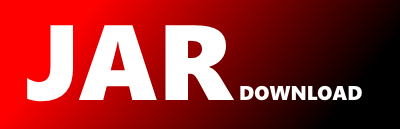
com.algolia.model.search.AroundRadius Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.algolia.utils.CompoundType;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import java.io.IOException;
import java.util.logging.Logger;
/**
* [Maximum
* radius](https://www.algolia.com/doc/guides/managing-results/refine-results/geolocation/#increase-the-search-radius)
* for a geographical search (in meters).
*/
@JsonDeserialize(using = AroundRadius.Deserializer.class)
@JsonSerialize(using = AroundRadius.Serializer.class)
public interface AroundRadius extends CompoundType {
static AroundRadius of(AroundRadiusAll inside) {
return new AroundRadiusAroundRadiusAll(inside);
}
static AroundRadius of(Integer inside) {
return new AroundRadiusInteger(inside);
}
class Serializer extends StdSerializer {
public Serializer(Class t) {
super(t);
}
public Serializer() {
this(null);
}
@Override
public void serialize(AroundRadius value, JsonGenerator jgen, SerializerProvider provider) throws IOException {
jgen.writeObject(value.get());
}
}
class Deserializer extends StdDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
public Deserializer() {
this(AroundRadius.class);
}
public Deserializer(Class> vc) {
super(vc);
}
@Override
public AroundRadius deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize AroundRadiusAll
if (tree.isObject()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
AroundRadiusAll value = parser.readValueAs(new TypeReference() {});
return AroundRadius.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf AroundRadiusAll (error: " + e.getMessage() + ") (type: AroundRadiusAll)");
}
}
// deserialize Integer
if (tree.isValueNode()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
Integer value = parser.readValueAs(new TypeReference() {});
return AroundRadius.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf Integer (error: " + e.getMessage() + ") (type: Integer)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public AroundRadius getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "AroundRadius cannot be null");
}
}
}
class AroundRadiusAroundRadiusAll implements AroundRadius {
private final AroundRadiusAll value;
AroundRadiusAroundRadiusAll(AroundRadiusAll value) {
this.value = value;
}
@Override
public AroundRadiusAll get() {
return value;
}
}
class AroundRadiusInteger implements AroundRadius {
private final Integer value;
AroundRadiusInteger(Integer value) {
this.value = value;
}
@Override
public Integer get() {
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy