/** Retrieves the conversion rate for all of your searches with at least one conversion event, including a daily
* breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getConversionRate(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetConversionRateResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getConversionRate`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/conversions/conversionRate")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetConversionRateResponse](request, requestOptions)
}
/** Retrieves the fraction of searches that didn't lead to any click within a time range, including a daily breakdown.
* By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getNoClickRate(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetNoClickRateResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getNoClickRate`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/searches/noClickRate")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetNoClickRateResponse](request, requestOptions)
}
/** Retrieves the fraction of searches that didn't return any results within a time range, including a daily
* breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getNoResultsRate(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetNoResultsRateResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getNoResultsRate`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/searches/noResultRate")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetNoResultsRateResponse](request, requestOptions)
}
/** Retrieves the purchase rate for all of your searches with at least one purchase event, including a daily
* breakdown. By default, the analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getPurchaseRate(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetPurchaseRateResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getPurchaseRate`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/conversions/purchaseRate")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetPurchaseRateResponse](request, requestOptions)
}
/** Retrieves revenue-related metrics, such as the total revenue or the average order value. To retrieve
* revenue-related metrics, sent purchase events. By default, the analyzed period includes the last eight days
* including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getRevenue(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetRevenue] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getRevenue`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/conversions/revenue")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetRevenue](request, requestOptions)
}
/** Retrieves the number of searches within a time range, including a daily breakdown. By default, the analyzed period
* includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getSearchesCount(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetSearchesCountResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getSearchesCount`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/searches/count")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetSearchesCountResponse](request, requestOptions)
}
/** Retrieves the most popular searches that didn't lead to any clicks, from the 1,000 most frequent searches.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getSearchesNoClicks(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetSearchesNoClicksResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getSearchesNoClicks`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/searches/noClicks")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetSearchesNoClicksResponse](request, requestOptions)
}
/** Retrieves the most popular searches that didn't return any results.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getSearchesNoResults(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetSearchesNoResultsResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getSearchesNoResults`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/searches/noResults")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetSearchesNoResultsResponse](request, requestOptions)
}
/** Retrieves the time when the Analytics data for the specified index was last updated. The Analytics data is updated
* every 5 minutes.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
*/
def getStatus(index: String, requestOptions: Option[RequestOptions] = None)(implicit
ec: ExecutionContext
): Future[GetStatusResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getStatus`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/status")
.withQueryParameter("index", index)
.build()
execute[GetStatusResponse](request, requestOptions)
}
/** Retrieves the countries with the most searches to your index.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getTopCountries(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetTopCountriesResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getTopCountries`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/countries")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetTopCountriesResponse](request, requestOptions)
}
/** Retrieves the most frequently used filter attributes. These are attributes of your records that you included in
* the `attributesForFaceting` setting.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param search
* Search query.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getTopFilterAttributes(
index: String,
search: Option[String] = None,
startDate: Option[String] = None,
endDate: Option[String] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetTopFilterAttributesResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getTopFilterAttributes`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/filters")
.withQueryParameter("index", index)
.withQueryParameter("search", search)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetTopFilterAttributesResponse](request, requestOptions)
}
/** Retrieves the most frequent filter (facet) values for a filter attribute. These are attributes of your records
* that you included in the `attributesForFaceting` setting.
*
* Required API Key ACLs:
* - analytics
*
* @param attribute
* Attribute name.
* @param index
* Index name.
* @param search
* Search query.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getTopFilterForAttribute(
attribute: String,
index: String,
search: Option[String] = None,
startDate: Option[String] = None,
endDate: Option[String] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetTopFilterForAttributeResponse] = Future {
requireNotNull(attribute, "Parameter `attribute` is required when calling `getTopFilterForAttribute`.")
requireNotNull(index, "Parameter `index` is required when calling `getTopFilterForAttribute`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/filters/${escape(attribute)}")
.withQueryParameter("index", index)
.withQueryParameter("search", search)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetTopFilterForAttributeResponse](request, requestOptions)
}
/** Retrieves the most frequently used filters for a search that didn't return any results. To get the most frequent
* searches without results, use the [Retrieve searches without results](#tag/search/operation/getSearchesNoResults)
* operation.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param search
* Search query.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getTopFiltersNoResults(
index: String,
search: Option[String] = None,
startDate: Option[String] = None,
endDate: Option[String] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetTopFiltersNoResultsResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getTopFiltersNoResults`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/filters/noResults")
.withQueryParameter("index", index)
.withQueryParameter("search", search)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetTopFiltersNoResultsResponse](request, requestOptions)
}
/** Retrieves the object IDs of the most frequent search results.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param search
* Search query.
* @param clickAnalytics
* Whether to include metrics related to click and conversion events in the response.
* @param revenueAnalytics
* Whether to include revenue-related metrics in the response. If true, metrics related to click and conversion
* events are also included in the response.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getTopHits(
index: String,
search: Option[String] = None,
clickAnalytics: Option[Boolean] = None,
revenueAnalytics: Option[Boolean] = None,
startDate: Option[String] = None,
endDate: Option[String] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetTopHitsResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getTopHits`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/hits")
.withQueryParameter("index", index)
.withQueryParameter("search", search)
.withQueryParameter("clickAnalytics", clickAnalytics)
.withQueryParameter("revenueAnalytics", revenueAnalytics)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetTopHitsResponse](request, requestOptions)
}
/** Returns the most popular search terms.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param clickAnalytics
* Whether to include metrics related to click and conversion events in the response.
* @param revenueAnalytics
* Whether to include revenue-related metrics in the response. If true, metrics related to click and conversion
* events are also included in the response.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param orderBy
* Attribute by which to order the response items. If the `clickAnalytics` parameter is false, only `searchCount`
* is available.
* @param direction
* Sorting direction of the results: ascending or descending.
* @param limit
* Number of items to return.
* @param offset
* Position of the first item to return.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getTopSearches(
index: String,
clickAnalytics: Option[Boolean] = None,
revenueAnalytics: Option[Boolean] = None,
startDate: Option[String] = None,
endDate: Option[String] = None,
orderBy: Option[OrderBy] = None,
direction: Option[Direction] = None,
limit: Option[Int] = None,
offset: Option[Int] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetTopSearchesResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getTopSearches`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/searches")
.withQueryParameter("index", index)
.withQueryParameter("clickAnalytics", clickAnalytics)
.withQueryParameter("revenueAnalytics", revenueAnalytics)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("orderBy", orderBy)
.withQueryParameter("direction", direction)
.withQueryParameter("limit", limit)
.withQueryParameter("offset", offset)
.withQueryParameter("tags", tags)
.build()
execute[GetTopSearchesResponse](request, requestOptions)
}
/** Retrieves the number of unique users within a time range, including a daily breakdown. Since this endpoint returns
* the number of unique users, the sum of the daily values might be different from the total number. By default,
* Algolia distinguishes search users by their IP address, _unless_ you include a pseudonymous user identifier in
* your search requests with the `userToken` API parameter or `x-algolia-usertoken` request header. By default, the
* analyzed period includes the last eight days including the current day.
*
* Required API Key ACLs:
* - analytics
*
* @param index
* Index name.
* @param startDate
* Start date of the period to analyze, in `YYYY-MM-DD` format.
* @param endDate
* End date of the period to analyze, in `YYYY-MM-DD` format.
* @param tags
* Tags by which to segment the analytics. You can combine multiple tags with `OR` and `AND`. Tags must be
* URL-encoded. For more information, see [Segment your analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
def getUsersCount(
index: String,
startDate: Option[String] = None,
endDate: Option[String] = None,
tags: Option[String] = None,
requestOptions: Option[RequestOptions] = None
)(implicit ec: ExecutionContext): Future[GetUsersCountResponse] = Future {
requireNotNull(index, "Parameter `index` is required when calling `getUsersCount`.")
val request = HttpRequest
.builder()
.withMethod("GET")
.withPath(s"/2/users/count")
.withQueryParameter("index", index)
.withQueryParameter("startDate", startDate)
.withQueryParameter("endDate", endDate)
.withQueryParameter("tags", tags)
.build()
execute[GetUsersCountResponse](request, requestOptions)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy