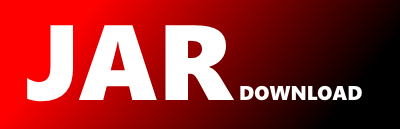
com.algolia.model.search.ConsequenceParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** ConsequenceParams */
public class ConsequenceParams {
@JsonProperty("similarQuery")
private String similarQuery;
@JsonProperty("filters")
private String filters;
@JsonProperty("facetFilters")
private FacetFilters facetFilters;
@JsonProperty("optionalFilters")
private OptionalFilters optionalFilters;
@JsonProperty("numericFilters")
private NumericFilters numericFilters;
@JsonProperty("tagFilters")
private TagFilters tagFilters;
@JsonProperty("sumOrFiltersScores")
private Boolean sumOrFiltersScores;
@JsonProperty("restrictSearchableAttributes")
private List restrictSearchableAttributes;
@JsonProperty("facets")
private List facets;
@JsonProperty("facetingAfterDistinct")
private Boolean facetingAfterDistinct;
@JsonProperty("page")
private Integer page;
@JsonProperty("offset")
private Integer offset;
@JsonProperty("length")
private Integer length;
@JsonProperty("aroundLatLng")
private String aroundLatLng;
@JsonProperty("aroundLatLngViaIP")
private Boolean aroundLatLngViaIP;
@JsonProperty("aroundRadius")
private AroundRadius aroundRadius;
@JsonProperty("aroundPrecision")
private AroundPrecision aroundPrecision;
@JsonProperty("minimumAroundRadius")
private Integer minimumAroundRadius;
@JsonProperty("insideBoundingBox")
private List insideBoundingBox;
@JsonProperty("insidePolygon")
private List insidePolygon;
@JsonProperty("naturalLanguages")
private List naturalLanguages;
@JsonProperty("ruleContexts")
private List ruleContexts;
@JsonProperty("personalizationImpact")
private Integer personalizationImpact;
@JsonProperty("userToken")
private String userToken;
@JsonProperty("getRankingInfo")
private Boolean getRankingInfo;
@JsonProperty("explain")
private List explain;
@JsonProperty("synonyms")
private Boolean synonyms;
@JsonProperty("clickAnalytics")
private Boolean clickAnalytics;
@JsonProperty("analytics")
private Boolean analytics;
@JsonProperty("analyticsTags")
private List analyticsTags;
@JsonProperty("percentileComputation")
private Boolean percentileComputation;
@JsonProperty("enableABTest")
private Boolean enableABTest;
@JsonProperty("attributesForFaceting")
private List attributesForFaceting;
@JsonProperty("attributesToRetrieve")
private List attributesToRetrieve;
@JsonProperty("ranking")
private List ranking;
@JsonProperty("customRanking")
private List customRanking;
@JsonProperty("relevancyStrictness")
private Integer relevancyStrictness;
@JsonProperty("attributesToHighlight")
private List attributesToHighlight;
@JsonProperty("attributesToSnippet")
private List attributesToSnippet;
@JsonProperty("highlightPreTag")
private String highlightPreTag;
@JsonProperty("highlightPostTag")
private String highlightPostTag;
@JsonProperty("snippetEllipsisText")
private String snippetEllipsisText;
@JsonProperty("restrictHighlightAndSnippetArrays")
private Boolean restrictHighlightAndSnippetArrays;
@JsonProperty("hitsPerPage")
private Integer hitsPerPage;
@JsonProperty("minWordSizefor1Typo")
private Integer minWordSizefor1Typo;
@JsonProperty("minWordSizefor2Typos")
private Integer minWordSizefor2Typos;
@JsonProperty("typoTolerance")
private TypoTolerance typoTolerance;
@JsonProperty("allowTyposOnNumericTokens")
private Boolean allowTyposOnNumericTokens;
@JsonProperty("disableTypoToleranceOnAttributes")
private List disableTypoToleranceOnAttributes;
@JsonProperty("ignorePlurals")
private IgnorePlurals ignorePlurals;
@JsonProperty("removeStopWords")
private RemoveStopWords removeStopWords;
@JsonProperty("keepDiacriticsOnCharacters")
private String keepDiacriticsOnCharacters;
@JsonProperty("queryLanguages")
private List queryLanguages;
@JsonProperty("decompoundQuery")
private Boolean decompoundQuery;
@JsonProperty("enableRules")
private Boolean enableRules;
@JsonProperty("enablePersonalization")
private Boolean enablePersonalization;
@JsonProperty("queryType")
private QueryType queryType;
@JsonProperty("removeWordsIfNoResults")
private RemoveWordsIfNoResults removeWordsIfNoResults;
@JsonProperty("mode")
private Mode mode;
@JsonProperty("semanticSearch")
private SemanticSearch semanticSearch;
@JsonProperty("advancedSyntax")
private Boolean advancedSyntax;
@JsonProperty("optionalWords")
private List optionalWords;
@JsonProperty("disableExactOnAttributes")
private List disableExactOnAttributes;
@JsonProperty("exactOnSingleWordQuery")
private ExactOnSingleWordQuery exactOnSingleWordQuery;
@JsonProperty("alternativesAsExact")
private List alternativesAsExact;
@JsonProperty("advancedSyntaxFeatures")
private List advancedSyntaxFeatures;
@JsonProperty("distinct")
private Distinct distinct;
@JsonProperty("attributeForDistinct")
private String attributeForDistinct;
@JsonProperty("replaceSynonymsInHighlight")
private Boolean replaceSynonymsInHighlight;
@JsonProperty("minProximity")
private Integer minProximity;
@JsonProperty("responseFields")
private List responseFields;
@JsonProperty("maxFacetHits")
private Integer maxFacetHits;
@JsonProperty("maxValuesPerFacet")
private Integer maxValuesPerFacet;
@JsonProperty("sortFacetValuesBy")
private String sortFacetValuesBy;
@JsonProperty("attributeCriteriaComputedByMinProximity")
private Boolean attributeCriteriaComputedByMinProximity;
@JsonProperty("renderingContent")
private RenderingContent renderingContent;
@JsonProperty("enableReRanking")
private Boolean enableReRanking;
@JsonProperty("reRankingApplyFilter")
private ReRankingApplyFilter reRankingApplyFilter;
@JsonProperty("query")
private ConsequenceQuery query;
@JsonProperty("automaticFacetFilters")
private AutomaticFacetFilters automaticFacetFilters;
@JsonProperty("automaticOptionalFacetFilters")
private AutomaticFacetFilters automaticOptionalFacetFilters;
public ConsequenceParams setSimilarQuery(String similarQuery) {
this.similarQuery = similarQuery;
return this;
}
/** Overrides the query parameter and performs a more generic search. */
@javax.annotation.Nullable
public String getSimilarQuery() {
return similarQuery;
}
public ConsequenceParams setFilters(String filters) {
this.filters = filters;
return this;
}
/**
* [Filter](https://www.algolia.com/doc/guides/managing-results/refine-results/filtering/) the
* query with numeric, facet, or tag filters.
*/
@javax.annotation.Nullable
public String getFilters() {
return filters;
}
public ConsequenceParams setFacetFilters(FacetFilters facetFilters) {
this.facetFilters = facetFilters;
return this;
}
/** Get facetFilters */
@javax.annotation.Nullable
public FacetFilters getFacetFilters() {
return facetFilters;
}
public ConsequenceParams setOptionalFilters(OptionalFilters optionalFilters) {
this.optionalFilters = optionalFilters;
return this;
}
/** Get optionalFilters */
@javax.annotation.Nullable
public OptionalFilters getOptionalFilters() {
return optionalFilters;
}
public ConsequenceParams setNumericFilters(NumericFilters numericFilters) {
this.numericFilters = numericFilters;
return this;
}
/** Get numericFilters */
@javax.annotation.Nullable
public NumericFilters getNumericFilters() {
return numericFilters;
}
public ConsequenceParams setTagFilters(TagFilters tagFilters) {
this.tagFilters = tagFilters;
return this;
}
/** Get tagFilters */
@javax.annotation.Nullable
public TagFilters getTagFilters() {
return tagFilters;
}
public ConsequenceParams setSumOrFiltersScores(Boolean sumOrFiltersScores) {
this.sumOrFiltersScores = sumOrFiltersScores;
return this;
}
/**
* Determines how to calculate [filter
* scores](https://www.algolia.com/doc/guides/managing-results/refine-results/filtering/in-depth/filter-scoring/#accumulating-scores-with-sumorfiltersscores).
* If `false`, maximum score is kept. If `true`, score is summed.
*/
@javax.annotation.Nullable
public Boolean getSumOrFiltersScores() {
return sumOrFiltersScores;
}
public ConsequenceParams setRestrictSearchableAttributes(List restrictSearchableAttributes) {
this.restrictSearchableAttributes = restrictSearchableAttributes;
return this;
}
public ConsequenceParams addRestrictSearchableAttributes(String restrictSearchableAttributesItem) {
if (this.restrictSearchableAttributes == null) {
this.restrictSearchableAttributes = new ArrayList<>();
}
this.restrictSearchableAttributes.add(restrictSearchableAttributesItem);
return this;
}
/**
* Restricts a query to only look at a subset of your [searchable
* attributes](https://www.algolia.com/doc/guides/managing-results/must-do/searchable-attributes/).
*/
@javax.annotation.Nullable
public List getRestrictSearchableAttributes() {
return restrictSearchableAttributes;
}
public ConsequenceParams setFacets(List facets) {
this.facets = facets;
return this;
}
public ConsequenceParams addFacets(String facetsItem) {
if (this.facets == null) {
this.facets = new ArrayList<>();
}
this.facets.add(facetsItem);
return this;
}
/**
* Returns
* [facets](https://www.algolia.com/doc/guides/managing-results/refine-results/faceting/#contextual-facet-values-and-counts),
* their facet values, and the number of matching facet values.
*/
@javax.annotation.Nullable
public List getFacets() {
return facets;
}
public ConsequenceParams setFacetingAfterDistinct(Boolean facetingAfterDistinct) {
this.facetingAfterDistinct = facetingAfterDistinct;
return this;
}
/**
* Forces faceting to be applied after
* [de-duplication](https://www.algolia.com/doc/guides/managing-results/refine-results/grouping/)
* (with the distinct feature). Alternatively, the `afterDistinct`
* [modifier](https://www.algolia.com/doc/api-reference/api-parameters/attributesForFaceting/#modifiers)
* of `attributesForFaceting` allows for more granular control.
*/
@javax.annotation.Nullable
public Boolean getFacetingAfterDistinct() {
return facetingAfterDistinct;
}
public ConsequenceParams setPage(Integer page) {
this.page = page;
return this;
}
/** Page to retrieve (the first page is `0`, not `1`). */
@javax.annotation.Nullable
public Integer getPage() {
return page;
}
public ConsequenceParams setOffset(Integer offset) {
this.offset = offset;
return this;
}
/**
* Specifies the offset of the first hit to return. > **Note**: Using `page` and `hitsPerPage` is
* the recommended method for [paging
* results](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/pagination/js/).
* However, you can use `offset` and `length` to implement [an alternative approach to
* paging](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/pagination/js/#retrieving-a-subset-of-records-with-offset-and-length).
*/
@javax.annotation.Nullable
public Integer getOffset() {
return offset;
}
public ConsequenceParams setLength(Integer length) {
this.length = length;
return this;
}
/**
* Sets the number of hits to retrieve (for use with `offset`). > **Note**: Using `page` and
* `hitsPerPage` is the recommended method for [paging
* results](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/pagination/js/).
* However, you can use `offset` and `length` to implement [an alternative approach to
* paging](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/pagination/js/#retrieving-a-subset-of-records-with-offset-and-length).
* minimum: 1 maximum: 1000
*/
@javax.annotation.Nullable
public Integer getLength() {
return length;
}
public ConsequenceParams setAroundLatLng(String aroundLatLng) {
this.aroundLatLng = aroundLatLng;
return this;
}
/**
* Search for entries [around a central
* location](https://www.algolia.com/doc/guides/managing-results/refine-results/geolocation/#filter-around-a-central-point),
* enabling a geographical search within a circular area.
*/
@javax.annotation.Nullable
public String getAroundLatLng() {
return aroundLatLng;
}
public ConsequenceParams setAroundLatLngViaIP(Boolean aroundLatLngViaIP) {
this.aroundLatLngViaIP = aroundLatLngViaIP;
return this;
}
/**
* Search for entries around a location. The location is automatically computed from the
* requester's IP address.
*/
@javax.annotation.Nullable
public Boolean getAroundLatLngViaIP() {
return aroundLatLngViaIP;
}
public ConsequenceParams setAroundRadius(AroundRadius aroundRadius) {
this.aroundRadius = aroundRadius;
return this;
}
/** Get aroundRadius */
@javax.annotation.Nullable
public AroundRadius getAroundRadius() {
return aroundRadius;
}
public ConsequenceParams setAroundPrecision(AroundPrecision aroundPrecision) {
this.aroundPrecision = aroundPrecision;
return this;
}
/** Get aroundPrecision */
@javax.annotation.Nullable
public AroundPrecision getAroundPrecision() {
return aroundPrecision;
}
public ConsequenceParams setMinimumAroundRadius(Integer minimumAroundRadius) {
this.minimumAroundRadius = minimumAroundRadius;
return this;
}
/**
* Minimum radius (in meters) used for a geographical search when `aroundRadius` isn't set.
* minimum: 1
*/
@javax.annotation.Nullable
public Integer getMinimumAroundRadius() {
return minimumAroundRadius;
}
public ConsequenceParams setInsideBoundingBox(List insideBoundingBox) {
this.insideBoundingBox = insideBoundingBox;
return this;
}
public ConsequenceParams addInsideBoundingBox(Double insideBoundingBoxItem) {
if (this.insideBoundingBox == null) {
this.insideBoundingBox = new ArrayList<>();
}
this.insideBoundingBox.add(insideBoundingBoxItem);
return this;
}
/**
* Search inside a [rectangular
* area](https://www.algolia.com/doc/guides/managing-results/refine-results/geolocation/#filtering-inside-rectangular-or-polygonal-areas)
* (in geographical coordinates).
*/
@javax.annotation.Nullable
public List getInsideBoundingBox() {
return insideBoundingBox;
}
public ConsequenceParams setInsidePolygon(List insidePolygon) {
this.insidePolygon = insidePolygon;
return this;
}
public ConsequenceParams addInsidePolygon(Double insidePolygonItem) {
if (this.insidePolygon == null) {
this.insidePolygon = new ArrayList<>();
}
this.insidePolygon.add(insidePolygonItem);
return this;
}
/**
* Search inside a
* [polygon](https://www.algolia.com/doc/guides/managing-results/refine-results/geolocation/#filtering-inside-rectangular-or-polygonal-areas)
* (in geographical coordinates).
*/
@javax.annotation.Nullable
public List getInsidePolygon() {
return insidePolygon;
}
public ConsequenceParams setNaturalLanguages(List naturalLanguages) {
this.naturalLanguages = naturalLanguages;
return this;
}
public ConsequenceParams addNaturalLanguages(String naturalLanguagesItem) {
if (this.naturalLanguages == null) {
this.naturalLanguages = new ArrayList<>();
}
this.naturalLanguages.add(naturalLanguagesItem);
return this;
}
/**
* Changes the default values of parameters that work best for a natural language query, such as
* `ignorePlurals`, `removeStopWords`, `removeWordsIfNoResults`, `analyticsTags`, and
* `ruleContexts`. These parameters work well together when the query consists of fuller natural
* language strings instead of keywords, for example when processing voice search queries.
*/
@javax.annotation.Nullable
public List getNaturalLanguages() {
return naturalLanguages;
}
public ConsequenceParams setRuleContexts(List ruleContexts) {
this.ruleContexts = ruleContexts;
return this;
}
public ConsequenceParams addRuleContexts(String ruleContextsItem) {
if (this.ruleContexts == null) {
this.ruleContexts = new ArrayList<>();
}
this.ruleContexts.add(ruleContextsItem);
return this;
}
/**
* Assigns [rule
* contexts](https://www.algolia.com/doc/guides/managing-results/rules/rules-overview/how-to/customize-search-results-by-platform/#whats-a-context)
* to search queries.
*/
@javax.annotation.Nullable
public List getRuleContexts() {
return ruleContexts;
}
public ConsequenceParams setPersonalizationImpact(Integer personalizationImpact) {
this.personalizationImpact = personalizationImpact;
return this;
}
/**
* Defines how much [Personalization affects
* results](https://www.algolia.com/doc/guides/personalization/personalizing-results/in-depth/configuring-personalization/#understanding-personalization-impact).
*/
@javax.annotation.Nullable
public Integer getPersonalizationImpact() {
return personalizationImpact;
}
public ConsequenceParams setUserToken(String userToken) {
this.userToken = userToken;
return this;
}
/**
* Associates a [user
* token](https://www.algolia.com/doc/guides/sending-events/concepts/usertoken/) with the current
* search.
*/
@javax.annotation.Nullable
public String getUserToken() {
return userToken;
}
public ConsequenceParams setGetRankingInfo(Boolean getRankingInfo) {
this.getRankingInfo = getRankingInfo;
return this;
}
/**
* Incidates whether the search response includes [detailed ranking
* information](https://www.algolia.com/doc/guides/building-search-ui/going-further/backend-search/in-depth/understanding-the-api-response/#ranking-information).
*/
@javax.annotation.Nullable
public Boolean getGetRankingInfo() {
return getRankingInfo;
}
public ConsequenceParams setExplain(List explain) {
this.explain = explain;
return this;
}
public ConsequenceParams addExplain(String explainItem) {
if (this.explain == null) {
this.explain = new ArrayList<>();
}
this.explain.add(explainItem);
return this;
}
/** Enriches the API's response with information about how the query was processed. */
@javax.annotation.Nullable
public List getExplain() {
return explain;
}
public ConsequenceParams setSynonyms(Boolean synonyms) {
this.synonyms = synonyms;
return this;
}
/** Whether to take into account an index's synonyms for a particular search. */
@javax.annotation.Nullable
public Boolean getSynonyms() {
return synonyms;
}
public ConsequenceParams setClickAnalytics(Boolean clickAnalytics) {
this.clickAnalytics = clickAnalytics;
return this;
}
/**
* Indicates whether a query ID parameter is included in the search response. This is required for
* [tracking click and conversion
* events](https://www.algolia.com/doc/guides/sending-events/concepts/event-types/#events-related-to-algolia-requests).
*/
@javax.annotation.Nullable
public Boolean getClickAnalytics() {
return clickAnalytics;
}
public ConsequenceParams setAnalytics(Boolean analytics) {
this.analytics = analytics;
return this;
}
/**
* Indicates whether this query will be included in
* [analytics](https://www.algolia.com/doc/guides/search-analytics/guides/exclude-queries/).
*/
@javax.annotation.Nullable
public Boolean getAnalytics() {
return analytics;
}
public ConsequenceParams setAnalyticsTags(List analyticsTags) {
this.analyticsTags = analyticsTags;
return this;
}
public ConsequenceParams addAnalyticsTags(String analyticsTagsItem) {
if (this.analyticsTags == null) {
this.analyticsTags = new ArrayList<>();
}
this.analyticsTags.add(analyticsTagsItem);
return this;
}
/**
* Tags to apply to the query for [segmenting analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
@javax.annotation.Nullable
public List getAnalyticsTags() {
return analyticsTags;
}
public ConsequenceParams setPercentileComputation(Boolean percentileComputation) {
this.percentileComputation = percentileComputation;
return this;
}
/** Whether to include or exclude a query from the processing-time percentile computation. */
@javax.annotation.Nullable
public Boolean getPercentileComputation() {
return percentileComputation;
}
public ConsequenceParams setEnableABTest(Boolean enableABTest) {
this.enableABTest = enableABTest;
return this;
}
/** Incidates whether this search will be considered in A/B testing. */
@javax.annotation.Nullable
public Boolean getEnableABTest() {
return enableABTest;
}
public ConsequenceParams setAttributesForFaceting(List attributesForFaceting) {
this.attributesForFaceting = attributesForFaceting;
return this;
}
public ConsequenceParams addAttributesForFaceting(String attributesForFacetingItem) {
if (this.attributesForFaceting == null) {
this.attributesForFaceting = new ArrayList<>();
}
this.attributesForFaceting.add(attributesForFacetingItem);
return this;
}
/**
* Attributes used for
* [faceting](https://www.algolia.com/doc/guides/managing-results/refine-results/faceting/) and
* the
* [modifiers](https://www.algolia.com/doc/api-reference/api-parameters/attributesForFaceting/#modifiers)
* that can be applied: `filterOnly`, `searchable`, and `afterDistinct`.
*/
@javax.annotation.Nullable
public List getAttributesForFaceting() {
return attributesForFaceting;
}
public ConsequenceParams setAttributesToRetrieve(List attributesToRetrieve) {
this.attributesToRetrieve = attributesToRetrieve;
return this;
}
public ConsequenceParams addAttributesToRetrieve(String attributesToRetrieveItem) {
if (this.attributesToRetrieve == null) {
this.attributesToRetrieve = new ArrayList<>();
}
this.attributesToRetrieve.add(attributesToRetrieveItem);
return this;
}
/**
* Attributes to include in the API response. To reduce the size of your response, you can
* retrieve only some of the attributes. By default, the response includes all attributes.
*/
@javax.annotation.Nullable
public List getAttributesToRetrieve() {
return attributesToRetrieve;
}
public ConsequenceParams setRanking(List ranking) {
this.ranking = ranking;
return this;
}
public ConsequenceParams addRanking(String rankingItem) {
if (this.ranking == null) {
this.ranking = new ArrayList<>();
}
this.ranking.add(rankingItem);
return this;
}
/**
* Determines the order in which Algolia [returns your
* results](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/).
*/
@javax.annotation.Nullable
public List getRanking() {
return ranking;
}
public ConsequenceParams setCustomRanking(List customRanking) {
this.customRanking = customRanking;
return this;
}
public ConsequenceParams addCustomRanking(String customRankingItem) {
if (this.customRanking == null) {
this.customRanking = new ArrayList<>();
}
this.customRanking.add(customRankingItem);
return this;
}
/**
* Specifies the [Custom ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/must-do/custom-ranking/). Use
* the `asc` and `desc` modifiers to specify the ranking order: ascending or descending.
*/
@javax.annotation.Nullable
public List getCustomRanking() {
return customRanking;
}
public ConsequenceParams setRelevancyStrictness(Integer relevancyStrictness) {
this.relevancyStrictness = relevancyStrictness;
return this;
}
/** Relevancy threshold below which less relevant results aren't included in the results. */
@javax.annotation.Nullable
public Integer getRelevancyStrictness() {
return relevancyStrictness;
}
public ConsequenceParams setAttributesToHighlight(List attributesToHighlight) {
this.attributesToHighlight = attributesToHighlight;
return this;
}
public ConsequenceParams addAttributesToHighlight(String attributesToHighlightItem) {
if (this.attributesToHighlight == null) {
this.attributesToHighlight = new ArrayList<>();
}
this.attributesToHighlight.add(attributesToHighlightItem);
return this;
}
/**
* Attributes to highlight. Strings that match the search query in the attributes are highlighted
* by surrounding them with HTML tags (`highlightPreTag` and `highlightPostTag`).
*/
@javax.annotation.Nullable
public List getAttributesToHighlight() {
return attributesToHighlight;
}
public ConsequenceParams setAttributesToSnippet(List attributesToSnippet) {
this.attributesToSnippet = attributesToSnippet;
return this;
}
public ConsequenceParams addAttributesToSnippet(String attributesToSnippetItem) {
if (this.attributesToSnippet == null) {
this.attributesToSnippet = new ArrayList<>();
}
this.attributesToSnippet.add(attributesToSnippetItem);
return this;
}
/**
* Attributes to _snippet_. 'Snippeting' is shortening the attribute to a certain number of words.
* If not specified, the attribute is shortened to the 10 words around the matching string but you
* can specify the number. For example: `body:20`.
*/
@javax.annotation.Nullable
public List getAttributesToSnippet() {
return attributesToSnippet;
}
public ConsequenceParams setHighlightPreTag(String highlightPreTag) {
this.highlightPreTag = highlightPreTag;
return this;
}
/** HTML string to insert before the highlighted parts in all highlight and snippet results. */
@javax.annotation.Nullable
public String getHighlightPreTag() {
return highlightPreTag;
}
public ConsequenceParams setHighlightPostTag(String highlightPostTag) {
this.highlightPostTag = highlightPostTag;
return this;
}
/** HTML string to insert after the highlighted parts in all highlight and snippet results. */
@javax.annotation.Nullable
public String getHighlightPostTag() {
return highlightPostTag;
}
public ConsequenceParams setSnippetEllipsisText(String snippetEllipsisText) {
this.snippetEllipsisText = snippetEllipsisText;
return this;
}
/** String used as an ellipsis indicator when a snippet is truncated. */
@javax.annotation.Nullable
public String getSnippetEllipsisText() {
return snippetEllipsisText;
}
public ConsequenceParams setRestrictHighlightAndSnippetArrays(Boolean restrictHighlightAndSnippetArrays) {
this.restrictHighlightAndSnippetArrays = restrictHighlightAndSnippetArrays;
return this;
}
/** Restrict highlighting and snippeting to items that matched the query. */
@javax.annotation.Nullable
public Boolean getRestrictHighlightAndSnippetArrays() {
return restrictHighlightAndSnippetArrays;
}
public ConsequenceParams setHitsPerPage(Integer hitsPerPage) {
this.hitsPerPage = hitsPerPage;
return this;
}
/** Number of hits per page. minimum: 1 maximum: 1000 */
@javax.annotation.Nullable
public Integer getHitsPerPage() {
return hitsPerPage;
}
public ConsequenceParams setMinWordSizefor1Typo(Integer minWordSizefor1Typo) {
this.minWordSizefor1Typo = minWordSizefor1Typo;
return this;
}
/**
* Minimum number of characters a word in the query string must contain to accept matches with
* [one
* typo](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/in-depth/configuring-typo-tolerance/#configuring-word-length-for-typos).
*/
@javax.annotation.Nullable
public Integer getMinWordSizefor1Typo() {
return minWordSizefor1Typo;
}
public ConsequenceParams setMinWordSizefor2Typos(Integer minWordSizefor2Typos) {
this.minWordSizefor2Typos = minWordSizefor2Typos;
return this;
}
/**
* Minimum number of characters a word in the query string must contain to accept matches with
* [two
* typos](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/in-depth/configuring-typo-tolerance/#configuring-word-length-for-typos).
*/
@javax.annotation.Nullable
public Integer getMinWordSizefor2Typos() {
return minWordSizefor2Typos;
}
public ConsequenceParams setTypoTolerance(TypoTolerance typoTolerance) {
this.typoTolerance = typoTolerance;
return this;
}
/** Get typoTolerance */
@javax.annotation.Nullable
public TypoTolerance getTypoTolerance() {
return typoTolerance;
}
public ConsequenceParams setAllowTyposOnNumericTokens(Boolean allowTyposOnNumericTokens) {
this.allowTyposOnNumericTokens = allowTyposOnNumericTokens;
return this;
}
/** Whether to allow typos on numbers (\"numeric tokens\") in the query string. */
@javax.annotation.Nullable
public Boolean getAllowTyposOnNumericTokens() {
return allowTyposOnNumericTokens;
}
public ConsequenceParams setDisableTypoToleranceOnAttributes(List disableTypoToleranceOnAttributes) {
this.disableTypoToleranceOnAttributes = disableTypoToleranceOnAttributes;
return this;
}
public ConsequenceParams addDisableTypoToleranceOnAttributes(String disableTypoToleranceOnAttributesItem) {
if (this.disableTypoToleranceOnAttributes == null) {
this.disableTypoToleranceOnAttributes = new ArrayList<>();
}
this.disableTypoToleranceOnAttributes.add(disableTypoToleranceOnAttributesItem);
return this;
}
/**
* Attributes for which you want to turn off [typo
* tolerance](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/).
*/
@javax.annotation.Nullable
public List getDisableTypoToleranceOnAttributes() {
return disableTypoToleranceOnAttributes;
}
public ConsequenceParams setIgnorePlurals(IgnorePlurals ignorePlurals) {
this.ignorePlurals = ignorePlurals;
return this;
}
/** Get ignorePlurals */
@javax.annotation.Nullable
public IgnorePlurals getIgnorePlurals() {
return ignorePlurals;
}
public ConsequenceParams setRemoveStopWords(RemoveStopWords removeStopWords) {
this.removeStopWords = removeStopWords;
return this;
}
/** Get removeStopWords */
@javax.annotation.Nullable
public RemoveStopWords getRemoveStopWords() {
return removeStopWords;
}
public ConsequenceParams setKeepDiacriticsOnCharacters(String keepDiacriticsOnCharacters) {
this.keepDiacriticsOnCharacters = keepDiacriticsOnCharacters;
return this;
}
/**
* Characters that the engine shouldn't automatically
* [normalize](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/normalization/).
*/
@javax.annotation.Nullable
public String getKeepDiacriticsOnCharacters() {
return keepDiacriticsOnCharacters;
}
public ConsequenceParams setQueryLanguages(List queryLanguages) {
this.queryLanguages = queryLanguages;
return this;
}
public ConsequenceParams addQueryLanguages(String queryLanguagesItem) {
if (this.queryLanguages == null) {
this.queryLanguages = new ArrayList<>();
}
this.queryLanguages.add(queryLanguagesItem);
return this;
}
/**
* Sets your user's search language. This adjusts language-specific settings and features such as
* `ignorePlurals`, `removeStopWords`, and
* [CJK](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/normalization/#normalization-for-logogram-based-languages-cjk)
* word detection.
*/
@javax.annotation.Nullable
public List getQueryLanguages() {
return queryLanguages;
}
public ConsequenceParams setDecompoundQuery(Boolean decompoundQuery) {
this.decompoundQuery = decompoundQuery;
return this;
}
/**
* [Splits compound
* words](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/language-specific-configurations/#splitting-compound-words)
* into their component word parts in the query.
*/
@javax.annotation.Nullable
public Boolean getDecompoundQuery() {
return decompoundQuery;
}
public ConsequenceParams setEnableRules(Boolean enableRules) {
this.enableRules = enableRules;
return this;
}
/**
* Incidates whether
* [Rules](https://www.algolia.com/doc/guides/managing-results/rules/rules-overview/) are enabled.
*/
@javax.annotation.Nullable
public Boolean getEnableRules() {
return enableRules;
}
public ConsequenceParams setEnablePersonalization(Boolean enablePersonalization) {
this.enablePersonalization = enablePersonalization;
return this;
}
/**
* Incidates whether
* [Personalization](https://www.algolia.com/doc/guides/personalization/what-is-personalization/)
* is enabled.
*/
@javax.annotation.Nullable
public Boolean getEnablePersonalization() {
return enablePersonalization;
}
public ConsequenceParams setQueryType(QueryType queryType) {
this.queryType = queryType;
return this;
}
/** Get queryType */
@javax.annotation.Nullable
public QueryType getQueryType() {
return queryType;
}
public ConsequenceParams setRemoveWordsIfNoResults(RemoveWordsIfNoResults removeWordsIfNoResults) {
this.removeWordsIfNoResults = removeWordsIfNoResults;
return this;
}
/** Get removeWordsIfNoResults */
@javax.annotation.Nullable
public RemoveWordsIfNoResults getRemoveWordsIfNoResults() {
return removeWordsIfNoResults;
}
public ConsequenceParams setMode(Mode mode) {
this.mode = mode;
return this;
}
/** Get mode */
@javax.annotation.Nullable
public Mode getMode() {
return mode;
}
public ConsequenceParams setSemanticSearch(SemanticSearch semanticSearch) {
this.semanticSearch = semanticSearch;
return this;
}
/** Get semanticSearch */
@javax.annotation.Nullable
public SemanticSearch getSemanticSearch() {
return semanticSearch;
}
public ConsequenceParams setAdvancedSyntax(Boolean advancedSyntax) {
this.advancedSyntax = advancedSyntax;
return this;
}
/**
* Enables the [advanced query
* syntax](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/#advanced-syntax).
*/
@javax.annotation.Nullable
public Boolean getAdvancedSyntax() {
return advancedSyntax;
}
public ConsequenceParams setOptionalWords(List optionalWords) {
this.optionalWords = optionalWords;
return this;
}
public ConsequenceParams addOptionalWords(String optionalWordsItem) {
if (this.optionalWords == null) {
this.optionalWords = new ArrayList<>();
}
this.optionalWords.add(optionalWordsItem);
return this;
}
/**
* Words which should be considered
* [optional](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/empty-or-insufficient-results/#creating-a-list-of-optional-words)
* when found in a query.
*/
@javax.annotation.Nullable
public List getOptionalWords() {
return optionalWords;
}
public ConsequenceParams setDisableExactOnAttributes(List disableExactOnAttributes) {
this.disableExactOnAttributes = disableExactOnAttributes;
return this;
}
public ConsequenceParams addDisableExactOnAttributes(String disableExactOnAttributesItem) {
if (this.disableExactOnAttributes == null) {
this.disableExactOnAttributes = new ArrayList<>();
}
this.disableExactOnAttributes.add(disableExactOnAttributesItem);
return this;
}
/**
* Attributes for which you want to [turn off the exact ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/in-depth/adjust-exact-settings/#turn-off-exact-for-some-attributes).
*/
@javax.annotation.Nullable
public List getDisableExactOnAttributes() {
return disableExactOnAttributes;
}
public ConsequenceParams setExactOnSingleWordQuery(ExactOnSingleWordQuery exactOnSingleWordQuery) {
this.exactOnSingleWordQuery = exactOnSingleWordQuery;
return this;
}
/** Get exactOnSingleWordQuery */
@javax.annotation.Nullable
public ExactOnSingleWordQuery getExactOnSingleWordQuery() {
return exactOnSingleWordQuery;
}
public ConsequenceParams setAlternativesAsExact(List alternativesAsExact) {
this.alternativesAsExact = alternativesAsExact;
return this;
}
public ConsequenceParams addAlternativesAsExact(AlternativesAsExact alternativesAsExactItem) {
if (this.alternativesAsExact == null) {
this.alternativesAsExact = new ArrayList<>();
}
this.alternativesAsExact.add(alternativesAsExactItem);
return this;
}
/**
* Alternatives that should be considered an exact match by [the exact ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/in-depth/adjust-exact-settings/#turn-off-exact-for-some-attributes).
*/
@javax.annotation.Nullable
public List getAlternativesAsExact() {
return alternativesAsExact;
}
public ConsequenceParams setAdvancedSyntaxFeatures(List advancedSyntaxFeatures) {
this.advancedSyntaxFeatures = advancedSyntaxFeatures;
return this;
}
public ConsequenceParams addAdvancedSyntaxFeatures(AdvancedSyntaxFeatures advancedSyntaxFeaturesItem) {
if (this.advancedSyntaxFeatures == null) {
this.advancedSyntaxFeatures = new ArrayList<>();
}
this.advancedSyntaxFeatures.add(advancedSyntaxFeaturesItem);
return this;
}
/**
* Allows you to specify which advanced syntax features are active when `advancedSyntax` is
* enabled.
*/
@javax.annotation.Nullable
public List getAdvancedSyntaxFeatures() {
return advancedSyntaxFeatures;
}
public ConsequenceParams setDistinct(Distinct distinct) {
this.distinct = distinct;
return this;
}
/** Get distinct */
@javax.annotation.Nullable
public Distinct getDistinct() {
return distinct;
}
public ConsequenceParams setAttributeForDistinct(String attributeForDistinct) {
this.attributeForDistinct = attributeForDistinct;
return this;
}
/**
* Name of the deduplication attribute to be used with Algolia's [_distinct_
* feature](https://www.algolia.com/doc/guides/managing-results/refine-results/grouping/#introducing-algolias-distinct-feature).
*/
@javax.annotation.Nullable
public String getAttributeForDistinct() {
return attributeForDistinct;
}
public ConsequenceParams setReplaceSynonymsInHighlight(Boolean replaceSynonymsInHighlight) {
this.replaceSynonymsInHighlight = replaceSynonymsInHighlight;
return this;
}
/**
* Whether to highlight and snippet the original word that matches the synonym or the synonym
* itself.
*/
@javax.annotation.Nullable
public Boolean getReplaceSynonymsInHighlight() {
return replaceSynonymsInHighlight;
}
public ConsequenceParams setMinProximity(Integer minProximity) {
this.minProximity = minProximity;
return this;
}
/**
* Precision of the [proximity ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/#proximity).
* minimum: 1 maximum: 7
*/
@javax.annotation.Nullable
public Integer getMinProximity() {
return minProximity;
}
public ConsequenceParams setResponseFields(List responseFields) {
this.responseFields = responseFields;
return this;
}
public ConsequenceParams addResponseFields(String responseFieldsItem) {
if (this.responseFields == null) {
this.responseFields = new ArrayList<>();
}
this.responseFields.add(responseFieldsItem);
return this;
}
/** Attributes to include in the API response for search and browse queries. */
@javax.annotation.Nullable
public List getResponseFields() {
return responseFields;
}
public ConsequenceParams setMaxFacetHits(Integer maxFacetHits) {
this.maxFacetHits = maxFacetHits;
return this;
}
/**
* Maximum number of facet hits to return when [searching for facet
* values](https://www.algolia.com/doc/guides/managing-results/refine-results/faceting/#search-for-facet-values).
* maximum: 100
*/
@javax.annotation.Nullable
public Integer getMaxFacetHits() {
return maxFacetHits;
}
public ConsequenceParams setMaxValuesPerFacet(Integer maxValuesPerFacet) {
this.maxValuesPerFacet = maxValuesPerFacet;
return this;
}
/** Maximum number of facet values to return for each facet. */
@javax.annotation.Nullable
public Integer getMaxValuesPerFacet() {
return maxValuesPerFacet;
}
public ConsequenceParams setSortFacetValuesBy(String sortFacetValuesBy) {
this.sortFacetValuesBy = sortFacetValuesBy;
return this;
}
/** Controls how facet values are fetched. */
@javax.annotation.Nullable
public String getSortFacetValuesBy() {
return sortFacetValuesBy;
}
public ConsequenceParams setAttributeCriteriaComputedByMinProximity(Boolean attributeCriteriaComputedByMinProximity) {
this.attributeCriteriaComputedByMinProximity = attributeCriteriaComputedByMinProximity;
return this;
}
/**
* When the [Attribute criterion is ranked above
* Proximity](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/#attribute-and-proximity-combinations)
* in your ranking formula, Proximity is used to select which searchable attribute is matched in
* the Attribute ranking stage.
*/
@javax.annotation.Nullable
public Boolean getAttributeCriteriaComputedByMinProximity() {
return attributeCriteriaComputedByMinProximity;
}
public ConsequenceParams setRenderingContent(RenderingContent renderingContent) {
this.renderingContent = renderingContent;
return this;
}
/** Get renderingContent */
@javax.annotation.Nullable
public RenderingContent getRenderingContent() {
return renderingContent;
}
public ConsequenceParams setEnableReRanking(Boolean enableReRanking) {
this.enableReRanking = enableReRanking;
return this;
}
/**
* Indicates whether this search will use [Dynamic
* Re-Ranking](https://www.algolia.com/doc/guides/algolia-ai/re-ranking/).
*/
@javax.annotation.Nullable
public Boolean getEnableReRanking() {
return enableReRanking;
}
public ConsequenceParams setReRankingApplyFilter(ReRankingApplyFilter reRankingApplyFilter) {
this.reRankingApplyFilter = reRankingApplyFilter;
return this;
}
/** Get reRankingApplyFilter */
@javax.annotation.Nullable
public ReRankingApplyFilter getReRankingApplyFilter() {
return reRankingApplyFilter;
}
public ConsequenceParams setQuery(ConsequenceQuery query) {
this.query = query;
return this;
}
/** Get query */
@javax.annotation.Nullable
public ConsequenceQuery getQuery() {
return query;
}
public ConsequenceParams setAutomaticFacetFilters(AutomaticFacetFilters automaticFacetFilters) {
this.automaticFacetFilters = automaticFacetFilters;
return this;
}
/** Get automaticFacetFilters */
@javax.annotation.Nullable
public AutomaticFacetFilters getAutomaticFacetFilters() {
return automaticFacetFilters;
}
public ConsequenceParams setAutomaticOptionalFacetFilters(AutomaticFacetFilters automaticOptionalFacetFilters) {
this.automaticOptionalFacetFilters = automaticOptionalFacetFilters;
return this;
}
/** Get automaticOptionalFacetFilters */
@javax.annotation.Nullable
public AutomaticFacetFilters getAutomaticOptionalFacetFilters() {
return automaticOptionalFacetFilters;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConsequenceParams consequenceParams = (ConsequenceParams) o;
return (
Objects.equals(this.similarQuery, consequenceParams.similarQuery) &&
Objects.equals(this.filters, consequenceParams.filters) &&
Objects.equals(this.facetFilters, consequenceParams.facetFilters) &&
Objects.equals(this.optionalFilters, consequenceParams.optionalFilters) &&
Objects.equals(this.numericFilters, consequenceParams.numericFilters) &&
Objects.equals(this.tagFilters, consequenceParams.tagFilters) &&
Objects.equals(this.sumOrFiltersScores, consequenceParams.sumOrFiltersScores) &&
Objects.equals(this.restrictSearchableAttributes, consequenceParams.restrictSearchableAttributes) &&
Objects.equals(this.facets, consequenceParams.facets) &&
Objects.equals(this.facetingAfterDistinct, consequenceParams.facetingAfterDistinct) &&
Objects.equals(this.page, consequenceParams.page) &&
Objects.equals(this.offset, consequenceParams.offset) &&
Objects.equals(this.length, consequenceParams.length) &&
Objects.equals(this.aroundLatLng, consequenceParams.aroundLatLng) &&
Objects.equals(this.aroundLatLngViaIP, consequenceParams.aroundLatLngViaIP) &&
Objects.equals(this.aroundRadius, consequenceParams.aroundRadius) &&
Objects.equals(this.aroundPrecision, consequenceParams.aroundPrecision) &&
Objects.equals(this.minimumAroundRadius, consequenceParams.minimumAroundRadius) &&
Objects.equals(this.insideBoundingBox, consequenceParams.insideBoundingBox) &&
Objects.equals(this.insidePolygon, consequenceParams.insidePolygon) &&
Objects.equals(this.naturalLanguages, consequenceParams.naturalLanguages) &&
Objects.equals(this.ruleContexts, consequenceParams.ruleContexts) &&
Objects.equals(this.personalizationImpact, consequenceParams.personalizationImpact) &&
Objects.equals(this.userToken, consequenceParams.userToken) &&
Objects.equals(this.getRankingInfo, consequenceParams.getRankingInfo) &&
Objects.equals(this.explain, consequenceParams.explain) &&
Objects.equals(this.synonyms, consequenceParams.synonyms) &&
Objects.equals(this.clickAnalytics, consequenceParams.clickAnalytics) &&
Objects.equals(this.analytics, consequenceParams.analytics) &&
Objects.equals(this.analyticsTags, consequenceParams.analyticsTags) &&
Objects.equals(this.percentileComputation, consequenceParams.percentileComputation) &&
Objects.equals(this.enableABTest, consequenceParams.enableABTest) &&
Objects.equals(this.attributesForFaceting, consequenceParams.attributesForFaceting) &&
Objects.equals(this.attributesToRetrieve, consequenceParams.attributesToRetrieve) &&
Objects.equals(this.ranking, consequenceParams.ranking) &&
Objects.equals(this.customRanking, consequenceParams.customRanking) &&
Objects.equals(this.relevancyStrictness, consequenceParams.relevancyStrictness) &&
Objects.equals(this.attributesToHighlight, consequenceParams.attributesToHighlight) &&
Objects.equals(this.attributesToSnippet, consequenceParams.attributesToSnippet) &&
Objects.equals(this.highlightPreTag, consequenceParams.highlightPreTag) &&
Objects.equals(this.highlightPostTag, consequenceParams.highlightPostTag) &&
Objects.equals(this.snippetEllipsisText, consequenceParams.snippetEllipsisText) &&
Objects.equals(this.restrictHighlightAndSnippetArrays, consequenceParams.restrictHighlightAndSnippetArrays) &&
Objects.equals(this.hitsPerPage, consequenceParams.hitsPerPage) &&
Objects.equals(this.minWordSizefor1Typo, consequenceParams.minWordSizefor1Typo) &&
Objects.equals(this.minWordSizefor2Typos, consequenceParams.minWordSizefor2Typos) &&
Objects.equals(this.typoTolerance, consequenceParams.typoTolerance) &&
Objects.equals(this.allowTyposOnNumericTokens, consequenceParams.allowTyposOnNumericTokens) &&
Objects.equals(this.disableTypoToleranceOnAttributes, consequenceParams.disableTypoToleranceOnAttributes) &&
Objects.equals(this.ignorePlurals, consequenceParams.ignorePlurals) &&
Objects.equals(this.removeStopWords, consequenceParams.removeStopWords) &&
Objects.equals(this.keepDiacriticsOnCharacters, consequenceParams.keepDiacriticsOnCharacters) &&
Objects.equals(this.queryLanguages, consequenceParams.queryLanguages) &&
Objects.equals(this.decompoundQuery, consequenceParams.decompoundQuery) &&
Objects.equals(this.enableRules, consequenceParams.enableRules) &&
Objects.equals(this.enablePersonalization, consequenceParams.enablePersonalization) &&
Objects.equals(this.queryType, consequenceParams.queryType) &&
Objects.equals(this.removeWordsIfNoResults, consequenceParams.removeWordsIfNoResults) &&
Objects.equals(this.mode, consequenceParams.mode) &&
Objects.equals(this.semanticSearch, consequenceParams.semanticSearch) &&
Objects.equals(this.advancedSyntax, consequenceParams.advancedSyntax) &&
Objects.equals(this.optionalWords, consequenceParams.optionalWords) &&
Objects.equals(this.disableExactOnAttributes, consequenceParams.disableExactOnAttributes) &&
Objects.equals(this.exactOnSingleWordQuery, consequenceParams.exactOnSingleWordQuery) &&
Objects.equals(this.alternativesAsExact, consequenceParams.alternativesAsExact) &&
Objects.equals(this.advancedSyntaxFeatures, consequenceParams.advancedSyntaxFeatures) &&
Objects.equals(this.distinct, consequenceParams.distinct) &&
Objects.equals(this.attributeForDistinct, consequenceParams.attributeForDistinct) &&
Objects.equals(this.replaceSynonymsInHighlight, consequenceParams.replaceSynonymsInHighlight) &&
Objects.equals(this.minProximity, consequenceParams.minProximity) &&
Objects.equals(this.responseFields, consequenceParams.responseFields) &&
Objects.equals(this.maxFacetHits, consequenceParams.maxFacetHits) &&
Objects.equals(this.maxValuesPerFacet, consequenceParams.maxValuesPerFacet) &&
Objects.equals(this.sortFacetValuesBy, consequenceParams.sortFacetValuesBy) &&
Objects.equals(this.attributeCriteriaComputedByMinProximity, consequenceParams.attributeCriteriaComputedByMinProximity) &&
Objects.equals(this.renderingContent, consequenceParams.renderingContent) &&
Objects.equals(this.enableReRanking, consequenceParams.enableReRanking) &&
Objects.equals(this.reRankingApplyFilter, consequenceParams.reRankingApplyFilter) &&
Objects.equals(this.query, consequenceParams.query) &&
Objects.equals(this.automaticFacetFilters, consequenceParams.automaticFacetFilters) &&
Objects.equals(this.automaticOptionalFacetFilters, consequenceParams.automaticOptionalFacetFilters)
);
}
@Override
public int hashCode() {
return Objects.hash(
similarQuery,
filters,
facetFilters,
optionalFilters,
numericFilters,
tagFilters,
sumOrFiltersScores,
restrictSearchableAttributes,
facets,
facetingAfterDistinct,
page,
offset,
length,
aroundLatLng,
aroundLatLngViaIP,
aroundRadius,
aroundPrecision,
minimumAroundRadius,
insideBoundingBox,
insidePolygon,
naturalLanguages,
ruleContexts,
personalizationImpact,
userToken,
getRankingInfo,
explain,
synonyms,
clickAnalytics,
analytics,
analyticsTags,
percentileComputation,
enableABTest,
attributesForFaceting,
attributesToRetrieve,
ranking,
customRanking,
relevancyStrictness,
attributesToHighlight,
attributesToSnippet,
highlightPreTag,
highlightPostTag,
snippetEllipsisText,
restrictHighlightAndSnippetArrays,
hitsPerPage,
minWordSizefor1Typo,
minWordSizefor2Typos,
typoTolerance,
allowTyposOnNumericTokens,
disableTypoToleranceOnAttributes,
ignorePlurals,
removeStopWords,
keepDiacriticsOnCharacters,
queryLanguages,
decompoundQuery,
enableRules,
enablePersonalization,
queryType,
removeWordsIfNoResults,
mode,
semanticSearch,
advancedSyntax,
optionalWords,
disableExactOnAttributes,
exactOnSingleWordQuery,
alternativesAsExact,
advancedSyntaxFeatures,
distinct,
attributeForDistinct,
replaceSynonymsInHighlight,
minProximity,
responseFields,
maxFacetHits,
maxValuesPerFacet,
sortFacetValuesBy,
attributeCriteriaComputedByMinProximity,
renderingContent,
enableReRanking,
reRankingApplyFilter,
query,
automaticFacetFilters,
automaticOptionalFacetFilters
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConsequenceParams {\n");
sb.append(" similarQuery: ").append(toIndentedString(similarQuery)).append("\n");
sb.append(" filters: ").append(toIndentedString(filters)).append("\n");
sb.append(" facetFilters: ").append(toIndentedString(facetFilters)).append("\n");
sb.append(" optionalFilters: ").append(toIndentedString(optionalFilters)).append("\n");
sb.append(" numericFilters: ").append(toIndentedString(numericFilters)).append("\n");
sb.append(" tagFilters: ").append(toIndentedString(tagFilters)).append("\n");
sb.append(" sumOrFiltersScores: ").append(toIndentedString(sumOrFiltersScores)).append("\n");
sb.append(" restrictSearchableAttributes: ").append(toIndentedString(restrictSearchableAttributes)).append("\n");
sb.append(" facets: ").append(toIndentedString(facets)).append("\n");
sb.append(" facetingAfterDistinct: ").append(toIndentedString(facetingAfterDistinct)).append("\n");
sb.append(" page: ").append(toIndentedString(page)).append("\n");
sb.append(" offset: ").append(toIndentedString(offset)).append("\n");
sb.append(" length: ").append(toIndentedString(length)).append("\n");
sb.append(" aroundLatLng: ").append(toIndentedString(aroundLatLng)).append("\n");
sb.append(" aroundLatLngViaIP: ").append(toIndentedString(aroundLatLngViaIP)).append("\n");
sb.append(" aroundRadius: ").append(toIndentedString(aroundRadius)).append("\n");
sb.append(" aroundPrecision: ").append(toIndentedString(aroundPrecision)).append("\n");
sb.append(" minimumAroundRadius: ").append(toIndentedString(minimumAroundRadius)).append("\n");
sb.append(" insideBoundingBox: ").append(toIndentedString(insideBoundingBox)).append("\n");
sb.append(" insidePolygon: ").append(toIndentedString(insidePolygon)).append("\n");
sb.append(" naturalLanguages: ").append(toIndentedString(naturalLanguages)).append("\n");
sb.append(" ruleContexts: ").append(toIndentedString(ruleContexts)).append("\n");
sb.append(" personalizationImpact: ").append(toIndentedString(personalizationImpact)).append("\n");
sb.append(" userToken: ").append(toIndentedString(userToken)).append("\n");
sb.append(" getRankingInfo: ").append(toIndentedString(getRankingInfo)).append("\n");
sb.append(" explain: ").append(toIndentedString(explain)).append("\n");
sb.append(" synonyms: ").append(toIndentedString(synonyms)).append("\n");
sb.append(" clickAnalytics: ").append(toIndentedString(clickAnalytics)).append("\n");
sb.append(" analytics: ").append(toIndentedString(analytics)).append("\n");
sb.append(" analyticsTags: ").append(toIndentedString(analyticsTags)).append("\n");
sb.append(" percentileComputation: ").append(toIndentedString(percentileComputation)).append("\n");
sb.append(" enableABTest: ").append(toIndentedString(enableABTest)).append("\n");
sb.append(" attributesForFaceting: ").append(toIndentedString(attributesForFaceting)).append("\n");
sb.append(" attributesToRetrieve: ").append(toIndentedString(attributesToRetrieve)).append("\n");
sb.append(" ranking: ").append(toIndentedString(ranking)).append("\n");
sb.append(" customRanking: ").append(toIndentedString(customRanking)).append("\n");
sb.append(" relevancyStrictness: ").append(toIndentedString(relevancyStrictness)).append("\n");
sb.append(" attributesToHighlight: ").append(toIndentedString(attributesToHighlight)).append("\n");
sb.append(" attributesToSnippet: ").append(toIndentedString(attributesToSnippet)).append("\n");
sb.append(" highlightPreTag: ").append(toIndentedString(highlightPreTag)).append("\n");
sb.append(" highlightPostTag: ").append(toIndentedString(highlightPostTag)).append("\n");
sb.append(" snippetEllipsisText: ").append(toIndentedString(snippetEllipsisText)).append("\n");
sb.append(" restrictHighlightAndSnippetArrays: ").append(toIndentedString(restrictHighlightAndSnippetArrays)).append("\n");
sb.append(" hitsPerPage: ").append(toIndentedString(hitsPerPage)).append("\n");
sb.append(" minWordSizefor1Typo: ").append(toIndentedString(minWordSizefor1Typo)).append("\n");
sb.append(" minWordSizefor2Typos: ").append(toIndentedString(minWordSizefor2Typos)).append("\n");
sb.append(" typoTolerance: ").append(toIndentedString(typoTolerance)).append("\n");
sb.append(" allowTyposOnNumericTokens: ").append(toIndentedString(allowTyposOnNumericTokens)).append("\n");
sb.append(" disableTypoToleranceOnAttributes: ").append(toIndentedString(disableTypoToleranceOnAttributes)).append("\n");
sb.append(" ignorePlurals: ").append(toIndentedString(ignorePlurals)).append("\n");
sb.append(" removeStopWords: ").append(toIndentedString(removeStopWords)).append("\n");
sb.append(" keepDiacriticsOnCharacters: ").append(toIndentedString(keepDiacriticsOnCharacters)).append("\n");
sb.append(" queryLanguages: ").append(toIndentedString(queryLanguages)).append("\n");
sb.append(" decompoundQuery: ").append(toIndentedString(decompoundQuery)).append("\n");
sb.append(" enableRules: ").append(toIndentedString(enableRules)).append("\n");
sb.append(" enablePersonalization: ").append(toIndentedString(enablePersonalization)).append("\n");
sb.append(" queryType: ").append(toIndentedString(queryType)).append("\n");
sb.append(" removeWordsIfNoResults: ").append(toIndentedString(removeWordsIfNoResults)).append("\n");
sb.append(" mode: ").append(toIndentedString(mode)).append("\n");
sb.append(" semanticSearch: ").append(toIndentedString(semanticSearch)).append("\n");
sb.append(" advancedSyntax: ").append(toIndentedString(advancedSyntax)).append("\n");
sb.append(" optionalWords: ").append(toIndentedString(optionalWords)).append("\n");
sb.append(" disableExactOnAttributes: ").append(toIndentedString(disableExactOnAttributes)).append("\n");
sb.append(" exactOnSingleWordQuery: ").append(toIndentedString(exactOnSingleWordQuery)).append("\n");
sb.append(" alternativesAsExact: ").append(toIndentedString(alternativesAsExact)).append("\n");
sb.append(" advancedSyntaxFeatures: ").append(toIndentedString(advancedSyntaxFeatures)).append("\n");
sb.append(" distinct: ").append(toIndentedString(distinct)).append("\n");
sb.append(" attributeForDistinct: ").append(toIndentedString(attributeForDistinct)).append("\n");
sb.append(" replaceSynonymsInHighlight: ").append(toIndentedString(replaceSynonymsInHighlight)).append("\n");
sb.append(" minProximity: ").append(toIndentedString(minProximity)).append("\n");
sb.append(" responseFields: ").append(toIndentedString(responseFields)).append("\n");
sb.append(" maxFacetHits: ").append(toIndentedString(maxFacetHits)).append("\n");
sb.append(" maxValuesPerFacet: ").append(toIndentedString(maxValuesPerFacet)).append("\n");
sb.append(" sortFacetValuesBy: ").append(toIndentedString(sortFacetValuesBy)).append("\n");
sb
.append(" attributeCriteriaComputedByMinProximity: ")
.append(toIndentedString(attributeCriteriaComputedByMinProximity))
.append("\n");
sb.append(" renderingContent: ").append(toIndentedString(renderingContent)).append("\n");
sb.append(" enableReRanking: ").append(toIndentedString(enableReRanking)).append("\n");
sb.append(" reRankingApplyFilter: ").append(toIndentedString(reRankingApplyFilter)).append("\n");
sb.append(" query: ").append(toIndentedString(query)).append("\n");
sb.append(" automaticFacetFilters: ").append(toIndentedString(automaticFacetFilters)).append("\n");
sb.append(" automaticOptionalFacetFilters: ").append(toIndentedString(automaticOptionalFacetFilters)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy