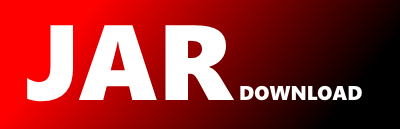
com.algolia.model.recommend.ConsequenceQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.recommend;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.algolia.utils.CompoundType;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import java.io.IOException;
import java.util.logging.Logger;
/**
* When providing a string, it replaces the entire query string. When providing an object, it
* describes incremental edits to be made to the query string (but you can't do both).
*/
@JsonDeserialize(using = ConsequenceQuery.Deserializer.class)
@JsonSerialize(using = ConsequenceQuery.Serializer.class)
public interface ConsequenceQuery extends CompoundType {
static ConsequenceQuery of(ConsequenceQueryObject inside) {
return new ConsequenceQueryConsequenceQueryObject(inside);
}
static ConsequenceQuery of(String inside) {
return new ConsequenceQueryString(inside);
}
class Serializer extends StdSerializer {
public Serializer(Class t) {
super(t);
}
public Serializer() {
this(null);
}
@Override
public void serialize(ConsequenceQuery value, JsonGenerator jgen, SerializerProvider provider) throws IOException {
jgen.writeObject(value.get());
}
}
class Deserializer extends StdDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
public Deserializer() {
this(ConsequenceQuery.class);
}
public Deserializer(Class> vc) {
super(vc);
}
@Override
public ConsequenceQuery deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize ConsequenceQueryObject
if (tree.isObject()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
ConsequenceQueryObject value = parser.readValueAs(new TypeReference() {});
return ConsequenceQuery.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest(
"Failed to deserialize oneOf ConsequenceQueryObject (error: " + e.getMessage() + ") (type: ConsequenceQueryObject)"
);
}
}
// deserialize String
if (tree.isValueNode()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
String value = parser.readValueAs(new TypeReference() {});
return ConsequenceQuery.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf String (error: " + e.getMessage() + ") (type: String)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public ConsequenceQuery getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "ConsequenceQuery cannot be null");
}
}
}
class ConsequenceQueryConsequenceQueryObject implements ConsequenceQuery {
private final ConsequenceQueryObject value;
ConsequenceQueryConsequenceQueryObject(ConsequenceQueryObject value) {
this.value = value;
}
@Override
public ConsequenceQueryObject get() {
return value;
}
}
class ConsequenceQueryString implements ConsequenceQuery {
private final String value;
ConsequenceQueryString(String value) {
this.value = value;
}
@Override
public String get() {
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy