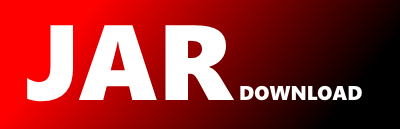
com.algolia.model.querysuggestions.QuerySuggestionsConfigurationWithIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.querysuggestions;
import com.fasterxml.jackson.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** Query Suggestions configuration. */
public class QuerySuggestionsConfigurationWithIndex {
@JsonProperty("indexName")
private String indexName;
@JsonProperty("sourceIndices")
private List sourceIndices = new ArrayList<>();
@JsonProperty("languages")
private Languages languages;
@JsonProperty("exclude")
private List exclude;
@JsonProperty("enablePersonalization")
private Boolean enablePersonalization;
@JsonProperty("allowSpecialCharacters")
private Boolean allowSpecialCharacters;
public QuerySuggestionsConfigurationWithIndex setIndexName(String indexName) {
this.indexName = indexName;
return this;
}
/** Query Suggestions index name. */
@javax.annotation.Nonnull
public String getIndexName() {
return indexName;
}
public QuerySuggestionsConfigurationWithIndex setSourceIndices(List sourceIndices) {
this.sourceIndices = sourceIndices;
return this;
}
public QuerySuggestionsConfigurationWithIndex addSourceIndices(SourceIndex sourceIndicesItem) {
this.sourceIndices.add(sourceIndicesItem);
return this;
}
/** Algolia indices from which to get the popular searches for query suggestions. */
@javax.annotation.Nonnull
public List getSourceIndices() {
return sourceIndices;
}
public QuerySuggestionsConfigurationWithIndex setLanguages(Languages languages) {
this.languages = languages;
return this;
}
/** Get languages */
@javax.annotation.Nullable
public Languages getLanguages() {
return languages;
}
public QuerySuggestionsConfigurationWithIndex setExclude(List exclude) {
this.exclude = exclude;
return this;
}
public QuerySuggestionsConfigurationWithIndex addExclude(String excludeItem) {
if (this.exclude == null) {
this.exclude = new ArrayList<>();
}
this.exclude.add(excludeItem);
return this;
}
/** Patterns to exclude from query suggestions. */
@javax.annotation.Nullable
public List getExclude() {
return exclude;
}
public QuerySuggestionsConfigurationWithIndex setEnablePersonalization(Boolean enablePersonalization) {
this.enablePersonalization = enablePersonalization;
return this;
}
/** Turn on personalized query suggestions. */
@javax.annotation.Nullable
public Boolean getEnablePersonalization() {
return enablePersonalization;
}
public QuerySuggestionsConfigurationWithIndex setAllowSpecialCharacters(Boolean allowSpecialCharacters) {
this.allowSpecialCharacters = allowSpecialCharacters;
return this;
}
/** Allow suggestions with special characters. */
@javax.annotation.Nullable
public Boolean getAllowSpecialCharacters() {
return allowSpecialCharacters;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
QuerySuggestionsConfigurationWithIndex querySuggestionsConfigurationWithIndex = (QuerySuggestionsConfigurationWithIndex) o;
return (
Objects.equals(this.indexName, querySuggestionsConfigurationWithIndex.indexName) &&
Objects.equals(this.sourceIndices, querySuggestionsConfigurationWithIndex.sourceIndices) &&
Objects.equals(this.languages, querySuggestionsConfigurationWithIndex.languages) &&
Objects.equals(this.exclude, querySuggestionsConfigurationWithIndex.exclude) &&
Objects.equals(this.enablePersonalization, querySuggestionsConfigurationWithIndex.enablePersonalization) &&
Objects.equals(this.allowSpecialCharacters, querySuggestionsConfigurationWithIndex.allowSpecialCharacters)
);
}
@Override
public int hashCode() {
return Objects.hash(indexName, sourceIndices, languages, exclude, enablePersonalization, allowSpecialCharacters);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class QuerySuggestionsConfigurationWithIndex {\n");
sb.append(" indexName: ").append(toIndentedString(indexName)).append("\n");
sb.append(" sourceIndices: ").append(toIndentedString(sourceIndices)).append("\n");
sb.append(" languages: ").append(toIndentedString(languages)).append("\n");
sb.append(" exclude: ").append(toIndentedString(exclude)).append("\n");
sb.append(" enablePersonalization: ").append(toIndentedString(enablePersonalization)).append("\n");
sb.append(" allowSpecialCharacters: ").append(toIndentedString(allowSpecialCharacters)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy