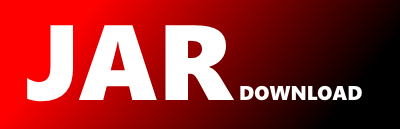
com.algolia.model.analytics.ConversionRateEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.analytics;
import com.fasterxml.jackson.annotation.*;
import java.util.Objects;
/** ConversionRateEvent */
public class ConversionRateEvent {
@JsonProperty("rate")
private Double rate;
@JsonProperty("trackedSearchCount")
private Integer trackedSearchCount;
@JsonProperty("conversionCount")
private Integer conversionCount;
@JsonProperty("date")
private String date;
public ConversionRateEvent setRate(Double rate) {
this.rate = rate;
return this;
}
/**
* [Click-through rate
* (CTR)](https://www.algolia.com/doc/guides/search-analytics/concepts/metrics/#click-through-rate).
* minimum: 0 maximum: 1
*/
@javax.annotation.Nonnull
public Double getRate() {
return rate;
}
public ConversionRateEvent setTrackedSearchCount(Integer trackedSearchCount) {
this.trackedSearchCount = trackedSearchCount;
return this;
}
/**
* Number of tracked searches. This is the number of search requests where the `clickAnalytics`
* parameter is `true`.
*/
@javax.annotation.Nonnull
public Integer getTrackedSearchCount() {
return trackedSearchCount;
}
public ConversionRateEvent setConversionCount(Integer conversionCount) {
this.conversionCount = conversionCount;
return this;
}
/** Number of converted clicks. */
@javax.annotation.Nonnull
public Integer getConversionCount() {
return conversionCount;
}
public ConversionRateEvent setDate(String date) {
this.date = date;
return this;
}
/** Date of the event in the format YYYY-MM-DD. */
@javax.annotation.Nonnull
public String getDate() {
return date;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConversionRateEvent conversionRateEvent = (ConversionRateEvent) o;
return (
Objects.equals(this.rate, conversionRateEvent.rate) &&
Objects.equals(this.trackedSearchCount, conversionRateEvent.trackedSearchCount) &&
Objects.equals(this.conversionCount, conversionRateEvent.conversionCount) &&
Objects.equals(this.date, conversionRateEvent.date)
);
}
@Override
public int hashCode() {
return Objects.hash(rate, trackedSearchCount, conversionCount, date);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConversionRateEvent {\n");
sb.append(" rate: ").append(toIndentedString(rate)).append("\n");
sb.append(" trackedSearchCount: ").append(toIndentedString(trackedSearchCount)).append("\n");
sb.append(" conversionCount: ").append(toIndentedString(conversionCount)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy