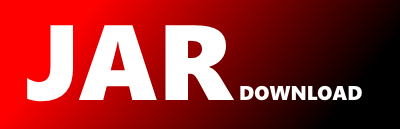
com.algolia.model.recommend.SnippetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.recommend;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.algolia.utils.CompoundType;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import java.io.IOException;
import java.util.List;
import java.util.logging.Logger;
/** SnippetResult */
@JsonDeserialize(using = SnippetResult.Deserializer.class)
@JsonSerialize(using = SnippetResult.Serializer.class)
public interface SnippetResult extends CompoundType {
static SnippetResult> of(List inside) {
return new SnippetResultListOfSnippetResultOption(inside);
}
static SnippetResult of(SnippetResultOption inside) {
return new SnippetResultSnippetResultOption(inside);
}
class Serializer extends StdSerializer {
public Serializer(Class t) {
super(t);
}
public Serializer() {
this(null);
}
@Override
public void serialize(SnippetResult value, JsonGenerator jgen, SerializerProvider provider) throws IOException {
jgen.writeObject(value.get());
}
}
class Deserializer extends StdDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
public Deserializer() {
this(SnippetResult.class);
}
public Deserializer(Class> vc) {
super(vc);
}
@Override
public SnippetResult deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize List
if (tree.isArray()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
List value = parser.readValueAs(new TypeReference>() {});
return SnippetResult.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest(
"Failed to deserialize oneOf List (error: " + e.getMessage() + ") (type: List)"
);
}
}
// deserialize SnippetResultOption
if (tree.isObject()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
SnippetResultOption value = parser.readValueAs(new TypeReference() {});
return SnippetResult.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf SnippetResultOption (error: " + e.getMessage() + ") (type: SnippetResultOption)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public SnippetResult getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "SnippetResult cannot be null");
}
}
}
class SnippetResultListOfSnippetResultOption implements SnippetResult> {
private final List value;
SnippetResultListOfSnippetResultOption(List value) {
this.value = value;
}
@Override
public List get() {
return value;
}
}
class SnippetResultSnippetResultOption implements SnippetResult {
private final SnippetResultOption value;
SnippetResultSnippetResultOption(SnippetResultOption value) {
this.value = value;
}
@Override
public SnippetResultOption get() {
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy