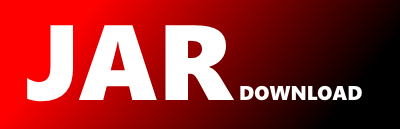
com.algolia.model.search.BrowseParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.algolia.utils.CompoundType;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import java.io.IOException;
import java.util.logging.Logger;
/** BrowseParams */
@JsonDeserialize(using = BrowseParams.Deserializer.class)
@JsonSerialize(using = BrowseParams.Serializer.class)
public interface BrowseParams extends CompoundType {
static BrowseParams of(BrowseParamsObject inside) {
return new BrowseParamsBrowseParamsObject(inside);
}
static BrowseParams of(SearchParamsString inside) {
return new BrowseParamsSearchParamsString(inside);
}
class Serializer extends StdSerializer {
public Serializer(Class t) {
super(t);
}
public Serializer() {
this(null);
}
@Override
public void serialize(BrowseParams value, JsonGenerator jgen, SerializerProvider provider) throws IOException {
jgen.writeObject(value.get());
}
}
class Deserializer extends StdDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
public Deserializer() {
this(BrowseParams.class);
}
public Deserializer(Class> vc) {
super(vc);
}
@Override
public BrowseParams deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize BrowseParamsObject
if (tree.isObject()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
BrowseParamsObject value = parser.readValueAs(new TypeReference() {});
return BrowseParams.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf BrowseParamsObject (error: " + e.getMessage() + ") (type: BrowseParamsObject)");
}
}
// deserialize SearchParamsString
if (tree.isObject()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
SearchParamsString value = parser.readValueAs(new TypeReference() {});
return BrowseParams.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf SearchParamsString (error: " + e.getMessage() + ") (type: SearchParamsString)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public BrowseParams getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "BrowseParams cannot be null");
}
}
}
class BrowseParamsBrowseParamsObject implements BrowseParams {
private final BrowseParamsObject value;
BrowseParamsBrowseParamsObject(BrowseParamsObject value) {
this.value = value;
}
@Override
public BrowseParamsObject get() {
return value;
}
}
class BrowseParamsSearchParamsString implements BrowseParams {
private final SearchParamsString value;
BrowseParamsSearchParamsString(SearchParamsString value) {
this.value = value;
}
@Override
public SearchParamsString get() {
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy